Introduction: CheerLightbridge
This project rejuvenates an old art project at the University of Surrey and connects it to the magical world of IoT!
Cheerlights is a global IoT 'variable' which can be updated by anybody (even you!) via Twitter, using #cheerlights and a tweet including one of 11 common colours listed on their site. The cool bit is that all over the world people are making IoT devices connected to coloured lighting and connecting them to the Cheerlights variable, including everything from Christmas trees to plaster casts. This means that if you tweet a colour, potentially thousands of lights across the continents will change colour together!
The lightbridge is an old art project by Tine Bech and Louis Christodoulou which used to show a cryptic display of academic achievement for the university. Now the original sponsors have finished with it, we can have some fun and thought of nothing better than to hook it up to a global IoT project. With this bridge being a prominent part of campus, we cannot change the whole colour every time someone updates #cheerlights (30 seconds to a few minutes typically). Instead, we pick a random light (there are 38 in total, across two floors) and change the colour of that one.
The next steps will delve into the hardware and software and the issues along the way.
Step 1: Hardware
The bridge installation consists of a small linux PC driving a string of LED parcans over DMX, an industry-standard lighting control protocol used in the entertainment industry and based on RS-485. The DMX is generated through an Entec DMX Pro USB to DMX adapter, which are one of the most common adapters available to buy. There is perfectly good open-source Arduino code available and DMX shields, but it's nice to already be provided with a professional option :)
The DMX and power is routed from the PC enclosure to the bridge itself. A couple of technicians installed the 38 parcans over a few days, along with a large amount of expensive diffusive acrylic sheets and some aluminium U-channel to hold it with. Slots were made in the sheeting to allow for the window handles, although these are cable-tied shut and are not in use. Only one side of the bridge is lit, as both sides are completely covered in glass, which was the architect's original vision (there are actually two bridges, and each has the opposite circular pattern of blue dots so they align at the right position).
DMX is a serial protocol with daisy-chained slave devices, and they must be manually addressed before use. This is typically done using DIP switched to set binary values, although on more modern or expensive lights there is a display and push buttons. Some even have touch screens! DMX supports 512 addresses, each of which can receive an 8 bit value. This group of 512 addresses is called a 'universe', and is sent down a single 5-core or 3-core cable (another story). A light may use several addresses in order to receive more data, so they are assigned a start address and the control software knows how many addresses they are listening on. These lights use 3 addresses, for 8-bit red, green and blue data. Some lights can also be configured to use more addresses for extra features. The different modes are called 'personalities'.
Step 2: Software - OLA
In order to drive the DMX output, there were several choices. There are many open source libraries in languages like C++, Python and NodeJS which allow you to send data to the Entec interface. However, another desire was to allow the lights to also be controlled from a conventional lighting desk. This is because the bridge backs on to the campus lake, where a stage has recently been built for concerts. Running a DMX cable from the lake stage would be difficult, but a very popular implementation of DMX over ethernet, Artnet, can be used instead. This is supported by most modern lighting desks, including the ones our Stage Crew society uses. The great thing about it using UDP packets over ethernet is that is can ride on the existing IT infrastructure which is prevalent at universities.
As an aside: Without Artnet, many modern shows would not be possible where hundreds of moving intelligent lights, which consume tens of addresses each, would require more DMX universes than the desks could give out on connectors at the back. Instead, Artnet can handle 32,768 universes on one network cable!
So, if our IoT controller outputs Artnet, which also has many libraries available in various languages (especially as it is a network protocol and requires no interface hardware), then we can use either the lightingn desk or our software to control the bridge. What is required is some sort of broker to receive the Artnet from different sources and output the data to the DMX interface. Luckily, there is an excellent open-source project called Open Lighting Architecture (OLA) which does just that!
Once OLA is installed, you can configure the included plugins (or download more) in order to support the protocols and interfaces you require. Then, you can add a new universe by selecting an input interface (in our case an Artnet input on our local network adapter). Once this is created, you can enter the configuration for the new universe and add an output, which for us was the Entec DMX Pro. The plugin required for the DMX Pro is Serial USB. This can be otherwise confusing to work out.
Once your universe is configured, you can test drive it using the DMX console tab on the web interface and change some values. Hopefully, you should see your lights change along with the monitor panel adjacent to the controls. This tab, and the DMX monitor tab, is very useful in debugging the system, although it doesn't have the fastest refresh rate..
