Introduction: RC Car to Robot
Converting an RC car into a robot is a quick and easy way to get started with robotics. At the very least, when you get bored of playing with your remote control car after three days, you can turn it into a robot that will do all of your evil bidding. Well, maybe not all of it, but at least a sizable amount.
In this Instructable I will go over the bare minimum you need to get started. Think of it as a template for making a basic robot that can be expanded with sensors, code, and additional hardware to do some really amazing things. You can now impress your family, make new friends, and scare your pets with the power of robot magic.
Check out my book Homemade Robots for more projects! |
Step 1: Go Get Stuff
You will need:
(x1) R/C Monster Truck
(x1) Arduino Uno REV 3
(x1) Arduino Motor Shield
(x1) Parallax Ping Sensor
(x1) Heavy-Duty 9V Snap Connectors
(x1) DC Barrel Power Plug
(x1) Multipurpose PC Board
(x1) Hookup Wire
(x1) 9 Volt Battery
(x1) 6" Heat-Shrink Tubing
(x1) Zip Ties
(Please note that some of the links on this page contain Amazon affiliate links. This does not change the price of any of the items for sale. However, I earn a small commission if you click on any of those links and buy anything. I reinvest this money into materials and tools for future projects.)
Step 2: Remove the Cover
Flip the RC car over and remove the two screws holding the cover in place.
Put these screws aside somewhere safe for later reassembly.
Step 3: Unplug
Unplug the motors from the connectors on the main board.
If your remote control car is hard wired to the motherboard, cut the motor wires loose.
Step 4: Remove the Controller
Remove the screws holding the controller board to the RC car and pull it free.
Step 5: Power
Remove the cover from the M-type power plug and slide it onto the wires for the 9V connector (such that you can twist it back on after you solder the wires).
Solder the red wire to the middle connection tab. Solder the black wire to the outer connection tab.
Twist the cover back onto the plug.
Step 6: Mark and Drill
Place the Arduino atop the bed of the cargo RC car.
Make marks where the mounting holes are in such a way that it will later be easy to zip tie the Arduino down.
Drill through each of these marks with a 1/8" drill bit.
Step 7: More Marking and Drilling
Place the 9V battery on the underside of the cargo bed. Make two marks on each side of the battery and drill them with a 1/8" drill bit.
Step 8: Attach
Plug the 9V clip to the 9V battery and zip tie it to the underside of the RC car.
Trim away the excess bits of zip tie.
Step 9: Shield
Plug the Arduino Motor Shield into the sockets of the Arduino Uno.
Step 10: Attach
Now that the Motor Shield is attached zip tie the Arduino to the back side of the RC Car.
Step 11: Trim
Using scissors or a paper cutter, trim the PC Board until is skinny enough that it slides neatly between the RC car's front grill.
Once made skinnier, shorten it such that it is just long enough to stick out the front.
Step 12: Solder
Solder the PING sensor centered on the front of the trimmed PC Board.
Connect 8" of green wire to the Signal pin, 8" of red wire to the 5V pin, and 8" of black wire to the Ground pin.
Step 13: Insert
Slide the circuit board into the front grill of the car and make sure the sensor is sitting level.
If it is not level, adjust it until it is.
Step 14: Drill and Fasten
Drill an 1/8" hole on each side of the circuit board and firmly secure it to the body of the RC car with zip ties.
Step 15: Extend
Trim away the connector for one of the motors. Connect an 8" red wire to one and an 8" black wire to the other.
Repeat this process with the second motor.
Finally, slide heat shrink tubing over each of the four exposed solder connections and shrink them into place with a heat gun.
Step 16: Clean Up
Zip tie each set of wires together to keep everything tidy.
You may even want to consider zip tying the sets together into a single bundle for the length of wire that passes over the body of the RC car.
Step 17: Put It Together
Put the body back onto the frame of the RC car and screw it back into place with the screws you set aside earlier.
