Introduction: The Story Clock: Arduino LCD W/ Cap Sensors
This is yet another Arduino LCD clock, but with a twist; this clock knows some holidays and birthdays, and can make calculations on the fly of how old someone is and then display the information on the LCD screen. I was inspired to make this clock because two dear friends had a big birthday party and asked for 'only stories' as gifts. This clock continually updates the story of their time together.
The clock can obtain power from a USB cord, 2 D cell batteries, or a wall wart (input 7-12 volts 500mA). It will run for about 2-3 months on the D-cells so that is not the optimal power supply. The color of the LCD backlight can be changed.
This is my second Instructable, and like the first one (a personality tester), it is designed for people like me; beginners with Arduino microcontrollers but who have some rudimentary woodworking skills. This Instructable is essentially divided into two parts; how I made the electronics and how I made the enclosure.
Step 1: Assemble the Parts
For this project, I used:
- an Arduino Uno
- a Tiny RTC 1307 (real time clock) module
- an Adafruit Cap1188 8-spot capacitance sensor
- an Adafruit Powerboost 500 module (helps the Arduino run off 2 D-cell batteries)
- an Adafruit RGB 16x2 LCD screen for displaying the time
- 2 10k pots that I bought cheap on Amazon (pack of 10 drop shipped from China)
- a single-pole single-throw on-off switch that I had lying around (probably came from All Electronics)
- a 2-battery D-cell holder
- some wire
- a box to hold the clock
- I used some burled maple plywood leftover from a different project and some scrap redwood for the legs and top. You could use a cigar box or almost any box you liked from a thrift store. I will explain how I made my enclosure later in this Instructable.
Step 2: How the Clock Works
There are two thick copper wire bands on the front of the clock. Touch the one on the left (as you face the clock) and it displays information about one person. Touch the copper wire on the right and another person's information is displayed. Press both wires at the same time and information about their combined time together is displayed.
To set the time, the back of the clock has a variety of copper sensor wires. Changing the time involves simultaneously touching the appropriate copper wires on the back of the clock – for example to set the minutes back by 5, simple touch the minutes wire and then tap the minus wire 5 times, once per second. The clock is set up to only advance the time by one unit per second, so if you need to set the clock ahead 30 minutes it will take 30 seconds of holding the wires. Setting the year requires simultaneously touching three wires; the month and day wires and then the plus or minus wire.
The battery on/off is useful for saving batteries and for resetting the clock. This should be switched off when the clock receives power from a wall wart or from USB. Two 10k-ohm potentiometers control the LCD brightness and color.
Step 3: Wiring the Clock
Wiring up the clock is pretty straightforward, or so it seems (as my second Arduino build).
There are the two parts that 'talk' to the Arduino using I2C; the clock and the cap1188 sensor breakouts. I wired the clock first to pins A4 and A5 of the Arduino. Then I 'daisy chained' the cap1188 breakout board in by wiring it to the SDA and SCK outs from the clock breakout board (essentially they are wired in parallel).
A wiring diagram for the exact RTC board I used is here:
http://smart-prototyping.com/Arduino-Tiny-RTC-I2C-...
There are two 10k-ohm potentiometers for controlling the backlighting color and contrast. They get 5v and ground to the outer posts (doesn't matter which side gets what) and the center posts go to either A3 of the Arduino or to pin 3 of the LCD screen.
The LCD screen is wired up exactly as explained on the Adafruit website:
https://learn.adafruit.com/character-lcds
as is the CAP1188 sensor:
https://learn.adafruit.com/adafruit-cap1188-breako...
As usual, I bought enough components to make one clock on the breadboard and another 'for real'. Thus, I had a working breadboarded version to look at as I wired up the real one into the clock enclosure.
Step 4: The Arduino Code
Download the Arduino code here and then open it in the Arduino editor (I wrote it using version 1.6.3). You will include a couple standard libraries (wire.h and LiquidCrystal.h) and SPI.h is included (but I don't think I used it, it is legacy from the original code I cobbled together to make the clock).
You will also need two special libraries. I have placed them here for download only because I wanted you to have the exact versions I used (so everything will work). However, I strongly suggest that you seek out the original files from Adafruit (Cap1188) and from David H Brown (for the real time clock library) because they might get updated and because these people put in a great deal of effort making these libraries for people like us.
Here is the basic logic of the clock:
The capacitance sensor determines what should happen, so check to see if it has been touched.
- Cap Touched?
- NO = display the time on the top line
- Check if it is a birthday or some other special day?
- YES = display a special message on the 2nd line
- NO = display the date on the second line
- Check if it is a birthday or some other special day?
