Introduction: Nerd Pocket Watch
This is my entry for the Remix 2.0 contest. It is based on the Nerd Watch instructable by othermachine. If you like this please vote for me.
I liked the idea of the original instructable, but I have not yet started etching my own printed circuit boards and my old eyes don't see well enough to solder SMD parts. I built this on a Perma Proto board from Adafruit that is designed to fit into a small Altoids tin, and to use all through hole parts.
You will need:
- Small Altoid tin
- Small piece of bubble wrap
- Small Mint Tin Size Breadboard PCB http://www.adafruit.com/products/1214
- Coin Cell Battery Holder https://www.sparkfun.com/products/783
- Coin Cell Battery - 20mm https://www.sparkfun.com/products/338
- Mini Pushbutton Switch https://www.sparkfun.com/products/97
- SPDT Slide Switch http://www.adafruit.com/products/805
- 8 pin IC socket https://www.sparkfun.com/products/7937
- ATtiny85 IC chip https://www.sparkfun.com/products/9378
- Yellow 3mm LED https://www.sparkfun.com/products/532
- Green 3mm LED https://www.sparkfun.com/products/9650
- 2 resistors 330-560 Ohm resistors (See Note)
(Note) This resistor kit from Sparkfun gives you a total of 500 resistors, 25 each of many common sizes:
https://www.sparkfun.com/products/10969
.
You will also need a way to program the ATtiny85 chip the Tiny AVR Programmer
from Sparkfun is a good choice. The directions for installation and use are here:
https://learn.sparkfun.com/tutorials/tiny-avr-programmer-hookup-guide/
There are many other options available to program the chip, just search instructables for "ATtiny".
Step 1: Assembly
Cut a piece of the bubble wrap to fit in the bottom of the Altoids tin. This is to prevent shorts.
Build the circuit according to the schematic.
The numbers on the outside of the box representing the chip are the physical pin numbers, and the digital pin numbers are listed inside the box.
Step 2: The Program
Download this program and put it on your ATtiny85.
You can change the value in the line that says
long calibrate = 313250; // Five minutes.
to make your watch run faster or slower. There is some variation in the ATtiny internal clock. It usually tends to run a little bit fast. The calibration is the value for my particular chip.
/************************************************************ * Digital nerd watch program * * Original program by othermachine on instructables.com * * Re-programmed by JRV31 * ************************************************************/ int greenLED = 1; // Pin for green LED. int yellowLED = 4; // Pin for yellow LED. int button = 0; // Pin for pushbutton switch. int blinkDelay = 350; // Length of blink in milleseconds. long calibrate = 313250; // Five minutes. long starttime; int hour = 0; int minute = 0; /************************************************************ * setup() function - required ************************************************************/ void setup() { pinMode(greenLED, OUTPUT); // Set LED pins to output. pinMode(yellowLED, OUTPUT); pinMode(button, INPUT_PULLUP); // Set button pin to input with pullup digitalWrite(yellowLED, HIGH); // Initial state of LEDs is off. digitalWrite(greenLED, HIGH); settime(); // function to set the time. starttime = millis(); // Mark starting time. while((millis()-starttime)<(calibrate/2)) // Wait 2 1/2 minutes before // advancing time for the first time. { if(digitalRead(button)==LOW) // Displaying time works { // during first 2 1/2 minutes. displaytime(hour); delay(blinkDelay*3); displaytime(minute); } } starttime = millis(); // 2 1/2 minuter have go by. minute++; // Advance the time. if(minute==12) hour++; if(minute>12) { minute = 1; if(hour>12) hour = 1; } } /************************************************************ * loop() function - required ************************************************************/ void loop() { if((millis()-starttime)>calibrate) // Advance time every 5 minutes. { starttime = millis(); minute++; if(minute==12) hour++; if(minute>12) { minute = 1; if(hour>12) hour = 1; } } if(digitalRead(button)==LOW) // Display time if button pressed. { displaytime(hour); delay(blinkDelay*3); displaytime(minute); } } /***************************************************************** * settime() function called by setup() to set the clock at start. *****************************************************************/ void settime() { int i; delay(3000); // Wait three seconds after start. i=0; while(i==0) { digitalWrite(greenLED, LOW); // Green LED blinks, starttime = millis(); while((millis()-starttime)<500) // count the blinks and { if(digitalRead(button)==LOW) i++; // press the button when } digitalWrite(greenLED, HIGH); // count equals hour hand. starttime = millis(); while((millis()-starttime)<500) { if(digitalRead(button)==LOW) i++; } hour++; } delay(3000); // Wait 3 seconds between hour and minute. i=0; while(i==0) { digitalWrite(yellowLED, LOW); // Yellow LED blinks, starttime = millis(); while((millis()-starttime)<500) // count the blinks { if(digitalRead(button)==LOW) i++; // press the button when } digitalWrite(yellowLED, HIGH); // count equals minute hand. starttime = millis(); while((millis()-starttime)<500) { if(digitalRead(button)==LOW) i++; } minute++; } if(hour>11) hour = 11; // Set hour to 11 and minute if(minute>12) minute = 12; if(hour==0) hour = 11; // to 12 if over or zero. if(minute==0) minute =12; while(digitalRead(button)==HIGH); // Wait for button press to start. while(digitalRead(button)==LOW); // debounce } /************************************************************ * displaytime() function displays the time. ************************************************************/ void displaytime(int hhmm) { if(hhmm>7) // If number to display is > 7 { blinker(greenLED); // blink green LED and hhmm-=8; // subtract eight. } else blinker(yellowLED); // else blink yellow LED. if(hhmm>3) { blinker(greenLED); hhmm-=4; } else blinker(yellowLED); if(hhmm>1) { blinker(greenLED); hhmm-=2; } else blinker(yellowLED); if(hhmm==1) { blinker(greenLED); } else blinker(yellowLED); } /************************************************************ * blinker() blinks one LED once. ************************************************************/ void blinker(int LED) { digitalWrite(LED, LOW); // Turn LED on. delay(blinkDelay); digitalWrite(LED, HIGH); // Turn LED off. delay(blinkDelay); }
Attachments
Step 3: Using the Nerd Pocket Watch
Every time you start your Nerd Pocket Watch the first thing you do is set the time.
When you turn it on with the toggle switch there is a short pause then the green LED starts blinking.
Count the blinks and press the pushbutton switch when it has blinked the right number of times needed to represent the hour hand on an analog clock.
Example: If the hour hand should be on six let it blink six times then push the button.
There is another short pause then the yellow LED starts blinking.
Again count the blinks and press the button when it has flashed the number of times for the minute hand.
Press the button one more time and nothing happens. The time is now set and the watch is running.
.
Now every time you press the button it will give you the time in a binary format, first the hour hand and then short pause then the minute hand.
The green light is a one and the yellow light is a zero.
Example: It flashes Yellow Green Green yellow, that is 0110. in binary that is six.
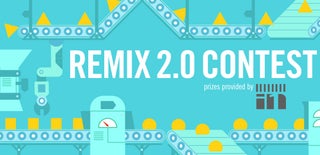
Participated in the
Remix 2.0 Contest