Introduction: Arduino Controlling a Servo and a Motor to Control a Train Set
We are going to step through how to make an arduino control a train set by using a servo and a motor. This circuit can be applied for other uses if you want to use a motor or a servo in your arduino. We will show you how to construct your circuit and code the arduino using Matlab. You can use other coding applications, but this is the one we used for this project, and we encourage you to look at other instructables if you are having trouble. This circuit consists of two parts. The first is a potentiometer that controls a servo, and the second is two buttons that control a motor to spin in both directions. We are going to first focus on the potentiometer and servo.
Step 1: Setting Up the Servo
Here we begin by plugging the positive end of the board into the 5v slot in the arduino and the negative side of the board into GND in the arduino. This will supply power to the components in the board. We now set up the servo by plugging the black wire into the negative side, plugging the red wire into the positive side, and plugging the other colored wire into a digital pin slot (we chose pin 9). A picture of the circuit so far is shown above.
Step 2: Attaching the Potentiometer
We now attach the potentiometer to the board. Plug the potentiometer into the board. The left side of the potentiometer will connect to the positive end of the board, the middle will connect to the analog pin A0, and the right side will connect to the negative side of the board. Another picture of the current board can be seen above.
Step 3: Constructing the Buttons With Lights
We will now begin constructing the other part of the circuit, the buttons to control the motor. We will start by adding buttons and lights to help visualize when a button is being pressed. We place two buttons on the board bridging across the middle section as shown in the picture. Bridge a 10K ohm resistor between the left side of each button and the positive side of the board. Connect the left side of each button to a digital pin (we used digital pin 2 and 3). Our red button is on the left and plugged into pin 3 while our green button is on the right and hooked to pin 2. The lights are put in the same orientation. The left (negative) side of the lights are attached to 330 ohm resistors which are connected to the positive end of the board. The right (positive) side of the lights are connected to a digital pin by a wire. We put the red light on digital pin 13, and the green light on pin 12 as shown in the picture.
Step 4: Attaching the Motor
We start by plugging a NPN transistor into the board. The middle pin will be connected to a 330 ohm resistor which is connected to digital pin 8 by a wire. The right side of the transistor is connected to the negative side of the board, and the left side of the transistor is connected to a separate column. This is wrong in the above picture and corrected in the next step picture. Connected to this column is a diode which is used to prevent the transistor and motor from being damaged. On the other side of the diode is a wire connected to the positive side of the board. Now attach the two wires of the motor to two separate columns. Normally you would attach the motor wires to the columns with the diode, but we want to make the motor turn both ways, so we can not do that. I will explain how we do this in the next step.
Step 5: Constructing a H-Bridge to Fit Inside the Motor Circuit
To make the motor turn both ways, you have to use a H-bridge. If you are not familiar with an H-bridge, please familiarize yourself so that you understand the next information. There are several ways to do this. If you have a H-bridge, use that and put it inside the motor circuit. We did not have one, so we made one in our circuit. You can do this using transistors, manual switches, and relays. They are all similar in construction, but we will show you how to construct a H-bridge out of relays. There are other instructables that explain how to make H-bridges out of transistors, so you can refer to those for more information. We are going to start by setting up the relays without connecting it to the motor yet. You will do the same thing for one relay a total of 4 times for 4 total relays. For reference, the relays will be called relay 1, 2, 3, and 4 from left to right. Plug a NPN transistor into the board. The left pin connects to a separate column, the middle pin connects to a 330 ohm resistor which connects to a digital pin (we used pins 4, 5, 6, and 7), and the right pin is connected to the negative side of the board. From the separate column, create a parallel circuit that contains a diode that goes across the middle section. Now connect a wire from the separate column into the controlling part of the relay. Depending on your relay, this may change where you put it. On our relay, there were two pins labeled + and -, and we put our wire into the - pin. Connect a wire from the other side of the relay (+ side) to a column across the middle section from the previous separate column. This column should be connected to the diode. Now attach this column to the positive side of the board. Use the picture for reference.
Step 6: Connecting the H-bridge to the Motor.
