Introduction: Simple Garden Watering System
There are some garden automation systems using Arduino which are fairly detailed. I wanted to develop a simple system for timed watering. One could always develop it using IC555, but the power fluctuations may disturb the watering cycle, and Arduino provides high customization of cycles (plus it's fun!). I tried to use as many household items as possible to keep it simple. Broadly speaking the project involves two steps:
- Making a watering hardware (assembling pumps, pipes and all)
- Driving the pump via a relay controlled by an arduino with a DS1307 RTC circuit.
Following is the list of stuff:
- An Arduino. I used Pro Mini. But any model would be fine.
- A water pump.
- Pipes and small tubes
- Relay
RTC circuit:
- DS1307
- Crystal
- Two 1k ohm resistors
- 3V coin battery
- PCB mount, connectors, wires etc.
- (OPTIONAL) Relay driving circuit:
- NPN transistor. (I used 2222. BC548 would do just fine)
- 1k ohm resistor
PCB mount, connectors, wires etc.
- Container to enclose the assembly
Let's start with the hardware assembly.
Step 1: Hardware Assembly
I tried to use the most basic technique to water my plants. This is not a high precision scientific technique, but it works for me and may as well work for you. (If you already have a motorized watering system in place you can skip this step.)
Pump
I used a small 18W submersible water pump used in aquariums etc,. (It cost me ₹220 (i.e. about $3)). It is small but sufficient for me because I have relatively smaller area to cover. I have like 20 odd pots. If you have larger garden you may want to use a larger pump.
Tubes
I used a tube (about a centimeter in diameter) that ran along the garden (transparent tube seen in the picture). These are the tubes used in household water purifiers. Further smaller tubes (about a size of a ballpen-refill) was pierced in the tube and taken to the pots (they are pinkish in color). They are clamped down by simple metal wires and bamboo twigs. You can use any other means to put them in place.
Depending upon your pump the amount of water coming out will differ. Moreover some pots may require more water than others. Therefore number of holes to pierce will really depend upon your garden. The smaller tubes are not absolutely essential. One can always pierce the holes in the tube and it may work. But the pipes have a tendency to block the holes.
Once you have the hardware in place test it manually and see if you can distribute appropriate amount of water everywhere. It is also advisable to check amount of time required for the pump to distribute, say a 10 liter bucket. This will help you decide how long you want the pump to run.
Once we have the hardware tested manually, we move on to automate it.
Step 2: Automation
The idea is that, at a specific time of day, and for a specific amount of time the Arduino will turn on the relay connected to the pump. To give the Arduino a sense of "specific time" we add a Real Time Clock (RTC) circuit. The RTC will tell the Arduino what time it is, and Arduino will control the relay. If you have a relay operating at 5V, you can directly connect the Arduino output to relay. But I had a 12V relay lying around, which meant I had to build a driver circuit for it. Let's first talk about the driver circuit.
Relay driver circuit
Again, if you have a 5V relay, you don't need this. If you have a higher voltage relay, you can drive it from 5V using an NPN transistor. There are several wonderful tutorials on how to build the driver circuit. See for example :
https://www.instructables.com/id/Connecting-a-12V-R...
http://www.electroschematics.com/8975/arduino-cont...
All you need is a transistor, a diode and a resistor. I used 2N2222 transistor and 1k ohm resistor. A schematic circuit diagram is attached here.
Real Time Clock (RTC) circuit
The RTC is an easy circuit to build. There is a beautiful and detailed instructable on RTC which I followed:
https://www.instructables.com/id/Arduino-Real-Time-...
Please note that Arduino's I2C A4 and A5 pins (SDA and SCL respectively) are specifically made to handle the type of data that DS1307 sends. That means you have to use these specific pins only.
Once you have RTC circuit ready, test it with the code given in the same link. Also adjust the time. This will be once and for all. Later on we'll not use this code. You could use any other code to set the RTC time (like this one, which is easier).
Step 3: Timing & Code
Once you've got the hardware, arduino, RTC circuit, (relay driver, if required) and a relay in place you are done, except for final bit of coding. We have to tell Arduino at what time to start the relay and for how much time. I find the Arduino Cron Library most suitable for this purpose. Just download the zip file and unzip it in library folder of Arduino package. Once you get the library you have to figure out how you want to configure your watering cycle.
