Introduction: 3D Printing a Bizarre Aircraft Design From the 1900's
In this Instructable, I will show you how I 3D printed a bizarre airplane design patented by my great-grandfather in 1909. Now, I realize that most of you do not have an aircraft patent from the early 1900's that you want to print. That is why this Instructable could actually be titled "Ideas and Tips You Might Use to 3D Print Something Interesting From 2D Source Material".
US Patent 922972 shows the design for what might be the most unlikely looking aircraft ever designed. There are two very important things to note about this aircraft. First, the front is the end with the pipe sticking out of it. Yes, that looks like the rear but it isn't. Second, there is no way this craft could have left the ground unless it was strapped to a solid rocket booster.
Nevertheless, this patent is a part of my family history and I decided to try to bring it off the page and into the 3D world. By the way, this aircraft was my first ever 3D printed project and this is my first Instructable. I probably should have started with a smaller project but that wouldn't have been nearly as much fun.
What you will need to create a similar project:
- Source material (in my case, a patent document with drawings and textual description of the aircraft)
- Microsoft Publisher or a full-featured PDF viewer
- OpenSCAD (http://www.openscad.org/)
- SolidPython (https://github.com/SolidCode/SolidPython)
- MeshLab (http://meshlab.sourceforge.net/)
- Access to a 3D printer or printing service
Why OpenSCAD and SolidPython? Some people may not like that toolset because it doesn't allow you to drag things around on the screen and draw them interactively. However, I feel very at home with it. By day I'm a C++ programmer but I use python for all my scripting needs. Building my aircraft components a piece at a time by writing python code works great for me.
I won't try to explain the details of this aircraft design in this Instructable. If you are interested, you can read the patent application linked above or if you are really, really interested, you can read the document I wrote that details my research. "The Flying Machines of George W. Thompson" is about 40 pages long and goes into quite a bit of detail about my research and motivation for this project. Or you can just keep reading to see how I went about creating a CAD drawing from a 100 year old patent and printing it on a 3D printer.
Attachments
Step 1: Getting Started
Make sure the tool-chain works
The very first step is to get all the tools installed. The steps for this will vary based on your operating system and whether you choose to build from source or install via a package manager. I will not even attempt to guide you through that process. Google is your friend.
Once everything was installed, my development process went like this:
- Write code in python to create OpenSCAD objects
- Run the python script
- Use OpenSCAD to display the resulting .scad file
- Check for proper appearance
- Export to a .stl file and load it into MeshLab
- Check for any open manifolds
- Repeat countless times until done
To do this, I had my python files open in gedit, a terminal window where I would run the python script and OpenSCAD open and set to automatically reload the .scad file when it changed.
Why did I use MeshLab in addition to OpenSCAD to check for errors? I found that MeshLab detected some problems that OpenSCAD did not. Of course, that might just be because I'm an OpenSCAD rookie and don't know how to use it properly.
Hello World
I'm not going to teach you how to use OpenSCAD, python and SolidPython but I will show you the code for creating a very simple shape. There are plenty of tutorials and references out there to give you more detail. I'll just say this – if you like writing code, you'll find it pretty easy to create complex shapes using this tool-chain.
Basically, everything in OpenSCAD is a combination of primitive geometric shapes. Want to draw a truncated ellipsoid? Figure out which primitives to add & subtract and it is pretty easy. Okay, easy might be overstating it a bit. I spent several weeks after work and on weekends to get all the pieces of the aircraft just right. Designing propellers, landing gear and wings took me a lot of time. In my defense, I'd never done any 3D modeling before so pretty much everything I did was brand-new and involved making a lot of mistakes.
To whet your appetite, here is some code to draw a sphere with a hole in it. This is the OpenSCAD version of the Hello World program that everyone writes when learning a new language. When you run this, it will create a file named HelloWorld.scad. Start OpenSCAD and load that file and you will see an image like the one above. Once you can do that much, you are well on your way to drawing remarkably complex objects.
#!/usr/bin/python
# Very basic OpenSCAD example - draw a sphere with a hole in it.from solid import * from solid.utils import *
SEGMENTS = 50 f = sphere(5) - down(10) (cylinder(r=3, h=20)) file_out = "HelloWorld.scad" scad_render_to_file( f, file_out, file_header='$fn = %s;'%SEGMENTS, include_orig_code=True)
Step 2: Make a Lot of Measurements
Extract critical dimensions from a 105 year old hand-drawn diagram
This was the key step in the whole process. Because the patent drawings didn't show any dimensions, I had to find a way to extract the dimensions of every part of the aircraft.
