Introduction: ARDUINO LED TESTER
A small LED tester project
I was looking on the NET for an LED tester and after seeing some idea I wanted to build this tester from zero
Step 1: The Schemat
To test an LED you need a current generator.
The LM317LZ datasheet provides a map, See the basic idea website
https://www.robotroom.com/LED-Tester-Pro-1.html
no code available from this site
the principle therefore is to measure several voltage at the terminals of the LED
VH anode voltage VL cathode voltage and subtract them (VH - VL)
then to measure the voltage at the terminal of the resistance traversed by the current of the LED IL and divided by its value R (VR/R=IL)
Attachments
Step 2: Code Arduino
For the Arduino code the basic idea was defined I wanted a display on OLED screen
the library is for a ssd1306
https://github.com/adafruit/Adafruit_SSD1306
For measuring different voltages see
https://www.instructables.com/id/Arduino-OLED-Volt...
For different menu choices see
http://it-edukacija.eu.hr/multidruino/default.html
To create icons see
http://javl.github.io/image2cpp/
we pass the test on a test board
code here:
// ================================================
// Author: PHILOUPAT
// Mars 2019 // version 1: one buttons, display modes OLED I2C, AREF default // ================================================
// I2C OLED DISPLAY: SCL = A5 , SDA = A4
// A0 input reference for calculation RL
// A1 input Vbat
// A2 input VL
// A7 input VH
// ================================================
#include
#include
#include
#include
#include
#include
#define SSD1306_128_64
#define SCREEN_WIDTH 128
// OLED display width, in pixels
#define SCREEN_HEIGHT 64
// OLED display height, in pixels
// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
#define XPOS 0
#define YPOS 1
#define DELTAY 2
//#if (SSD1306_LCDHEIGHT != 64)
//#error("Height incorrect, please fix Adafruit_SSD1306.h!");
//#endif
const unsigned char PROGMEM batterie [] = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xfe, 0x00, 0x20, 0x00, 0x00, 0x02, 0x00, 0x20, 0x00, 0x00, 0x02, 0x00, 0x20, 0x00, 0x00, 0x02, 0x00, 0x20, 0x00, 0x00, 0x03, 0xe0, 0x20, 0x00, 0x00, 0x02, 0x20, 0x24, 0x00, 0x00, 0x82, 0x20, 0x24, 0x00, 0x01, 0xe2, 0x20, 0x24, 0x00, 0x00, 0x82, 0x20, 0x20, 0x00, 0x00, 0x82, 0x20, 0x20, 0x00, 0x00, 0x03, 0xe0, 0x20, 0x00, 0x00, 0x03, 0x00, 0x20, 0x00, 0x00, 0x02, 0x00, 0x20, 0x00, 0x00, 0x02, 0x00, 0x3f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 };
const unsigned char PROGMEM resis [] = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x10, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x10, 0x00, 0x00, 0x00, 0x10, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x3c, 0x00, 0x00, 0x00, 0x0f, 0x00, 0x00, 0x00, 0x7f, 0x80, 0x00, 0x3f, 0xf8, 0x00, 0x00, 0x7e, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00, 0xe0, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x07, 0xfe, 0x00, 0x00, 0xff, 0x80, 0x00, 0x00, 0x78, 0x00, 0x00, 0x00, 0x1e, 0x00, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x01, 0xe0, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x00, 0x7e, 0x00, 0x00, 0x3f, 0xf0, 0x00, 0x01, 0xf8, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x00, 0x07, 0x00, 0x00, 0x00, 0x01, 0xc0, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x0f, 0xf8, 0x00, 0x03, 0xff, 0x00, 0x00, 0x03, 0xc0, 0x00, 0x00, 0x00, 0xe0, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00, 0x00, 0x0c, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x10, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff };
