Introduction: Automatically Save Screenshots in Windows With a Python Script
Usually in Windows 7, to save a screenshot(print screen) first we need to take a screenshot and then open paint, then paste it and then finally save it.
Now, I will teach you how to make a python program to automate it.
This program will create a folder named 'shots' in your desktop and save screenshots in a new folder within shots with the time it was taken on when you press the PrtScn key and exit the program when Ctrl + PtrScn is pressed.
You will need python 3.7 installed, a text editor(I used Sublime Text 3), autopy and pynput python packages
Step 1: Installing Autopy and Pynput
After installing python 3.7, open cmd(command prompt) and type in the following:
pip install autopy
press enter. This will install the autopy package. After this is done, type:
pip install pynput
to install pynput package.
Step 2: Coding
Open your text editor, and type in the following:
import datetimeimport osimport autopyfrom pynput.keyboard import Key, Listener
then type:
exit_combination = {Key.ctrl_l, Key.print_screen}currently_pressed = set()
this sets the key combination to exit the program when the user presses a combination of keys, in this case, it's Left Ctrl + PrtScn.
Then type:
path="c://Users//"+os.getlogin()+"//Desktop//shots//"+str(datetime.date.today())try: os.makedirs(path)except FileExistsError: pass
This will make a folder named shots in your desktop and within it another folder with the current date.The os.getlogin() is used to get the current user.
Then type:
with Listener(on_press=on_press,on_release=on_release) as listener:
listener.join()
here the Listener function listens for keystrokes and the join() is used to collect them until released.
Now let's define the functions, type them right after the import statements, before 'exit_combination'.
We need to define 3 functions: on_press, on_release, and check_key.
on_press and on_release are functions that are required by the Listener function.
def on_press(key):
check_key(key) if key in exit_combination: currently_pressed.add(key) if currently_pressed == exit_combination: listener.stop()
this function takes the parameter 'key' and passes it on to the check_key(key) function. Then it checks if the key is in the exit combination, i.e the combination of keys to press to exit the program, if it is, then it stops executing the listener function.
Then type:
def on_release(key):
try: currently_pressed.remove(key) except KeyError: pass
this removes the key from the currently pressed set.
Then type:
def check_key(key):
if key == Key.print_screen: shot = autopy.bitmap.capture_screen() now = datetime.datetime.now() timenow = now.strftime("%H_%M_%S") path = "c://Users//"+os.getlogin()+"//Desktop//shots//"+str(datetime.date.today()) try: shot.save(path+'//'+timenow+'.png') except FileNotFoundError: os.makedirs(path) shot.save(path+'//'+timenow+'.png')
This function compares the currently pressed key with the specified key(print_screen key), if it matches, then it takes a screenshot using the autopy library's capture_screen() and saves it to the variable 'shot'.
Then it redefines the path variable to use the current date(this is done to create a new folder with the current date so that even if the user does not restart the program after 12:00 pm, the screenshots will be saved in a new folder with the updated date.
A try statement is used to save the picture to a folder with the current date. If the folder does not exist, it will produce a FileNotFoundError which is handled by the except statement by making the folder and then saving it.
Now save the code with a .py extension.
Check the attached python file if it's not clear~
Attachments
Step 3: Testing the Code and Running Without the Console Window.
If your text editor supports running the code, then run it. If not, then double click the python file to run it.
If you don't get any errors, then congrats.
Now, if you don't want to see the console window every time you run the code, then change the file extension from .py to .pyw.
The default key combination to exit the program is left ctrl + prtscn, you can change this by changing it in exit_combination.
If you liked this instructable, please vote for me in the contest
Step 4:
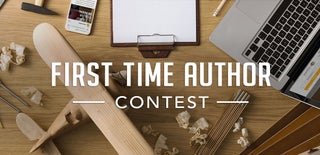
Participated in the
First Time Author Contest