Introduction: Javascript Generated Laser Cut Jewellery
Javascript can be used to generate Scalable Vector Graphics (SVGs) that can be converted into DXF files and cut on a laser cutter. Using simple geometry you can make jewellery such a pendants and earrings. Math Rocks!
There are plenty of javascript libraries that can help with building of SVG. The most populare ones that I have found are http://raphaeljs.com/ and http://d3js.org/. Any library that can export to SVG would work. In this tutorial we will be using raphael.js
You will need a basic understanding of javascript to follow along with this tutorial. If you have never touched javascript before, I would suggest you start with Mozilla getting started with javascript articles.
Step 1: Download the Required Libraries and Tools
- http://raphaeljs.com/ - The main graphics libary
- https://github.com/ElbertF/Raphael.Export - This plugin for raphael.js will help with the downloading of the SVG file from the Canvas.
- http://www.inkscape.org/en/- SVG Vector editor that can be used to clean up the SVG file.
If you have never used raphael.js, before you may wish to look at the startup guide and get familiar with the library first. Raphael.js is well documented and has many code examples
Extract the javascript files into a working directory
Step 2: Create a Html File With the Basic Canvas
Create a index html file and save it into your working directory.
The source code for this step can be found in my Gist account https://gist.github.com/funvill/11351242
Step 3: Define the Unit of Measurement
Raphael.js uses pixels as its unit of measurement. This makes sense as most people use Raphael.js for making graphics on a monitor. Since we are making physical objects we will want to use a unit of measurement that make sense for us. Converting from pixels to centimeters is dependent on your screen resolution and is described here http://www.pixelcalculator.com/. For my 22 inch monitor its approximately 38 pixels per centimeters.
var ONE_CM_IN_PX = 38 ;
Step 4: Define the Brushes
When we are laser cutting our design we will want to cut order some cuts before others. This is normally done by changing the colors of the different lines reordering them in the laser cutter software. In this tutorial we define the black lines to be cut first and the blue lines to be cut second, green lines to be etched.
var BRUSH_CUT_FIRST = {fill: "none", stroke: "#000000", "stroke-width": "1" } ; var BRUSH_CUT_SECOND = {fill: "none", stroke: "#0000FF", "stroke-width": "1" } ; var BRUSH_ETCH = {fill: "#00FF00", stroke: "#00FF00", "stroke-width": "1" } ;
Step 5: Set Up the Canvas
Here we set up the canvas that we are going to use. In this tutorial I made the canvas 15cm by 15cm. We define the the CANVAS_HEIGHT and CANVAS_WIDTH as variables so we can use them later in the script.
// Make a working area that is 15cm x 15cm. var CANVAS_HEIGHT = ONE_CM_IN_PX*15; var CANVAS_WIDTH = ONE_CM_IN_PX*15; // Create the Raphael object to hold the SVG elements. var paper = Raphael(100, 100, CANVAS_WIDTH, CANVAS_HEIGHT);
Step 6: Drawing Circles to the Screen
In this section we have added 4 circles on the canvas using the ellipse function.
Paper.ellipse(x, y, rx, ry)
http://raphaeljs.com/reference.html#Paper.ellipse
Parameters
- x - x coordinate of the centre
- y - y coordinate of the centre
- rx - horizontal radius
- ry - vertical radius
We are drawing the first circle with its center one cm from the top left of the canvas. with a radius of 1 cm.
paper.ellipse( ONE_CM_IN_PX, ONE_CM_IN_PX, ONE_CM_IN_PX, ONE_CM_IN_PX).attr( BRUSH_CUT_FIRST );
The next circle’s center starts 2cm on the x cord and on the same y cords. These two lines will produce two circles that overlap each other.
paper.ellipse( ONE_CM_IN_PX*2, ONE_CM_IN_PX, ONE_CM_IN_PX, ONE_CM_IN_PX).attr( BRUSH_CUT_FIRST );
We add two more circles using the same method.
paper.ellipse( ONE_CM_IN_PX, ONE_CM_IN_PX*2, ONE_CM_IN_PX, ONE_CM_IN_PX).attr( BRUSH_CUT_FIRST ); paper.ellipse( ONE_CM_IN_PX*2, ONE_CM_IN_PX*2, ONE_CM_IN_PX, ONE_CM_IN_PX).attr( BRUSH_CUT_FIRST );
Source code for this step can be found on my Gist
https://gist.github.com/funvill/11351377
Step 7: Make a Helper Function to Draw Circles
If we were to cut this shape as is it would produce 13 different pieces that are not connected to each other. What we want to do it make the circles into thick lines and merge the lines together then cut around the thick lines. We can do this by adding a new custom func
tion.