For the H-bridge to work correctly, the relays have to be plugged in a certain order. Here are labels for the columns in this step. Column 1 is the column with the yellow wire and diode for the motor. Column 2 is the column with the orange wire and diode for the motor. Column 3 is the column with the green wire connected to the motor. Column 4 is the column with the yellow wire connected to the motor. The relays are numbered the same as the last step, from 1-4 from left to right. Each relay has its own colored wired. These wires will be plugged into the "switch" part of the relay. First, connect column 2 and relay 1 with a wire. Connect relay 1 and column 3 with a wire. Connect column 4 and relay 4 with a wire. Connect relay 4 and column 1 with a wire. Connect column 2 and relay 3 with a wire. Connect relay 3 and column 4 with a wire. Connect column 3 and relay 2 with a wire. Connect relay 2 and column 1 with a wire. Ignoring the diode, you should have three wires in columns 1, 2, 3, and 4. This is to check if you made the right connections.
Step 7: Final Circuit
Your circuit is now ready to be coded and used. Here is a picture of the final circuit and a picture of our personal circuit.
Step 8: Matlab Code for the Circuit
The following code is from Matlab, sorry if you are using a different coding application. Before using this code, you must connect to your arduino and servo. Connecting to these is different for Mac and PCs, so make sure you connect correctly. The information on how to connect can be found on the mathworks site. Here is the link to get to the matlab package support help for arduino.
https://www.mathworks.com/help/supportpkg/arduinoi...
Here is the code we used with comments.
function arduino_project_2017_04_09(a,s)
writeDigitalPin(a,'D4',1);writeDigitalPin(a,'D5',1);writeDigitalPin(a,'D6',1);writeDigitalPin(a,'D7',1); %shuts all the relays to the off position
writeDigitalPin(a,'D8',1); %turns the motor on, but the motor does not spin until the relays are switched on
while true %infinite while loop
degrees=readVoltage(a,'A0')./5; %reads the voltage across analog pin A0 which is hooked to a potentimeter
writePosition(s,degrees) %turns the servo to a certain position depending on the angle on the potentimeter
red=readDigitalPin(a,'D3'); %the value for the red button
green=readDigitalPin(a,'D2'); %the value for the green button
if green==0 %if the green button is pressed
writeDigitalPin(a, 'D11', 1) %turn the green light on
writeDigitalPin(a,'D5',0);writeDigitalPin(a,'D6',0) %activate relays 2 and 3 to make the motor spin cw
else %if the green button is not pressed
writeDigitalPin(a, 'D11', 0) %turn the green light off
writeDigitalPin(a,'D5',1);writeDigitalPin(a,'D6',1) %switch relays 2 and 3 to the off position
end %end if statement
if red==0 %if red button is pressed
writeDigitalPin(a, 'D13', 1) %turn red light on
writeDigitalPin(a,'D4',0);writeDigitalPin(a,'D7',0) %switch relays 1 and 4 to the on position to make the motor turn ccw
else %if the red button is not pressed
writeDigitalPin(a, 'D13', 0) %turn the red light off
writeDigitalPin(a,'D4',1);writeDigitalPin(a,'D7',1) %switch relays 1 and 4 to the off position
end %end if statement
end %end infinite while loop
end %end function
Step 9: Using to Control a Train Set.
One can use this setup to control a train set. We do this by connecting the servo to a mechanical switch that switches lanes in the track that the train will go on. We connect the motor to a knob that controls the speed of the train. You have to connect the servo and motor to their respective parts by making the parts to connect them to. We made our part out of wood and 3-D printed material, but we have not got them to work exactly to our needs yet. By using the arduino and code, we can control the speed and which track the train goes on by turning the potentiometer and pressing the buttons.
Step 10: Problems That We Had During Our Construction and Coding Process.
We had and still have some problems with our circuit, and you might experience these problems as well. Our servo constantly twitches, and it does not like to move in the positions in between its limits. In other words, the servo can move from 0 degrees to 180 degrees, but it has trouble getting from 0 to 180. Building the H-bridge manually from scratch was very difficult and time consuming. This is because we were not sure how one worked or fit inside the circuit, so we would highly recommend buying an h-bridge and using it. We have not yet been able to integrate our motor and servo into the train set yet, but we know that the concept works.
Step 11: Conclusion
We hope you had fun making your circuit and coding your circuit. If you have any questions, please ask in the comments.
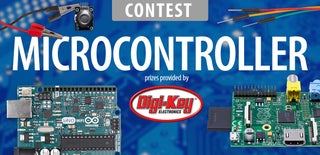
Participated in the
Microcontroller Contest 2017