Introduction: Arduino With Dual Motor Tank Coded in TinkerCad Codeblocks and L293D Driver Chip
This instructable will use TinkerCad to design a circuit for a dual-motor tank, then create the program to run the tank with TinkerCad CodeBlocks.
TinkerCad Circuits is a free site that allows you to design, build and code circuits, and even includes Arduino's.
One of the interesting challenges with Arduino's and motors is how to make them go both forward and reverse. Since the Arduino can only provide power in one direction. To accomplish this we can use a motor driver. In this instructable I use a L293D Dual H-Bridge motor driver. Some advantages of this chip are:
- It can drive two small DC motors at the same time independently.
- The motors can move in either direction
- Power can be provided to the chip independent of the Arduino.
- Two outputs from the Arduino control each motor.
- The Arduino may also use a PWM output to control speed.
Fortunately, TinkerCad Curcuits includes these IC chips in its software, and its FREE
Supplies
This is a virtual demonstration that will download Arduino code at the end.
Supplies:
- A browser just like the one you are currently on.
Optional: ( if you want to build the tank):
- Tamiya 70168 Double Gearbox L/R Independent 4-Speed ( amazon)
- Tamiya Tracked Vehicle Chassis Kit (amazon)
- Arduino UNO R3
- Arduino Uno Prototype board or Mini breadboard.
- Battery holder with batteries ( 4AA or a 9volt) - connect the battery to Vin on the Arduino.
- Solder and Soldering Iron might be needed to attach wires to the motor.
- Wires.
- L293D Dual H-Bridge Driver Chip
- LEDs and 220Ohm resistors are used in the example so you can tell if you make a mistake in wiring.
Step 1: Login to Tinker Cad and Pick Circuit
Login or Join TinkerCad. TinkerCad will let you join with your existing google or apple accounts!
then "Create a new Circuit" project.
Step 2: Building the Circuit
Here is my Tinkercad CodeBlocks example.
Build the circuit:
- Drag out a breadboard. Small is fine.
- Drag out an Arduino Uno
- Put two LEDs on the board.
- I use one for power. Its color is green and its on the whole time.
- A Blue one we turn on each time the motors are turned on.
- Drag out two resistors
- set value to 220 ohm
- Rotate them 90 degrees
- Set these so one end it on the cathode side of the LED. You can mouse over the LED terminals to see it.
- Next Search on DC Motor and pull out two one on top and one on bottom.
- Name them Right and left.
- Search on L293D and put it in the exact middle of the breadboard so it straddles the grove in the board.
- At this point I also connect the rails on each side of the breadboard, so we can use both rails equally.
- + to + with red wire
- - to - with green wire
Step 3: Wire Up the LEDs
Wire the project:
- Connect Arduino GND to the - rail on the breadboard. ( any of the GND's will work).
- Connect Arduino 5V to the + rail on the breadboard. (if using a separate voltage for the motors connect it here instead, but also connect ground to the - rail on the breadboard along with the Arduino ground.
- Connect Arduino Pin13 to the anode of the Blue LED with a blue wire.
- Connect the - rail to the end of the resistor connected to the cathode side of the led. Use a green wire.
- Using a red wire connect the + rail to the Anode side of the Red LED.
- Using a green wire connect the - rail to the resistor connected to the cathode side of the Red LED.
- This should now light when you click simulate.
Step 4: Wire Up the L293D Dual H-Bridge Driver
Wiring up L293D:
To understand the wiring you need to see the pinout of the L293D chip. TinkerCAD will show you pin names when you mouse over them.
- Connect the first pin to the + rail with a red wire. The first pin is labeled "Enable 1 & 2"
- Connect the last pin to the + rail with a red wire. The pin is labeled "Enable 3&4"
- With a green wire connect the four ground pins to the - rail on the board.
- Connect "Power 1" pin to the + rail using a red wire.
- Connect "Power 2" pin to the + rail using a red wire.
- On the Right motor:
- Connect the Red DC motor pin to the "Output 1" pin on the L293D
- Connect the Black DC motor pin to the "Output 2" pin on the L293D
- On the Left motor:
- Connect the Red DC motor pin to the "Output 3" pin on the L293D
- Connect the Black DC motor pin to the "Output 4" pin on the L293D
Connect Arduino to the L293D Dual H-Bridge Driver:
We use 4 pins on the Arduino. Pins are 3,4,10,11.