const unsigned char oms [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x0c, 0x30, 0x18, 0x18, 0x18, 0x0c, 0x30, 0x0c, 0x30, 0x0c, 0x30, 0x0c, 0x30, 0x0c, 0x18, 0x0c, 0x08, 0x18, 0x0c, 0x30, 0x06, 0x30, 0x1e, 0x3c, 0x1c, 0x3c, 0x00, 0x00, 0x00, 0x00 };
const unsigned char PROGMEM led [] = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xe0, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x00, 0xff, 0xfc, 0x00, 0x00, 0xff, 0xfe, 0x00, 0x01, 0xff, 0xff, 0x00, 0x03, 0xff, 0xff, 0x00, 0x03, 0xff, 0xff, 0x80, 0x03, 0xff, 0xff, 0x80, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x07, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xf7, 0xff, 0xf0, 0x1f, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xf8, 0x0f, 0xcf, 0xfe, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xe0, 0x0e, 0x00, 0x01, 0xe0, 0x0e, 0x00, 0x00, 0xe0, 0x0e, 0x00, 0x01, 0xe0, 0x0e, 0x00, 0x01, 0xe0, 0x0e, 0x00, 0x00, 0xe0, 0x0e, 0x00, 0x01, 0xe0, 0x0e, 0x00, 0x01, 0xe0, 0x0e, 0x00, 0x01, 0xe0, 0x0e, 0x00, 0x00, 0xe0, 0x0e, 0x00, 0x00, 0xe0, 0x0e, 0x00, 0x00, 0xc0, 0x0e, 0x00, 0x00, 0xc0, 0x0c, 0x00, 0x00, 0xc0, 0x04, 0x00, 0x00, 0xc0, 0x0c, 0x00, 0x00, 0xc0, 0x0c, 0x00, 0x00, 0xc0, 0x0c, 0x00, 0x00, 0xc0, 0x0c, 0x00, 0x00, 0xc0, 0x04, 0x00, 0x00, 0x40, 0x04, 0x00, 0x00, 0x00, 0x0c, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 };
const unsigned char accueil [] PROGMEM = {
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x03, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0xe1, 0xd8, 0xc3, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0xc1, 0xd9, 0x83, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe6, 0xff, 0xf9, 0x83, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc4, 0xe1, 0xb9, 0x83, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc4, 0xf9, 0xb1, 0xa3, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x83, 0xf3, 0x07, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe1, 0x83, 0x73, 0x0f, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x3f, 0xff, 0xf3, 0xf8, 0x02, 0x03, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xe3, 0xf8, 0x06, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xe3, 0xf1, 0xfc, 0x30, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x3f, 0xff, 0xe3, 0xf1, 0xfc, 0x78, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xff, 0xc7, 0xf3, 0xbc, 0x7c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc7, 0xe0, 0x1c, 0x7c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x04, 0x00, 0x0f, 0xff, 0xc7, 0xe0, 0x3c, 0x7c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x07, 0xff, 0xcf, 0xe1, 0xfc, 0x7c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0x8f, 0xe3, 0xf8, 0x7c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0x8f, 0xc7, 0xf8, 0xf8, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0x8f, 0xc7, 0xf8, 0xf0, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x07, 0xff, 0x88, 0x07, 0x88, 