Introduction: Piano Safe With Melody 'key'
A box that only opens when you play the right melody.
Why?
In The Netherlands it is a known custom to celebrate 'Sinterklaas' on the 5th of December. When children are still young, Sinterklaas will come with his friends to bring presents. When the kids get bigger, this tradition transforms in a different variant. Everybody will secretly pull one other person and provide a present for this person. (something like secret Santa?!) The present itself is normally something cheap, like some nice chocolate, but the most important thing is the packaging.
You are supposed to build something big, strange or special that says something about the person you are building it for, and put the present inside. (You are also supposed to write a cheesy poem about that person.)
The person that I had to make a present for, is a great pianist, so that is why I made this piano-safe.
How it will work:
I'll put the present in the piano. The piano will automatically open when you play a certain melody on the piano, so you can get the present.
When you play something else, the piano will close again.
Step 1: You Will Need
For the box
Materials:
- Scraps of ply
- Screws
- M4 treated wire
- Nuts and Bolts
- Wire
Tools:
- 3D printer for the keys and hinges (or make them any way you like)
- Saw
- Drill
- Screwdriver
- Sanding paper
- Pliers
- Wood glue
- Hot glue
For the electronics
Materials:
- 8 (or more) push buttons
- 8 (or more) 300 Ohm (or something close) resistors
- 2 100 Ohm resistors
- Small servo
- Wire
- Arduino NANO (Works the same with an UNO or Pro or whatever you have)
- Piezo
- Battery (box)
- on/off switch
Tools:
- Soldering stuff
Step 2: Breadboarding the Electronics
To get a lot of buttons on only one (analog) port, I used this clever method that I found on the internet. (thanx SVGLOBE.COM)
There are many tricks to put more buttons on few ports, but this one worked perfectly for my 8 buttons.
By connecting one site of the buttons together and separating the other side with resistors, the analog value on the analog pin on the Arduino will vary according to the button that is pushed. (this works only when only one button is pushed at the same time)
Before soldering it all together, I first tried it on a breadboard.
Just connect the buttons, resistors, servo and piezo as is shown on the picture.
Step 3: Add the Code
To get the code working was harder than I thought. I choose a traditional 'Sinterklaas' song to open the box and that song has repeating tones in it what didn't work for the first code I wrote.
The opening melody for my box is gcceddFbbdc.
You need to understand some Arduino coding if you want to change this in to your own melody. It is not a long or difficult sketch so you probably will manage if you have just a little experience with an Arduino.
The Sketch:
// gcceddFbbdc 25576684465
#include
Servo myservo;
int buttonPin = A0;
int button = 0;
int piezo = 3;
int toon = 0; // f=1 g=2 a=3 b=4 c=5 d=6 e=7 F=8
int volgende = true;
int teller = 1;
void setup() {
// Serial.begin(9600); //debugging
pinMode(13, OUTPUT);
myservo.attach(9);
myservo.writeMicroseconds(1000); }
void loop() {
delay(30); //debounce
button = analogRead(buttonPin);
//Serial.print(toon);
//Serial.print(volgende);
//Serial.println(button); //debugging
if (button > 400){tone(piezo, 349); toon=8; volgende=false;} // F
else {if (button > 150){tone(piezo, 330); toon=7; volgende=false;} // e
else {if (button > 100){tone(piezo, 294); toon=6; volgende=false;} // d
else{if (button > 80){tone(piezo, 262); toon=5; volgende=false;} // c
else{if (button > 60){tone(piezo, 247); toon=4; volgende=false;} // b
else{if (button > 48){tone(piezo, 220); toon=3; volgende=false;} // a
else{if (button > 38){tone(piezo, 196); toon=2; volgende=false;} // g
else{if (button > 20){tone(piezo, 175); toon=1; volgende=false;} // f
else{noTone(piezo); volgende=true;}}}}}}}}
if (teller == 1 && toon == 2 && volgende == true){teller = 2; volgende = false;}
if (teller == 2 && toon == 5 && volgende == true){teller = 3; volgende = false;} else{if(volgende == true && teller == 2 && toon != 2){teller = 1;}}
if (teller == 3 && toon == 5 && volgende == true){teller = 4; volgende = false;} else{if(volgende == true && teller == 3 && toon != 2){teller = 1;}}
if (teller == 4 && toon == 7 && volgende == true){teller = 5; volgende = false;} else{if(volgende == true && teller == 4 && toon != 7 && toon != 5){teller = 1;}}
