Introduction: Remote Controlled Arduino Car
I always liked to go to camps, etc where there are a lot of my friends, I always liked having a lot of friends, and one day as I was looking up youtube for remote control cars, and how they were made, I figured out that I could make one for myself from the components I had,
Please vote for this in the Micro-controller Contest --->>>(Thanks in advance)
HERE
All I needed for this make was :
So the total cost for the beginners version is : 69$ with the arduino
And the total cost for the pro version is(Like the one I made with all the optional stuff) : 90$ with the arduino
Here is a video of the whole project, this is the video of the 1st mode of the project, it shows the robot communicating with the computer via USB cable and wirelessly and it shows how the shooter works.
In the code that I will provide there will be only the basic code, which is without the optional parts, if you want the code for any of the other parts, request me in the comments section and Iwill give you the code for that particular part,
In my final version, where the code is quite long, there are 4 modes
1st mode
The robot connects to the computer wirelessly via blue-tooth, and it listens to all the commands, including
(Turn Left, Turn Right, Forward, Target turn Left, Target turn Right, Shoot and more...)
The target stuff is basically, that there is a servo with an LED mounted on it, which acts as a turret as you can see in my other instructable (Star-Wars X-Wing 2)
2nd mode
This is the mode where I use a distance sensor
The distance sensor constantly give in values of how far the things in front are, and if something is close by, then the robot will stop and turn away, or go reverse.
You might like to mount the distance sensor on top of a servo, because that would give you a much wider range.
3rd mode
This is where the sound sensor takes action, When any sound above a certain level is detected as specified in the code, or if a stored tune is herd, then the robot will move accordingly to get away from the sound as much as possible, Its really easy to reverse this so that the robot comes towards you.
4th mode
This one is the line tracking mode, where the robot follows lines, I used a pre-made sensor, but you can find instructions on how to make your own, if you look up Google. In this mode there are another 2 mini modes, one is to follow a line, and the other is to stay inside a line.
One good tip is to make sure that the sensor is placed as close to the ground as possible.
You must be wondering how I switched the modes, well you could do that by using the computer, but I used a 5 way switch for the four modes and one is for off, and for the fourth mode, to switch between the mini modes, I just used a normal switch.
But after all this I realized it was very messy and would be a lot easier and better, if you changed mode from the computer, so I did that later.
There was a huge use of power as I used 4 nine volt batteries, as I had to use two for the two motors, one for the servo and one for the arduino, the servo needed a lot more current than the arduino can provide, so I had to use another power device and add a voltage regulator to it, as the servo could only handle 5V and I thought that adding a lot of 1.5V batteries increases the weight a lot, so I just used my trusty 9 V battery and the voltage regulator.
The motors had to be powered by a separate power supply because, if I connected the motors to the arduino then, there would not be enough power to connect the 3 sensors and the blue-tooth transceiver, and the motors would not go fast if I connected them to the arduino, so I used a transistor for each motor and connected it to the arduino.
And then the last step for me was to make a base for this to make it look cool, so I made a dome sort of thing to cover the entire robot and it looks really cool, you can make any kind of dome you like.
Here is the main code for this robot, but if you want the code for the optional parts then just ask in the comments section :
#include Servo.h
int LedPin1 = 12;
int LedPin = 13;
int MotorPin1 = 9;
int MotorPin2 = 10;
int servoPin = 6;
Servo VishalServo;
void setup()
{
digitalWrite(LedPin, HIGH);
Serial.begin(9600);
Serial.println(Robotic tank);
pinMode(LedPin, OUTPUT);
pinMode(LedPin1, OUTPUT);
pinMode(MotorPin1, OUTPUT);
pinMode(MotorPin2, OUTPUT);
VishalServo.attach(servoPin);
VishalServo.writeMicroseconds(1500);
Serial.println(Wait for 1 second);
delay(1000);
Serial.println(You can now give commands);
}
void loop()
{
while (Serial.available() == 0);
int val = Serial.read() - '0';
int i = VishalServo.read();
if (val == 2)
{
Serial.println(Robot is On);
digitalWrite(MotorPin1, HIGH);
digitalWrite(MotorPin2, HIGH);
delay (500);
digitalWrite(MotorPin1, LOW);
digitalWrite(MotorPin2, LOW);
delay(5);
}
if (val == 1)
{
Serial.println(Robot is turning left);
digitalWrite(MotorPin2, HIGH);
delay(500);
digitalWrite(MotorPin2, LOW);
delay(5);
}
if (val == 3)
{
Serial.println(Robot is turning right);
digitalWrite(MotorPin1, HIGH);
delay(500);
digitalWrite(MotorPin1, LOW);
delay(5);
}
if (val == 9)
{
Serial.println(Turning right);
VishalServo.write(i+5);
delay(5);
}
if (val == 7)
{
Serial.println(Turning left);
if (i = 165)
{
delay(5);
}
if(i = 165)
{
VishalServo.write(i-5);
delay(5);
}
}
if (val == 5)
{
Serial.println(Shoot);
digitalWrite(LedPin1, HIGH);
delay(50);
digitalWrite(LedPin1, LOW);
delay(5);
}
if (val == 8)
{
Serial.println(Centering);
VishalServo.writeMicroseconds(1500);
delay(5);
}
else
{
delay(5);
}
}
This is the code for only the first method as I consider this as the basic / main part of this robot.
