Introduction: The Idiot's Guide to Programming AVR's on the Cheap (with the Arduino IDE!)
Microcontrollers are, without a doubt, amazing little things. They are versatile, powerful, and extremely tiny. Unfortunately, the latter trait is also shared by both my wallet and my programming skills. My understanding of C is poor, and I can hardly afford to buy something like an Arduino or a decent ISP. And in any case, the Arduino would be overkill for many of my projects, which only need simple IC's.
But as many of you know, DIY always finds a way. This tutorial is meant for those among us with no budgets or programming experience who want to start using these little machines. It is not based around the ATmega328 (the Arduino Uno chip), but rather the Attiny line of chips (the Atiny85 and Attiny2313, to be specific). The total cost of this project can go as lower than $15 if you know where to buy from, and you can still use the original Arduino IDE and language to program your projects in the end. Keep in mind that you will need some soldering skills to get this project done.
But as many of you know, DIY always finds a way. This tutorial is meant for those among us with no budgets or programming experience who want to start using these little machines. It is not based around the ATmega328 (the Arduino Uno chip), but rather the Attiny line of chips (the Atiny85 and Attiny2313, to be specific). The total cost of this project can go as lower than $15 if you know where to buy from, and you can still use the original Arduino IDE and language to program your projects in the end. Keep in mind that you will need some soldering skills to get this project done.
Step 1: So What Programmer Do I Get?
My, my, I thought you'd never ask. There's a large number to choose from, so I'll give you a rundown of the most popular ones.
• The USBasp is quite possibly the cheapest programmer out there, but you should be careful when buying them; some versions use outdated firmware or are missing jumpers. Make sure that your model has three jumpers (or three pairs of holes with J1, J2, and J3 printed next to them). You can find them on eBay starting at only $3. Be wary of the shipping times that the Chinese ones come with.
• A parallel port programmer is around the same price and can even be made at home. It, obviously, will only work on computers with a parallel port.
• The USBTinyISP is a step up from the USBasp. It costs $22 from Ladyada, but knockoffs can be had from eBay for around $12. Keep in mind that it cannot program certain high end Atmega devices due to memory limitations.
• The AVRISP mk.II is Atmel's official programmer for the AVR line of chips. It is without a doubt the most powerful of these programmers and can be used with AVR Studio (complete with debugger!), but costs around $35 to get.
Your programmer might come with either a 10 pin connector or a 6 pin connector. The only difference between the two is that the 10 pin connector has 4 redundant/unused pins.
Due to my aforementioned lack of money, my programmer was a USBasp. Once again, make sure you get one with all three jumpers; mine looks nice and comes with a fancy case, but is missing 2 of them. Thankfully, it still works.
• The USBasp is quite possibly the cheapest programmer out there, but you should be careful when buying them; some versions use outdated firmware or are missing jumpers. Make sure that your model has three jumpers (or three pairs of holes with J1, J2, and J3 printed next to them). You can find them on eBay starting at only $3. Be wary of the shipping times that the Chinese ones come with.
• A parallel port programmer is around the same price and can even be made at home. It, obviously, will only work on computers with a parallel port.
• The USBTinyISP is a step up from the USBasp. It costs $22 from Ladyada, but knockoffs can be had from eBay for around $12. Keep in mind that it cannot program certain high end Atmega devices due to memory limitations.
• The AVRISP mk.II is Atmel's official programmer for the AVR line of chips. It is without a doubt the most powerful of these programmers and can be used with AVR Studio (complete with debugger!), but costs around $35 to get.
Your programmer might come with either a 10 pin connector or a 6 pin connector. The only difference between the two is that the 10 pin connector has 4 redundant/unused pins.
Due to my aforementioned lack of money, my programmer was a USBasp. Once again, make sure you get one with all three jumpers; mine looks nice and comes with a fancy case, but is missing 2 of them. Thankfully, it still works.
Step 2: Is That All?
Unless you plan on programming air, you might want to get a microcontroller and some parts for a programming cradle.
Most AVR microcontrollers will work with the programmers I listed on the previous step, but only some can be used with the Arduino language.
• The Attiny85 and Attiny45 are tiny 8 pin chips with 6 functional inputs and outputs with a max of 8kb of memory.
• The Attiny84 and Attiny44 are 14 pin clones of the chips above.
• The Attiny2313 is a 20 pin chip with 2kb of memory.
• The Atmega328 is the chip used on the actual Arduino. You'll have to edit a few things if you want to use it without its crystal.
Take your pick; any of these will work well.
In addition to a chip, you'll want a few parts to make a cradle; otherwise, you'll have to breadboard out all of the connections whenever you want to program anything. For that, you'll need:
• A chip socket (pick one that'll accommodate the chip you plan on using).
• Male pin headers, 2 sets of 5 (if your programmer has a 6 pin cable, go for 2 sets of 3.
