Introduction: SYNTHDUINO
The synthduino is a fun arduino project to do and as a beginner it is a great way to learn new things about arduino's many features. With only using household items to build the synthduino you will be satisfied with the finishing project and feel accomplished. the synthduino uses the six potentiometers on the controllers joysticks and triggers and uses the buttons for various functions the code also incorporates a sleep function for the arduino to go to sleep automatically upon start up and for you to wake it up in case of accidental starting. This code incorporates a hello world at the beginning of the code to let you know if the code in on and working.
Step 1: Supply List
I used only household supplies
1. An old controller to game system (I used xbox)
2. 4 resistors ( I just soldered them off of an old rc car)
3. Lots of wire(I used the wire used wires from an old computer)
4. Arduino( I used uno but anything that has the same amount or more pins than uno will work)
5. Speaker or audio adapter
6. Led
7. Power sorce for controller(or you can use arduino’s 5v power )
Tools
1. Drill
2. Soldering iron
3. Solder
4. Hot glue gun
5. Glue sticks
6. Tape
7. Crazy glue
8. Screwdrivers (whatever kind works for you)
Step 2: Take Apart Controller
This step is a short step because I don’t know what controller you are using so this step can take you two minutes to ten minutes but I’d rather not bore you with how I took mine apart
Step 3: Strip a LOT of Wires
You want to strip all of your wires to be able to solder them to the controller and to the pin inputs and outputs
Step 4: Solder Like Crazy
1. You want to solder four of the controllers buttons that are relatively close to each other these will be the tone buttons
• First locate the buttons
• find the type… if it’s a push button then solder it to just one of the four pins on the button… if it is not find the little circle next to the button and solder your wire there
• cut the rubber button pressers to fit around the wires make sure the rubber lines up with the black circles on the controller board
2. Next you want to solder the triggers and joysticks(potentiometers)
• These are easier you just locate the axis slider and solder to the middle pin on all of them
3. Last you want to solder the wake up and melody buttons you can choose where to put those
• First locate the buttons
• find the type… if it’s a push button then solder it to just one of the four pins on the button… if it is not find the little circle next to the button and solder your wire there
• cut the rubber button pressers to fit around the wires make sure the rubber lines up with the black circles on the controller board
4. on the two joysticks are two push buttons those will be used for this project so don't forget to solder them
Step 5: Speaker, Wake Up, Touch Keys
Speaker: the speaker will need to be connected to a resistor before connected to arduino an 8 ohm speaker takes a 100 ohm resistor
Wake up: the wake up function uses pin two and pin 1 aka the RX pin so the rx pin will need to connect to pin 2 with a 220 ohm resistor and pin 2 to a button
Touch keys: the two touch keys will have to have 100 ohm resistors connected to it before connected to arduino also after being connected to pin 0 or TX.
Making touch keys
1. Cut aluminum foil or copper paper squares
2. Glue them to controller
3. Cut two smaller pieces
4. Glue the wire on top of the big pieces using the smaller ones
5. Drill a hole above each key
6. Feed wire through hole
7. From the TX pin have two wires that go to two separate resistors then to the key wires
8. Connect key wires to pins
Step 6: Connect
Drill holes where need be and feed the wires through
Then connect all of the wires to the appropriate pin on arduino
0 two wires in to two resistors
1. Wire with resistor
2. Wire from button to wake up arduino
3. Speaker wire
4. Button on joystick one
5. Button on joystick two
6. Melody button
7. Touch key one
8. Touch key two
9. Tone 1
10. Tone 2
11. Tone 3
12. Tone 4
13. Led
14. Ground for speaker
Analog pins
0. Joystick1 axis1
1. Joystick 1 axis2
2. Trigger 1
3. Joystick 2 axis 1
4. Trigger 2
5. Joystick 2 axis 2
Extra pins
0. Ground pins for anything grounded
1. 5v out pin to the controllers power in (red and black wires)
Step 7: Code
//this code is owned by kevin cross as a whole but i am not the owner the the individual unedited parts of the code//
//the synthduino//
void setup(){
setupSleep();
setuphello();
setuphello1();
setupRun();
setupTone();
setupMelody();
setupcap();
}
void loop(){
loopSleep();
loophello();
loophello1();
loopRun();
loopTone();
loopMelody();
loopcap();
}
/* Sleep Demo Serial
* -----------------
* Example code to demonstrate the sleep functions in a Arduino. Arduino will wake up
* when new data is received in the serial port USART
* Based on Sleep Demo Serial from http://www.arduino.cc/playground/Learning/ArduinoSleepCode
*
* Copyright (C) 2006 MacSimski 2006-12-30
* Copyright (C) 2007 D. Cuartielles 2007-07-08 - Mexico DF
*
* With modifications from Ruben Laguna 2008-10-15
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*
*/
#include <avr/power.h>
#include <avr/sleep.h>
int sleepStatus = 0; // variable to store a request for sleep
int count = 0; // counter
void setupSleep()
{
Serial.begin(9600);
}
void sleepNow()
{
/* Now is the time to set the sleep mode. In the Atmega8 datasheet
* http://www.atmel.com/dyn/resources/prod_documents/doc2486.pdf on page 35
* there is a list of sleep modes which explains which clocks and
* wake up sources are available in which sleep modus.
