Introduction: Simple Clock in Language C
The Idea is to create simple clock in C, but first we need to set up our software and get to know some of the things we are going to use.
Step 1: Step 1:
- Choose Visual Studio, Code Blocks or any other similar software (i would recommend visual studio 2015).
- I will use Visual Studio 2015, so type in google "Visual Studio 2015 Community", download and install.
- After installation, run Visual Studio, press New/Project/Console Application.
- In Console Application Wizard press next, then deselect Precompiled header and select Empty project, then Finish.
- On your right you will have Solution Explorer, right click on Source Files, Add/New Item/C++ file (.cpp), but change name to Source.c and add.
- Now you have C project ready to start.
Step 2: Step 2: Writing Our Code and Learning New Things
This is our code:
#include<stdio.h>
#include <windows.h>//we are including windows file (it's connected to function sleep()), which means that this will only work for windows, so if you're using another OS, search in google for other versions of sleep().
int main()
{
int h, m, s;//we add hours, minutes and seconds to our program
int D = 1000;//we add Delay of 1000 milliseconds, which makes a second and we will use that in sleep().
printf("Set time:\n");//printf writes on screen text that is inside of (" ") and \n writes in a new row.
scanf("%d %d %d", &h, &m, &s);//scanf is where we insert our time, or our values.
if (h > 12) { printf("ERROR!\n"); exit(0); } //in this if function we examine if the inserted value is bigger than 12.
if (m > 60) { printf("ERROR!\n"); exit(0); }//similar here and if it's bigger, program writes ERROR! and exits
if (s > 60) { printf("ERROR!\n"); exit(0); } //similar
while (1)//while (1) is an infinity loop and anything inside repeats itself to infinity.
{
s += 1;// this tells program to increase seconds for 1, everytime the while loop comes to this part.
if (s > 59) { m += 1; s = 0; }//if the seconds are more than 59, it increases the minutes and sets seconds to 0.
if (m > 59) { h += 1; m = 0; }//similar
if (h > 12) { h = 1; m = 0; s = 0; } //similar
printf("\n Clock");
printf("\n%02d:%02d:%02d", h, m, s);//this writes our time in this format "00:00:00"
Sleep(D);//this is our function sleep which slows down the while loop and makes it more like a clock.
system("cls");//this clears the screen.
}
getchar();
return 0;
}
*Everything behind '//' is a comment and does not change the program, so it can be deleted.
**Visual Studio sometimes won't run the program because it consists "scanf", so you need to go to the Solution Explorer>right click on the surface>Properties (Something as in picture should pop up)>in configuration select All Configurations>Configuration properties>C/C++>Preprocessor>in Preprocessor Definitions write _CRT_SECURE_NO_WARNINGS>Save.
Step 3: Step 3: We Insret Our Time to Be Over the Set Boundaries
- Insert random numbers, so that the h is >12, m is > 60, s is > 60.
- Program writes ERROR! and exits.
- So far success!
Step 4: Step 4:
- Insert random numbers, so that the h is < 12, m is < 60, s is < 60.
- numbers change to 00:00:00 format and the clocks starts "ticking".
- SUCCESS indeed.
*After the clock passes 12, 'hours' change to 01 and 'minutes' and 'seconds' to 00.
Have fun!
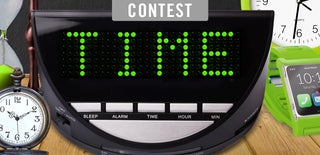
Participated in the
Time Contest