Introduction: Navigate the Raspberry Pi's Software: Part 2
This lesson is a continuation of your command-line education. As you work with the Raspberry Pi you will undoubtedly be installing new software to learn, try out, and create with. In this lesson, you will learn how to install software packages and how to search and upgrade them. You will also write and run your first program using the CLI!
Step 1: Installing Packages
There are plenty of fun and useful software packages (packages for short) available to use on the Raspberry Pi. To download and install packages on your Raspberry Pi you will primarily use the command apt-get . This command is used to install, remove, and update APT (Advanced Packaging Tool) packages. It is a tool handed down from the OS Debian that Raspbian is built from. This means that if you find a package that works for Debian and the Raspberry Pi's ARM6 architecture it will most likely work for Raspbian.
Throughout your Raspberry Pi adventures, you will download many packages. ImageMagick is a software package that will be used later on in the class so it's the perfect one to begin with.
Before installing a software package, you need to first update the Raspberry Pi's current list of packages that are available to apt-get with apt-get update. Like so:
apt-get update
You will get an error saying "permission denied" and asking if you are root. Why is that? In order to make these kinds of changes to the Raspberry Pi's software, we need the permissions granted only to the superuser root. Luckily, you already know how to act as root while logged in as the user pi by using sudo. User accounts without root permissions will have to enter the root password to execute sudo commands.
sudo apt-get update
This time, the update will execute successfully.
Using sudo before the command.
Update complete.
After updating, you are now ready to install a package. To download ImageMagick use apt-get with the install command plus the name of the package (don't forget sudo!):
sudo apt-get install imagemagick
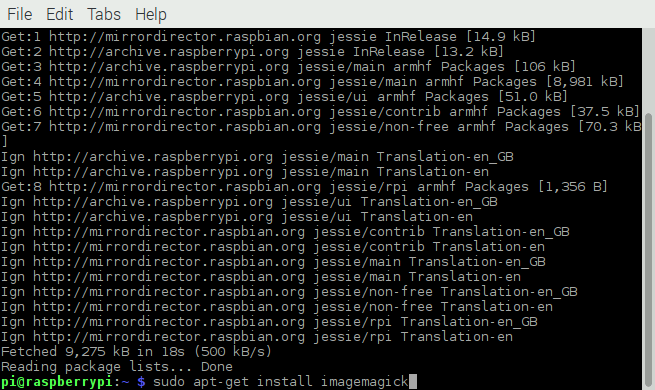
Before the process completes, you will be told how much storage space the application will take up and if you want to continue. Type "y" for yes and then "enter".
Type "y" to continue install.
Install complete.
After using the install command, you will often get asked if you want to continue the install. There's a trick to get around needing to type "y" every time to continue the process. Use the -y flag. This invokes an apt-get option that gives an automatic "yes" to any yes/no prompts that follow the install command. Do you remember how to take a look at a command-line tool's options available for use?
Dependencies
Sometimes when you install packages they need other packages installed to operate. These required files and packages are called dependencies. Later on, you will learn how to look up a package's dependencies.
Step 2: Uninstalling Packages
To uninstall and completely remove a package use the purge command with apt-get. This will uninstall the package and all of its configuration files that came with the install.
sudo apt-get purge packageName
After using purge, use the autoremove command to remove any packages on the Raspberry Pi that aren't needed. This is done because it will remove any dependencies that came with the original install of the package you are purging. For example, if you installed package Z, it might install package X and Y in order for Z to run correctly. When you use purge to uninstall package Z it does not remove packages X and Y. Autoremove does that:
sudo apt-get autoremove
Step 3: How to Create a Text File
Creating and editing text documents is useful or configuring your Raspberry Pi and writing programs. There are command-line text editors just like there are editors for use via the desktop environment like Leaf on the Raspberry Pi and Microsoft Word on Windows. You can write, edit, and save text files using the command-line editor Nano. Nano is a simple text editor that is installed on many Linux distros including Raspbian. It's easy to use and very beginner friendly.
Let's start by opening a new file:
nano
This opens a new buffer which is the same as a blank unnamed text file. In the upper left corner, you will find the name of the application and version number. The name of the file is at top center which by default is called "new buffer". At the bottom of the window, there are three lines. The top line states the status of the file you are editing. Right now, it tells us that the file we are editing is a "New File". The two lines under that are a collection of keyboard shortcuts. The shortcuts you see are the ones most commonly used but there are many more. To see all the shortcuts available plus a description of how to use them, press Ctrl + G. This shortcut brings up the help page. To exit the help page press Ctrl + X.
As stated in the help page, to save a file press Ctrl + O. Alternatively, if you exit Nano using Ctrl + X you will be asked to save the file before exiting.
Step 4: Create a Shell Script!
So far we have been executing single-line commands. Commands can be combined together into one file, saved then ran by the Raspberry Pi executing them all from top to bottom. This is called a shell script. A script is simply a text file that contains multiple commands and is saved with the .sh suffix. You can create a script using any text editor but since you have just discovered Nano, let's stick with it.
Create a new text file in Nano named helloMe by typing:
nano helloMe.sh
For the first line of your program type:
#!/bin/sh
This line is called a shebang. It identifies your text file as a script that Bash needs to execute. If the wrong character appears when you try to type #, revisit your keyboard layout configuration.
For your first shell script, you will write a timelapse script for the camera module. It will automatically take one photo then another every 2 seconds over a total period of 10 seconds.