*
* In the avr/sleep.h file, the call names of these sleep modus are to be found:
*
* The 5 different modes are:
* SLEEP_MODE_IDLE -the least power savings
* SLEEP_MODE_ADC
* SLEEP_MODE_PWR_SAVE
* SLEEP_MODE_STANDBY
* SLEEP_MODE_PWR_DOWN -the most power savings
*
* the power reduction management <avr/power.h> is described in
* http://www.nongnu.org/avr-libc/user-manual/group__avr__power.html
*/
set_sleep_mode(SLEEP_MODE_IDLE); // sleep mode is set here
sleep_enable(); // enables the sleep bit in the mcucr register
// so sleep is possible. just a safety pin
power_adc_disable();
power_spi_disable();
power_timer0_disable();
power_timer1_disable();
power_timer2_disable();
power_twi_disable();
sleep_mode(); // here the device is actually put to sleep!!
// THE PROGRAM CONTINUES FROM HERE AFTER WAKING UP
sleep_disable(); // first thing after waking from sleep:
// disable sleep...
power_all_enable();
}
void loopSleep()
{
// display information about the counter
Serial.print("Awake for ");
Serial.print(count);
Serial.println("sec");
count++;
delay(1000);
// waits for a second
// compute the serial input
if (Serial.available()) {
int val = Serial.read();
if (val == 'S') {
Serial.println("Serial: Entering Sleep mode");
delay(100); // this delay is needed, the sleep
//function will provoke a Serial error otherwise!!
count = 0;
sleepNow(); // sleep function called here
}
if (val == 'A') {
Serial.println("Hola Caracola"); // classic dummy message
}
}
// check if it should go asleep because of time
if (count >= 10) {
Serial.println("Timer: Entering Sleep mode");
delay(100); // this delay is needed, the sleep
//function will provoke a Serial error otherwise!!
count = 0;
sleepNow(); // sleep function called here
}
}
//Arduino Sound Hello World
//Created by David Fowler of uCHobby.com
//Define the I/O pin we will use for our sound output
#define SOUNDOUT_PIN 3
void setuphello(void){
//Set the sound out pin to output mode
pinMode(SOUNDOUT_PIN,OUTPUT);
}
void loophello(void){
//Generate sound by toggling the I/O pin High and Low
//Generate a 1KHz tone. set the pin high for 500uS then
//low for 500uS to make the period 1ms or 1KHz.
//Set the pin high and delay for 1/2 a cycle of 1KHz, 500uS.
digitalWrite(SOUNDOUT_PIN,HIGH);
delayMicroseconds(500);
//Set the pin low and delay for 1/2 a cycle of 1KHz, 500uS.
digitalWrite(SOUNDOUT_PIN,LOW);
delayMicroseconds(500);
}
void setuphello1() {
pinMode(13, OUTPUT);
}
void loophello1() {
delay(3000); // 3 сек втик
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // ..
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // ...
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .... H
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // . E
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .
delay(125);
digitalWrite(13, HIGH);
delay(375);
digitalWrite(13, LOW); // . -
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .-.