0x60, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x08, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf1, 0xf9, 0xff, 0xcf, 0xff, 0x80, 0x00, 0x08, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1f, 0xf8, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0xe0, 0x1c, 0x01, 0xc0, 0xe0, 0x1c, 0x01, 0x0f, 0x18, 0x07, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0x80, 0x04, 0x03, 0x00, 0x00, 0x04, 0x01, 0x1f, 0x98, 0x03, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0x88, 0x88, 0xfe, 0x1e, 0x00, 0x40, 0xff, 0x1f, 0x90, 0xe1, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0x38, 0xf8, 0xfe, 0x3f, 0xb8, 0xf8, 0xfe, 0x1f, 0x91, 0xe1, 0xff, 0xff, 0x1f, 0xf0, 0xff, 0xff, 0xf9, 0xf8, 0xfe, 0x3f, 0xf8, 0xf8, 0xfe, 0x3f, 0x91, 0xc3, 0xff, 0xff, 0x1f, 0xf9, 0xff, 0xff, 0xf1, 0xf0, 0x0f, 0x07, 0xf8, 0xf8, 0x0e, 0x3f, 0x93, 0x87, 0xff, 0xff, 0x1f, 0xf9, 0xff, 0xff, 0xf1, 0xf0, 0x1f, 0xc1, 0xf8, 0xf0, 0x0e, 0x3f, 0xa0, 0x0f, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xf1, 0xf0, 0xff, 0xf8, 0x70, 0xf0, 0xfe, 0x3f, 0x20, 0x1f, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xf1, 0xf1, 0xff, 0xfc, 0x71, 0xf1, 0xfe, 0x3f, 0x20, 0x0f, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xe1, 0xe3, 0xfd, 0xfc, 0x71, 0xf1, 0xfe, 0x3f, 0x23, 0x8f, 0xff, 0xff, 0xbf, 0xf9, 0xff, 0xff, 0xe1, 0xe3, 0xfc, 0xf8, 0x61, 0xe3, 0xfe, 0x1e, 0x63, 0x87, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xe1, 0xe3, 0xc4, 0x00, 0x61, 0xe1, 0xc6, 0x00, 0x63, 0xc7, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xe1, 0xe0, 0x04, 0x00, 0xe1, 0xe0, 0x07, 0x00, 0xe3, 0xc3, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xe1, 0xe0, 0x07, 0x03, 0xe1, 0xe0, 0x07, 0x01, 0xc3, 0xc3, 0xff, 0xff, 0x9f, 0xf9, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf9, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf9, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfb, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff };
int mode=0;
int pause=500;
int RL= 0;
int R1= 10000;
int R2= 10000;
int R3= 47;
float raw= 0;
float val= 0;
float ref= 0;
float vbat=0;
float vin= 0.00;
float voutL= 0.00;
float voutH= 0.00;
float vref= 0.00;
float vled= 0.00;
float vr= 0.00;
float IL= 0.00;
float vinref = 0.00;
float vinbat=0;
void setup()
{
pinMode(5,INPUT_PULLUP);
pinMode(4,INPUT_PULLUP);
pinMode(3,INPUT_PULLUP);
attachInterrupt(1, bouton, FALLING);
// by default, we'll generate the high voltage from the 3.3v line internally! (neat!) display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the I2C addr 0x3C (for the 128x32)
}
void loop()
{
float vref=readVcc()/1000.0;
val = analogRead(A7);
raw = analogRead(A2);
voutH = (val * vref) / 1024.0; // see text
voutL = (raw * vref)/1024.0;
if (voutL>0)
{
if(mode==0) //caracteristique led
{
float vref=(readVcc()/1000.0);
val = analogRead(A7);
raw = analogRead(A2);
ref = analogRead(A0);
voutH = (val * vref) / 1024.0; // see text
voutL = (raw * vref)/1024.0;
vled = (voutH-voutL)*(R1+R2)/R2;
vr = voutL;
IL = vr/R3;
display.clearDisplay();
display.setTextColor(WHITE);
display.drawBitmap(96, 0, led, 32, 64, 1);
display.setFont();
display.setFont(&FreeSans9pt7b);
display.setCursor(0,12);
display.print("LED feature");
display.setCursor(0,35);
display.print("VL:");