If you have calculated how much time it takes to empty your bucket and if you know how many buckets you need to water you can do the math to figure out how much amount of time you want your pump to be driven (number of buckets * time to empty one bucket). Moreover you can choose whether to water at once or schedule it throughout the day. So suppose it takes 180 sec to empty one bucket and 2 bucket is the total amount of water I want to supply to my garden, then I have to drive the pump for 360 sec. Further I want to distribute this water not at once but in 24 hour period. In that case my code would look like this:
//THIS IS JUST AN EXAMPLE CODE // Do not remove the include below #include "Wire.h" #include "RTClib.h" #include "SwitchOnCommand.h" #include "TimedCommand.h" #include "Cron.h" #include "SwitchOffCommand.h" // I have made the trivial changes in the Switch*Command.h files to prevent them from // writing on Serial Console. // create objects for each command SwitchOnCommand sonCommand; SwitchOffCommand sOffCommand; // I am using pin "9" for relay driver. // The cron command has following sequence : sec.min.hr.day.Mon.year, command, pin // '*' means 'every': which means 10,*,*,*,*,* means 10th second of every minute of // every hour of every day of every month of every year. TimedCommand command1("00.00.00.*.*.*",&sonCommand,"9"); // Turn on the pin 9 at 12AM everyday TimedCommand command2("20.00.00.*.*.*",&sOffCommand,"9");// Turn it off at 12:00:20, everyday TimedCommand command3("00.00.03.*.*.*",&sonCommand,"9");// 3AM in the night for 20 sec TimedCommand command4("20.00.03.*.*.*",&sOffCommand,"9"); TimedCommand command5("00.00.06.*.*.*",&sonCommand,"9"); // 6 AM for 20 sec TimedCommand command6("20.00.06.*.*.*",&sOffCommand,"9"); TimedCommand command7("00.00.08.*.*.*",&sonCommand,"9"); //8 AM for 60 sec TimedCommand command8("60.00.08.*.*.*",&sOffCommand,"9"); TimedCommand command9("00.00.10.*.*.*",&sonCommand,"9"); //10 AM for 30 sec TimedCommand command10("30.00.10.*.*.*",&sOffCommand,"9"); TimedCommand command11("00.00.12.*.*.*",&sonCommand,"9"); TimedCommand command12("30.00.12.*.*.*",&sOffCommand,"9"); TimedCommand command13("00.00.14.*.*.*",&sonCommand,"9"); TimedCommand command14("60.00.14.*.*.*",&sOffCommand,"9"); TimedCommand command15("00.00.16.*.*.*",&sonCommand,"9"); TimedCommand command16("20.00.16.*.*.*",&sOffCommand,"9"); TimedCommand command17("00.00.18.*.*.*",&sonCommand,"9"); TimedCommand command18("60.00.18.*.*.*",&sOffCommand,"9"); TimedCommand command19("00.00.21.*.*.*",&sonCommand,"9"); TimedCommand command20("40.00.21.*.*.*",&sOffCommand,"9"); // create an array of timed commands TimedCommand *tCommands[] = { &command1, &command2, &command3, &command4, &command5, &command6, &command7, &command8, &command9, &command10, &command11, &command12, &command13, &command14, &command15, &command16, &command17, &command18, &command19, &command20 }; // create a cron object and pass it the array of timed commands // and the count of timed commands Cron cron(tCommands,20 ); void setup() { //Serial.begin(9600); // In case you want to see the serial output //Serial.println("Starting ArduinoCronLibrary Example"); // sets the time to be the date and time of the compilation // cron.setTime(DateTime(__DATE__, __TIME__)); pinMode(8, OUTPUT); digitalWrite(8, LOW); // I am using pin 8 for Relay ground. } // The loop function is called in an endless loop void loop() { // the loop function checks if a timed command // is due to be executed and executes if it is cron.loop(); }
Once you upload the code you are done. Your pump will follow the cycle you have programmed.
Be careful when operating the relay at high voltage.
If this tutorial helps, do let me know!
Cheers!
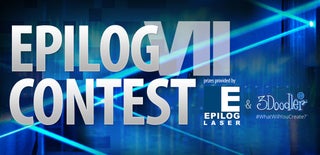
Participated in the
Epilog Contest VII