If you want the led to stay longer just change the delay between LED pin1 HIGH and LED pin 1 LOW.(Or you might just ask me to do that if you think its too hard or if you are a beginner.
And make sure that the servo is connected to a PWM enabled pin, I used pin 6(PWM means Pulse Width Modulation)
Here is the code you need to use in processing(Its a program that programs the computer)(You can download it for free from the internet, just type in processing and the first link is the one)This code is used to post updates to twitter saying what your robot is doing when you give it commands :
/*************
* based on: http://processing.org/reference/libraries/serial/serialEvent_.html
*************/
import processing.serial.*;
Serial myPort; // The serial port
PFont myFont; // The display font
String inString; // Input string from serial port
int lf = 10; // ASCII linefeed
Twitter twitter; // Twitter
//Going to get oAuth working instead of this, but this will do for now
String username = "YOUR-TWITTER-USERNAME"; // you Twitter Username Here
String password = "YOUR-TWITTER-PASSWORD"; // your Twitter Password Here
void setup() {
size(400,200);
twitter = new Twitter(username,password);
myFont = loadFont("AppleGothic-48.vlw");
textFont(myFont, 18);
println(Serial.list());
myPort = new Serial(this, Serial.list()[0], 9600);
myPort.bufferUntil(lf); //wiat for line feed to specify end of serial buffer
}
void draw() {
background(100);
text("color selected: " + inString, 10,50);
}
void serialEvent(Serial p) {
inString = p.readString();//read serial string
//For some reason this only wanted to work in a try catch
try
{
Status status1 = twitter.updateStatus("Arduino's favorite color is "+inString);//update twitter status
}
catch( TwitterException e) {
println(e.getStatusCode());
}
}
Change username and password with your twitter username and password, I tried it and it works perfectly.
If you want to post updates to an application on twitter go and have a look at my other instructable :
https://www.instructables.com/id/Touch-Me-NotWithout-Ethernet-Sheild/
This is the code for using the ultrasonic range finder instead of a distance sensor, like I did : )
int blinkLed=13; // Where the led will blink
int sensorPin=0; // Analog Pin In
int sum=0; // Variable to calculate SUM
int avgrange=50; // Quantity of values to average
int sensorValue; // Value for te average
int i,media,d; // Variables
float cm,inch; // Converted to cm
void setup()
{
Serial.begin(9600); // To check what is being read on the Serial Port
}
void loop() {
d=analogRead(sensorPin); // Read the analog value
digitalWrite(blinkLed,HIGH); // Turn on LED
delay(d); // Delay changes with the analogread
digitalWrite(13,LOW); // Turn off LED
delay(d); // Another delay
cm = (d / 2) * 2.4; // Convert the value to centimeters
inch = d/2; // Value in inches
Serial.println(cm); //Print average of all measured values
// This is the code if you want to make an average of the read values
/*
for(i = 0; i < avgrange ; i++) {
sum+=analogRead(sensorPin);
delay(10);
}
media = sum/avgrange;
Serial.println(media); //Print average of all measured values
sum=0;
media=0;
*/
}
If you want the schematic for this project, or if you want specific code for different things or if you need help related to this project, then just leave a comment in the comments section, or if you want more details on how to connect any of the below, please ask.
And if you want this to run with commands from remote, all you need to do is use this infrared remote and you can control the whole robot wirelessly with a remote, or if you want it to be more like a remote control car, then you can add an actual remote control car controller or any game-pad for pc, links below...