Step 18: Program
Program the car with the following Arduino code:
<pre>/* RC Car to Robot Conversion by Randy Sarafan Used to convert an RC car into a robot that uses a PING sensor to avoid obstacles, and an Arduino motor shield for motor control. For more information see: https://www.instructables.com/id/RC-Car-to-Robot/ Built atop Ping example code by Tom Igoe */ // this constant won't change. It's the pin number // of the sensor's output: const int pingPin = 7; void setup() { //establish motor direction toggle pins pinMode(12, OUTPUT); //drive motor -- HIGH = forwards and LOW = backwards pinMode(13, OUTPUT); //turn motor -- HIGH = left and LOW = right //establish motor brake pins pinMode(9, OUTPUT); //brake (disable) the drive motor pinMode(8, OUTPUT); //brake (disable) the turn motor //Turns brake off for drive motor digitalWrite(9, LOW); //Turns brake on for turn motor digitalWrite(8, HIGH); //Sets initial speed of drive motor analogWrite(3, 200); //Sets initial direction of drive motor digitalWrite(12, HIGH); } void loop() { // establish variables for duration of the ping, // and the distance result in inches and centimeters: long duration, inches, cm; // The PING))) is triggered by a HIGH pulse of 2 or more microseconds. // Give a short LOW pulse beforehand to ensure a clean HIGH pulse: pinMode(pingPin, OUTPUT); digitalWrite(pingPin, LOW); delayMicroseconds(2); digitalWrite(pingPin, HIGH); delayMicroseconds(5); digitalWrite(pingPin, LOW); // The same pin is used to read the signal from the PING))): a HIGH // pulse whose duration is the time (in microseconds) from the sending // of the ping to the reception of its echo off of an object. pinMode(pingPin, INPUT); duration = pulseIn(pingPin, HIGH); // convert the time into a distance inches = microsecondsToInches(duration); // //if objects are less than 12 inches away //the robot reverses and turns to the right //for 2 seconds // if (inches < 12){ //brake drive motor and pause 1/10 second digitalWrite(9, HIGH); delay(100); // //setting turn motor // //turn off brake for turn motor digitalWrite(8, LOW); //set turn motor direction digitalWrite(13, HIGH); //activate turn motor analogWrite(11, 255); // //setting drive motor // //turn off brake of drive motor digitalWrite(9, LOW); //set drive motor backwards direction digitalWrite(12, LOW); //activate the drive motor analogWrite(3, 200); //backup for 2 seconds delay(2000); // //stopping // //brake both motors digitalWrite(8, HIGH); digitalWrite(9, HIGH); } // //when nothing is within 12" //the robot simply drives forwards // else{ // //Setting drive motor // //set drive motor forward direction digitalWrite(12, HIGH); //turn off brake of drive motor digitalWrite(9, LOW); //activate drive motor analogWrite(3, 200); } delay(100); } long microsecondsToInches(long microseconds) { // According to Parallax's datasheet for the PING))), there are // 73.746 microseconds per inch (i.e. sound travels at 1130 feet per // second). This gives the distance travelled by the ping, outbound // and return, so we divide by 2 to get the distance of the obstacle. // See: http://www.parallax.com/dl/docs/prod/acc/28015-PING-v1.3.pdf return microseconds / 74 / 2; }
For help getting started with the Arduino, check out the Intro to Arduino Instructable.
Attachments
Step 19: Wire It Up
The ping sensor:
- Connect the green wire from the Ping sensor to digital pin 7.
- Connect the black wire from the Ping sensor to ground.
- Connect the red wire to the power input screw socket on the motor shield.
The front turn motor:
- Connect the red wire from the front motor to+ port on channel B of the motor shield.
- Connect the black wire from the front motor to - port on channel B of the motor shield.
The rear drive motor:
- Connect the red wire from the rear motor to the + port on channel A of the motor shield.
- Connect the black wire from the rear motor to - port on channel A of the motor shield.
The motor shield:
- Connect the 5V socket to the power input power screw socket on the motor shield (in addition to the Ping power wire already connected).
- Connect the ground socket on the shield to the input ground screw socket on the motor shield.
Step 20: Go!
Insert the 9V plug into the power socket on the Arduino to power up your robot.
Note: If you decide that you want to reprogram your Arduino, before you plug in the USB cable, disconnect both the 9V battery and the power connection between the Arduino power socket and the motor shield.
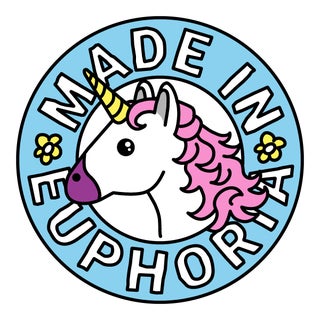
Did you find this useful, fun, or entertaining?
Follow @madeineuphoria to see my latest projects.
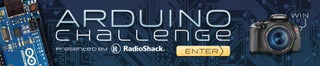
Participated in the
Arduino Challenge
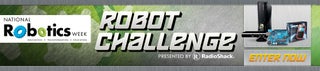
Participated in the
Robot Challenge