Introduction: IBeacon Bluetooth BLE Geocache Hide
I have been a Geocacher for over 10 years and I love to make interesting geocache containers. What is Geocaching you ask? Imagine a treasure hunt but on a planetary scale (even Space!) and involving millions of people. Every day, people hide and find containers or physical locations world-wide after being given a set of coordinates and using the Global Positioning System (GPS). You can find out more by searching the word Geocaching.
I wanted to make a Beacon Cache and place it in my office to protect and power it, but also make it accessible to everyone with a Bluetooth enabled device. Since Bluetooth can transmit to over 60 feet away to a device, placing the completed project on my office windowsill - overlooking a parking lot - would be just the ticket.
Using seven BLE modules is truly overkill for most Beacon Geocaches. You can easily use just one BLE module, change the broadcast name to some GPS coordinates and be done with it. However, I am adding a secret set of GPS coordinates to the end of every day's name so seven it is, for now. Once the secret location is found, I will probably drop this hide down to one BLE module.
Although this Instructable uses the HC-05 Bluetooth modules, I will replace these with the HM-10 BLE devices. You cannot use HC-05 modules for a Geocaching Beacon Cache since iOS does not recognize its protocols. Beacon Geocaches must be open to all operating systems.
Things you will need:
- Arduino-based microcontroller ( Mini Pro 5V used for this project - an Arduino Uno was used to program the RTC, Pro-Mini and HM-10 modules)
- Real-Time Clock Module (DS3231 AT24C32 IIC Real Time Clock used for this project)
- Breadboard
- Through-hole header pins
- 7) HM-10 BLE Bluetooth Modules
- Male-Female jumper wires
- Soldering station and basic soldering skills
- Small wires (I used mostly 30 Ga. wire-wrapping wire)
- Spare USB cable you can sacrifice to make an easy-to-connect power source to your computer or power supply.
- Rubber pads - about 4
Step 1: Wiring the Beacon Cache
Placement of the seven BLE modules on the perf board is fairly straightforward. Only one module will be in operation every day and it will use less than 20 mA of current. This is good because the Arduino Pro Mini can provide no more than 40 mA per Digital Output (DO) pin. I am using the DO pins # 2 through 8 to sequence each BLE module per day. Sunday = Pin #2, Monday = Pin #3, Tuesday = Pin #4, etc... I wanted to be able to take each BLE module in and out as needed for programming so sockets were used to provide mounting points. Only VCC and GND is needed per module after programming, so all the GND pins were tied together down to a common rail and this is attached to one GND point on the Pro Mini. Each BLE's VCC pin was assigned a DO pin from the Arduino.
I used 30 gauge wire wrapping wire for the power and most of the board connections. I did not have any black or green covered wire and only a little bit of red covered wire on hand so the wiring color code is not accurate but acceptable for my project. This wire is thin, flexible and very easy to solder as it tin-plated. Wire wrapping seems to be a lost art and I am surprised more people don't use it on projects in general. Wire wrapping makes great connections that are better than soldering.
After programming, the RTC clock will only need the VCC and GND from a modified USB cable to keep updating the Mini Pro. Even if power is lost, the CR2302 battery on the RTC should last for up to a year to keep the time up to date. The Mini Pro needs VCC, GND and Analog Output (AO) pins # 2 and 3 connected to the RTC's Serial Data (SDA) and Serial Clock (SCL) pins, respectively, so it keeps track of the day of the week and turns on the correct BLE module at midnight and turns off the previous day's BLE module.
Step 2: Programming the BLE Modules
As stated before, the HC-05 BLE modules are only being used for demonstration purposes. HM-10 modules will be replacing these.The pin locations are the same between both module series.
Programming the HC-05 modules require a computer, USB A to B cable, the Arduino IDE and a suitable Arduino. I used an Uno since it was laying around my office. Connect the Arduino Uno's 5V, GND, Analog Pin # 2 and #4 to the BLE module's VCC, GND, TX and RX respectfully. The program I used is part of a great Instructable from Hazim Bitar found here:
/* AUTHOR: Hazim Bitar (techbitar) DATE: Aug 29, 2013 LICENSE: Public domain (use at your own risk) CONTACT: techbitar at gmail dot com (techbitar.com) */ #include SoftwareSerial BTSerial(4, 2); // RX | TX void setup()<br>{ pinMode(9, OUTPUT); // this pin will pull the HC-05 pin 34 (or key pin) HIGH to switch module to AT mode digitalWrite(9, HIGH); Serial.begin(9600); Serial.println("Enter AT commands:"); BTSerial.begin(38400); // HC-05 default speed in AT command more } void loop() { // Keep reading from HC-05 and send to Arduino Serial Monitor if (BTSerial.available()) Serial.write(BTSerial.read()); }
Pin #4 (RX) goes from the Arduino to the BLE module's TX line. Pin #2 (TX) should go though a voltage divider to the BLE module's RX line. Holding the BLE Reset button down when applying 5 Volts to the module puts it into programming mode and resets any previous changes made to it. You can use the pin #9 reset connection as well by adding a jumper from the Arduino DO Pin #9 to the KEY pin of the BLE module.
Remember to set your baud rate to match the BLE module.
You are limited to a 32 length name for each module, but you can make it fit. I am able to code in the GPS coordinates and add the bonus set of GPS numbers for the mystery cache location. If you get a
code (3) error, you have too many letters in the name. It's a tight fit, but it works!