Write these two lines in your open text file:
raspistill -w 800 -h 600 -t 10000 -tl 2000 -o image%02d.jpg convert -delay 10 -loop 0 image*.jpg animateMe.gif
Let's go over what is happening in these two lines.
By default, the camera takes images with a resolution of 3280 × 2464 pixels at 72 ppi (pixels per inch). This is quite large and consequently, the images will take longer to process. In Raspistill images can be resized by stating the width and the height.
- -w and -h are used to resize the image to 800 x 600 pixels
- -t states the total amount of time the whole process takes in milliseconds
- -tl how often to take a photo
- -o output file name
- image%02d.jpg auto names the photos with image plus two spaces to the right for a generated counter. For example:
- image00.jpg
- image01.jpg
- image02.jpg
If you feel that you may take more than 99 photos you can create 3 or even 4 spaces so you can save thousands of images using image%03d.jpg and image%04d.jpg.
convert is a command from ImageMagick. This line takes all the saved jpegs with the prefix of image and converts them into an animated GIF with a delay (-delay) of 10/100 of a second.
-loop 0 means the GIF will loop forever.
Press Ctrl + X to exit Nano and press "y" to save it these two lines as helloMe.sh.
Step 5: Run a Shell Script
You have your first script but it is not ready to run yet. To run a script simply means to start. Before running a shell script it first needs to be made executable. This is done by using chmod +x in front of the script's name.
chmod +x helloMe.sh
Once made executable, the script is now ready to run. Find a subject to point the camera at (yourself!) and get ready. Remember that by default the camera will display a preview for 5 seconds before taking a photo. This is how much time you will have to prepare before it starts snapping shots.
Run this script by using the sh command before the script's name:
sh helloMe.sh
Alternatively, you can put bash before the name telling the Raspberry Pi to run it using Bash:
bash helloMe.sh
To run a script you need to be in the same directory as where it is saved. If you find you are not in the same directory use cd to navigate there.
Check to see the photos and your GIF saved correctly:
ls
Open the animateMe.gif and check out your time-lapse shot (or double click it in File Manager):
xdg-open animateMe.gif
Step 6: Upgrading Packages
Upgrading a package means updating it to its latest version. To upgrade all the packages on the Raspberry Pi the command upgrade is used. Before you run upgrade, you first need to run apt-get update:
sudo apt-get update
sudo apt-get upgrade
This ensures that any potential dependencies for newly installed software are up to date and can potentially fix any bugs. The upgrade command will take several minutes to finish, and may require your verification/interaction during the process, depending on which packages are being upgraded. Using update and upgrade regularly will keep your Raspberry Pi's OS image up to date. It is essentially the same as downloading the most recent image of Raspbian available.
If you want to upgrade a specific package simply download it again:
sudo apt-get install packageNameUWant2Update
If you already have the newest version of a package APT will tell you in the terminal window that you are "...already running the latest version.."
Step 7: Finding and Searching Packages
There are thousands of packages available to download for Raspbian. To look at the list of available packages go here. To search this cache of software, you use the tool apt-cache. You can use apt-cache with commands to find out other information about a specific package or to see if one exists and what dependencies it needs to operate. Listed below are some useful apt-cache commands. For a complete list visit linux.die.net.
To search the available packages for a keyword, use search plus the keyword you would like to search for:
apt-cache search music
This will result in a list of packages that contain the word "music".
It also gives a short description next to each result which makes it a great way to learn more about a specific package if you already know the name. For example, I saw a package mentioned in an article called amsynth. To get a short description of what it is I can type:
apt-cache search amsynth
For a longer description along with version number, size, homepage, and more use show:
apt-cache show amsynth
To search for a specific name of a package use the command pkgnames. If it is available, it will reveal itself:
apt-cache pkgnames amsynth
If a package has dependencies those will need to be downloaded too. To find the dependencies for a package use the command depends plus the package name.
apt-cache depends amsynth
Step 8: Shutdown + Reboot From CLI
Shutting down and rebooting in LXTerminal is the preferred method for this class as you continue to use the command-line, but you may perform the same functions by using the mouse and taskbar.
As mentioned earlier, the Raspberry Pi's OS must be shut down correctly to avoid any potential damage. To shut down the system involves an orderly termination of all the processes on the system as well as some vital housekeeping chores. There are four commands that can perform this function: halt, poweroff, reboot, and shutdown.
With the shutdown command, you can specify which of the actions to perform (halt, power down, or reboot), and provide a time delay to the shutdown event. Specifying "now" performs the event immediately. To learn more about what each of the four commands do take a look at their man pages.
Shutdown
sudo halt
sudo shutdown -h now
Once you shut down the system the ACT LED on the Raspberry Pi will blink then become steady. Once it is steady remove the power supply.
Reboot
sudo reboot
sudo shutdown -r now
Step 9: Configuring the Raspberry Pi From CLI
Remember when we went through and configured the Raspberry Pi through the desktop GUI? The Raspberry Pi can also be configured through the CLI using the raspi-config command:
sudo raspi-config
At the menu use the up and down arrows keys to move along the options. Use the right arrow to select Finish when you are done and ready to exit. You don't need to set up anything else for this class but now that you are quickly becoming a pro in LXTerminal you can put this in your pocket as a way to configure the Raspberry Pi.
This is an example of how to enable the camera with raspi-config (no need to enable it, you already did in the Get Set Up lesson).
Step 10: Upload a Photo!
Upload one of the pictures taken by running your first shell script (GIFs are not supported at this time, unfortunately).