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .-.. L
delay(125);
digitalWrite(13, LOW); // .
delay(125);
digitalWrite(13, HIGH);
delay(375);
digitalWrite(13, LOW); // . -
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .-.
delay(125);
delay(125);
digitalWrite(13, HIGH);
delay(125);
digitalWrite(13, LOW); // .-.. L
delay(125);
digitalWrite(13, HIGH);
delay(375);
digitalWrite(13, LOW); // -
delay(125);
digitalWrite(13, HIGH);
delay(375);
digitalWrite(13, LOW); // --
delay(125);
digitalWrite(13, HIGH);
delay(375);
digitalWrite(13, LOW); // --- O
delay(3000); // 3 сек втик
}
/// Auduino, the Lo-Fi granular synthesiser
//
// by Peter Knight, Tinker.it http://tinker.it
//
// Help: http://code.google.com/p/tinkerit/wiki/Auduino
// More help: http://groups.google.com/group/auduino
//
// Analog in 0: Grain 1 pitch
// Analog in 1: Grain 2 decay
// Analog in 2: Grain 1 decay
// Analog in 3: Grain 2 pitch
// Analog in 4: Grain 3 pitch
// Analog in 5: Grain 3 decay
//
// Digital 4: Grain repition frequency
// Digital 5: Grain amplitude frequency
//
// Digital 3: Audio out (Digital 11 on ATmega8)
//
// Changelog:
// 19 Nov 2008: Added support for ATmega8 boards
// 21 Mar 2009: Added support for ATmega328 boards
// 7 Apr 2009: Fixed interrupt vector for ATmega328 boards
// 8 Apr 2009: Added support for ATmega1280 boards (Arduino Mega)
#include <avr/io.h>
#include <avr/interrupt.h>
uint16_t syncPhaseAcc;
uint16_t syncPhaseInc;
uint16_t ampPhaseAcc;
uint16_t ampPhaseInc;
uint16_t grainPhaseAcc;
uint16_t grainPhaseInc;
uint16_t grainAmp;
uint8_t grainDecay;
uint16_t grain2PhaseAcc;
uint16_t grain2PhaseInc;
uint16_t grain2Amp;
uint8_t grain2Decay;
uint16_t grain3PhaseAcc;
uint16_t grain3PhaseInc;
uint16_t grain3Amp;
uint8_t grain3Decay;
// Map Analogue channels
#define GRAIN3_FREQ_CONTROL (4)
#define GRAIN3_DECAY_CONTROL (5)
#define GRAIN_FREQ_CONTROL (0)
#define GRAIN_DECAY_CONTROL (2)
#define GRAIN2_FREQ_CONTROL (3)
#define GRAIN2_DECAY_CONTROL (1)
//Map Digital channels
#define SYNC_CONTROL (4)
#define AMP_CONTROL (5)
// Changing these will also requires rewriting audioOn()
#if defined(__AVR_ATmega8__)
//
// On old ATmega8 boards.
// Output is on pin 11
//
#define LED_PIN 13
#define LED_PORT PORTB
#define LED_BIT 5
#define PWM_PIN 11
#define PWM_VALUE OCR2
#define PWM_INTERRUPT TIMER2_OVF_vect
#elif defined(__AVR_ATmega1280__)
//
// On the Arduino Mega
// Output is on pin 3
//
#define LED_PIN 13
#define LED_PORT PORTB
#define LED_BIT 7
#define PWM_PIN 3
#define PWM_VALUE OCR3C
#define PWM_INTERRUPT TIMER3_OVF_vect
#else
//
// For modern ATmega168 and ATmega328 boards
// Output is on pin 3
//
#define PWM_PIN 3
#define PWM_VALUE OCR2B