• Female pin headers (same number of pins at your socket) OR more male pin headers if you want it to plug into a breadboard.
• Strip board.
• Wire.
• Basic soldering equipment.
If you want to get these fast, try Digikey. If you want to get these parts cheap with 2 week shipping, go with Tayda Electronics.
Most AVR microcontrollers will work with the programmers I listed on the previous step, but only some can be used with the Arduino language.
• The Attiny85 and Attiny45 are tiny 8 pin chips with 6 functional inputs and outputs with a max of 8kb of memory.
• The Attiny84 and Attiny44 are 14 pin clones of the chips above.
• The Attiny2313 is a 20 pin chip with 2kb of memory.
• The Atmega328 is the chip used on the actual Arduino. You'll have to edit a few things if you want to use it without its crystal.
Take your pick; any of these will work well.
In addition to a chip, you'll want a few parts to make a cradle; otherwise, you'll have to breadboard out all of the connections whenever you want to program anything. For that, you'll need:
• A chip socket (pick one that'll accommodate the chip you plan on using).
• Male pin headers, 2 sets of 5 (if your programmer has a 6 pin cable, go for 2 sets of 3.
• Female pin headers (same number of pins at your socket) OR more male pin headers if you want it to plug into a breadboard.
• Strip board.
• Wire.
• Basic soldering equipment.
If you want to get these fast, try Digikey. If you want to get these parts cheap with 2 week shipping, go with Tayda Electronics.
Step 3: Small Scale MIG Welding, Anyone?
To start out, lets assemble a simple (ghetto) cradle for our chips. Most of these chips have pinouts that differ quite a bit, so only the Attiny85 and Attiny2313 can be programmed on the same socket. The design I used for mine takes advantage of this.
First, arrange your parts on the stripboard as shown and solder them down. Try not to make your joints as ugly as mine.
Our goal is to match up all six essential pins on our programmer with the corresponding pins on our microcontroller; these pins would be MISO , MOSI , SCK , RESET/RST, GND , and VCC/VTG. The two rows of male headers will be where our programmer plugs in. I've included diagrams for most of the chips you can use. I've also included a diagram that shows which pin is which on your header. Go slow, and make sure that you don't mix up any pins.
Once you are done, test out your pins with a continuity tester and make sure that they all go to the right places. You may want to seal up your circuit to prevent damage. In my case, I just drenched it in hot glue. Thankfully, FCC regulations do not apply to my circuit.
First, arrange your parts on the stripboard as shown and solder them down. Try not to make your joints as ugly as mine.
Our goal is to match up all six essential pins on our programmer with the corresponding pins on our microcontroller; these pins would be MISO , MOSI , SCK , RESET/RST, GND , and VCC/VTG. The two rows of male headers will be where our programmer plugs in. I've included diagrams for most of the chips you can use. I've also included a diagram that shows which pin is which on your header. Go slow, and make sure that you don't mix up any pins.
Once you are done, test out your pins with a continuity tester and make sure that they all go to the right places. You may want to seal up your circuit to prevent damage. In my case, I just drenched it in hot glue. Thankfully, FCC regulations do not apply to my circuit.
Step 4: Setting Up Your Not-so-micro Computer.
Now that our chip is ready to be programmed, we need to set up the programmer. If you have a USBasp and run windows, download the driver from http://www.fischl.de/usbasp/ and install it. If you have a USBtinyISP and run windows, get your driver from http://learn.adafruit.com/usbtinyisp/download. Check in "Device Manager" to see if your programmer shows up when you plug it in; if so, you are ready!
Download the latest version of the Arduino IDE from http://arduino.cc/en/Main/Software and install it. To get this to work with chips other than the ATmega328, we'll need to add a few plugins. Go to https://code.google.com/p/arduino-tiny/ and download the latest zip of Arduino-tiny. Go to "C:/(Your install directory)/Arduino/hardware" and create a new folder called "tiny". Unzip the contents of arduino-tiny into this folder, and rename the file called "Prospective Boards.txt" to "boards.txt".
Then, open up the Arduino IDE. First, go to Tools->Programmer and select your programmer from the drop down list. Then, go to Tools->Boards and select the chip that you want to program. There might be multiple versions of the same chip; make sure that you select the "1 MHz" version, as factory chips are tuned to run at that frequency out of the box.
If you are using a USBasp, you should short out Jumper3. This slows down the programming speed enough for you to program chips that work at 1 MHz.
Finally, give your new chip a whirl! Find "Fade" in the File->Examples->01-Basics menu and change the line
int led = 9; // the pin that the LED is attached to
to
int led = 1; // the pin that the LED is attached to
since your chip may not have a pin 9. Then, hit upload.
You might get a few warnings, bu if everything works out...you should have successfully programmed your first chip!
