Introduction: ESP32 Scrolling WordClock on LED Matrix
In this project I create a Scrolling WordClock with an ESP32, LED Matrix and a cigar box.
A WordClock is a clock that spells the time rather than just print it on the screen or have hands you can read. This clock will tell you it's 10 minutes past 3 o'clock in the afternoon, or noon. I even programmed it to use the Prevening (from Big Bang Theory) the name for the ambiguous period of time between afternoon and evening. Starts 4:00 PM.
The ESP32 is awesome, they are so much fun and so inexpensive, If you love programming Arduino's you will really be amazed at what an ESP32 can do for under $10. They use the Arduino IDE and are easy to program. I will demonstrate how to do that in this instructable.
Supplies
- ESP32 - around $10 on amazon
- LED Matrix (max7219) (and wires) - $9 amazon
- Cigar box
- USB power cable
- Optional 3d printer for esp32 stand
Step 1: Setup Your Arduino IDE to Support ESP32
Get the Arduino IDE:
- In a browser go to https://www.arduino.cc/
- Click on Software then Downloads to get ARDUINO 1.8.12
Add ESP32 Support to your Arduino IDE:
- Start the Arduino IDE
- Click on the File Menu then Preferences.
Once in "Preferences" add the manufacturer support by adding the following line to the "Additional Board Manager"
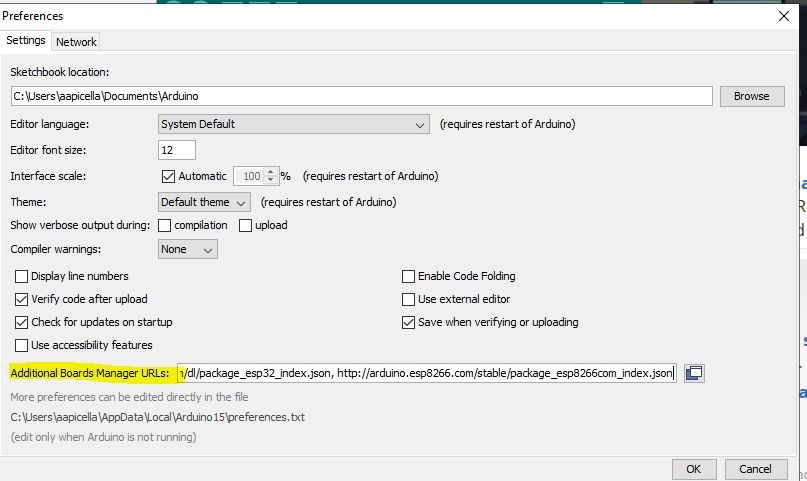
https://dl.espressif.com/dl/package_esp32_index.js
This will give us access to add the board to the IDE
Go to the Tools Menu and then Boards and go to the board manager
Next search for "ESP" and add the package by Expressif.
Lastly we want to go back to the "Tools" Menu, then "Board" again and scroll down to find your ESP32 device.
Mine is a "ESP32 Wrover Module"
Step 2: Connect the LED Matrix to the ESP32
The LED matrix is made up of four 8x8 blocks of LEDs and uses a MAX7219 chip. That gives us 8x32 LEDs in the Matrix or 256 LEDs!!!.
The esp32 is sitting on a hold I created in TinkerCad. My holder holds the pins facing up so you can attach wires.
The LED Matrix connects to the ESP32 using SPI (Serial Peripheral Interface).
Use Female/Female Wires and Connect pins as follows:
- ESP32 - 5v to VCC on Matrix
- ESP32 - GND to GND on Matrix
- ESP32 - PIN5(G5) to CS on Matrix
- ESP32 - PIN23 (G23) to Din on Matrix
- ESP32 - Pin 18 (G18) to CLK on Matrix
It is possible to use other pins or if your ESP32 has a different pinout.
Attachments
Step 3: Putting It Together
Next I cut the cigar box to give me a place to put the LED display. The box is soft and I was able to use a razor knife. Then I sanded to smooth it out.
