Introduction: Smart Holster: Hall Sensor and GPS Sensor for Geo Location
The following instructable describes how to connect your Intel Edison using the Grove GPS sensor, Hall sensor, LED, button, and the Base Shield. The data is then captured an uploaded to the could using Firebase.
Please note that the Grove GPS does not come standard in the Gove Starter Kit Plus.
Step 1: Hardware Needed:
- Intel Edison chip (Included in Grover Starter Kit Plus)
- Arduino Expansion board (Included in Grover Starter Kit Plus)
- Base Shield (Included in Grover Starter Kit Plus)
- Grove GPS sensor
Step 2: Software Requirements
-Eclipse C++ (https://software.intel.com/en-us/articles/install-eclipse-ide-on-intel-iot-platforms)
- Drivers (list for various OS https://software.intel.com/en-us/installing-drive...
-Tool Lite (view installation for OS https://software.intel.com/en-us/using-flash-tool...
-Intel XDK
By now you should be familiar with your Arduino Expansion board parts.
To refresh your memory please visit http://www.intel.com/support/edison/breakout-bd/sb/CS-035653.htm
Step 3: Assemble of Board
If this is your first using your Arduino Expansion board and Intel Edison please follow the ‘Assembling the Intel® Edison board with the Arduino expansion board’ tutorial to get your board assembled and ready for use. -Assembling the Intel® Edison board with the Arduino expansion board https://software.intel.com/en-us/assembling-intel...
-Driver installation and updating firmware (enter link https://software.intel.com/en-us/installing-drive... -Updating firmware with ToolLite https://software.intel.com/en-us/installing-drive...
-Flashing for mac https://software.intel.com/en-us/installing-drive... Once you successfully updated your firmware in a terminal follow the next steps:
~# screen /dev/tty.usbserial 115200 -L
~# root (no password) ~# configure_edison --setup
You will now be prompted to name your Edison. Please ensure that your name is unique as there might be a conflict if the name given to the board is the same as that of another user.Make sure that both the machine you are using to connect to your Edison is using the same network (wifi) as your Edison. If you run into any issues with your wifi and need to re configure it please follow the next steps:
~# configure_edison --wifi
o verify that wifi connectivity is working try pinging a familiar web page such as google.com Also, from another terminal outside your Edison terminal try pinging your the Edison IP. The IP can be found by running ifconfig from within your Edison terminal.
~# ping <ip>
Step 4: Creating Your Project in Eclipse C++
1. Launch your Eclipse C++ and Navigate to “File > New > Intel IoT C/C++ project”
2. Enter a name for your project (note no spaces are allowed).
Step 5: Getting the Code Ready
Below is a snipet of the code used.Please note that there is also an LED and button being used in the code along with integration with FireBase.
Full code for the GPS, Hall sensor and LEDs its at https://github.com/tsmilesagain/safety_holster
#include "mraa.hpp"
#include "UdpClient.hpp"
#include <grove.h>
#include <signal.h>
#include <ublox6.h>
#include <a110x.h>
#include <stdio.h>
#include <curl/curl.h>
#include <iostream>
#include <sstream>
#include<string>
using namespace upm; using namespace std;
const size_t bufferLength = 256;
define NODE "localhost"
#define SERVICE "41234"
#define COMP_NAME "temperature"
int main() {
// Create the Grove LED object using GPIO pin 4
upm::GroveLed* ledRed = new upm::GroveLed(4);
upm::GroveLed* ledGreen = new upm::GroveLed(3);
// create an analog input object from MRAA using pin A0
mraa::Aio* a_pin = new mraa::Aio(0);
// Create the button object using GPIO pin 8
upm::GroveButton* button = new upm::GroveButton(8);
// Instantiate a Ublox6 GPS device on uart 0.
upm::Ublox6* nmea = new upm::Ublox6(0);
int gunDrawn = 100;
int magFieldAvg = 0;
int magFieldCurrent = 0;
int magField[10];
int tempIndex = 0;
int numSamples = 2;
string tempData;
// check that we are running on Galileo or Edison
mraa_platform_t platform = mraa_get_platform_type();
if ((platform != MRAA_INTEL_GALILEO_GEN1) && (platform != MRAA_INTEL_GALILEO_GEN2) && (platform != MRAA_INTEL_EDISON_FAB_C))
{ std::cerr << "Unsupported platform, exiting" << std::endl;
return MRAA_ERROR_INVALID_PLATFORM; }
// Read in hall sensor data
if (a_pin == NULL)
{ std::cerr << "Can't create mraa::Aio object, exiting" << std::endl;
return MRAA_ERROR_UNSPECIFIED; }
// GPS Setup
// make sure port is initialized properly. 9600 baud is the default.
if (!nmea->setupTty(B9600))
{ cerr << "Failed to setup tty port parameters" << endl;
return 1; }
// Curl setup
//followed this curl example: http://curl.haxx.se/libcurl/c/http-post.html
CURL *curl;
CURLcode res;
// In windows, this will init the winsock stuff
curl_global_init(CURL_GLOBAL_ALL);
// get a curl handle
curl = curl_easy_init();
// First set the URL that is about to receive our POST. This URL can
// just as well be a http://curl.haxx.se/libcurl/c/http-post.html if that is what should receive the
// data.