if (teller == 5 && toon == 6 && volgende == true){teller = 6; volgende = false;} else{if(volgende == true && teller == 5 && toon != 7){teller = 1;}}
if (teller == 6 && toon == 6 && volgende == true){teller = 7; volgende = false;} else{if(volgende == true && teller == 6 && toon != 7){teller = 1;}}
if (teller == 7 && toon == 8 && volgende == true){teller = 8; volgende = false;} else{if(volgende == true && teller == 7 && toon != 6 && toon != 8){teller = 1;}}
if (teller == 8 && toon == 4 && volgende == true){teller = 9; volgende = false;} else{if(volgende == true && teller == 8 && toon != 8){teller = 1;}}
if (teller == 9 && toon == 4 && volgende == true){teller = 10; volgende = false;} else{if(volgende == true && teller == 9 && toon != 8){teller = 1;}}
if (teller == 10 && toon == 6 && volgende == true){teller = 11; volgende = false;} else{if(volgende == true && teller == 10 && toon != 4){teller = 1;}}
if (teller == 11 && toon == 5 && volgende == true){teller = 12; volgende = false;} else{if(volgende == true && teller == 11 && toon != 6){teller = 1;}}
if(teller == 12 && volgende == true){ teller = 13; digitalWrite(13, HIGH); myservo.writeMicroseconds(2000); delay(5000);} //2000 is the value for the open servo
if(teller == 13 && volgende == true && toon != 5){ digitalWrite(13, LOW); teller = 1; myservo.writeMicroseconds(1000);} //1000 is the value for the closed servo
}
Step 4: Printing the Piano Keys
I designed a simple piano-key to print for this project. (I also printed the hinges, because I was to lazy to go to the store and buy some)
123D-Design is still my favorite program to design for the 3D-printer.
I also added the 123D-files so you can change things if you want.
Step 5: Making the Box
The shape that this box ended up having, has a couple of reasons:
- the materials I had
- the present that I had to fit in
- it has to look a bit like a piano
- my skills and time
You can make this as nice and as difficult as you want. For me it had to stay cheap, quick and simple.
So I just glued, and the dimensions are mostly the sizes that the scrap ply-wood already had.
I thought about painting it black, but.... no.
Step 6: Fitting the Buttons
First I marked where the buttons will come.
I have more buttons than will actually work. So some are just for show. (It would not be hard to get them all working, but I didn't have enough push-buttons)
Bend out the leads of the push buttons and glue them in place with hot-glue.
Bend, cut and solder the resistors in place.
Bend, cut and solder the connecting wires on the other side of the buttons.
Solder wires to go to the +5V, GND and analog port of your Arduino.
Step 7: Finish the Electronics
Now you can solder the buttons, servo, piezo and battery-supply to the Arduino NANO.
I also added an on/off switch between the battery and the VIN port of the Arduino, so you can switch the piano off and safe some battery life.
When all the electronics are in place, you can put the piano-keys in, by pushing a piece of M4 wire trough all the keys and two holes in the sides of the box to hold them in place.
Now you can 'test run' the piano again.
With everything still accessible it is still easy to fix some mistakes that you may have made. (I always do)
Step 8: Fitting the Mechanics
Mark where you want the servo and the on-off switch.
Cut a slot to fit the servo and one to fit the switch.
Glue the servo and switch in place with hot-glue.
Bend a wire that will push open the lid of the box.
Put the wire through one eye in the servo.
Drill a small hole in a small piece of ply, where the other side of the push-wire will go trough.
Put the wire trough the hole in the ply and keep it in the position where it should be when the box is closed. (make sure that the servo is also in the closed position)
Put some glue on the ply piece and put the lid on the box.
If you did it right, the piece of ply is now glued to the lid of the box.
Wait for the glue to dry.
Screw the hinges on.
Step 9: Surprise
Your box should be ready now.
Add a present in the box.
Write a epic poem that makes clear that you should play a melody to open the box and let everyone be amazed.
Have fun!
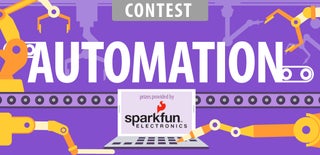
Runner Up in the
Automation Contest 2016
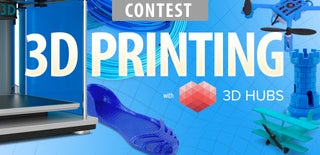
Participated in the
3D Printing Contest 2016