Introduction: Creating a Community Controlled Robot Using Discord Chat
I spend most of my day now managing the developer community for Viam Robotics on Discord, and hanging out with robotics enthusiasts and software engineers have given me a new perspective on the work I do as a Developer Advocate. Typically, my role involves crafting tutorials and demos to highlight our product’s features. After spending time with our community, I have been reflecting on how I can create more robot demos that can engage not just people in a room or someone reading a blog post, but how do I create something a whole online community can play with?
So, here’s a thought that’s been buzzing in my mind: How easy would it be to create a Robot Bot? Discord Bots are very common and pretty easy to make yourself thanks to awesome open source documentation in the Discord Developer Portal. Viam is an open source framework for building and programming robotics with flexible SDKs, so it is easy to build other interfaces on top of them.
So here I am going to show you how to control a physical robot running viam-server, all through simple DIY Discord chat commands in less than an hour.
Supplies
- A free account on app.viam.com
- A robot of your choice with a wheeled base- I used a Viam Rover
- A free Discord account
- A Discord server you manage, where you have permissions to deploy a Discord Bot
Step 1: Set Up a Real Physical Robot
- Build a robot. Yes, like an actual physical robot. I built a Viam rover with a wheeled base for simple movements.
- Prepare a board for viam-server installation. You can check out how on the Viam Docs site. I chose a Raspberry Pi for my robot. Create SSH credentials and log into your robot in your terminal.
- Set up a new robot on app.viam.com. If you don’t have an account, it is free to use and easy to sign up.
Step 2: Configure Your Robot on the Viam App
- Configure a robot, and test it in the control tab. I used a Viam rover, so setting up a pre-configured Fragment took just a few seconds. Head to the Control tab and make sure all your parts are working and you can move your base and see the camera.
Step 3: Prepare Your Development Environment
I used Python, and to manage Python packages efficiently I recommend creating a virtual environment.
- Create your project directory in your terminal and make sure you are in the right folders.
$ mkdir discordBot
$ cd discordBot
- Create your virtual environment and activate it.
$ sudo apt-get install python3-venv
$ python3 -m venv virtualenvironment
$ source virtualenvironment/bin/activate
- Install the necessary packages. I used viam-sdk and discord.py
$ pip install viam-sdk
$ pip3 install discord.py
Step 4: Prepare Your Discord Developer Configurations
After being logged into your Discord account, go to discord.com/developers/applications and select New Application. Name it and head to the Bot tab. I named this bot RobotBot (I know, very creative).
- Enable Privileged Gateway Intents permissions for MESSAGE CONTENT INTENT. This means you’re allowing the bot to send and receive messages. Set desired bot permissions as well, I picked Send Messages.
- Head to OAuth2 tab and select OAuth2 URL Generator
- Select Bot, and add specific bot permissions (Send Messages, Send TTS Messages, Manage Messages, Attach Files)
- Copy the URL and go to the URL in the browser.
- Add to authorized server
Step 5: Testing the Discord Bot
- Go back to the Bot tab in the Discord Developer Portal and reveal your token and copy it.
- Add to to the following script. This script is pretty boilerplate as a simple example of a Discord Chat Bot. I used environment variables to set the token.
import discord
import os
from discord.ext import commands
discord_token = os.getenv('DISCORD_TOKEN')
intents = discord.Intents.default()
intents.message_content = True
bot = commands.Bot(command_prefix='/', intents=intents)
@bot.command()
async def hello(ctx):
await ctx.send('hello from robotbot')
bot.run(discord_token)
Run your code, your bot should go online in your Discord server.
Now say hello in the chat, your Discord bot will greet you back if the connections are all correct.
Step 6: Add Your Robot Controls to the Discord Bot Using Viam’s Python SDK
Integrate Viam robot code into your discord bot. Create a bot command and corresponding robot movement. I grabbed the boilerplate code from the Code Sample tab in the Viam App, and removed components I was not using for the sake of this example. I am only using the Base API.
Here are some examples of methods in the Base API that can help simply control my rover. I am going to use the MoveStraight() method.
I’ve used Environment Variables to set my API Key and Robot Address which are unique to your robot. Those can also be conveniently found in the Code Sample tab.
Create a forward() function (or really any movement you want) following the same logic. This logic can apply to any robot functionality you want to write using a Discord Bot to control a Viam robot. Here I am describing what is happening in async def forward(ctx):
- Use the @bot.command decorator to start off your function. These decorators are used to register functions as bot commands, and they can’t be nested inside other functions. They should be used at the top level in your script.
- Create a variable in scope of that function for your component you want to control.
- Write the command response for the bot to auto reply in discord.
- Write the function that moves the robot.
import discord
import asyncio
import os
from viam.robot.client import RobotClient
from viam.rpc.dial import Credentials, DialOptions
from viam.components.base import Base
from discord.ext import commands
api_key = os.getenv('API_KEY')
api_key_id = os.getenv('API_KEY_ID')
robot_address = os.getenv('ROBOT_ADDRESS')
discord_token = os.getenv('DISCORD_TOKEN')
intents = discord.Intents.default()
intents.message_content = True
bot = commands.Bot(command_prefix='/', intents=intents)
async def connect():
opts = RobotClient.Options.with_api_key(
api_key,
api_key_id
)
return await RobotClient.at_address(robot_address, opts)
@bot.command()
async def forward(ctx):
robot = await connect()
viam_base = Base.from_robot(robot, "viam_base")
await ctx.send('i`m going forward')
await viam_base.move_straight(distance=10, velocity=50)
await viam_base.stop()
@bot.command()
async def hello(ctx):
await ctx.send('hello from robotbot')
bot.run(discord_token)
Step 7: Make Your Robot Move With Discord Chat Commands
Easy peasy. Run your code, and the bot will instantly go online. Type in your command in the chat
Your robot should be moving!
You can apply this logic to control any robot functions using Viam and a Discord Bot. This simple project was completed in under an hour, demonstrating the power of open source and the true flexibility of Viam’s SDK’s. For my next iteration of this project, I think it would be pretty fun and chaotic to let a server of thousands of people drive this rover around my house. Maybe I should create a SLAM map of my house and use Viam’s Motion Planning to make this project a bit more robust.
Let me know if you try this! You can find me and our amazing developer community on Discord — tag your projects in #built-on-viam to be featured on our socials.
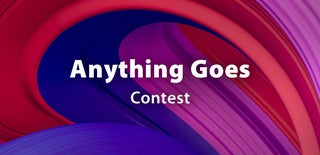
Participated in the
Anything Goes Contest