Introduction: Fun With Arduino, Nothing Else Needed, Part 2
I was curious to see how an Arduino would work for math and simple text based graphics so I decided to do a little experimenting. These programs are better suited for a computer but it is interesting to see that an Arduino can run them. Have fun.
While you are here please check out part one of this series:
https://www.instructables.com/id/Fun-with-Arduino-nothing-else-needed/
It is also included in my beginning Arduino collection located here:
Step 1: Electronic Dice
This program uses the serial monitor to display the roll of two dice. Copy/Paste the program into the Arduino IDE and open the serial monitor. Enter any character to roll the dice. The character you enter is not used for anything. The first time through the loop it seeds the random number generator using millis().
/******************************************************* * Filename: ArdDice.ino * * An electronic dice roller. Open the serial monitor, * enter any character and press Enter to roll the dice. * *******************************************************/ int firsttime = 1; // Set first time flag void setup() { Serial.begin(9600); } void loop() { Serial.println("Enter any character and press Enter"); char choice = ' '; // Unused character. while(Serial.available() == 0); choice = Serial.read(); // Get character. if(firsttime==1) // If first time through loop, { randomSeed(millis()); // seed random number generator, firsttime=0; // and clear first time flag. } Serial.println(" "); Serial.println(" "); dice(); // Roll and print first die. dice(); // Roll and print second die. } /*********************************************** * dice() - Function to roll and print one die. ***********************************************/ void dice() { int rnd=random(1,7); switch(rnd) { case 1: Serial.println(" "); Serial.println(" * "); Serial.println(" "); Serial.println(" "); Serial.println(" "); break; case 2: Serial.println(" * "); Serial.println(" "); Serial.println(" *"); Serial.println(" "); Serial.println(" "); break; case 3: Serial.println(" * "); Serial.println(" * "); Serial.println(" *"); Serial.println(" "); Serial.println(" "); break; case 4: Serial.println(" * *"); Serial.println(" "); Serial.println(" * *"); Serial.println(" "); Serial.println(" "); break; case 5: Serial.println(" * *"); Serial.println(" *"); Serial.println(" * *"); Serial.println(" "); Serial.println(" "); break; case 6: Serial.println(" * *"); Serial.println(" * *"); Serial.println(" * *"); Serial.println(" "); Serial.println(" "); break; } }
Attachments
Step 2:
This program creates a graph of the numbers rolled on two dice. Copy/Paste the code into the Arduino IDE, upload it to your Arduino, open the serial monitor and set the baud rate to 115200.
Each asterisk represents five rolls of the number.
********************************************************************
* Filename: DiceGraph.ino * * This program creates a graph of the numbers rolled on two dice. * Copy/Paste the code into the Arduino IDE, upload it to your * Arduino, open the serial monitor and set the baud rate to 115200. * Each astrisk represents five rolls of the number. ******************************************************************** / Accumulators to count the number // of times each number occures. int accum[13]{0,0,0,0,0,0,0,0,0,0,0,0,0}; int firsttime=1; // Set first time flag. void setup() { Serial.begin(115200); } void loop() { if(firsttime==1) // If first time through loop. { Serial.println("Enter any character and press Enter to start:"); char choice = ' '; // Unused character. while(Serial.available() == 0); choice = Serial.read(); // Get character, just to start program. randomSeed(millis()); // Seed random number generator, firsttime=0; // and clear first time flag. } int d1=random(1,7); // Roll dice. int d2=random(1,7); int dice=d1+d2; accum[dice]++; // Increment the accumulator. for(int i=2;i<13;i++) { if(i<10) // Print the numbers. { Serial.print(" "); // Space to keep columns aligned. Serial.print(i); } else { Serial.print(i); } Serial.print(" - "); if(accum[i]>4) // Print one * for every 5 times munber occures. { for(int j=1; j<=accum[i]/5; j++) { Serial.print("*"); } Serial.println(""); } else Serial.println(""); } delay(100); Serial.println(""); Serial.println(""); Serial.println(""); Serial.println(""); }
Attachments
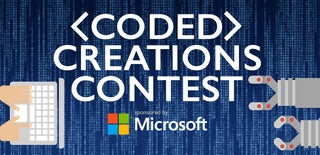
Participated in the
Coded Creations