Hook up the GND pin of your chip to the negative terminal of a 5v supply and VCC to the positive. Then connect an LED (with a resistor) between the negative terminal and pin 1 of your chip. If it slowly fades in and out, you win!
Download the latest version of the Arduino IDE from http://arduino.cc/en/Main/Software and install it. To get this to work with chips other than the ATmega328, we'll need to add a few plugins. Go to https://code.google.com/p/arduino-tiny/ and download the latest zip of Arduino-tiny. Go to "C:/(Your install directory)/Arduino/hardware" and create a new folder called "tiny". Unzip the contents of arduino-tiny into this folder, and rename the file called "Prospective Boards.txt" to "boards.txt".
Then, open up the Arduino IDE. First, go to Tools->Programmer and select your programmer from the drop down list. Then, go to Tools->Boards and select the chip that you want to program. There might be multiple versions of the same chip; make sure that you select the "1 MHz" version, as factory chips are tuned to run at that frequency out of the box.
If you are using a USBasp, you should short out Jumper3. This slows down the programming speed enough for you to program chips that work at 1 MHz.
Finally, give your new chip a whirl! Find "Fade" in the File->Examples->01-Basics menu and change the line
int led = 9; // the pin that the LED is attached to
to
int led = 1; // the pin that the LED is attached to
since your chip may not have a pin 9. Then, hit upload.
You might get a few warnings, bu if everything works out...you should have successfully programmed your first chip!
Hook up the GND pin of your chip to the negative terminal of a 5v supply and VCC to the positive. Then connect an LED (with a resistor) between the negative terminal and pin 1 of your chip. If it slowly fades in and out, you win!
Step 5: Get Programming!
Check out the Arduino website for more examples and tutorials; it's an easy language to learn. Keep in mind that some commands and libraries might not work or take a bit more effort to get working.
Here are a few last tips to remember for the ride:
• If you want to make your device run at 8MHz, select the "8MHz" version of your chip and hit "Burn Bootloader". Keep in mind that most speeds higher than that will need an external oscillator.
• If you want a bigger programming challenge, learn to code with AVR Studio. You'll have to manually use AVRdude to actually burn the code onto the chip, but you will have MUCH more control over how your program works.
• If you plan on programming a fresh Atmega328 (Arduino Uno chip) with this setup, you (ironically) can't directly use the Arduino IDE to do so. I've yet to try this, but you should be able to fix this with the following. You'll have to edit the boards.txt in "Arduino/hardware/arduino" and change a few lines in the "Arduino Uno" section. Replace the lines
uno.bootloader.low_fuses=0xff
uno.bootloader.high_fuses=0xde
uno.bootloader.extended_fuses=0x05
with
uno.bootloader.low_fuses=0xE2
uno.bootloader.high_fuses=0xDF
uno.bootloader.extended_fuses=0xFD
and change
uno.build.f_cpu=16000000L
to
uno.build.f_cpu=8000000L
That'd be it! With a day's work and nothing but cheap parts from China, you've just built a (hopefully) functioning programmer.
If you see any mistakes in my ible or have any questions, leave a comment. Good luck.
Here are a few last tips to remember for the ride:
• If you want to make your device run at 8MHz, select the "8MHz" version of your chip and hit "Burn Bootloader". Keep in mind that most speeds higher than that will need an external oscillator.
• If you want a bigger programming challenge, learn to code with AVR Studio. You'll have to manually use AVRdude to actually burn the code onto the chip, but you will have MUCH more control over how your program works.
• If you plan on programming a fresh Atmega328 (Arduino Uno chip) with this setup, you (ironically) can't directly use the Arduino IDE to do so. I've yet to try this, but you should be able to fix this with the following. You'll have to edit the boards.txt in "Arduino/hardware/arduino" and change a few lines in the "Arduino Uno" section. Replace the lines
uno.bootloader.low_fuses=0xff
uno.bootloader.high_fuses=0xde
uno.bootloader.extended_fuses=0x05
with
uno.bootloader.low_fuses=0xE2
uno.bootloader.high_fuses=0xDF
uno.bootloader.extended_fuses=0xFD
and change
uno.build.f_cpu=16000000L
to
uno.build.f_cpu=8000000L
That'd be it! With a day's work and nothing but cheap parts from China, you've just built a (hopefully) functioning programmer.
If you see any mistakes in my ible or have any questions, leave a comment. Good luck.
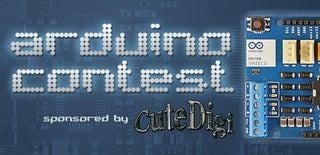
Participated in the
Arduino Contest
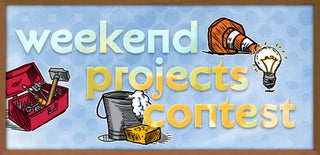
Participated in the
Weekend Projects Contest