So now you should be able to send DMX to the hostname or IP address of your OLA server, and it should send that data to the output interface you configured :)
Step 3: Software - Node-RED
In order to receive updates from #cheerlights and convert the colour names to red, green and blue pixel values for our Artnet data, we need another piece of software. This is essentially our IoT software. For ease of use and rapid prototyping we chose Node-RED, a visual data-flow programming environment running on NodeJS.
Instead of using a Twitter input node, which could monitor the #cheerlights hashtag, we used Andy Stanford-Clark's MQTT topic (mqtt://iot.eclipse.org with the topic cheerlights). This should use less processing on our side, as well as supporting a nice lightweight IoT protocol to keep the internet pipes a bit clearer. The main essence of the code, located in the 'Colour to Channels' function, converts the colour value to an RGB value array, and sends it to the Artnet output node, which is pointed at the OLA server on localhost.
var rgbMap = {};<br>rgbMap.red = [255, 0, 0]; rgbMap.green = [0, 255, 0]; rgbMap.blue = [0, 0, 255]; rgbMap.cyan = [0, 255, 255]; rgbMap.white = [255, 255, 255]; rgbMap.oldlace = [253, 245, 230]; rgbMap.purple = [128, 0, 128]; rgbMap.magenta = [255, 0, 255]; rgbMap.yellow = [255, 255, 0]; rgbMap.orange = [255, 165, 0]; rgbMap.pink = [255, 20, 147]; rgbMap.black = [255, 255, 255]; values = rgbMap[msg.payload]; numCans = 18*2; var offset = 3*Math.floor(Math.random()*numCans); msg.payload = {offset: offset, data: values}; return msg;
You may notice that 'black' is set to white RGB colours. Black is not an officially supported cheerlights colour, but is used by some people, and gets through to the MQTT topic. We didn't want any of the lights to turn off on the bridge, as it may look like they were dead, so we replaced that value with white!
The rest of the code supports some useful functionality. The top row of the flow checks every minute to see if it is dark enough to see the lights on the bridge, and if not, turns them off by sending zeros to all addresses (i.e., black to all lights). In order to keep the colours going while the bridge is off, and to start in the evening with a full set, a global state is used as a buffer for the colour values. A lot of the other function blocks are used to update and read that state. Finally, the nodes at the bottom of the flow are used to submit black or random colours to the entire array of lights, in order to light up the bridge if the state is lost on a restart, or if you just want to even out the colours.
In addition to the back-end code, which is the main part of Node-RED, we are also using the new dashboard as a UI for testing and control. The main flow shows several controls (buttons and a toggle switch) which are used for blackout and fill control, and the second flow shows the large array of colour-picker controls (contributed by the author :-) ) which are used to show the current state of the lights.
The flow is available at http://flows.nodered.org/flow/1a27d1e9c1846224f264165f28645ed1
Step 4: The Result
The result has been great to watch, knowing that the mosaic of colours shining out at night is a result of people all over the world tweeting their colours, not knowing where they end up! It's also really responsive locally, as within a second or so of tweeting a new colour by the bridge, a light flicks to your new colour!
This is far from the end plan for this project. We aim to implement a Google calendar solution, still through Node-RED, which allows the events department at the university to book preset patterns and chases for certain days, like LGBT and charity fundraisers. We have several other LED installations across campus, and it looks as though they will be integrated into the same system soon enough, which would be far more difficult without tools like Node-RED and the other open-source software used in this project (especially Linux of course, the biggest one of them all!).
To improve, there are a few changes we would like to make. The Artnet node needs rewriting, and we want to support fading to new colours. There is an OLA node available which does this, but via HTTP requests for every fade step of every channel to the OLA server. We tried this, but it was very glitchy and had to go with the Artnet node instead. The OLA node is under active development so this issue may be addressed soon!
We also want to allow users to edit frames of animations live using the same colour-picker display as in the preview page. This would be pretty cool, especially with a speed and fade control!
All in all it has been a great Christmas project which was great to watch while you're playing with code, especially when things go wrong and it starts strobing like crazy!!! Although many people may not have the resources or bridges to copy this instructable, the same software can be applied to IoT control of conventional entertainment lights, or to LED pixel strips, with modification.
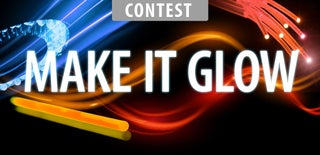
Participated in the
Make it Glow Contest 2016
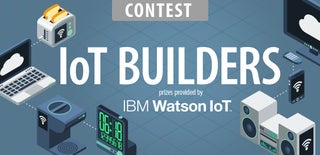
Participated in the
IoT Builders Contest