Infrared Remote :
http://cgi.ebay.com/Arduino-IR-Remote-Control-0038B-Module-DIY-Kit-/280645829263?pt=LH_DefaultDomain_0&hash=item4157cb828f
RC remote :
http://www.amazon.com/dp/B0000AW9RE/ref=asc_df_B0000AW9RE1583100?smid=A3O0M985ZCU7ZK&tag=pgmp-440-95-20&linkCode=asn&creative=395105&creativeASIN=B0000AW9RE
Steering Wheel :
http://www.amazon.com/Genius-Twin-Vibration-F1-Wheel/dp/B000ODVKUC/ref=sr_1_1?s=electronics&ie=UTF8&qid=1308547391&sr=1-1
I made my own dome for this project which fits on top of all the mess and makes the RC Car look like an RC car, but I didn't add any pictures of the dome as I would like you people to make your own personalized domes, and not just copy me...
And of course you can expand upon this project, and if you make something cool share a picture of it by adding a picture while posting a comment, as I would like to see how this instructable has inspired you people to come up with something great.
Links :
Arduino : http://cgi.ebay.com/Arduino-UNO-ATMEGA328P-ATMEGA8U2-FREE-USB-Cable-/320707209315?pt=LH_DefaultDomain_0&hash=item4aaba3a863
LEDs :http://cgi.ebay.com/10-x-3mm-Bright-UV-Purple-5000-mcd-LED-Bulb-Light-/160587866645?pt=LH_DefaultDomain_0&hash=item2563c86215
Motors :http://cgi.ebay.com/Low-Cost-Gear-Motor-2-Pieces-DC-robot-chassis-/280660704676?pt=LH_DefaultDomain_0&hash=item4158ae7da4
Servo :http://cgi.ebay.com/Micro-9g-Servo-RC-Futaba-walkera-Hitec-HS-55-TREX-450-/320711895465?pt=Radio_Control_Parts_Accessories&hash=item4aabeb29a9
Where to download the Arduino IDE : http://arduino.cc/en/Main/Software
Where to download Processing : http://processing.org/download/
I really didn't like the interface of the Arduino as you had to click enter after entering each and every command, so I used putty
Where to download Putty : http://www.chiark.greenend.org.uk/~sgtatham/putty/download.html
Sorry about some blurry pictures... :(
Please vote for this in the Micro-controller Contest --->>>(Thanks in advance)
HERE
All I needed for this make was :
- Arduino(20$)
- Breadboard(3$)
- Some Capacitors(3$)
- Resistors 1K,10K(2$)
- 2 Motors(10$)
- 1 Servo(3$)
- 1 Distance sensor (Optional)(10$)
- 1 Sound sensor (Optional)(5$)
- 1 Line tracking sensor (Optional)(6$)
- LEDs(1$)
- Jumper Cables(2$)
- Voltage Regulators (1 for the servo)(2$)
- Transistors((NPN)For the Motors)(2$)
- 4 pcs 9 V batteries(8$)
- Blue-tooth Transceiver(13$)
So the total cost for the beginners version is : 69$ with the arduino
And the total cost for the pro version is(Like the one I made with all the optional stuff) : 90$ with the arduino
Here is a video of the whole project, this is the video of the 1st mode of the project, it shows the robot communicating with the computer via USB cable and wirelessly and it shows how the shooter works.
In the code that I will provide there will be only the basic code, which is without the optional parts, if you want the code for any of the other parts, request me in the comments section and Iwill give you the code for that particular part,
In my final version, where the code is quite long, there are 4 modes
1st mode
The robot connects to the computer wirelessly via blue-tooth, and it listens to all the commands, including
(Turn Left, Turn Right, Forward, Target turn Left, Target turn Right, Shoot and more...)
The target stuff is basically, that there is a servo with an LED mounted on it, which acts as a turret as you can see in my other instructable (Star-Wars X-Wing 2)
2nd mode
This is the mode where I use a distance sensor
The distance sensor constantly give in values of how far the things in front are, and if something is close by, then the robot will stop and turn away, or go reverse.
You might like to mount the distance sensor on top of a servo, because that would give you a much wider range.
3rd mode
This is where the sound sensor takes action, When any sound above a certain level is detected as specified in the code, or if a stored tune is herd, then the robot will move accordingly to get away from the sound as much as possible, Its really easy to reverse this so that the robot comes towards you.
4th mode
This one is the line tracking mode, where the robot follows lines, I used a pre-made sensor, but you can find instructions on how to make your own, if you look up Google. In this mode there are another 2 mini modes, one is to follow a line, and the other is to stay inside a line.
One good tip is to make sure that the sensor is placed as close to the ground as possible.
You must be wondering how I switched the modes, well you could do that by using the computer, but I used a 5 way switch for the four modes and one is for off, and for the fourth mode, to switch between the mini modes, I just used a normal switch.
But after all this I realized it was very messy and would be a lot easier and better, if you changed mode from the computer, so I did that later.