#define LED_PIN 13
#define LED_PORT PORTB
#define LED_BIT 5
#define PWM_INTERRUPT TIMER2_OVF_vect
#endif
// Smooth logarithmic mapping
//
uint16_t antilogTable[] = {
64830,64132,63441,62757,62081,61413,60751,60097,59449,58809,58176,57549,56929,56316,55709,55109,
54515,53928,53347,52773,52204,51642,51085,50535,49991,49452,48920,48393,47871,47356,46846,46341,
45842,45348,44859,44376,43898,43425,42958,42495,42037,41584,41136,40693,40255,39821,39392,38968,
38548,38133,37722,37316,36914,36516,36123,35734,35349,34968,34591,34219,33850,33486,33125,32768
};
uint16_t mapPhaseInc(uint16_t input) {
return (antilogTable[input & 0x3f]) >> (input >> 6);
}
// Stepped chromatic mapping
//
uint16_t midiTable[] = {
17,18,19,20,22,23,24,26,27,29,31,32,34,36,38,41,43,46,48,51,54,58,61,65,69,73,
77,82,86,92,97,103,109,115,122,129,137,145,154,163,173,183,194,206,218,231,
244,259,274,291,308,326,346,366,388,411,435,461,489,518,549,581,616,652,691,
732,776,822,871,923,978,1036,1097,1163,1232,1305,1383,1465,1552,1644,1742,
1845,1955,2071,2195,2325,2463,2610,2765,2930,3104,3288,3484,3691,3910,4143,
4389,4650,4927,5220,5530,5859,6207,6577,6968,7382,7821,8286,8779,9301,9854,
10440,11060,11718,12415,13153,13935,14764,15642,16572,17557,18601,19708,20879,
22121,23436,24830,26306
};
uint16_t mapMidi(uint16_t input) {
return (midiTable[(1023-input) >> 3]);
}
// Stepped Pentatonic mapping
//
uint16_t pentatonicTable[54] = {
0,19,22,26,29,32,38,43,51,58,65,77,86,103,115,129,154,173,206,231,259,308,346,
411,461,518,616,691,822,923,1036,1232,1383,1644,1845,2071,2463,2765,3288,
3691,4143,4927,5530,6577,7382,8286,9854,11060,13153,14764,16572,19708,22121,26306
};
uint16_t mapPentatonic(uint16_t input) {
uint8_t value = (1023-input) / (1024/53);
return (pentatonicTable[value]);
}
void audioOn() {
#if defined(__AVR_ATmega8__)
// ATmega8 has different registers
TCCR2 = _BV(WGM20) | _BV(COM21) | _BV(CS20);
TIMSK = _BV(TOIE2);
#elif defined(__AVR_ATmega1280__)
TCCR3A = _BV(COM3C1) | _BV(WGM30);
TCCR3B = _BV(CS30);
TIMSK3 = _BV(TOIE3);
#else
// Set up PWM to 31.25kHz, phase accurate
TCCR2A = _BV(COM2B1) | _BV(WGM20);
TCCR2B = _BV(CS20);
TIMSK2 = _BV(TOIE2);
#endif
}
void setupRun() {
pinMode(PWM_PIN,OUTPUT);
audioOn();
pinMode(LED_PIN,OUTPUT);
}
void loopRun() {
// The loop is pretty simple - it just updates the parameters for the oscillators.
//
// Avoid using any functions that make extensive use of interrupts, or turn interrupts off.
// They will cause clicks and poops in the audio.
// Smooth frequency mapping
//syncPhaseInc = mapPhaseInc(digitalRead(SYNC_CONTROL)) / 4;
//ampPhaseInc = mapPhaseInc(digitalRead(AMP_CONTROL)) / 5;
// Stepped mapping to MIDI notes: C, Db, D, Eb, E, F...