- YES, the #1 cap sensor wire was touched
- Calculate things about person 1 and display them for a couple seconds
- YES, the #2 sensor has been touched
- Calculate and display things about person 2
- YES, both caps 1 and 2 have been touched!
- Calculate and display information about how long the couple have been together.
- NO = display the time on the top line
(Now we look for cap touches that are related to time keeping)
- YES caps 3 & 7 have been touched; move hours ahead 1
- YES caps 3 & 8; move hours back 1
- YES caps 4 & 7; move minutes ahead 1
- YES caps 4 & 8; move minutes back 1
- YES caps 5 & 7; move months ahead 1
- YES caps 5 & 8; move months back 1
- YES caps 6 & 7; move days ahead 1
- YES caps 6 & 8; move days back 1
- YES caps 5,6 & 7; move years ahead 1
- YES caps 5,6 & 8; move years back 1
- YES but the touches are not described above; do nothing and move on.
Finally, check if the knobs on the back for color have been moved - if so, change the color of the LCD screen.
Then loop back to the beginning of the program.
Step 5: Programming the Clock for Beginners: Cap Sensors
Capacitance sensors are neat little devices; you see them all the time because they are used for things like lamps that change brightness just by being touched. The breakout board I used, from Adafruit, outputs whether the wires have been touched using I2C and sends numbers as powers of 2 (I am just learning this, maybe you are too). For most of you this will be too simplistic, but it still confuses me, so I made a table of how the cap responds to being touched.
The call to see if something has been touched is "touched" and can be coded like this:
if (touched == 1) { do something when the first wire -and only the first one- is touched }
For example, if the 5th wire is touched, the Arduino 'sees' the number 16. Further, suppose that the 3rd wire and 6th wires are touched at the same time, the Arduino would see the number 36. Why? because the wires attached to the capacitance sensor are numbered from 0-7 and the 3rd wire has the number 2^2=4 and the 6th wire has the number 2^5=32 and the sum of the two numbers is 4+32=36.
Still confused? Try this video as a place to start... (it helped me).
So, with 8 wires attached to the capacitance sensor, we can have lots of different combinations [(2^8)-1 = 255 combinations] to do different things. I only coded the clock for 13 different things. They are: something about person 1, something about person 2, something about them together, plus hours, minus hours, plus minutes, minus minutes, plus days, minus days, plus months, minus months, plus years, and minus years.
If you look at the Arduino code you will see where I have the Arduino check for the different combinations that have meaning. Each little snippet like this one:
else if (touched == 36)
{ k = plusmin(); }
calls for a tiny subroutine to do something specific. The example above calls for the subroutine to set the minutes ahead by one minute.
Step 6: Programming the Clock for Beginners: the Math
The clock has this nifty (at least I think it is nifty) feature where if you touch one of the wires on the front of the clock, it calculates how old the clock owner is and displays it in either years, months or days. The math is pretty simple, but if you haven't done math using an Arduino, here is a little guidance. As with the previous step, skip this if you are already an expert.
The picture above is of the 'calcs' subroutine. It will calculate and store the age of the two clock owners into 6 different integers, and also calculate how long they have been together (3 integers). It works like this:
- Is it before or after Ruth's birthday this year?
- After: calculate her age as 35 + years (where 35 is how old Ruth was on this date back in the year 2000, and 'years' is what year it is now)
- Before: calculate her age as 34 + years (because her birthday hasn't arrived yet this year).
- Store this calculation as an integer called 'ruth_y' (you should change this to the name of the person you are making your clock for)
- Convert from years to months and store that number. Use this formula: 'ruth_y' * 12 + how many months since her birthday
- Convert from months to days and store that number. Here I 'cheated' and used the average number of days in a month over a 4-year period (taking into account the leap year = 30.43) and then added the number of days in the current month. It is not completely accurate, but oh well...
repeat this for the other things to calculate.
Step 7: Programming the Clock for Beginners: Setting the Time
I admit that it took me a great while to figure this out. It seems simple now... Here is one of the subroutines I use:
//subroutine for setting day ahead
int plusday() {
RTC.setDay(dates + 1);
RTC.setClock();
delay(500);
}
It works like this: set the 'dates' number ahead by 1, then set the clock, then delay for 1/2 a second before doing it again. Two things to note: You have to set the thing you are setting and then set the clock (e.g. have the two statements in there together and in the right order). If you have them in the wrong order, or if you omit the setClock(); statement, it won't work. Second, the 1/2 second delay is in there to keep the clock from adding lots and lots of time from a brief touch of the wires.