There was a huge use of power as I used 4 nine volt batteries, as I had to use two for the two motors, one for the servo and one for the arduino, the servo needed a lot more current than the arduino can provide, so I had to use another power device and add a voltage regulator to it, as the servo could only handle 5V and I thought that adding a lot of 1.5V batteries increases the weight a lot, so I just used my trusty 9 V battery and the voltage regulator.
The motors had to be powered by a separate power supply because, if I connected the motors to the arduino then, there would not be enough power to connect the 3 sensors and the blue-tooth transceiver, and the motors would not go fast if I connected them to the arduino, so I used a transistor for each motor and connected it to the arduino.
And then the last step for me was to make a base for this to make it look cool, so I made a dome sort of thing to cover the entire robot and it looks really cool, you can make any kind of dome you like.
Here is the main code for this robot, but if you want the code for the optional parts then just ask in the comments section :
#include Servo.h
int LedPin1 = 12;
int LedPin = 13;
int MotorPin1 = 9;
int MotorPin2 = 10;
int servoPin = 6;
Servo VishalServo;
void setup()
{
digitalWrite(LedPin, HIGH);
Serial.begin(9600);
Serial.println(Robotic tank);
pinMode(LedPin, OUTPUT);
pinMode(LedPin1, OUTPUT);
pinMode(MotorPin1, OUTPUT);
pinMode(MotorPin2, OUTPUT);
VishalServo.attach(servoPin);
VishalServo.writeMicroseconds(1500);
Serial.println(Wait for 1 second);
delay(1000);
Serial.println(You can now give commands);
}
void loop()
{
while (Serial.available() == 0);
int val = Serial.read() - '0';
int i = VishalServo.read();
if (val == 2)
{
Serial.println(Robot is On);
digitalWrite(MotorPin1, HIGH);
digitalWrite(MotorPin2, HIGH);
delay (500);
digitalWrite(MotorPin1, LOW);
digitalWrite(MotorPin2, LOW);
delay(5);
}
if (val == 1)
{
Serial.println(Robot is turning left);
digitalWrite(MotorPin2, HIGH);
delay(500);
digitalWrite(MotorPin2, LOW);
delay(5);
}
if (val == 3)
{
Serial.println(Robot is turning right);
digitalWrite(MotorPin1, HIGH);
delay(500);
digitalWrite(MotorPin1, LOW);
delay(5);
}
if (val == 9)
{
Serial.println(Turning right);
VishalServo.write(i+5);
delay(5);
}
if (val == 7)
{
Serial.println(Turning left);
if (i = 165)
{
delay(5);
}
if(i = 165)
{
VishalServo.write(i-5);
delay(5);
}
}
if (val == 5)
{
Serial.println(Shoot);
digitalWrite(LedPin1, HIGH);
delay(50);
digitalWrite(LedPin1, LOW);
delay(5);
}
if (val == 8)
{
Serial.println(Centering);
VishalServo.writeMicroseconds(1500);
delay(5);
}
else
{
delay(5);
}
}
This is the code for only the first method as I consider this as the basic / main part of this robot.
If you want the led to stay longer just change the delay between LED pin1 HIGH and LED pin 1 LOW.(Or you might just ask me to do that if you think its too hard or if you are a beginner.
And make sure that the servo is connected to a PWM enabled pin, I used pin 6(PWM means Pulse Width Modulation)
Here is the code you need to use in processing(Its a program that programs the computer)(You can download it for free from the internet, just type in processing and the first link is the one)This code is used to post updates to twitter saying what your robot is doing when you give it commands :
/*************
* based on: http://processing.org/reference/libraries/serial/serialEvent_.html
*************/
import processing.serial.*;
Serial myPort; // The serial port
PFont myFont; // The display font
String inString; // Input string from serial port
int lf = 10; // ASCII linefeed
Twitter twitter; // Twitter
//Going to get oAuth working instead of this, but this will do for now
String username = "YOUR-TWITTER-USERNAME"; // you Twitter Username Here
String password = "YOUR-TWITTER-PASSWORD"; // your Twitter Password Here
void setup() {
size(400,200);
twitter = new Twitter(username,password);
myFont = loadFont("AppleGothic-48.vlw");
textFont(myFont, 18);
println(Serial.list());
myPort = new Serial(this, Serial.list()[0], 9600);
myPort.bufferUntil(lf); //wiat for line feed to specify end of serial buffer
}
void draw() {
background(100);
text("color selected: " + inString, 10,50);
}
void serialEvent(Serial p) {
inString = p.readString();//read serial string
//For some reason this only wanted to work in a try catch
try
{
Status status1 = twitter.updateStatus("Arduino's favorite color is "+inString);//update twitter status
}
catch( TwitterException e) {
println(e.getStatusCode());
}
}
Change username and password with your twitter username and password, I tried it and it works perfectly.
