Introduction: Absolute Beginner’s Arduino and Coding Tutorial
Concepts: Block coding, Text coding, Variables, Simple Functions, Abstraction, Iterative Design Process, Code Libraries, and Unit Testing
Overview:
This project is the first in a series to build student skills throughout the semester. While I currently teach university level engineering courses at Western Carolina University, I've used this with middle school to high school summer camps and Fab Labs as well. The ultimate goal is for students to combine what they learned all semester in a PBL project in which they design and build a small robot that can solve a simple maze and extinguish a flame.
Assessment:
Students are given a couple of in-class challenges, and a homework assignment with this lesson. The homework assignment is to have the LED flash their first name in Morse code. They are assessed by their submission of a narrated video or screencast of their circuit flashing the appropriate letters in the correct order. Silent videos earn no points in my classes, students must provide context. For latter assignments in this series, student must embed their videos in their portfolio blog pages with a short description.
Lesson :
- Getting Started With A Starter
- Transmitting Data
- In-Class Challenge 1: Make The Block Code Flash The Letter “S” In Morse Code
- From Blocks To Text
- Just Text Me
- In Class Challenge 2: Copy Pasta
- In Class Challenge 3: Abstract Even More!
- Onward!
- Homework
- Closing Remarks
I don’t need intro students to write all of their own code from scratch because there are too many syntactical details required for code to work. I encourage them to copy and paste as much as possible. We will begin with a seed project that provides all the code required to blink an LED provided by Tinkercad. Then we will add functions to this project, testing the code along the way to verify each new addition works correctly. This is similar to what is called “unit testing” in industry. This method of developing a project follows the iterative design process where we begin with a seed, next the project grows with each new challenge, and then the design is tested to verify functionality before moving to the next challenge.
The final working code for flashing "Hey You" in Morse code is available in the last step of this instructable.
Supplies
- We will use Tinkercad Circuits, a free online circuit simulation tool below to learn software engineering concepts in a very simple way. You can build and simulate projects (including custom code) before ever spending money on hardware. This is great for cheap introductions of basic components and concepts for a whole class. This can also help to debug whether issues you might be having are in the code (software issues) or the wiring (hardware issues). You can also create an invitation code for the students in your class by visiting this page once you have set put your free account.
- Optional: Feel free to build the circuits using real hardware if available. Make sure to get the code working in TinkerCad first before building. For this particular lesson you will need:
- an Arduino
- USB cable
- This lesson only uses the built-in LED with the Arduino, but in addition to the Arduino, you could also use:
- a 330-ohm resistor
- an LED
- a breadboard
- wires for breadboard
Step 1: Getting Started With a Starter
Open up Tinker Circuits by going to www.tinkercad.com. You can sign up for free and since it is owned by AutoDesk (like instructables), you can just use that to log in. Once you are logged in, go to the left menu bar and click "Designs". Then you can click the blue "+ Create" button and select "Circuit" to get a blank canvas.
On the right side of the screen, you should see some parts. Clicking the “Components Basic” dropdown, you can select “Arduino”. This will have a lot of starter circuits, many with starter code and code blocks already written.
Click the “Blink” thumbnail and position the circuit in the middle of the blank canvas to the left of the screen.
Now you can see the code and code blocks by clicking the “Code” button.
The window that pops up shows you the code designed to blink the LED in the circuit in the rights-most pane and the other possible output code blocks in the left pane.
Each block’s color means something different. The Grey blocks are comments, which the computer ignores when you try to simulate the code. The blue blocks are outputs commands used to activate some transducer (fancy name for all motors, speaker, lights, etc.). The Yellow blocks are Control blocks. In this case, it is a delay function which pauses the execution for 1 second.
Click the “Simulate” button and watch the red LED on your circuit. Computer code executes line by line from the top to the bottom. Understanding this should help you understand what it should be doing at any moment. This code should flash the LED on for 1 second, then off for one second, then repeat.