The first time I did this, I used a labor-intensive manual process that was quite prone to error. I printed the pdf of the patent drawing and used a ruler to draw horizontal and vertical lines through key points on the body of the aircraft. Then I used the ruler to measure the distances between these lines. The problem with this approach is that I'm not very good at drawing perfectly horizontal and vertical lines. As a result, some of my measurements were off, which made everything fit together like something made by M.C. Escher.
Eventually I realized that there is a much easier way to do this. I simply loaded the pdf straight from the patent into Publisher and used that tool to draw lines through the key points. With the built-in ruler and cursor location on the status bar, it was very easy to get the correct values for these measurements. Obviously you can use any tool that allows you to draw straight lines on an image and measure the distance between points. I used Publisher because it was convenient.
Create a python module to define all these measurements
Then I entered all these values into a python module and wrote a little code to perform some consistency checks. If the same point appeared in two or more perspectives in my great-grandfather's drawing, I wanted to make sure that the different measurements for it matched. These checks revealed a few minor inconsistencies between the three perspectives. I did my best to resolve these but in a couple of cases, I had to flip a coin to figure out which measurement best represented his intention.
This module is key because when you actually start writing OpenSCAD code, this is where the dimensions for all your plane parts come from. However, it is important to note that this module is completely independent of the CAD program. If I want to use some other technology in the future, this module can be used as-is.
Step 3: Write Functions to Define Each of the Components of the Airframe
To keep this Instructable reasonably short, I'm not going to walk you through all my code. Instead, I'll give a brief summary of how I built each part and the challenges I faced. If you download the code, you can immerse yourself in all the details of how each part was constructed. It is two files totaling about 1100 lines of python and is reasonably well commented.
Fuselage
This was certainly the most complicated part of the aircraft. The way I drew the body of the aircraft was to start with a hemisphere on one end, a tapered cylinder in the middle and another hemisphere of different radius on the other end. I joined these all together to make a roughly egg-shaped object. Then I created flat planes that I would use to intersect that part and create the flat surfaces. I also had to make an inner shell that I could subtract from the outer shell so that what was left was hollow. There were a lot of errors and dead-ends in this step. My first several tries generated a lot of open manifolds, which are not a good thing when you go to print it.
Wings and Fin
These were straight-forward. I created a rectangle and then subtracted off other rectangles at various angles to give the wings the angles you see on the side and rear. The only tricky part was the inclined part of the wing at the front of the craft. Fortunately, a little bit of trigonometry using the measurements I took earlier made it all come together okay. The fin was a simple rectangle with the top edge sliced to give it a slight angle.
Landing Gear
By experimenting with cylinders and toruses (tori?) of different sizes, I was able to combine shapes until I had something that looked like a tire mounted on a wheel. The axles were a flat bar combined with a segment cut from an ellipse. These all took a little playing around to make them look right but it wasn't terribly hard.
Propellers
I was afraid this would be hard but it wasn't because I cheated. A real propeller has a twist around the axis running from the hub to the tip of the blade. Not mine. I just created a thin cylinder and cut off an end to make one blade. Then I stuck the blades to a cylindrical hub at an angle. This looks fine for my purposes but probably wouldn't move much air in real life.
Intake Pipe
The pipe running down the middle of the craft us supposed to feed air to the propellers. This is just a long hollow cylinder. The only strange part is that the design shows the drive-shaft for the propellers mounted inside this pipe! That would have played havoc with airflow but aerodynamics was a brand-new science back then so I guess Thompson can be forgiven for not realizing that. I was sorely tempted to "improve" the design and move the drive-shaft to another location. However, I resisted that urge and left the design as he drew it.
Step 4: Development and Debugging Considerations
Many Ways to Get There
When building complex shapes in OpenSCAD, there can be many different ways to accomplish the same goal. One way might look better but another might render more quickly. Another way might look right but end up generating a lot open manifolds. Part of the fun was in figuring out how to combine a bunch of primitives into something that looked like what I wanted.