Here are the calls you can use if you are using the RealTimeClockDS1307.h library:
getHours getMinutes getSeconds getYear getMonth getDate getDay getDayOfWeek (pretty self explanatory)
setClock (have to use after each of the following things, but not for the calls above)
setSeconds setMinutes setHours
setAM setPM set24h switchTo24h switchTo12h
setDayOfWeek setDate setDay setMonth setYear
stop start (to start and stop the clock, if you want to do that)
sqwEnable sqwDisable writeData readData (square wave output stuff I didn't use)
is12hour isPM isStopped getFormatted getFormatted2k (useful for talking to the clock via the serial port)
There are two ways to initially get the clock going.
- Use a separate program to start the clock. That is what I did - below is a link for you to download the little program I used. You set the time in the program and run it once.
- Or you can simply temporarily add one line of code to the main program at the very beginning of the void loop. Add this: start clock(); then run the program once and it should say that it is 00:00:00 on Jan 1 2000. Then comment out the line, run the program again, and use the buttons on the back of the clock to set the time. This will be slow.
Attachments
Step 8: Build the Enclosure
You can use most anything as an enclosure - I am building one using a cigar box, but for this project my enclosure was constructed from some scrap 1/4 inch particle board that has a nice maple burl veneer on one side. I cut it so that a 4 D-cell battery holder would fit inside (I wound up using only a 2-battery holder) and made the depth of the box slightly larger than the Arduino. I test-fit it several times, then measured for the placement of the LCD screen. I drilled a set of holes and then used a file to slowly shape the rectangular hole so that the LCD screen fit into it snugly.
For the legs, I took a piece of scrap redwood and penciled in a curved shape, cut the legs on a band saw and then used double-sided tape to hold the 4 legs together as a single piece. Then, as a single piece, I shaped it on a belt sander. This trick is great because it helps all 4 legs to be identical (they weren't identical after the band saw cutting).
Then I set up a router with a bit to cut a 90 degree groove into the legs. I don't remember who taught me this trick, but it helps add the legs to the box and keeps the box construction simple. I test fit everything several times, then glued the box together, then glued the legs onto the box.
At this point I realized that I needed holes for the Arduino USB and power cables. Too late! It was already glued up. So I cut them by hand, and if you look at the pictures you can see that this mistake led to the veneer peeling on those cuts. It looks poor; luckily it is on the back of the clock and not the front.
Step 9: Finishing the Enclosure
The lid for the clock is another piece of scrap redwood. I also cut two small pieces of wood to form rails to hold it to the clock. Imagine an upside-down railroad track. The lid is held in place by friction and a brass screw on the back side that holds one of the rails to the clock. I then sanded the entire thing so it was smooth. I sprayed it all with shellac, several coats, and then let it all dry. Time to add the electronics!
Step 10: Add the Electronics to the Box
I measured and then re-measured where the copper wire for the capacitance sensors should go, then drilled small holes to accept the wire. The copper wire is from Home Depot, but it was just something I had lying around from another electrical job. It is 14 gauge. I bent it to shape then pushed it through the holes and bent the back sides. Then I soldered some colored stranded wire to it for connecting to the cap1188 breakout board.
I also drilled two holes for the 10k potentiometers and soldered wires to them. Finally, I drilled a hole for the on/off switch. At this point the clock was just a mess of wires sticking out the top of the enclosure with no electronics inside.
I used an ice pick to 'pre-drill' some small holes in the box and then screwed the Arduino and the RTC clock boards in place. I wired that all up to the Arduino then added the cap sensor and wired that up last. I did it that way because I was lazy and didn't feel like making more small holes for the cap sensor to be screwed to the inner walls of the clock enclosure. It simply sits atop all the wires.
I tested it several times along the way and noticed that the cap sensors were very sensitive to moving the wiring inside the box. Luckily, the on/off switch resets the sensors, and the lid helps keep everything in place nice and snug.
Lastly I soldered the battery booster to the 2 D-cell battery pack, wired it up and shoved it in the bottom of the clock. It is held in place by friction and a small scrap of wood that I glued to the bottom. I should have thought that part through more, but oh well, it works fine and nobody can see it unless they actively look.
Step 11: Final Thoughts
I really enjoyed building this clock for our friends, and I will build one for my own family. Simple things I will add will be more holidays and family birthdays, and I'll probably add a buzzer alarm and a little light sensor to automatically turn the brightness down at night. There are lots of tutorials here on Instructables for adding these things and now that I am a little familiar with the real time clock library, adding more functionality should be within my grasp.
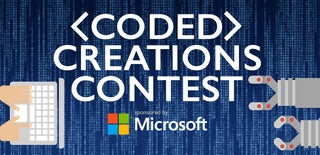
Participated in the
Coded Creations