Introduction: How to Code Arduinos
So I was looking through the help section of the community when I noticed some people where having trouble with coding Arduinos, so I'm making this to help.
For this Instructable you will need:
Computer
Arduino IDE
Some parts of this may be different for 3.3v Arduinos, i use a 5v Arduino so i will be teaching according to that.
Step 1: Input Commands
Here where gonna look at Input Commands. There are two main types of pins on the Arduino, Digital I/O(Input/Output) and Analog Input, the Digital pins can be both Inputs and Outputs, and the Analog pins are special inputs that can detect the current Voltage.
PinMode
Before we can use any sensors or actuators we need to tell the Arduino what pins they are on.
int sensorPin = A1; Void setup(){ pinMode(sensorPin, INPUT); }
This tells the Arduino that there is a input on pin sensorPin, which is a integer standing for the number A1. The reason we do this is because if we need to change the pin number for some reason, we can change it in the one place at the top instead of going trough the entire code and having to change the number every time its referenced.This command goes in Void setup, most commands go in Void loop so put them there unless told otherwise.
Digital Read
This is the most basic way to input into a Arduino. The signal well either be On or Off, this is used for detecting the state of a light switch or button. Here is how to use it.
int DsensorVal = digitalRead(DsensorPin);
This assigns int DsensorVal to equal what DsensorPin is picking up. In this case it will be a 1 or 0, On or Off.
Analog Read
The Analog Read command is for detecting voltage on one of the Analog pins of the Arduino. This is used to connect to any sensor that gives off a voltage as data, such as a Light Dependent Resister, Trimmer pot, some Temperature sensors, etc.
Int AsensorVal = analogRead(AsensorPin);
This assigns int AsensorVal to the voltage on AsensorPin. this will be a value between 0 and 1023, according to a voltage between 0 and 5 volts, ex. 1.25v = 256, 2.5v = 512, 3.25v = 768.
We'll see how to use these in the Next Step.
Step 2: How to Use Input Values
Now that we have values from our sensors lets see how to use them.
IF
Yes i said IF, the IF command is the most important command of all. It is the main bridge between our actuators and sensors. Here's how it's used.
if (a == b){ action here(We'll discuss this in the next step) }
The brackets is where we will place the code we want to run. The == means equal to, this has to be two equals because only one would make a = b and make it always true. The == can be replaced any one of the following,
- != not equal
- > greater then
- < less then
- >= greater then or equal to
- <= less then or equal to
So IF a == b then we run the brackets. We can also have more then one Criteria to fulfill
if ((a == b) && (a < c)){ }
Now we added the && which means AND, so as long as a == b AND a < c, the IF statement will run the code. we can also use || (OR) and ! (NOT) in the place of && to get the appropriate response.
That is the most basic and most used Control command. We can take this command one step further by adding a ELSE to it. This makes it so that when IF is not active the ELSE is.
if (a == b){ { else{ }
Now anything in the ELSE brackets will be run when IF is negative.
Digital
So the way we use the digital input is like this.
If (DsenserVal == 1){ }
1 is the same as writing HIGH. So when there is 3-5volts placed on DsenserPin, the action you have set will happen. sometimes buttons are set in reverse, so that it equals 0 when the button is pressed, if so just change the 1 to a 0 and it will work.
Analog
This is where it gets fun.
We have the normal if command.
if (AsensorVal < 500){ }
Where IF is checking the value of AsensorVal and reacting to it, analog signals are more meant to be used to edit the response of a robot instead of making it respond. We also have a couple commands to edit the signal so its better for end use.
Map
The map command is useful when you need to change the number for a certain range. So say I have a int value that is going to be between 300 and 500, and i want to use it to control a servo that goes 180 degrees, well i can use the command like this
AsensorVal = map(AsensorVal, 300, 500, 0, 180);
Now the value is a number from 0 to 180 that is prepositional to the difference of 300 to 500. So think of a line that is 200 units long, this changes it so the line is the same length but now is only 180 units long. This takes a lot of complex math, but the good folks behind Arduino have made it easy.
Constrain
This one makes the number have to stay in certain bounds. So if i set it up like this.
AsensorVal = map(AsensorVal, 0, 180);
It now will only allow AsensorVal to be between 0 and 180, if it is lower then 0 then it turns it into 0, if it's above 180 it will change it to 180, these numbers are the min and the max that it can be.
Let's go see how to use Actuators in the next Step.
Step 3: Actuators
So you want to see how to use actuators, well your at the right Step.
PinMode
yes where using PinMode again, but this time where using it to register a OUTPUT.
void setup(){ pinMode(actuatorPin, OUTPUT); }
Now we have declared ActuatorPin a output, so now we can use it as one. This goes in the Void setup.
myServo.attach
I wasn't going to leave servos out don't worry. Servos require a little more setup but its still easy.
