Introduction: Tello Drone Python Programming, Control Drone With Keyboard and Capture Video From Drone Camera! Using Python Module OpenCV and PyGame!
This Tutorial Will Cover On How You Can Control Drone From Keyboard And How To Initiate Drone Camera Displayed On The Computer!
Supplies
Required Python Version:
- <3.10 And >3.7
- Flexible Python IDE
Required Python Library:
- djitellopy module
- pygame module
- opencv-python module
Required Material:
Amazon Discount - The Best Price Available:
Ebay Discount With My Affiliate Links, Best Price Avalible!:
Step 1: Install the Required Python Module
Import The Required Module:
Enter This Command In The Shell IDE
- Install opencv-python module
pip install pip install opencv-python
- Install pygame module
pip install pygame
- Install djitellopy module
pip install djitellopy
Step 2: Create Module for the Keyboard Input Initialization
Create The First File For Keyboard Initialization Module In Python:
- Named The File as "KeyboardTelloModule.py"
- Write The Lines Of Code (With Explanation):
Import PyGame Python Module:
import pygame
Create Initialization Function:
def init():
#initialize pygame library
pygame.init()
#Set Control Display as 400x400 pixel
windows = pygame.display.set_mode((400,400))
Create Main If Statement:
if __name__ == '__main__':
init()
while True:
main()
- Final Program:
import pygame
def init():
#initialize pygame library
pygame.init()
#Set Control Display as 400x400 pixel
windows = pygame.display.set_mode((400,400))
if __name__ == '__main__':
init()
while True:
main()
After It's Done, Move To The Next Step To Create The Main Python File That Will Be Executed To The Tello Drone.
Step 3: Create Main Tello Python File for Execution
Create The Second File That Would Be The Main Python File:
- Named The File "MainTello.py"
- Write The Lines Of Code (With Explanation):
Import All Python Module Required:
#import module for tello:
from djitellopy import tello
#import module for time:
import time
#import the previous keyboard input module file from the first step:
import KeyboardTelloModule as kp
#import opencv python module:
import cv2
Global Some Important Variable:
#Global Variable
global img
Create a Returning Function For Controlling Drone Based On Keyboard Input:
def getKeyboardInput():
#LEFT RIGHT, FRONT BACK, UP DOWN, YAW VELOCITY
lr, fb, ud, yv = 0,0,0,0
speed = 80
liftSpeed = 80
moveSpeed = 85
rotationSpeed = 100
if kp.getKey("LEFT"): lr = -speed #Controlling The Left And Right Movement
elif kp.getKey("RIGHT"): lr = speed
if kp.getKey("UP"): fb = moveSpeed #Controlling The Front And Back Movement
elif kp.getKey("DOWN"): fb = -moveSpeed
if kp.getKey("w"): ud = liftSpeed #Controlling The Up And Down Movemnt:
elif kp.getKey("s"): ud = -liftSpeed
if kp.getKey("d"): yv = rotationSpeed #Controlling the Rotation:
elif kp.getKey("a"): yv = -rotationSpeed
if kp.getKey("q"): Drone.land(); time.sleep(3) #Landing The Drone
elif kp.getKey("e"): Drone.takeoff() #Take Off The Drone
if kp.getKey("z"): #Screen Shot Image From The Camera Display
cv2.imwrite(f"tellopy/Resources/Images/{time.time()}.jpg", img)
time.sleep(0.3)
return [lr, fb, ud, yv] #Return The Given Value
Initialize a Connection With Drone And Keyboard Input:
#Initialize Keyboard Input
kp.init()
#Start Connection With Drone
Drone = tello.Tello()
Drone.connect()
#Get Battery Info
print(Drone.get_battery())
#Start Camera Display Stream
Drone.streamon()
Main Loop Function:
while True:
#Get The Return Value And Stored It On Variable:
keyValues = getKeyboardInput() #Get The Return Value And Stored It On Variable
#Control The Drone:
Drone.send_rc_control(keyValues[0],keyValues[1],keyValues[2],keyValues[3])
#Get Frame From Drone Camera Camera
img = Drone.get_frame_read().frame
img = cv2.resize(img, (1080,720))
#Show The Frame
cv2.imshow("DroneCapture", img)
cv2.waitKey(1)
Final Program:
#import module for tello:
from djitellopy import tello
#import module for time:
import time
#import the previous keyboard input module file from the first step:
import KeyboardTelloModule as kp
#import opencv python module:
import cv2
#Global Variable
global img
def getKeyboardInput():
#LEFT RIGHT, FRONT BACK, UP DOWN, YAW VELOCITY
lr, fb, ud, yv = 0,0,0,0
speed = 80
liftSpeed = 80
moveSpeed = 85
rotationSpeed = 100
if kp.getKey("LEFT"): lr = -speed #Controlling The Left And Right Movement
elif kp.getKey("RIGHT"): lr = speed
if kp.getKey("UP"): fb = moveSpeed #Controlling The Front And Back Movement
elif kp.getKey("DOWN"): fb = -moveSpeed
if kp.getKey("w"): ud = liftSpeed #Controlling The Up And Down Movemnt:
elif kp.getKey("s"): ud = -liftSpeed
if kp.getKey("d"): yv = rotationSpeed #Controlling the Rotation:
elif kp.getKey("a"): yv = -rotationSpeed
if kp.getKey("q"): Drone.land(); time.sleep(3) #Landing The Drone
elif kp.getKey("e"): Drone.takeoff() #Take Off The Drone
if kp.getKey("z"): #Screen Shot Image From The Camera Display
cv2.imwrite(f"tellopy/Resources/Images/{time.time()}.jpg", img)
time.sleep(0.3)
return [lr, fb, ud, yv] #Return The Given Value
#Initialize Keyboard Input
kp.init()
#Start Connection With Drone
Drone = tello.Tello()
Drone.connect()
#Get Battery Info
print(Drone.get_battery())
#Start Camera Display Stream
Drone.streamon()
while True:
#Get The Return Value And Stored It On Variable:
keyValues = getKeyboardInput() #Get The Return Value And Stored It On Variable
#Control The Drone:
Drone.send_rc_control(keyValues[0],keyValues[1],keyValues[2],keyValues[3])
#Get Frame From Drone Camera Camera
img = Drone.get_frame_read().frame
img = cv2.resize(img, (1080,720))
#Show The Frame
cv2.imshow("DroneCapture", img)
cv2.waitKey(1)
Step 4: Preparing Drone Connection
- Turn On The Tello Drone From The Left Side
- Wait Until it Blink Yellow
- Find The Tello Wifi Connection
- Connect Your Device To Tello Wifi Connection
Step 5: Execute the Program
After The Tello Wifi Connection Connected, Click Run Program If You Are On VSCode Or Execute The Program
Step 6: Final Result
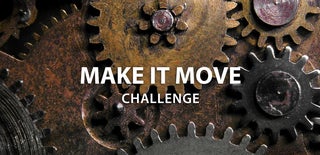
Participated in the
Make it Move Challenge