display.setCursor(28,35);
display.print(vled);
display.setCursor(72,35);
display.print("V");
display.setCursor(0,60);
display.print("IL:");
display.setCursor(28,60);
display.print(IL*1000);
display.setCursor(75,60);
display.print("mA");
display.display();
delay(pause);
}
if(mode==1)
{// resistance
float vref=(readVcc()/1000.0);
val = analogRead(A7);
raw = analogRead(A2);
ref = analogRead(A0);
voutH = (val * vref)/1024.0;
voutL = (raw * vref)/1024.0;
vinref = (ref * vref*2)/1024.0;
vled = (voutH-voutL)*(R1+R2)/R2;
IL = voutL/R3;
RL= (vinref-vled)/IL;
display.clearDisplay();
display.drawBitmap(96, 0, resis, 32, 64, 1);
display.setFont();
display.setFont(&FreeSans9pt7b);
display.setCursor(0,12);
display.print("Resistance:");
display.setCursor(0,35);
display.print("Vin:");
display.setCursor(32,35);
display.print(vinref);
display.setCursor(75,35);
display.print("V");
display.setCursor(0,60);
display.print("RL:");
if(RL<0)
{
display.setCursor(30,60);
display.print("empty");
}
else
{
display.setCursor(30,60);
display.print(RL);
display.drawBitmap(73, 46, oms, 15, 15, 1);
}
display.display();
delay(pause);
}
if(mode==2)
{//baterie
float vref=(readVcc()/1000.0);
vbat = analogRead(A1);
vinbat = (vbat*vref*2.3)/1024.0;
isplay.clearDisplay();
display.drawBitmap(90, -2, batterie, 36, 21, 1);
display.setFont();
display.setFont(&FreeSans9pt7b);
display.setCursor(0,14);
display.print("Batterie:");
//display.fillRect(10,35,90,20,WHITE);
//display.fillRect(12,37,88,18,BLACK);
display.drawRoundRect(15, 40, 95, 24, 8, WHITE);
display.setCursor(0,35);
display.print("Vbat:");
display.setCursor(50,35);
display.print(vinbat);
display.setCursor(90,35);
display.print("V");
if(vinbat > 0 && vinbat < 7.0)
{ //one bar
display.fillRect(20,45,10,15,BLACK);
}
else if(vinbat > 7.0 && vinbat < 7.8)
{ //two bar
display.fillRect(20,45,10,15,BLACK);
display.fillRect(30,45,5,15,WHITE);
display.fillRect(35,45,10,15,BLACK);
}
else if(vinbat > 7.8 && vinbat < 8.4)
{ //three bar
display.fillRect(20,45,10,15,BLACK);
display.fillRect(30,45,5,15,WHITE);
display.fillRect(35,45,10,15,BLACK);
display.fillRect(45,45,5,15,WHITE);
display.fillRect(50,45,10,15,BLACK);
}
else if(vinbat > 8.4 && vinbat < 8.7)
{ //four bar
display.fillRect(20,45,10,15,BLACK);
display.fillRect(30,45,5,15,WHITE);
display.fillRect(35,45,10,15,BLACK);
display.fillRect(45,45,5,15,WHITE);
display.fillRect(50,45,10,15,BLACK);
display.fillRect(60,45,5,15,WHITE);
display.fillRect(65,45,10,15,BLACK);
}
else if(vinbat > 8.7 && vinbat < 9.0)
{ //five bar
display.fillRect(20,45,10,15,BLACK);
display.fillRect(30,45,5,15,WHITE);
display.fillRect(35,45,10,15,BLACK);
display.fillRect(45,45,5,15,WHITE);
display.fillRect(50,45,10,15,BLACK);
display.fillRect(60,45,5,15,WHITE);
display.fillRect(65,45,10,15,BLACK);
display.fillRect(75,45,5,15,WHITE);
display.fillRect(80,45,10,15,BLACK);
}
else if(vinbat > 9.0 && vinbat < 9.4)
{
display.fillRect(20,45,10,15,BLACK);
display.fillRect(30,45,5,15,WHITE);
display.fillRect(35,45,10,15,BLACK);
display.fillRect(45,45,5,15,WHITE);
display.fillRect(50,45,10,15,BLACK);
display.fillRect(60,45,5,15,WHITE);
display.fillRect(65,45,10,15,BLACK);
display.fillRect(75,45,5,15,WHITE);
display.fillRect(80,45,10,15,BLACK);