I also cut a whole in the back for the power cord. I just used USB power. I could have even added a USB phone charger battery inside the cigar box if I had wanted it to be wireless.
Step 4: The Arduino IDE and INO File.
The best part is the code. But first we must add a library to allow the program to use the display in a more simple way. I added the MD_MAX72xx library.
To add the library click on the "Sketch" menu, then "Include Library" and "Manage Libraries" this will load and allow you to search for MD_MAX72xx library. Just click on install and you have it.
Next get my Arduino INO File:
https://github.com/aapicella/wordClock/blob/master/Word_Clock_LED_NTP_final_.ino
Load the ino file into your Arduino IDE,
Connect the USB from the ESP32 to your computer.
Click on the "Sketch" then Upload
At this point the clock will not work, the ESP32 will not display anything. Why? we need to add your WIFI to the code because the WordClock connects to the internet to get the time. Thats right...next step ->.
Step 5: The Code
We finally made it to my favorite part. The program. I will cover it from top to bottom if you are interested, To get the program to work with your home internet you just need to change these lines.
// Add your network information
const char *ssid = "xxxxxx";
const char *password = "xxxxxx";
The program is kinda complex, but very fun.
To disable "Prevening" just change the value to false:
const boolean PREVENING=true; //Big Bang theory. <a href="https://www.urbandictionary.com/define.php?term=prevening"> https://www.urbandictionary.com/define.php?term=p...>
To disable displaying the digital time after scrolling change this to false.
const boolean DISPLAY_DIGITAL=true; //turn on displaying digital time after scrolling.
Scrolling the Time:
- I get the time from a time server on the internet using NTP (Network Time Protocol). The time is held in a variable called timeinfo and we get the hour and minute from it.
int hour = timeinfo.tm_hour; ///0-23
int minute = timeinfo.tm_min; //0-59
- Next check AM or PM
Its AM if hour < 12
- I create a string called "theTime" and start it with:
theTime="It's ";
- To get the number as words, I created an array of words for numbers up to 30.
const char *numbers[] = { "0", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Quarter", "Sixteen", "Seventeen", "Eighteen", "Nineteen", "Twenty", "Twenty-One", "Twenty-Two", "Twenty-Three", "Twenty-Four", "Twenty-Five", "Twenty-Six", "Twenty-Seven", "Twenty-Eight", "Twenty-Nine", "Half Past"};
So when its 12:05 or five minutes past twelve its really to the Arduino
numbers[5] minutes past numbers[12]
To determine if its "Minutes Past" or "Minutes To" we just look at the minutes. If the minutes are < 31 it is "Minutes Past" if the minutes are greater than 31 we use "Minutes To" but use numbers[ 60-minutes] so 12:50 would be 60-50 minute or numbers[10] which would give us 10 Minutes To 12 0'clock.
Of course there are other rules like on 15,30,45 we don't use minutes its just half past or quarter to, and if the minute is 0 the time is just "Ten O'clock" or Noon.
So to put it together, I append all the items to the String theTime then display it on the LED Matrix. I use a bunch of If statements. I probably should have used case but it was just easier to keep adding them.
For our example 12:05
theTime="It's "
if minute < 31 the use "Minutes Past" otherwise its "Minute To"
theTime+= numbers[5 ] + " Minutes Past" + number [12] + "O'Clock" //Note: The notation += is append.
Next we look at the hour to determine if its morning, afternoon or evening.
theTime+= "In the Evening"
The final String going to the matrix is:
"It's Five Minutes Past Twelve O'Clock in the Evening"
This project was very fun to write. If you like it please click the Heart and Vote for me please.
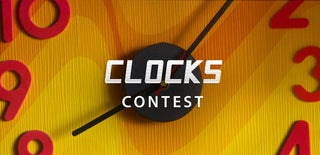
Participated in the
Clocks Contest