Introduction: Raspberry PI & Arduino - Blynk Stepper Control
This tutorial will show you how to control a stepper motor with an Arduino, a Raspberry Pi and the Blynk Application.
In a nut shell, the app sends requests to the Raspberry Pi via Virtual Pins, the Pi then sends HIGH/LOW signal to the Arduino and the Arduino then deals with the stepper motor.
I think that it is easier to use that approch since most people are used to work with Arduino and not a lot with node.js on the Raspberry Pi.
Components needed for this :
- Raspberry PI ( I am using the Raspberry Pi 3 model b )
- Arduino ( I am using an Arduino Nano )
- Servo Motor ( I am using a 28BYJ-48 5VDC with its controller )
- Some jumper wires
- Power source (5VDC 2A.)
The Arduino sketch and the Node.js code is available to download. Just look up the files.
Attachments
Step 1: Creating the App on Your Phone
Download Blynk from the AppStore or GooglePlay
Open the App and create a user or Log In with facebook.
- Create a new project
Name your project : MyProject
Choose Device : Rasapberry Pi 3 B
Connection Type : Wifi (or Ethernet if your Pi is wired to your network)
- Click Create
Check your email for your Token
(looks like this 3aa19bb8a9e64c90af11e3f6b0595b3c)
This Token is bonded with your current app. If you do another app, you will generate another token.
In the App add the following widgets (see picture)
- Add 3 buttons
- Add 1 LCD
- Edit the button
name the first one Command1, set the Pin as Virtual Pin 1 and set the Mode as SWITCH
name the second one CW, set the Pin as Virtual Pin 2 and set the Mode as PUSH
name the third one CCW, set the Pin as Virtual Pin 3 and set the Mode as PUSH
- Edit the LCD
set the Pins as Virtual Pin 4 and Virtual Pin 5 and set the Mode to PUSH
Step 2: Getting the PI Ready
First, you need to install Node.js.
Before updating Node.js, please be sure to remove old versions:
Open Terminal and type
sudo apt-get purge node nodejs
node.js -ysudo apt-get autoremove
Automatic Node.js installation Add repositories:
curl -sL https://deb.nodesource.com/setup_6.x | sudo -E bash -
Install Node.js
sudo apt-get update && sudo apt-get upgrade
sudo apt-get install build-essential nodejs -y
Once Node.js is installed, Install Blynk
sudo npm install blynk-library -g
sudo npm install onoff -g
Step 3: Creating Your Project
Start by changing directory (cd command) to the pi directory
Open Terminal and type:
cd /home/pi/
Then, create a directory where your project will reside
mkdir MyProject
Change directory to MyProject, type the following in the Terminal
cd MyProject
Check the content of the directory (it should be empty). Just type the following in the Terminal
ls
Next, type the following to create your project description (package.json)
npm init
Just type the project name, author, version, etc...
Once this is done, install the Blynk library, the onoff library and the system-sleep library in YOUR project directory. Type the following in your Terminal
npm install blynk-library --save
npm install onoff --save
npm install system-sleep --save
Finally, create your .js file (This will be where your code will reside). Type the following in the Terminal
nano MyProject.js
Once you execute that command, nano (terminal text editor) will open.
Step 4: MyProject.js
In nano, write the following lines of code
var Blynk = require('blynk-library');
var AUTH = ' ****************** '; THIS IS YOUR TOKEN
var blynk = new Blynk.Blynk(AUTH);
var Gpio = require('onoff').Gpio,
command1 = new Gpio(18, 'out'), //Will be connected to Arduino D2
commandCW = new Gpio(23, 'out'), //Will be connected to Arduino D3
commandCCW = new Gpio(24, 'out'); //Will be connected to Arduino D4
var sleep = require('system-sleep');
var v1 = new blynk.VirtualPin(1); //this is your Command1 button in the app
var v2 = new blynk.VirtualPin(2); //this is your CW button in the app
var v3 = new blynk.VirtualPin(3); //this is your CCW button in the app
var v4 = new blynk.VirtualPin(4); //this is your LCD line 1 in the app
var v5 = new blynk.VirtualPin(5); //this is your LCD line 2 in the app
v1.on('write', function (param) //Check for the Command1 button in the app
{
if (param == 1) //If the button is pressed (which is 1) then do the following
{
v4.write("Executing"); //Write "Executing" on the first line of the LCD
v5.write("Command"); //Write "Command" on the second line of the LCD
command1.writeSync(1); //Set the GPIO18 (which is variable command1) to 1 (HIGH)
sleep(4000); //Wait for 4 seconds
command1.writeSync(0); //Set the GPIO18 (which is variable command1) to 0 (LOW)
v4.write("Done"); //Write "Done" on the first line of the LCD
v5.write(" "); //Write " " (nothing) on the second line of the LCD
v1.write(0); //Write 0 to your Command1 button, that will reset it to the OFF position
}
});
v2.on('write', function (param) //Check for the CW button in the app
{
if (param == 1) //If the button is pressed (which is 1) then do the following
{
commandCW.writeSync(1); //Set the GPIO23 (which is variable commandCW) to 1 (HIGH)
}
else if (param == 0) //If the button not pressed (which is 0) then do the following
{
commadCW.writeSync(0); //Set the GPIO23 (which is variable commandCW) to 0 (LOW)
}
});
v3.on('write', function (param) //Check for the CCW button in the app
{
if (param == 1) //If the button is pressed (which is 1) then do the following
{
commandCCW.writeSync(1); //Set the GPIO24 (which is variable commandCCW) to 1 (HIGH)
}
else if (param == 0) //If the button not pressed (which is 0) then do the following
{
commandCCW.writeSync(0); //Set the GPIO24 (which is variable commandCCW) to 1 (HIGH)
}
});
------------------------------------------------------------------------------------------------------------------------------------------------------
Save it and exit nano
- to save CTRL+O
- to quit CTRL+X
You are done with the Raspberry Pi.