Looking at the code blocks, we can see what we are doing.
the built-in LED to a HIGH. This means to turn it on. In computer speak, “HIGH” and “LOW” are the 1s and 0s we always hear about when we talk about computers. HIGH is a ‘1’ and LOW is a ‘0’. Here are some other things we call these 1s and 0s that you might see when researching coding:
Names for "1" | Names for "0"
HIGH | LOW
True | False
on | off
5v | 0v (Ground)
The first code block says to set the built-in LED to high. This is the little white rectangle labeled ‘L’ directly under our LED. Take a look again at the blinking picture above. Notice that it turns yellow when our LED lights up. This LED is connected to Pin 13 on pretty much all Arduinos, and any time you set that pin HIGH, it’ll turn on. This is super helpful when troubleshooting your code.
The next block is Yellow, which is a control block. It says to wait for 1 second. So basically the program will sit on that block for 1 full second before moving forward. The third block is blue and says to set the Built-in LED to LOW, which turns this LED off. Then we have another WAIT statement for 1 second.
When you come to the end of the code the arduino board will automatically start back at the top because it is within a forever loop.
Step 2: Transmitting Data
So that is the basics of the code, let’s hack around with it to make it more useful. Morse code is a simple way of encoding information using short pulses and long pulses in a pattern to represent letters of the alphabet. This can be used to send messages with sound, lights, electrical signals, even by blinking your eyes! Morse code was one of the first methods for sending messages on telegraph lines and while it may seem old-fashioned, it is still used and is the perfect way to introduce several key concepts about programming!
If there is only one message you ever learn in Morse code, it should be “SOS” which means “Save Our Souls” or “Save our Ship”. This has been used to save countless lives over the years in emergency situations.
The Wikipedia page for Morse code has a chart of all the letters.
And there are some simple rules for Morse code:
- "Dots" shall be 1 unit long
- "Dashes" shall be 3 units long
- There shall be 1 unit separating parts of the same letter
- There shall be a total of 3 units between each letter
We can define a “unit” as being 0.5 seconds for our purposes.
Step 3: In-class Challenge 1: Make the Block Code Flash the Letter “S” in Morse Code
Your code shall flash the Morse code of “S” repeatedly. Looking at the Morse Code Chart, the letter S is simply 3 short flashes. Given our definition of a “unit” as 0.5 seconds, we can simply modify the blocks from the flash example to do this.
This code will need 6 blue output statements with yellow wait statements between them. I added an extra 1 second delay after my last 0.5 second delay for a total of 1.5 seconds between this letter and the next.
Step 4: From Blocks to Text
At this point, let’s take a look at the real code that is being run on the Arduino. Tinkercad gives users the ability to compare the blocks and the real code that the blocks generate which can help students make connections with how coding actually works. In the left code pane, at the top you can select “Blocks + Text” from the dropdown menu.
The new pane shows you actual Arduino code (which is a type of embedded C++). It doesn’t look so scary though, does it?
You can see the text is different colors. This is called syntax highlighting and it helps you understand and troubleshoot your code. Orange is a comment and all comments begin with a double forward slash “//”. This tells the compiler that it can ignore any text to the right of those slashes on any line. Comments are ignored by the compiler, but they are essential to writing good code. I can’t tell you the number of times I have had to either go back into my old code to fix or change something and been mad at past-Adam for not putting enough comments. I’ve even worked on many projects including consultant jobs where they hand me uncommented code someone else wrote and expect me to figure it out, then add or fix features. This is nearly impossible and I’ve honestly had to throw the code out completely and start from scratch just so I know what the heck the code is trying to do. Because of all that, nowadays I almost over comment my code just to be safe.
Arduino Text Code:
All Arduino code has two main functions: setup() and loop(). All the code within these functions live within the curly brackets { and }. The code in the setup() function runs only one time, as soon as the code is started. This is usually where you put tasks you only want to run once such as setting pins to be either outputs or inputs. In our code, since we are setting the value of the built-in LED (which is attached to pin 13) we need to declare pin 13 as an output. We do this with a special command as seen on line 10:
pinMode(LED_BUILTIN, OUTPUT);
LED_BUILTIN is a special keyword in arduino. Any time the compiler sees this, it replaces it with the number 13 (the pin the LED is attached to).