DrawCircle( x, y, r, size, brush )
Draw two circles, one inside of the other
Parameters:
- X - X Offset to the center of the circle
- Y - Y Offset to the center of the circle
- R - The radius of the circle.
- Size - The size between the two lines of the circle.
- Brush - The brush to use to draw these two circles.
function DrawCircle( x, y, r, size, brush ) { paper.ellipse( x, y, r-(size/2), r-(size/2)).attr( brush ); paper.ellipse( x, y, r+(size/2), r+(size/2)).attr( brush ); }
We can then update our four circles to use this new function.
DrawCircle(ONE_CM_IN_PX+10,ONE_CM_IN_PX+10,ONE_CM_IN_PX,ONE_MM_IN_PX*2,BRUSH_CUT_FIRST); DrawCircle(ONE_CM_IN_PX+10,ONE_CM_IN_PX*2+10,ONE_CM_IN_PX,ONE_MM_IN_PX*2,BRUSH_CUT_FIRST); DrawCircle(ONE_CM_IN_PX*2+10,ONE_CM_IN_PX+10,ONE_CM_IN_PX,ONE_MM_IN_PX*2,BRUSH_CUT_FIRST); DrawCircle(ONE_CM_IN_PX*2+10,ONE_CM_IN_PX*2+10,ONE_CM_IN_PX,ONE_MM_IN_PX*2,BRUSH_CUT_FIRST);
Source for this step
https://gist.github.com/funvill/11351553
Step 8: Make the Download Button
Now we want to make a button on the screen to download the SVG file. I found this question on Stackoverflow to help me make this function to download the SVG file.
function CreateDownloadLink( linkName ) { // Create the download link. var svgString = paper.toSVG(); var a = document.getElementById(linkName); a.download = 'mySvg.svg'; a.type = 'image/svg+xml'; bb = new Blob([svgString]); a.href = (window.URL || webkitURL).createObjectURL(bb); }
Refresh the page and click the download link.
Source code for this step
https://gist.github.com/funvill/11351653
Step 9: Clean It Up in Inkscape.
- Open the SVG file in inkscape
- Select the “Edit path by nodes” tool
- Select the outer circle of one of the pairs of circles. In the image i have highlighted the circle in red.
- From the menu, click “Object” and select “Lower to Bottom”. This will push this circle to the bottom layer. The image will not visually change.
- While holding down the [SHIFT] button select the inner circle. This should select both circles.
- From the menu click “Path” then “Difference.
- From the path menu click ‘differnce’. This will cut the smaller circle out of the center of the larger circle, creating a ring. I have highlighted the ring in red.
- Repeat the process for the other three sets of circles. I have color the circles all differently.
- This made all the circles join each other into a single object.
- While the entire image is selected, from the menu click “Path” then “Break Apart” This will split the different sections of the image in smaller sub sections.
- Select the outline and change its color to a different color from the rest. This will help you when you are setting up your laser cutter order of cuts.
- You can now export the svg as a DFX file ready for your laser cutter.
Step 10: Play Around With the Script
At this point you should have the basics to start playing around to make more advanced jewellery. This example shows how to make a snowflake https://gist.github.com/funvill/11352179
Post your results !
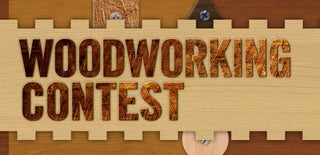
Participated in the
Woodworking Contest
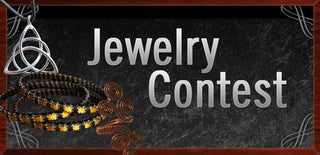
Participated in the
Jewelry Contest
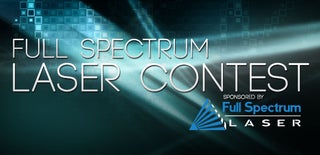
Participated in the
Full Spectrum Laser Contest