curl_easy_setopt(curl, CURLOPT_URL, "https://flickering-inferno-5440.firebaseio.com/data.json");
//this is only intended to collect NMEA data and not process it
// should see output on console
char nmeaBuffer[bufferLength];
while(1)
{ uint16_t pin_value = a_pin->read();
magFieldAvg = 0;
magField[magFieldCurrent++] = pin_value;
if (magFieldCurrent >= numSamples)
{ magFieldCurrent = 0; }
for (int i = 0;i < numSamples; i++
{magFieldAvg += magField[i]; }
magFieldAvg /= numSamples;
sleep(1);
if(magFieldAvg < gunDrawn)
{ ledRed->off();
ledGreen->on(); }
else {
if (nmea->dataAvailable()) {
int rv = nmea->readData(nmeaBuffer, bufferLength);
if (rv > 0) {
write(1, nmeaBuffer, rv);
std::cout << nmeaBuffer << std::endl; }
else {
// some sort of read error occurred
cerr << "Port read error." << endl;
break;
}
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "{\"gunDrawn\":\"true\"}");
// Perform the request, res will get the return code
res = curl_easy_perform(curl);
// Check for errors
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
// add some LEDs
ledRed->on();
ledGreen->off(); }
if (button->value() == 1)
{ break; }
}
// Delete the Grove LED object
ledGreen->off();
ledRed->off();
delete ledGreen;
delete ledRed;
delete a_pin;
delete button;
delete nmea;
return MRAA_SUCCESS; }
Step 6: Getting the Hardware and Sensors Connected
The GPS sensor:
The GPS sensor is linked to the UART input of the Base Shield (included in the Grove Starter Kit Plus). Once connected you should see the green light on the sensor turned on, this indicates its properly connected and receiving power.
The Hall sensor:
The Hall sensor is connected to A0 input of the Base Shield.
Take it a step further:
In addition, if you are feeling ambitious the LED sensor is connected to D4 and D3 (one for red and one for green) and the button sensor is connected to D8.
Step 7: Seeing the GPS Data in Action
Note that the GPS data is in NMEA format.
Once you have your the sensors connected to the board you are ready to build and deploy the code. Once you deploy the code you should see the following in your console in Eclipse.
Step 8: Getting Intel IOT C++ to CURL JSON to Firebase
While Intel Edison provides instructions for having the Intel IOT Edison perform REST API calls with the python and javascript library, the documentation for C++ is lacking. This example code (http://curl.haxx.se/libcurl/c/http-post.html) along with the instructions below describes how to get the Intel IOT Edison board’s C++ environment to perform CURL calls.
1. Include the .h files at the top of your cpp file:
#include <stdio.h>
#include <curl/curl.h>
2. Ensure curl is linked in the project properties setup.
In order to function, the cURL library must be linked. If you get complier errors, do the following to add the cURL library: Right click on the project and select properties. Then navigate to
C/C++ Build -->Settings --> Cross G++ Linker --> Libraries --> Click the green plus button,and add the “curl” library
3. Set up the code. This will be in your main() cpp code. Note that the highlighted yellow dummy link needs to be replaced with your own Firebase URL.
//CURL Setup
CURL *curl;
CURLcode res;
// In windows, this will init the winsock stuff
curl_global_init(CURL_GLOBAL_ALL);
// get a curl handle
curl = curl_easy_init();
// First set the URL that is about to receive our POST. This URL can
// just as well be a https:// URL if that is what should receive the
// data.
curl_easy_setopt(curl, CURLOPT_URL, "https://.firebaseio.com/data.json");
4. cURL Call. In the infinite loop portion of the code, perform the CURL
call when the appreciate trigger occurs. You will need to replace the JSON data highlighted in yellow with your own data.
if (button->value()==1){
std::cout << button->name() << " value is " << button->value() << std::endl;
// Now specify the POST data
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "{\"lat\":23.343,\"long\":234.45345}");
// Perform the request, res will get the return code
res = curl_easy_perform(curl);
// Check for errors
std::cout << "curl output: " << res << std::endl;
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res)); }
Step 9: Getting All the Parts Together in a Prototype
We used the hall sensor, GPS sensor, LED and button to protoype a smart safety holster. The idea was to trigger when an object its removed and start capturing the GPS location of where the object was removed. The data would then be uploaded to the could.
Once the object its placed back and the hall sensor detects the object is back in position the GPS data will stopped to be collected. This can be applied to any objects to which the hall effect aplies. The GPS and LED functionality can be re used for other projects.
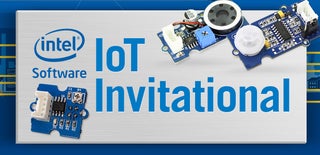
Participated in the
Intel® IoT Invitational
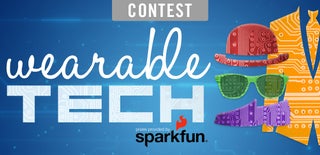
Participated in the
Wearable Tech Contest