Step 3: Programming the Arduino Mini Pro / RTC
Programming the Mini Pro was easily done by modifying existing Arduino code and the DS2312 library found at Rinky-Dink Electronics
http://www.rinkydinkelectronics
Download DS3231 from the Libraries and unzip the necessary files into your Arduino folder(s).
I had to change where the Mini Pro connected to the RTC module to Analog pins # A2 and A3. I also added lines of code to change the Digital Output (DO) pins from LOW to HIGH as a new day of the week started. Programming the initial Day of the Week (rtc.setDOW(FRIDAY) uses all caps. However, the Arduino has to be searching for Friday. Note lower case conditions to make the correct data string match in the example below.
Remember: you have to program twice! Once to set the current date and time to the RTC, and then comment out the date/time setting commands and re-flash the Arduino. If you don't, the RTC will revert back to old dates and times.
// DS3231_Serial_Easy<br>// Copyright (C)2015 Rinky-Dink Electronics, Henning Karlsen. All right reserved // web: <a href="http://www.RinkyDinkElectronics.com/" rel="nofollow"> http://www.RinkyDinkElectronics.com/ </a> // // A quick demo of how to use my DS3231-library to // quickly send time and date information over a serial link // // To use the hardware I2C (TWI) interface of the Arduino you must connect // the pins as follows: // // Arduino Uno/2009: // ---------------------- // DS3231: SDA pin -> Arduino Analog 4 or the dedicated SDA pin // SCL pin -> Arduino Analog 5 or the dedicated SCL pin // // Arduino Leonardo: // ---------------------- // DS3231: SDA pin -> Arduino Digital 2 or the dedicated SDA pin // SCL pin -> Arduino Digital 3 or the dedicated SCL pin // // Arduino Mega: // ---------------------- // DS3231: SDA pin -> Arduino Digital 20 (SDA) or the dedicated SDA pin // SCL pin -> Arduino Digital 21 (SCL) or the dedicated SCL pin // // Arduino Due: // ---------------------- // DS3231: SDA pin -> Arduino Digital 20 (SDA) or the dedicated SDA1 (Digital 70) pin // SCL pin -> Arduino Digital 21 (SCL) or the dedicated SCL1 (Digital 71) pin // // The internal pull-up resistors will be activated when using the // hardware I2C interfaces. // // You can connect the DS3231 to any available pin but if you use any // other than what is described above the library will fall back to // a software-based, TWI-like protocol which will require exclusive access // to the pins used, and you will also have to use appropriate, external // pull-up resistors on the data and clock signals. // #include String DayofWeek; // Init the DS3231 using the hardware interface DS3231 rtc(A3, A2); // (SDA & SCL) void setup() { // Setup Serial connection Serial.begin(115200); pinMode(2, OUTPUT); pinMode(3, OUTPUT); pinMode(4, OUTPUT); pinMode(5, OUTPUT); pinMode(6, OUTPUT); pinMode(7, OUTPUT); pinMode(8, OUTPUT); // Initialize the rtc object rtc.begin(); // The following lines can be uncommented to set the date and time // rtc.setDOW(FRIDAY); // Set Day-of-Week to FRIDAY //rtc.setTime(14, 55, 00); // Set the time to 14:55:00 (24hr format) // rtc.setDate(5, 11, 2018); // Set the date to May 11th, 2018 } void loop(){ // Send Day-of-Week // Serial.print(rtc.getDOWStr()); // Serial.print(" "); DayofWeek = (rtc.getDOWStr()); // delay (200); // Send date // Serial.print(rtc.getDateStr()); // Serial.print(" -- "); // Send time // Serial.println(rtc.getTimeStr()); if (DayofWeek == "Sunday") { digitalWrite(8, LOW); delay (20); digitalWrite(2, HIGH); } if (DayofWeek == "Monday") { digitalWrite(2, LOW); delay (20); digitalWrite(3, HIGH); } if (DayofWeek == "Tuesday") { digitalWrite(3, LOW); delay (20); digitalWrite(4, HIGH); } if (DayofWeek == "Wednesday") { digitalWrite(4, LOW); delay (20); digitalWrite(5, HIGH); } if (DayofWeek == "Thursday") { digitalWrite(5, LOW); delay (20); digitalWrite(6, HIGH); } if (DayofWeek == "Friday") { digitalWrite(6, LOW); delay (20); digitalWrite(7, HIGH); } if (DayofWeek == "Saturday") { digitalWrite(7, LOW); delay (2); digitalWrite(8, HIGH); } }
Step 4: Reading the Bluetooth Address
This is the fun part where I test each BLE module to see if I have coded the name correctly. It works! It was over 80 feet away down a hallway and I could still find it on my flip phone!
Step 5: Final Deployment to My Window and to Geocaching.com
After testing everything and making one final check of the BLE modules, I set the unit up on my office windowsill. My office faces a small parking lot and there is plenty of room to walk up, bike up or (very temporarily) park to get the Bluetooth data. I had a range of over 60 feet away from my window and using my flip phone. I still have to change the BLE modules out when they arrive from eBay but this will be a minor programming change and setup. I hope this encourages other to make beacon caches. I can imagine hiding a lot of these with 3.3 V lithium batteries and the the low-power draw BLE modules out in the woods.
Now to publish my Geocache! GC79Y51 will be live soon!
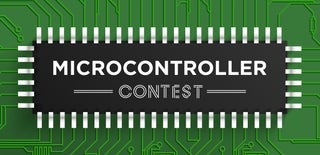
Participated in the
Microcontroller Contest