The code is case-sensitive which means be careful of capital letters. There is no space between “pin” and “Mode”. The next part of the command tells the compiler which pin we are talking about. In this case, pin 13 and we tell it to be an output. We must then close the parenthesis ‘)’ and finish the line with the ever-important semicolon. Probably 80% of the time when you have an error, it’s because you forgot to put the semicolon at the end of the line. The compiler needs this to tell it when you have finished the command.
After the setup, we can turn on our LED. As we saw earlier, this code loops forever until we hit the stop button on the simulator. That’s a hint that the loop() function is being used for this part of the code. Since we are using HIGH and LOW, we’ll be using the digitalWrite command on line 15.
digitalWrite(LED_BUILTIN, HIGH);
You can guess what the 13 and HIGH are referring to here. This line is executing the task in our first blue code block: turning the LED on. Then we have a delay(500); on line 16. This corresponds to the yellow “Wait 0.5 seconds” block of our code. Computers do things very fast (your computer can do more than 1 billion instructions a second!) so we do not count in seconds, we count in milliseconds, which is what this delay function uses. There are 1000 milliseconds in 1 second so we use half of that, 500 as our delay time for half a second.
The next blue block on line 17 sets the LED pin to LOW, turning the LED off. Then we have our other delay(500); on line 18.
All this together flashes the LED on, then off once. Since the “S” requires 3 flashes, we do this same thing 3 times (lines 19-26).
At the end, we want to clarify we are finished with the letter, so we added a 1 second delay (line 29) to the previous 0.5 second delay to give us a total of 1.5 seconds delay. Why not just add a 1.5 second delay and call it done? I’ll explain below how this will help us in the future.
The loop ends with the closing curly bracket } on line 30. Once the arduino reaches this line, it will start the top of the loop over again.
Step 5: Just Text Me
At this point, we want to take advantage of the features of the text version of our code. This makes it easier. In Tinkercad, click “Blocks + Text” and change it to just “Text”. It will give you a warning saying basically once you go to just text, you can’t go back to blocks on this particular file. That’s okay, we’ve outgrown the blocks, so just continue.
In the code we’ve got here, we are flashing the LED in a pattern to represent the Morse code for “S”. In Morse code, we call these short flashes “dots”. It takes a lot of code just to do this. Also, if you assume you used Morse code to send a whole sentence to someone, it would be hard to tell which letter is which just by looking at the code. This is the perfect opportunity for us to use a function in our code. A function is a bit of code that you name and place somewhere else in your code, and when you want to use it in your main loop, you just call that function by name. This way of simplifying the main code is called abstraction and it is the engineer’s super power! Imagine if you had to understand every line of code your browser used before you could get on the internet. Very few people would use the internet right? No sending cat videos to grandma! Abstraction allows us to hide the details of the guts of the code so that we can build more useful things.
In fact, we are actually already using a lot of functions without realizing it. setup(), loop(), pinMode(), digitalWrite(), and delay() are all functions in arduino. They are defined somewhere in the arduino’s base code and we can just use them freely in our code without seeing exactly how they work. We need to define our own custom functions however.
Let’s start by breaking down the “S” into its parts. We can create a function called “dot()” that will do all the tasks required to flash a dot in Morse code. Then we can use this function 3 times in a row to flash an S in our main loop.
On line 7 (above your void setup()) add the following code:
void dot(){
}//end dot
This is called a function declaration. This is basically the code we want to run anytime the dot function is called. Think of it like a blueprint. By defining it once here it saves us from having confusing code later. The other two function declarations in our code are void setup and void loop. Note that functions can only be declared outside of other functions, but they must be called from inside a function. I’m sure this sounds confusing, but you’ll see what I mean.
Let’s copy and paste the code for a single “dot” from our loop code into this function declaration.
Once this is done, we can use it in our loop to flash our “S” in Morse code. I’ll add a comment to remind myself this is the letter S.