- Arduino pin 3 connects to L293D pin "Input 4"
- Arduino pin 4 connects to L293D pin "Input 1"
- Arduino pin 10 connects to L293D pin "Input 2"
- Arduino pin 11 connects to L293d pin "Input 3"
Step 5: Program the Arduino
Finally we are ready to program the Arduino and make our tank move.
Click the "</code" button and pick both Blocks and Text. The text is read only but its good to see the program being created.
Clear the sample block by draggin it to the trash.
First I click on the "Notation" section and pull out a title block.
- I add comments here. (hint use /n to move to the next line "newline" to the comment if needed)
- I add my name and date and comments I might need.
I also add a comment to remind me how the motor driver works:
RIGHT WHEEL pin4 Pin10 Spinning Direction Low(0) Low(0) Motor OFF High(1) Low(0) Forward Low(0) High(1) Backward High(1) High(1) Motor OFF LEFT WHEEL pin3 Pin 11 Spinning Direction Low(0) Low(0) Motor OFF High(1) Low(0) Forward Low(0) High(1) Backward High(1) High(1) Motor OFF
So in pin 4 and 11 are the same the motor stops and different the motor moves.
Next I created a variable called "wait" by clicking on variabled and putting in wait in the name. This will be the delay we use between each action. CodeBlocks will automatically initialize it to 0;
Set the wait value to 5 and drag it under the comments. This is our first line of code.
I then add the Comment "Move Forward"
Now we set the outputs to move the Tank forward.
- Click on the Output Block.
- Add "set 10 to Low"
- Add "set 11 to Low"
- Add "Set 3 to High"
- add "Set 4 to High"
- The go to control block and pick "Wait 1 secs"
- Click on the Variables Block
- Pick the round wait variable and drop it on the 1 in the Wait 1 secs.
- We can now control the wait.
Next we need to stop it. The easiest way is to copy the above block except the "Set wait to 5"
We copy by right clicking on the Comment "Go Forward" and then click on "Duplicate" Paste the code at the end of the program.
- Change comment "Go Forward" to Stop.
- Change set Built-in LED to LOW ( blue led off when stopped).
- Set Pin 3 LOW
- Set Pin 4 LOW.
If Pin 3,4,10 and 11 are the same the tank will stop.
wait 5 seconds then restart.
Next we can program the Tank to pivot turn by making the right motor go in reverse and the left motor go forward.
You can duplicate the whole block so far since we want to stop after turning. Right click on the "Go Forward" comment and paste at the end of the code.
- change the comment "Go Forward" to "Turn Right"
- Set pin 10 HIGH
- Set pin 4 to LOW ( right motor in reverse).
- Leave the rest of the code the same.
The DC motors will show RPMS one should be negative and one positive. When wiring the tank you may need to reverse one or both motors to get forward and reverse to be correct.
The new code segment looks like:
Step 6: What Next, How About Speed?
While running the code you may have noticed your motors run at a standard speed. We can adjust the motor speed by adding a wire to the Enable 1&2 (pin1), and Enable 3&4 (pin 9) on the L293D chip.
If we connect those pins to the Arduino we can now change the speed of the motor by increasing the value of the output. The Arduino allows us to go from 0-255, where 0 is no voltage and 255 is 5v. Although the Arduino does only send out 0 or 5v to make the range lower it creates square waves call Pulse Width Modulation or PWM.
- Remove wire on this pin, and connect Arduino pin 5 to Enable 3&4 (pin 9) on L293D
- Remove wire on this pin, and connect Arduino pin 6 to Enable 1&1 (pin 1)on L293D
We will initialize pins 5 and 6 to 0 then create a loop that will increase the value ever 100 milliseconds.
This type of loop is a "For Loop" for counter goes from 0 to 255 by 1increment per loop.
- In the loop we set Outputs 5 and 6 to the Counter value.
- the first loop sets the outputs to 1 the second loop is two.
Here is the whole project finished.
If you enjoy these types of instructables let me know.
Good luck and have fun!
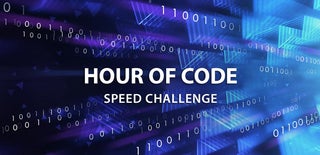
Second Prize in the
Hour of Code Speed Challenge