Introduction: DJI PHANTOM SIMULATION
Dà-Jiāng Innovations Science and Technology Co., Ltd (DJI) is a Chinese technology company
headquartered in Shenzhen, Guangdong. It manufactures unmanned aerial vehicles (UAV), also known as drones, for aerial photography and videography, gimbals, flight platforms, cameras, propulsion systems, camera stabilizers and flight controllers. It was funded 11 years ago, and since then it has been the most outstanding company in the sector. Nowadays it controls the 70% of the drone market and has achieved more than one billion dollars in sales.
One of its greatest inventions has been the PHANTOM series (currently in 4th generation). For this reason, today we are going to study how one of these magnificent drones works.
For this Instructables, we have divided the process in two: the first one is about the coding and construction of the drone, while the second one is about the controller.
Step 1: DRONE - Materials
For the construction of the drone we will need the following components:
1. Arduino Uno: for this project, a plate Uno is enough to control the 4 motors, since we are going to focus on making them work, without getting into stability or flight direction.
2. Brushless motors (x4): We are going to build a quadcopter drone, which means that it has a total of 4 motors, one per extremity. The advantages of a brushless motor over brushed motors are high power to weight ratio, high speed, and electronic control. Brushless motors find applications in such places as computer peripherals (disk drives, printers), hand-held power tools, and vehicles ranging from model aircraft to automobiles.
3. Cables: to connect the different components. It is recommended to have different measures, since some of them are going to be welded.
4. Base Board: where different welded components will go.
5. ESC (Electronic Speed Controller x4): essential for coordinating and controlling brushless motors, they need to be of 20A.
6. Power distribution board: this will connect the 4 motors in one single OUTPUT, making it easier to connect with the Arduino.
7. Battery or power supply: that gives at least 11.1V.
8. HC06 Bluetooth: also known as the “slave” one, it will receive information from the one in the controller.
Step 2: DRONE - Connect the Components & Code
This is the scheme to follow to connect the components to the arduino and to the Lipo battery. Although it may seem complicated, once you have looked at it well it is not so.
Just connect:
ESC - to Battery - (Using the Power Distribution board) (RED)
ESC + to Battery + (Using the Power Distribution board) (BLACK)
ESC signal to Arduino Pins (9,10,11,12) (YELLOW)
ESC servo - to Arduino GND (BROWN)
ESC servo + to Arduino 3,3V (BLUE)
The connection between ESCs is very simple. The ESC central cable is connected to the central cable of the brushless motor (blue). The side cables (PINK & GREEN) are connected depending on the direction of rotation. The drones have two motors that rotate in one direction (1 & 3) and two that rotate towards the other (2 & 4) to have a good stability.
(a). Blue cables are not essential, but this way the arduino uses the electricity of the Lipo battery. If we do not connect the blue cables, the arduino must be fed with a battery or with a USB.
Here is the code to control the brushless motors from the computer. This code hasn't the bluetooth controller.
***********
#include //Using servo library to control ESC
Servo esc; //Creating a servo class with name as esc
void setup() {
esc.attach(9);
esc.attach(10);
esc.attach(11);
esc.attach(11);
esc.writeMicroseconds(1000); //initialize the signal to 1000
Serial.begin(9600);
}
void loop() {
int val; //Creating a variable val
val = analogRead(A0); //Read input from analog pin a0 and store in val
val = map(val, 0, 1023, 1000, 2000); //mapping val to minimum and maximum(Change if needed) esc.writeMicroseconds(val); //using val as the signal to esc
}
***********
Step 3: DRONE - Bluetooth Configuration (Slave)
We will need: Arduino UNO, 4 jumpers, HC06.
Connect the Bluetooth module:
VCC-pin12 (current)
GND-GND arduino
TXD-RX arduino
RXD-TX arduino
The first step is to set the digital pin 12 as LOW to load the sketch and then the command will be given from the computer to change it to HIGH and so the sketch could be loaded.