Low-resolution vs High-resolution
One of the parameters you set in OpenSCAD is how many segments to use when drawing circular shapes, like the fuselage. A lot of segments will make the surface look smoother but it will take a long time to render. A really, really long time. I did all my development on a four year old laptop and the STL file I sent to the printing service took 4+ hours to render. Obviously I couldn't endure that kind of wait during development so I had to turn that parameter down until I was ready for the final version. For quick iterations I used 50 segments per circle but for the final one, I set it to 120.
Eureka!
Even though I had looked at all the drawings in the patent application and read all the text, I couldn't really visualize the aircraft. Once I had all the pieces looking right and drew the entire aircraft at once, the most amazing thing happened! The top, front and side perspectives all matched the patent drawings in the finest detail. And when I rotated the aircraft to see a more 3Dish view, I really understood what it was supposed to look like! It was awesome to see the flat patent drawings spring off the screen in a 3D view. I never met my great-grandfather but I wonder what he would think about being able to see his design in 3D without having to saw, weld and rivet hardware together. For me, it was pretty cool.
Attachments
Step 5: Get Your Design Printed
Now here's the problem... I don't have a 3D printer! (This is a deficiency which I hope to remedy by winning the Instructable's 3D printing contest :)
But until I buy, win or make one, I have to use a 3D printing service. So I looked around and found an in-state company that seemed reputable. I contacted them, uploaded my STL files, got a quote and a few weeks later, received 3 copies of the aircraft in different sizes.
I was really very surprised at how easy this step was. The online ordering and uploading of the STL file was painless. For some reason I imagined it would be much more complicated.
The Cool Part
Unpacking the box and seeing an actual, tangible version of what I drew in OpenSCAD was incredibly gratifying. As soon as I had the model in hand, I knew that I was hooked on 3D printing.
Lessons Learned
It turns out that the company I used wasn't able to accurately print the design I shipped them. The final product was mostly right but had one very significant flaw. There was a flat surface in the print that wasn't in the design. This surface occupied part of the propeller chamber and the propellers were embedded in it. This is partially visible in the pictures above. The printing service couldn't figure out how to print the design without that extraneous surface so I was stuck with three prints that didn't really match the design. Lesson - print one small copy first before committing to a larger, more expensive order.
The other problem was just one of scale. The models I printed had overall lengths of 6" and 4". At that size, the landing gear were very fragile and broke soon after I unpacked them. I simply reattached the broken axle with Gorilla Glue, which is why the damage isn't apparent in the photos. (Handy tip - give the glue several hours to dry before trying to move the print.)
Eventually I will revise the design and make the landing gear beefier. Then I will find a different printing service and do a trial print of a 4" model. If the axles hold up and there aren't any parts that aren't supposed to be there, I'll order larger prints.
Step 6: Flight Test
As I mentioned earlier, there's no way that this aircraft could have flown. It has too many aerodynamic flaws to make it airworthy. But with the miracle of mono-filament thread, scotch tape and your favorite image editing software, the illusion of flight can be achieved.
- Get some clear or white mono-filament thread
- Tape that to the far side of the longitudinal fin
- Have one person standing and holding the suspended model at arm's length
- Have another person taking pictures from below, keeping the sky in the picture
- Use gimp (or similar software) to crop out the person holding the thread and any unwanted cars, houses, etc
- Do NOT post this as evidence of UFO visitation
Step 7: Summary
While there are a lot of different ways to get started with 3D printing, I knew that I didn't want to do something that had been done before. For me, this project drove home the fantastic potential of this technology. I'm not a model builder, a machinist or a designer but I was able to make something physical that was previously just drawings on a 100 year old patent diagram. Bringing my great-grandfather's aircraft design to life was a fascinating and rewarding experience. I hope this Instructable gives you ideas about how to build something unique and important to you.
If you found this Instructable to be informative and entertaining, I hope you will vote for it in the Instructable's 3D Printing Contest. I'm in the early phases of a fun robotics project which will be roughly modeled on NASA's Mars rovers and will perform autonomous exploration of the open field near my house. Being able to print parts for that would make the build easier and more affordable.
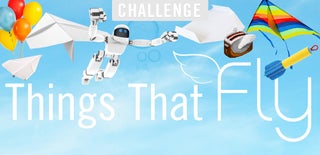
Participated in the
Things That Fly Challenge
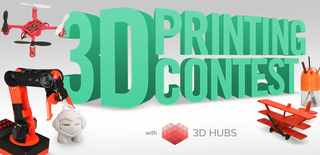
Participated in the
3D Printing Contest