Now test it to see if have any kind of errors (most of the time are Typo errors)
To test it, simply type the following in your Terminal
node MyProject.js
You should get an output that looks like this
OnOff mode
Connecting to: blynk-cloud.com 8441
SSL authorization...
Authorized
Step 5: MyProject in Arduino
Ok so now we have 2/3 things completed!
Now we just need to write some code for the Arduino.
- Create a new Arduino sketch and type the following code.
#include
#define STEPS_PER_MOTOR_REVOLUTION 32
#define STEPS_PER_OUTPUT_REVOLUTION 32 * 64 //2048
//The pin connections need to be pins 8,9,10,11 connected
// to Motor Driver In1, In2, In3, In4
// Then the pins are entered here in the sequence 1-3-2-4 for proper sequencing
Stepper small_stepper(STEPS_PER_MOTOR_REVOLUTION, 8, 10, 9, 11);
int Steps2Take;
int Command1;
int CommandCW;
int CommandCCW;
void setup()
{
pinMode(2, INPUT);
pinMode(3, INPUT);
pinMode(4, INPUT);
//(Stepper Library sets pins as outputs)
}
void loop()
{
Command1 = digitalRead(2);
CommandCW = digitalRead(3);
CommandCCW = digitalRead(4);
if(Command1 == 0)
{
//do nothing
}
else
{
ExecutionFunction();
}
if(CommandCW == 1)
{
small_stepper.setSpeed(700);
small_stepper.step(-1);
delay(1);
}
if(CommandCCW ==1)
{
small_stepper.setSpeed(700);
small_stepper.step(1);
delay(1);
}
}
void ExecutionFunction()
{
Steps2Take = STEPS_PER_OUTPUT_REVOLUTION / 4 ; // Rotate CCW 1/4 turn
small_stepper.setSpeed(700);
small_stepper.step(Steps2Take); // You can replace Steps2Take with any value between 0 and 2048
delay(500);
Steps2Take = - STEPS_PER_OUTPUT_REVOLUTION / 4; // Rotate CW 1/4 turn
small_stepper.setSpeed(700);
small_stepper.step(Steps2Take); // You can replace Steps2Take with any value between 0 and 2048
delay(2000);
}
Compile and upload to your Arduino.
Now make sure you connect everything correctly! See the next step for wiring.
Step 6: Wiring
Connect Arduino D3 to RaspberryPi GPIO18 (which is actually pin 12)
Connect Arduino D4 to RaspberryPi GPIO23 (which is actually pin 16)
Connect Arduino D4 to RaspberryPi GPIO24 (which is actually pin 18)
Connect Arduino GND to RaspberryPi GND (pin 6)
Connect Arduino D8 to Stepper Controller In1
Connect Arduino D9 to Stepper Controller In2
Connect Arduino D10 to Stepper Controller In3
Connect Arduino D11 to Stepper Controller In4
Connect 5VDC to Arduino, Raspberry Pi and Stepper Controller
Step 7: That's It!
Check the video, and you should be finished!
Thanks and enjoy!
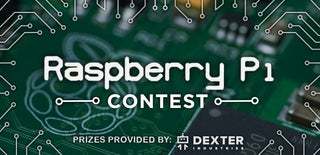
Participated in the
Raspberry Pi Contest 2017