We put the code:
************
const int LED = 13; //using the arduino led
const int BTPWR = 12; //digital pin of our bluetooth (POWER)
char nameBT [10] = "DJIslave"; //Bluetooth name (slave)
char velocity = '4'; //9600 bps
char pin [5] = "0000"; //password
void setup() {
pinMode(LED, OUTPUT); //Pin 13 as output
pinMode(BTPWR, OUTPUT); //Pin 12 as output
digitalWrite(LED, LOW); //The led is used to know when the configuration of the bluetooth has finished digitalWrite(BTPWR, HIGH);
Serial.begin(9600); // TX and RX are the serial port. Velocity of the serial port, the same as the bluetooth. Serial.print("AT"); //I'm going to start with AT comands.
delay(1000); //to know that the commmands has end
Serial.print("AT+NAME"); Serial.print(nameBT); delay(1000);
Serial.print("AT+BAUD"); Serial.print(velocity); delay(1000);
Serial.print("AT+PIN"); Serial.print(pin); delay(1000);
digitalWrite(LED, HIGH); //To know that the bluetooth has been configurated }
void loop() {
//no code }
************
The arduino led TX (center of the board) will be started, this means that it is being configured. When the LED TX is switched off and the arduino led 13 is turned on it means that the slave Bluetooth configuration is finished.
We can try with the mobile phone if the bluetooth we have configured appears. The phone has a master bluetooth, so it can connect with it.
The bluetooth led is all the time flashing. It will stop when a master bluetooth connects with it.
Step 4: DRON - Bluetooth Connection + Code
Now you have to connect the bluetooth, as shown in the scheme, inside the set of brushless motors.
The following code must be added to the code of the brushless motors. It will be used to control the power of the brushless motors from another bluetooth. This bluetooth is the slave.
Our HC-06 is set to use 9600 baud rate. If yours is using a different speed then you will need to change the sketch. Change the 9600 to what ever speed your HC-06 is using.
Code:
************
void setup() {
Serial.begin(9600);
Serial.println("Arduino with HC-06 is ready");
// HC-06 default baud rate is 9600
BTSerial.begin(9600);
Serial.println("BTserial started at 9600");
}
void loop()
{
if (BTSerial.available())
Serial.write(BTSerial.read());
if (Serial.available())
BTSerial.write(Serial.read());
}
************
Step 5: CONTROLLER - Materials
For the construction of the controller we will need the following components:
1. Arduino Uno: to connect the components together.
2. Cables
3. Potentiometers (x2): one of them will control the speed of the motors, letting more or less current to go through them. The other controls the brightening of the LCD.
4. LCD: will display the total current that is going through the motors.
5. Base board: to weld all the components.
6. HC05 Bluetooth: or the “Master” one that will send the information of the Potentiometers right to the slave one, allowing us to control the motor’s speed from the controller.
7. 9V Power Supply.
Step 6: CONTROLLER - Connect the Components & Code
This is the scheme to follow to connect the components to the arduino. It has two parts. The first one, is the connection of the LCD to the Arduino board. And, the second one, is the potentiometer connection .
It is not important the resistance of the potentiometer because we will use it only as analog. With it we will be able to modify from 0 to 1023 the speed of the motors.
The LCD will show a value depending on the position of the potentiometer. For example, if the potentiometer is in the position 450 (between 0 and 1023, where 0 is minimum and 1023 is maximum), using the "map" function in the code, the value will make a relation between 0% to 100%. This way, the 450 its a 43,99% and the motors will rotate with a 43,99% of its maximum speed.
This code hasn't the bluetooth controller.
**********
#include LiguidCrystal.h
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
void setup() {
lcd.begin(30, 45);
Serial.begin(9600);
}
void loop() {
lcd.setCursor(0, 0);
int sensorValue = analogRead(A0); sensorValue = map(sensorValue, 0, 1023, 0, 100);
lcd.print("Pot: ");
lcd.print(sensorValue );
lcd.print("%");
delay(1); // delay in between reads for stability
}
**********
Step 7: CONTROLLER - Bluetooth Configuration (Master)
We will need: Arduino UNO, 4 jumpers, HC05.
Connect the Bluetooth module:
VCC-pin12 (current)
GND-GND arduino
TXD-RX arduino
RXD-TX arduino
EN (it is used to activate the configuration mode. If there is a push button it is done later. If there is no push button, a cable is connected to 3.3V). In this case we will not connect cable because we have a button.