//syncPhaseInc = mapMidi(digitalRead(SYNC_CONTROL));
// Stepped pentatonic mapping: D, E, G, A, B
syncPhaseInc = mapPentatonic(digitalRead(SYNC_CONTROL));
grainPhaseInc = mapPhaseInc(analogRead(GRAIN_FREQ_CONTROL)) / 2;
grainDecay = analogRead(GRAIN_DECAY_CONTROL) / 8;
grain2PhaseInc = mapPhaseInc(analogRead(GRAIN2_FREQ_CONTROL)) / 2;
grain2Decay = analogRead(GRAIN2_DECAY_CONTROL) / 4;
grain3PhaseInc = mapPhaseInc(analogRead(GRAIN3_FREQ_CONTROL)) /2;
grain3Decay = analogRead(GRAIN2_DECAY_CONTROL) / 2;
}
SIGNAL(PWM_INTERRUPT)
{
uint8_t value;
uint16_t output;
syncPhaseAcc += syncPhaseInc;
if (syncPhaseAcc < syncPhaseInc) {
// Time to start the next grain
grainPhaseAcc = 0;
grainAmp = 0x7fff;
grain2PhaseAcc = 0;
grain2Amp = 0x7fff;
grain3PhaseAcc = 0;
grain3Amp = 0x7fff;
LED_PORT ^= 1 << LED_BIT; // Faster than using digitalWrite
}
// Increment the phase of the grain oscillators
grainPhaseAcc += grainPhaseInc;
grain2PhaseAcc += grain2PhaseInc;
// Convert phase into a triangle wave
value = (grainPhaseAcc >> 7) & 0xff;
if (grainPhaseAcc & 0x8000) value = ~value;
// Multiply by current grain amplitude to get sample
output = value * (grainAmp >> 8);
// Repeat for second grain
value = (grain2PhaseAcc >> 7) & 0xff;
if (grain2PhaseAcc & 0x8000) value = ~value;
output += value * (grain2Amp >> 8);
// Repeat for third grain
value = (grain3PhaseAcc >> 7) & 0xff;
if (grain3PhaseAcc & 0x8000) value = ~value;
output += value * (grain3Amp >> 8);
// Make the grain amplitudes decay by a factor every sample (exponential decay)
grainAmp -= (grainAmp >> 8) * grainDecay;
grain2Amp -= (grain2Amp >> 8) * grain2Decay;
grain3Amp -= (grain3Amp >> 8) * grain3Decay;
// Scale output to the available range, clipping if necessary
output >>= 9;
if (output > 255) output = 255;
// Output to PWM (this is faster than using analogWrite)
PWM_VALUE = output;
}
/* Heavily based on http://ardx.org/src/circ/CIRC06-code.txt
* and also http://ardx.org/src/circ/CIRC07-code.txt
* Circuit information at http://www.oomlout.com/oom.php/products/ardx/circ-06
* and http://www.oomlout.com/oom.php/products/ardx/circ-07
* may also help
*
* The calculation of the tones is made following the mathematical
* operation:
*
* timeHigh = period / 2 = 1 / (2 * toneFrequency)
*
* where the different tones are described as in the table:
*
* note frequency period timeHigh
* c 261 Hz 3830 1915
* d 294 Hz 3400 1700
* e 329 Hz 3038 1519
* f 349 Hz 2864 1432
* g 392 Hz 2550 1275
* a 440 Hz 2272 1136
* b 493 Hz 2028 1014
* C 523 Hz 1912 956
*
* http://www.arduino.cc/en/Tutorial/Melody
*/
int inputPin1 = 9;
int inputPin2 = 10;
int inputPin3 = 11;
int inputPin4 = 12;
int speakerPin = 3;
int val = 0;
int length = 1; // the number of notes
char notes[] = {
'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C' }; // a space represents a rest
int beats[] = {
1 };
int tempo = 300;
void playTone(int tone, int duration) {
for (long i = 0; i < duration * 1000L; i += tone * 2) {
digitalWrite(speakerPin, HIGH);
delayMicroseconds(tone);
digitalWrite(speakerPin, LOW);
delayMicroseconds(tone);
}
}
void playNote(char note, int duration) {
char names[] = {
'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C' };
int tones[] = {
1915, 1700, 1519, 1432, 1275, 1136, 1014, 956 };
// play the tone corresponding to the note name
for (int i = 0; i < 8; i++) {
if (names[i] == note) {
playTone(tones[i], duration);
}
}
}
void setupTone() {
pinMode(speakerPin, OUTPUT);
pinMode(inputPin1, INPUT);
pinMode(inputPin2, INPUT);
pinMode(inputPin3, INPUT);
pinMode(inputPin4, INPUT);
}
void loopTone() {