If you want to post updates to an application on twitter go and have a look at my other instructable :
https://www.instructables.com/id/Touch-Me-NotWithout-Ethernet-Sheild/
This is the code for using the ultrasonic range finder instead of a distance sensor, like I did : )
int blinkLed=13; // Where the led will blink
int sensorPin=0; // Analog Pin In
int sum=0; // Variable to calculate SUM
int avgrange=50; // Quantity of values to average
int sensorValue; // Value for te average
int i,media,d; // Variables
float cm,inch; // Converted to cm
void setup()
{
Serial.begin(9600); // To check what is being read on the Serial Port
}
void loop() {
d=analogRead(sensorPin); // Read the analog value
digitalWrite(blinkLed,HIGH); // Turn on LED
delay(d); // Delay changes with the analogread
digitalWrite(13,LOW); // Turn off LED
delay(d); // Another delay
cm = (d / 2) * 2.4; // Convert the value to centimeters
inch = d/2; // Value in inches
Serial.println(cm); //Print average of all measured values
// This is the code if you want to make an average of the read values
/*
for(i = 0; i < avgrange ; i++) {
sum+=analogRead(sensorPin);
delay(10);
}
media = sum/avgrange;
Serial.println(media); //Print average of all measured values
sum=0;
media=0;
*/
}
If you want the schematic for this project, or if you want specific code for different things or if you need help related to this project, then just leave a comment in the comments section, or if you want more details on how to connect any of the below, please ask.
And if you want this to run with commands from remote, all you need to do is use this infrared remote and you can control the whole robot wirelessly with a remote, or if you want it to be more like a remote control car, then you can add an actual remote control car controller or any game-pad for pc, links below...
Infrared Remote :
http://cgi.ebay.com/Arduino-IR-Remote-Control-0038B-Module-DIY-Kit-/280645829263?pt=LH_DefaultDomain_0&hash=item4157cb828f
RC remote :
http://www.amazon.com/dp/B0000AW9RE/ref=asc_df_B0000AW9RE1583100?smid=A3O0M985ZCU7ZK&tag=pgmp-440-95-20&linkCode=asn&creative=395105&creativeASIN=B0000AW9RE
Steering Wheel :
http://www.amazon.com/Genius-Twin-Vibration-F1-Wheel/dp/B000ODVKUC/ref=sr_1_1?s=electronics&ie=UTF8&qid=1308547391&sr=1-1
I made my own dome for this project which fits on top of all the mess and makes the RC Car look like an RC car, but I didn't add any pictures of the dome as I would like you people to make your own personalized domes, and not just copy me...
And of course you can expand upon this project, and if you make something cool share a picture of it by adding a picture while posting a comment, as I would like to see how this instructable has inspired you people to come up with something great.
Links :
Arduino : http://cgi.ebay.com/Arduino-UNO-ATMEGA328P-ATMEGA8U2-FREE-USB-Cable-/320707209315?pt=LH_DefaultDomain_0&hash=item4aaba3a863
LEDs :http://cgi.ebay.com/10-x-3mm-Bright-UV-Purple-5000-mcd-LED-Bulb-Light-/160587866645?pt=LH_DefaultDomain_0&hash=item2563c86215
Motors :http://cgi.ebay.com/Low-Cost-Gear-Motor-2-Pieces-DC-robot-chassis-/280660704676?pt=LH_DefaultDomain_0&hash=item4158ae7da4
Servo :http://cgi.ebay.com/Micro-9g-Servo-RC-Futaba-walkera-Hitec-HS-55-TREX-450-/320711895465?pt=Radio_Control_Parts_Accessories&hash=item4aabeb29a9
Where to download the Arduino IDE : http://arduino.cc/en/Main/Software
Where to download Processing : http://processing.org/download/
I really didn't like the interface of the Arduino as you had to click enter after entering each and every command, so I used putty
Where to download Putty : http://www.chiark.greenend.org.uk/~sgtatham/putty/download.html
Sorry about some blurry pictures... :(
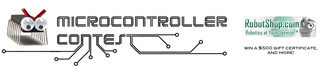
Participated in the
Microcontroller Contest
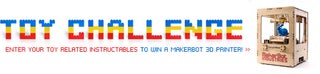
Participated in the
Toy Challenge
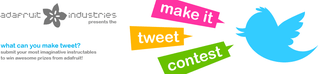
Participated in the
Adafruit Make It Tweet Challenge