Now that we are dealing with straight text, I like to introduce another tip. Every closing curly bracket, you should comment what it goes to, like I did at the end of the dot function. I like adding these comments to the end of the setup and the loop functions. This really helps troubleshooting later on. Probably the second most common error I see in coding is making sure my curly brackets are correct. Curly brackets are used to help the logical flow of your program.
Great! Now our main code is much easier to read! Notice how we only use the name of the function and the parenthesis. The keyword “void” is only used to define this type of function.
Let’s make sure this still functions the same as before. We can consider this a unit test. Unit tests are how programmers test their code. Once they implement a single thing, they must verify it works as expected before moving forward to the next feature. Go ahead and click “Simulate” to make sure the LED still flashes correctly as before.
Step 6: In Class Challenge 2: Copy Pasta
Let’s take a look at the chart to see how to flash the Morse code for the letter O in our SOS message. O in Morse code is dash-dash-dash. Using our rules from before where a dash is 3 “units”, and we defined a unit to be 0.5 seconds, we can write some code to make a dash.
Instead of starting from scratch, notice how similar this is to our dot function. Let’s just copy, paste, and modify the code to make our dash function. Be sure to copy/paste first since we will still need to use the dot function as well. In the code below, I highlighted the three changes I made to my copy/pasted dot function to make it a dash.
Now to make the LED flash correctly, we can simply call the dash function 3 times after the S.
Simulate to test this functionality and you should see 3 short flashes, then 3 long flashes before the pattern repeats. We are almost there!!!! Our loop is much easier to read than it would be if we didn’t have our handy dot() and dash() functions. But if we needed to send a lot of letters, this would get pretty long. That’s when we need to abstract a bit more…
Step 7: In Class Challenge 3: Abstract Even More!
To simplify this further, let’s replace the S and O code here with two functions of the same names. To do this, I’m simply going to add declarations to the top of my code
void S(){
}//end S
void O(){
}//end O
Then copy and paste in my code from the loop into them as needed.
Now all we need in our loop is the following:
void loop(){
S();
O();
S();
}//end loop
This loop is much easier to read! Press Simulate to watch the flashing to make sure you can now identify your final SOS code. If something isn’t flashing correctly, you should be able to count the flashes from the moment you begin the simulation to know where to start debugging your code.
Step 8: Onward!
Imagine if you had to sentence in Morse code. You would need to make a function for each letter in the phrase “Hello world”, then call the letters in the correct order in the main loop to flash the message.
For example, here’s the code to flash a similar phrase, “Hey You”. I reformatted my S and O functions to save space as shown in the images.
The loop is easy to see what is going on (click to see the full second image with the code).
I’m keeping the functions for the letters in alphabetical order so you can fill in the other letters from the chart above. Once you have created a function for all letters (and numbers too if you’d like) you have just created what we call in coding a library of functions for Morse code. A library helps simplify and abstract concepts in programming to make it easier to accomplish tasks. in this case, we now have 2 levels of abstraction. We started with digitalWrite(). The next level was to cerate the dot() and dash() functions, and now we don’t have to think about dots and dashes, we can simply think about calling a function for each letter.
We will utilize libraries for more advanced designs which interface with other components that would otherwise require a lot of background knowledge to understand before using them.
Here is the final working code for flashing "Hey you" in Morse code:
Step 9: Homework
For homework, create a Morse Code library of functions and shall submit a narrated video of their Arduino flashing your name (at least 4 different letters long). Doing it on a real physical arduino with LED and breadboard will earn 5pts extra credit.
Step 10: In Closing
Now that you know how basic understanding of how Arduino code works and how libraries work in general, check out instructables and other project repositories for ideas for your next projects! You’ll notice a lot of them use libraries to interface different sensors and actuators.
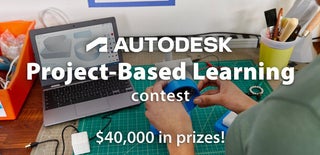
Participated in the
Project-Based Learning Contest