display.fillRect(90,45,5,15,WHITE);
display.fillRect(95,45,10,15,BLACK);
}
display.display(); delay(pause); }
}else
{
display.clearDisplay();
display.drawBitmap(0, 0, accueil, 128, 64, 1);
display.display();
delay(700);
display.display();
delay(1000);
}
}
void bouton()
{
static unsigned long last_interrupt_time = 0;
unsigned long interrupt_time = millis();
// If interrupts come faster than 200ms, assume it's a bounce and ignore
if (interrupt_time - last_interrupt_time > 220) {
mode++;
tone(6,2250,50);
if(mode>2)
mode=0;
}
last_interrupt_time = interrupt_time;
}
long readVcc()
{
// Read 1.1V reference against AVcc
// set the reference to Vcc and the measurement to the internal 1.1V reference
#if defined(__AVR_ATmega32U4__) || defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__) ADMUX = _BV(REFS0) | _BV(MUX4) | _BV(MUX3) | _BV(MUX2) | _BV(MUX1);
#elif defined (__AVR_ATtiny24__) || defined(__AVR_ATtiny44__) || defined(__AVR_ATtiny84__) ADMUX = _BV(MUX5) | _BV(MUX0);
#elif defined (__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) ADMUX = _BV(MUX3) | _BV(MUX2);
#else ADMUX = _BV(REFS0) | _BV(MUX3) | _BV(MUX2) | _BV(MUX1);
#endif
delay(2); // Wait for Vref to settle
ADCSRA |= _BV(ADSC); // Start conversion
while (bit_is_set(ADCSRA,ADSC)); // measuring
uint8_t low = ADCL; // must read ADCL first - it then locks ADCH uint8_t high = ADCH; // unlocks both
long result = (high<<8) | low;
result = 1125300L / result; // Calculate Vcc (in mV); 1125300 = 1.1*1023*1000
return result; // Vcc in millivolts
}
Attachments
Step 3: Realization
Schemat and PCB. I use EAGLES for realize the shem and pcb
a good site for manufacturing
List of the material:
-1 Arduino mini pro 5v
https://www.banggood.com/Wholesale-New-Ver-Pro-Min...
-1 0.96 Inch OLED Module 12864 128x64 Yellow Blue SSD1306 Driver I2C Serial Self-Luminous Display Board for Arduino Raspberry PI or other
warning to polarity Gnd Vcc or Vcc Gnd
https://www.amazon.com/UCTRONICS-SSD1306-Self-Lumi...
https://www.ebay.fr/itm/0-96-I2C-IIC-SPI-Serial-12...
-1 LM317 LZ
https://www.ebay.fr/itm/5Pcs-LM317LZ-LM317L-LM317-...
https://www.amazon.com/LM317LZ-Voltage-Regulator-I...
-1 MCP1702 5V
https://www.ebay.fr/itm/Microchip-MCP1702-500-TO-9...
https://www.amazon.com/5002E-MCP1702-1702-5002E-MC...
-2x10K 2x470K 2x 47R 1/8W
-1x500R variable TSR3386F 3/8
https://www.ebay.fr/itm/Suntan-TSR3386F-3-8-1-turn...
-1x10K varable TSR3386F 3/8
https://www.ebay.fr/itm/Suntan-TSR3386F-3-8-1-turn...
-1xsitch on/off SS12D00G4 SPDT 1P2T 2 Position 3 Pin PCB
https://www.ebay.fr/itm/20pcs-SS12D00G4-SPDT-1P2T-...
-2x 1µF tantale
-3x100nF
-1x1N4148
Possible Power on 9V batterie or batterie lipo 3.7V
-1x3.7V 9V 5V 2A Adjustable Step Up 18650 Lithium Battery Charging Discharge Integrated Module
https://www.banggood.com/3pcs-3_7V-9V-5V-2A-Adjust...
-1xLIPO batterie 3.7V 400mAh
Step 4: Code Loading and Test and Put in Box
To load the code use FTDI
and test with different LEDs and diodes
make the box 3d print
get to thingverse:
https://www.thingiverse.com/thing:3594143
assembled and enjoy
this project uses several software is therefore a good Educational chalenge pcb code 3d electronic calculator etc
Step 5:
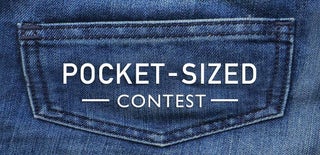
Participated in the
Pocket Sized Contest