The procedure is practically the same as when configuring the slave module. The main difference is that module HC 05 can work as a slave and as a master. Instead, module HC06 only as a slave. So the only thing we have to do is tell the HC05 that he is a master. To do this, we create the "modo" function in the code. If we say "modo = 1" we are telling him that he is a master. If we put "0" we are telling him that he is a slave.
Upload the code:
**********
const int LED = 13; //using the arduino led
const int BTPWR = 12; //digital pin of our bluetooth (POWER)
char nameBT [11] = "DJImaster"; //Bluetooth name (master)
char velocity = '4'; //9600 bps
char pin [5] = "0000"; //pasword
char modo = '1'; //It can be 1 if its master or 0 if its slave for de HC05
void setup(){
pinMode(LED, OUTPUT);
pinMode(BTPWR, OUTPUT);
Serial.begin(38400); //Velocity of the BT in configuration Mode (NOT 9600!!)
digitalWrite(BTPWR, HIGH);
delay(3000);
Serial.print("AT\r\t"); //to start the configuration (return and line jump)
Serial.print("At+NAME:");
Serial.print(nameBT);
Serial.print("\r\t"); //command has finished
Serial.print("At+PIN:"); //AT+PSWD:
Serial.print(pin);
Serial.print("\r\t"); //command has finished
Serial.print("At+BAUD:");
Serial.print(velocity);
Serial.print("\r\t"); //command has finished
Serial.print("At+MODE:"); //AT+PSWD:
Serial.print(modo);
Serial.print("\r\t"); //command has finished
digitalWrite(LED, HIGH); //To know that the configuration of the bluetooth has finished }
void loop(){
//no code
}
**********
After charging the code, the led 13 remains fixed for a moment. The button of the bluetooth is pressed until the LED of the bluetooth is started. After a few seconds the led 13 will start again. And the bluetooth will be configured. The bluetooth is still in configuration mode (flashes slowly). If we unplug it the bluetooth module and then plug it again (from the current) the LED will blink faster. Now it is well configured. When blinking fast, its looking for the slave to connect to it.
Step 8: CONTROLLER - Bluetooth Connection + Code
Now you have to connect the bluetooth, as shown in the scheme, inside the set of LCD and Potentiometer.
The following code must be added to the code of the LCD and potentiometer. It will be used to control the power of the brushless motors. This bluetooth is the master.
**********
#include SoftwareSerial.h
SoftwareSerial BTserial(2, 3); //RX/TX
char c = ' ';
void setup() {
Serial.begin(9600);
Serial.println("Arduino with HC-05 is ready");
// start communication with the HC-05 using 38400
BTserial.begin(38400);
Serial.println("BTserial started at 38400");
}
void loop() {
// Keep reading from HC-05 and send to Arduino Serial Monitor
if (BTserial.available()) {
c = BTserial.read();
Serial.write(c);
}
// Keep reading from Arduino Serial Monitor and send to HC-05
if (Serial.available()) {
c = Serial.read();
Serial.write(c);
BTserial.write(c);
}
}
**********
The bluetooth master's function is to send the A0 value from the controller, regulated with the potentiometer, to the drone. This way, the dron will read this value and the motors will rotate more or less quickly depending on the potentiometer position.
Step 9: PROTOTYPING
To make the model of the drone, we started with a 3D file taken from the GrabCad website.
With the SolidWorks program, the necessary adjustments were made so the drone could contain all the computer systems and the extremities would fit the motors. Once that was done, the body was divided into two so that it could be milled with a CNC milling machine. For that reason we chose wood as the main material of the drone's body, since it is the easiest to machine and has more resistance than expanded polyurethane.
Step 10:
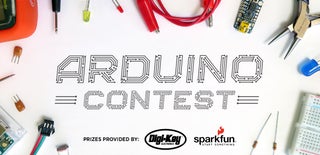
Participated in the
Arduino Contest 2017
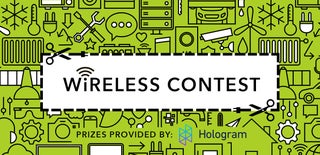
Participated in the
Wireless Contest