Introduction: RGB LED With LinkIt ONE
We'll be learning how to control the colors of an RGB LED using some potentiometers and output from the LinkIt ONE development board. To do this, we'll use three separate potentiometers to control the output of each color in the LED, and using these potentiometers to mix and blend these colors.
Step 1: Materials Needed
1. LinkIt ONE board
2. RGB LED
3. Potentiometers (3)
4. 220 Ohm resistors (3)
5. Breadboard/Protoboard
6. Prototyping/Breadboard wires
7. Preinstalled LinkIt ONE SDK
Step 2: Wiring
First, draw power and ground wires from the LinkIt ONE and connect these to the corresponding power rails on the breadboard that you will be using. This way, we can use central power from a single source.
Connect the LED's anode lead to +5v and connect the 3 RGB cathode leads to digital pins 9, 10, 11 respectively. Use 220 Ohm resistors on each pin of the cathode pins.
Wire up each potentiometer's first pin to +5v, the third pin to ground, and the second pin to analog pins 0, 1, 2. 0 is for Red, 1 is for Green, and 2 is for Blue.
Step 3: Programming
// Init the Pins used for PWM
const int redPin = 9; const int greenPin = 10; const int bluePin = 11;
// Init the Pins used for 10K pots const int redPotPin = 0; const int greenPotPin = 1; const int bluePotPin = 2;
// Init our Vars int currentColorValueRed; int currentColorValueGreen; int currentColorValueBlue;
void setup() { pinMode(redPin, OUTPUT); pinMode(greenPin, OUTPUT); pinMode(bluePin, OUTPUT); }
void loop() { // Read the voltage on each analog pin then scale down to 0-255 and inverting the value for common anode currentColorValueRed = (255 - map( analogRead(redPotPin), 0, 1024, 0, 255 ) ); currentColorValueBlue = (255 - map( analogRead(bluePotPin), 0, 1024, 0, 255 ) ); currentColorValueGreen = (255 - map( analogRead(greenPotPin), 0, 1024, 0, 255 ) );
// Write the color to each pin using PWM and the value gathered above analogWrite(redPin, currentColorValueRed); analogWrite(bluePin, currentColorValueBlue); analogWrite(greenPin, currentColorValueGreen);
}
Attachments
Step 4: Conclusion
Now, trying turning the knobs the potentiometers. The three different colors within the RGB LED should change their intensity when you do this. Try experimenting!
Turn different combinations to get different colors!
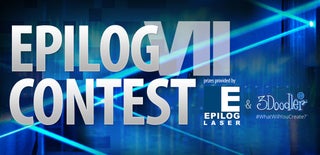
Participated in the
Epilog Contest VII