if (digitalRead(inputPin1) == HIGH) {
playNote(notes[0], 300);
}
else if (digitalRead(inputPin2) == HIGH) {
playNote(notes[1], 300);
}
else if (digitalRead(inputPin3) == HIGH) {
playNote(notes[2], 300);
}
else if (digitalRead(inputPin4) == HIGH) {
playNote(notes[3], 300);
}
else {
digitalWrite(speakerPin, LOW);
}
}
/* Original code created 21 Jan 2010
/ modified 30 Aug 2011
/ by Tom Igoe (http://pastebin.com/tCYvfky9) and configured by me 2013 (my code below)
/
/ When you firgure out how to connect the arduino and made it play the basic melody. You should start coding
/
/ First of you will need the notes you can copy paste mine or just copy it from http://arduino.cc/en/Tutorial/Tone
/
*/
// we begin with definining all of the notes
#define NOTE_B0 31
#define NOTE_C1 33
#define NOTE_CS1 35
#define NOTE_D1 37
#define NOTE_DS1 39
#define NOTE_E1 41
#define NOTE_F1 44
#define NOTE_FS1 46
#define NOTE_G1 49
#define NOTE_GS1 52
#define NOTE_A1 55
#define NOTE_AS1 58
#define NOTE_B1 62
#define NOTE_C2 65
#define NOTE_CS2 69
#define NOTE_D2 73
#define NOTE_DS2 78
#define NOTE_E2 82
#define NOTE_F2 87
#define NOTE_FS2 93
#define NOTE_G2 98
#define NOTE_GS2 104
#define NOTE_A2 110
#define NOTE_AS2 117
#define NOTE_B2 123
#define NOTE_C3 131
#define NOTE_CS3 139
#define NOTE_D3 147
#define NOTE_DS3 156
#define NOTE_E3 165
#define NOTE_F3 175
#define NOTE_FS3 185
#define NOTE_G3 196
#define NOTE_GS3 208
#define NOTE_A3 220
#define NOTE_AS3 233
#define NOTE_B3 247
#define NOTE_C4 262
#define NOTE_CS4 277
#define NOTE_D4 294
#define NOTE_DS4 311
#define NOTE_E4 330
#define NOTE_F4 349
#define NOTE_FS4 370
#define NOTE_G4 392
#define NOTE_GS4 415
#define NOTE_A4 440
#define NOTE_AS4 466
#define NOTE_B4 494
#define NOTE_C5 523
#define NOTE_CS5 554
#define NOTE_D5 587
#define NOTE_DS5 622
#define NOTE_E5 659
#define NOTE_F5 698
#define NOTE_FS5 740
#define NOTE_G5 784
#define NOTE_GS5 831
#define NOTE_A5 880
#define NOTE_AS5 932
#define NOTE_B5 988
#define NOTE_C6 1047
#define NOTE_CS6 1109
#define NOTE_D6 1175
#define NOTE_DS6 1245
#define NOTE_E6 1319
#define NOTE_F6 1397
#define NOTE_FS6 1480
#define NOTE_G6 1568
#define NOTE_GS6 1661
#define NOTE_A6 1760
#define NOTE_AS6 1865
#define NOTE_B6 1976
#define NOTE_C7 2093
#define NOTE_CS7 2217
#define NOTE_D7 2349
#define NOTE_DS7 2489
#define NOTE_E7 2637
#define NOTE_F7 2794
#define NOTE_FS7 2960
#define NOTE_G7 3136
#define NOTE_GS7 3322
#define NOTE_A7 3520
#define NOTE_AS7 3729
#define NOTE_B7 3951
#define NOTE_C8 4186
#define NOTE_CS8 4435
#define NOTE_D8 4699
#define NOTE_DS8 4978
/*
Button
Turns on and off a light emitting diode(LED) connected to digital
pin 13, when pressing a pushbutton attached to pin 7.
The circuit:
* LED attached from pin 13 to ground
* pushbutton attached to pin 6 from +5V
* 10K resistor attached to pin 6 from ground
* Note: on most Arduinos there is already an LED on the board
attached to pin 13.
created 2005
by DojoDave <http://www.0j0.org>
modified 17 Jun 2009
by Tom Igoe
http://www.arduino.cc/en/Tutorial/Button
*/
// constants won't change. They're used here to
// set pin numbers:
const int buttonPin = 6; // the number of the pushbutton pin
int Buzzer1 = 3;
// variables will change:
int buttonState = 0; // variable for reading the pushbutton status
void setupMelody() {
// initialize the piezo as output:
pinMode(Buzzer1, OUTPUT);
// initialize the pushbutton pin as an input:
pinMode(buttonPin, INPUT);
}
void loopMelody(){
// read the state of the pushbutton value:
buttonState = digitalRead(buttonPin);
// check if the pushbutton is pressed.