#include <Servo.h> Servo myServo; # Makes a servo object Int servoPin = 9; # The number for this has to one of these pins, 11, 10, 9, 6, 5, 3. Void setup(){ myServo.attach(servoPin); # Attaching the servo to servoPin, which is equal to 9; }<br>
So first we import the servo library, and then we make the object myServo. Then we make a int that is equal to the pin we want to place it on. This has to be a special pin that supports PWM which are marked on the Arduino board. We then attach myServo on the pin we set eariler. Put the attach command in Void setup.
now that we got those setup, lets look at actual outputting signals.
DigitalWrite
This is the basic On/Off output you'd use to control a LED, Reley, and anything else that is either On or Off.
digitalWrite(actuatorPin, HIGH);
Now actuatorPin is set to High, so anything on it will start to run. To turn it off we use.
digitalWrite(actuatorPin, LOW);
AnalogWrite
The AnalogWrite command is used to control Motor Speed, LED Brightness, etc. Now most Arduinos dont have actual Analog outputs, but they are able to mimic it. They have special Pins that are PWN(Pulse Width Modulated) that impersonate an analog pin. We control it like this
analogWrite(actuatorPin, Amount);
actuatorPin is the pin the actuator is on. Amount can be a number between 0 and 255.
myServo.write
Let's use some servos.
myServo.write(Val);
First we tell what servo, in this case myServo. Then we say write with a value between 0 and 180. and just like that your moving servos.
Step 4: Debug
Its vary important to be able to debug a script. The way to do this is by setting up a serial connection with the Arduino then having it tell when it gets to certain points. You open the Serial monitor by going into the IDE and pressing the button in the upper right with the magnifying glass on it.
Serial.begin
first step would be starting our Serial connection like this
Serial.begin(9600);
This goes in Void setup. The number is the Baud Rate, just leave it at 9600 for now.
Serial.print
Here is how we print in inputs
Serial.print("Hello world"); Serial.print(AsensorVal);
The first one prints Hello World into the serial monitor. The second prints the value of our Analog Input.
Serial.println
Serial.println is the same as Serial.print but it starts a new line with it. So, for example. If I keep printing in 3 with the Serial.print command, it will receive it as 333333333333. Now if we use the Serial.println to print it in, it will recieve it as
3
3
3
get the idea.
Step 5: Example
Here I'll show you a script that shows everything we learned. If you want to build it follow the schematic at the top. The Potentiometer on the left is pot1 and controls the servo, the one on the right it pot2 and controls the brightness of the LED. The Button controls whether the LED is on or off.
#include <Servo.h> Servo myServo; // Registering our Servo int servoPin = 9; // The Pin our Servo is on int lightPin = 3; // The Pin our LED is on int potPin1 = A1; // The Pin our Potentiometer that controls the Servo is on int potPin2 = A0; // The Pin our Potentiometer that controls the LED is on int buttPin = 6; // The Pin our Button is on void setup(){ myServo.attach(servoPin); // Attaching our servo pinMode(lightPin, OUTPUT); // Registering our LED Pin as a Output pinMode(potPin1, INPUT); // Registering our Servo Potentiometer Pin as a Input pinMode(potPin2, INPUT); // Registering our LED Potentiomerer Pin as a Input pinMode(buttPin, INPUT); // Registering our Button Pin as a Input Serial.begin(9600); // Starts the Serial communacation } void loop(){ int pot1Val = analogRead(potPin1); // Gets Pot1's current value pot1Val = map(pot1Val, 200, 823, 0, 180); // Mapping it so that it removes the beginning and the end of the Pots range pot1Val = constrain(pot1Val, 0, 180); // Insures that the Servo Input stays between 0 and 180 myServo.write(pot1Val); // Makes the servo move to the current location Serial.print("Pot1 value is: "); // Starts printing in Debug Info Serial.println(pot1Val); // It's now set up so it will say "Pot1 value is: (Current Value)" int pot2Val = analogRead(potPin2); // Gets Pot2's current value pot2Val = map(pot2Val, 0, 1023, 0, 255); // Maps it accordingly Serial.print("Pot2 value is: "); // starts printing in Debug Info Serial.println(pot2Val); // It will say "Pot2 value is: (Current Value)" int button = digitalRead(buttPin); // Gets the state of the Button if(button == 1){ // The way the buttons is set up, 1 means it is being pressed analogWrite(lightPin, pot2Val); // If its true then turn on the led at this brightness } else{ // Else digitalWrite(lightPin, LOW); // If ELSE is true, which means IF is false, then turn off led } delay(50); // Makes a small delay so it isn't running every millisecond. }
Step 6: Thanks
Without further ado i must bid you good bye and thanks for reading. I hope the information in here made sense.
If you have any questions please ask in the comments below, if you have any suggestions either comment ||(or) message me at my instrucatables account.
Please vote for me in the Sensors Contest
It would be much appreciated and vary encouraging.
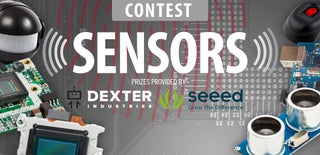
Participated in the
Sensors Contest 2016