Introduction: Truly-Random Digital Die
Dice are subject to tampering and potential defected products. However, a digital die, generating a roll from a digitally randomized result produces a truly random result at each roll. This project uses 6 different coloured LEDs to make a digital die, wherein the Arduino processes the input to find a random number from 0 to 6. This number is then displayed on the die. In addition, the die will roll for a random amount of time, showing the different random die number that is rolling before it eventually stops.
Step 1: Tools and Materials
- Arduino Uno
- Breadboard
- 6 LEDs
- 6 pieces of 100Ω Resistors
- Jumper Cables
- Push button
- 10K Ω Resistor
Step 2: Connecting the LEDs to the Arduino
1. Connect each of the LED's anodes to a 100Ω resistor.
2. Connect each of the LED's cathodes to ground.
3. Connect the open-end of the resistor to digital pins 3, 4, 7, 8, 9, 11 of the Arduino.
Step 3: Connecting the Push Button to the Arduino
4. Connect one pin of the push button to ground.
5. Connect the remaining pin to a 10K Ω resistor which then connects to the 3.3V power rail.
6. Connect the same pin to pin 2 on the Arduino.
7. Then, connect the 3.3V pin from the Arduino to the 3.3V power rail on the breadboard
8. Finally, complete the circuit by connecting the ground pin from the Arduino to the common ground rail on the breadboard.
Step 4: Coding
int ledPins[7] = {2, 3, 4, 7, 8, 9, 11};<br>
int dicePatterns[7][7] = { {0, 0, 0, 0, 0, 0, 1}, // 1 {0, 0, 1, 1, 0, 0, 0}, // 2 {0, 0, 1, 1, 0, 0, 1}, // 3 {1, 0, 1, 1, 0, 1, 0}, // 4 {1, 0, 1, 1, 0, 1, 1}, // 5 {1, 1, 1, 1, 1, 1, 0}, // 6 {0, 0, 0, 0, 0, 0, 0} // BLANK }; int switchPin = 10; int blank = 6; void setup() { for (int i = 0; i < 7; i++) { pinMode(ledPins[i], OUTPUT); digitalWrite(ledPins[i], LOW); } randomSeed(analogRead(0)); } void loop(){ if (digitalRead(switchPin)) { rollTheDice(); } delay(100); } void rollTheDice() { int result = 0; int lengthOfRoll = random(15, 25); for (int i = 0; i < lengthOfRoll; i++) { result = random(0, 6); // result will be 0 to 5 not 1 to 6 show(result); delay(50 + i * 10); } for (int j = 0; j < 3; j++) { show(blank); delay(500); show(result); delay(500); } } void show(int result) { for (int i = 0; i < 7; i++) { digitalWrite(ledPins[i], dicePatterns[result][i]); } }
Step 5: Demo
When the push button is pressed, the die is rolled for a random time, to generate a digitally randomized LED pattern corresponding to a number rolled by the die. In the demo, I rolled a four.
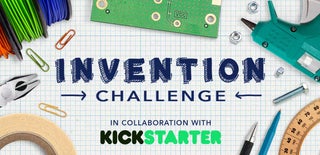
Participated in the
Invention Challenge 2017
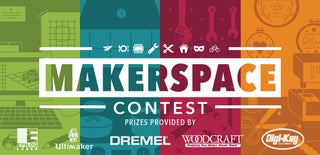
Participated in the
Makerspace Contest 2017