// if it is, the buttonState is HIGH:
if (buttonState == HIGH) {
// play th Music
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,450,225);
delay(300);
tone(Buzzer1,450,225);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,450,200);
delay(300);
tone(Buzzer1,600,300);
delay(300);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,700,300);
delay(300);
tone(Buzzer1,700,300);
delay(500);
tone(Buzzer1,600,300);
delay(300);
tone(Buzzer1,400,200);
delay(1000);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,650,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,650,200);
delay(300);
tone(Buzzer1,650,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,400,200);
delay(1000);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,600,300);
delay(500);
tone(Buzzer1,600,300);
delay(500);
tone(Buzzer1,800,300);
delay(500);
tone(Buzzer1,800,300);
delay(500);
tone(Buzzer1,400,200);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,400,200);
delay(500);
tone(Buzzer1,300,150);
delay(500);
tone(Buzzer1,300,150);
delay(500);
tone(Buzzer1,300,150);
delay(500);
tone(Buzzer1,300,150);
delay(500);
tone(Buzzer1,300,150);
tone(Buzzer1,300,150);
tone(Buzzer1,300,150);
tone(Buzzer1,300,150);
}
}
/*
Capacitive-Touch Arduino Keyboard Piano
Plays piano tones through a buzzer when the user taps touch-sensitive piano "keys"
Created 18 May 2013
Modified 23 May 2013
by Tyler Crumpton and Nicholas Jones
This code is released to the public domain. For information about the circuit,
visit the Instructable tutorial at https://www.instructables.com/id/Capacitive-Touch-Arduino-Keyboard-Piano/
*/
#include <CapacitiveSensor.h>
#define COMMON_PIN 0 // The common 'send' pin for all keys
#define BUZZER_PIN 3 // The output pin for the piezo buzzer
#define NUM_OF_SAMPLES 10 // Higher number whens more delay but more consistent readings
#define CAP_THRESHOLD 150 // Capactive reading that triggers a note (adjust to fit your needs)
#define NUM_OF_KEYS 2 // Number of keys that are on the keyboard
// This macro creates a capacitance "key" sensor object for each key on the piano keyboard:
#define CS(Y) CapacitiveSensor(0, Y)
// Each key corresponds to a note, which are defined here. Uncomment the scale that you want to use:
int notes1[]={
NOTE_C4,NOTE_D4,NOTE_E4,NOTE_F4,NOTE_G4,NOTE_A4,NOTE_B4,NOTE_C5}; // C-Major scale
//int notes1[]={NOTE_A4,NOTE_B4,NOTE_C5,NOTE_D5,NOTE_E5,NOTE_F5,NOTE_G5,NOTE_A5}; // A-Minor scale
//int notes1[]={NOTE_C4,NOTE_DS4,NOTE_F4,NOTE_FS4,NOTE_G4,NOTE_AS4,NOTE_C5,NOTE_DS5}; // C Blues scale
// Defines the pins that the keys are connected to:
CapacitiveSensor keys[] = {
CS(7), CS(8)};
void setupcap() {
// Turn off autocalibrate on all channels:
for(int i=0; i<8; ++i) {
keys[i].set_CS_AutocaL_Millis(0xFFFFFFFF);
}
// Set the buzzer as an output:
pinMode(BUZZER_PIN, OUTPUT);
}
void loopcap() {
// Loop through each key:
for (int i = 0; i < 8; ++i) {
// If the capacitance reading is greater than the threshold, play a note:
if(keys[i].capacitiveSensor(NUM_OF_SAMPLES) > CAP_THRESHOLD) {
tone(BUZZER_PIN, notes[i]); // Plays the note corresponding to the key pressed
}
}
}
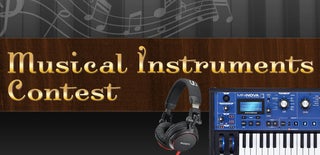
Participated in the
Musical Instruments Contest
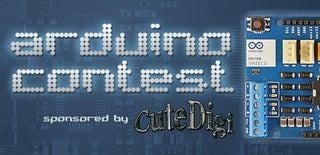
Participated in the
Arduino Contest