Introduction: Lily∞Bot With Raspberry PI Pico W: Obstacle Avoidance With 3.3V Sonar Sensor
I updated the open-source robot, Lily∞Bot to work with the Raspberry PI Pico W programmed in MicroPython.
Supplies
- Lily∞Bot robot from NoireSTEMinist.com/shop
- Or build the Lily∞Bot using the CAD and 3d printer files on Github at https://github.com/berry123/Lily-Bot
- Microcontroller should be a Raspberry PI Pico W
- Need one LED with 220 ohm resistor
- One HC-SR04 3.3V sonar sensor
Step 1: Wire Up the Circuit
- The figures below the text indicate how to connect circuit elements using the nodes on the breadboard, the pinout for the Raspberry Pi Pico W and the Fritizing schematic for this circuit.
- Use the following steps to wire the TB6612 motor driver, sonar and LED to the Raspberry PI Pico W
- Put the TB6612 motor driver in one end of the breadboard with the USB connection at the end near the end of the breadboard.
- Connect the left motor to motor A (red, blk) on the TB6612
- Connect the right motor to motor B (red, blk) on the TB6612
- Use two wires to connect VCC and VM on the TB6612 to VBUS on the Raspberry PI Pico W. One option is to put VBUS on a row of the breadboard and use wires to connect there.
- Use a wire to connect PWMA on the TB6612 motor controller to pin 28 on Raspberry PI Pico W
- Use a wire to connect AIN2 on the TB6612 motor controller to pin 27 on Raspberry PI Pico W
- Use a wire to connect AIN1 on the TB6612 motor controller to pin 26 on Raspberry PI Pico W
- Use a wire to connect BIN1 on the TB6612 motor controller to pin 22 on Raspberry PI Pico W
- Use a wire to connect BIN2 on the TB6612 motor controller to pin 21 on Raspberry PI Pico W
- Use a wire to connect PWMB on the TB6612 motor controller to pin 20 on Raspberry PI Pico W
- Use these steps to wire up the LED and sonar circuit before writing the program.
- The following first two circuits show the nodes for meeting points on a breadboard and the Raspberry PI Pico W wiring diagram
- Put the 220 ohm resistor on the breadboard with each leg in a different row.
- Put the LED in the breadboard with the short leg on the same row as the 220 ohm resistor
- Put the long leg of the LED on a different row and connect the long leg of the LED to pin 18
- Use a wire to connect the other leg of the 220 ohm resistor to ground.
- Use the sonar mount to connect the sonar to the front of Lily∞Bot.
- Use a wire to connect the VCC pin on the sonar to the 3.3 V (OUT) pin on the Raspberry PI Pico W. This pin is 5th from the top on the right
- Use a wire to connect the GND pin on the sonar to any ground pin on the Raspberry PI Pico W.
- Use a wire to connect the TRIG pin on the sonar to pin 17 on the Raspberry PI Pico W.
- Use a wire to connect the ECHO pin on the sonar to pin 16 on the Raspberry PI Pico W.
Step 2: Write the Code
- Open up PlatformIO Visual Studio and create a.py file
- Configure the file as a MicroPico project
- Copy the file code into the file and then save.
- Right click on the file name and select "Run current file on Pico"
#obstacleAvoicance.py CAB 9.17.23
#This code will drive the LilyBot forward
#then turn when obstacle is detected with sonar
#https://www.noiresteminist.com/shop
#Carlotta Berry 9.14.23
from machine import Pin, ADC, PWM
from utime import ticks_us, sleep_us, sleep_ms
#define inputs and outputs
ledPin = 18
triggerPin = 17
echoPin = 16
trigger = Pin(triggerPin, Pin.OUT)
echo = Pin(echoPin, Pin.IN)
led = Pin(ledPin, Pin.OUT)
pin = Pin("LED", Pin.OUT)
#define inputs and outputs
PWMA = PWM(Pin(28))
AIN2 = Pin(27, Pin.OUT)
AIN1 = Pin(26, Pin.OUT)
PWMA.freq(60)
BIN1 = Pin(22, Pin.OUT)
BIN2 = Pin(21, Pin.OUT)
PWMB = PWM(Pin(20))
PWMB.freq(60)
motorSpeed = 65535
def reverse():
AIN1.value(1)
AIN2.value(0)
BIN1.value(1)
BIN2.value(0)
PWMA.duty_u16(motorSpeed)
PWMB.duty_u16(motorSpeed)
def forward():
AIN1.value(0)
AIN2.value(1)
BIN1.value(0)
BIN2.value(1)
PWMA.duty_u16(motorSpeed)
PWMB.duty_u16(motorSpeed)
def pivot():
AIN1.value(0)
AIN2.value(0)
BIN1.value(0)
BIN2.value(1)
PWMA.duty_u16(motorSpeed)
PWMB.duty_u16(motorSpeed)
def stop():
AIN1.value(0)
AIN2.value(0)
BIN1.value(0)
BIN2.value(0)
def distance():
timepassed=0
signalon = 0
signaloff = 0
trigger.low()
sleep_us(2)
trigger.high()
sleep_us(5)
trigger.low()
while echo.value() == 0:
signaloff = ticks_us()
while echo.value() == 1:
signalon = ticks_us()
#print(signalon)
#print(signaloff)
timepassed = signalon - signaloff
#print(timepassed)
dist_cm = (timepassed*0.0343)/2
if dist_cm>60:
dist_cm=60
return dist_cm
print("Obstacle Avoidance on Lily∞Bot...")
while True:
reading = distance()
print(reading)
if reading<10:
led.value(1)
stop()
sleep_ms(100)
reverse()
sleep_ms(500)
pivot()
sleep_ms(500)
reading = distance()
else:
led.value(0)
forward()
sleep_ms(100)
reading = distance()
Attachments
Step 3: Verify It Works
- Watch the following video to confirm that your circuit is working correctly.
- If it is not, try to debug the code and trouble shoot the circuit wiring.
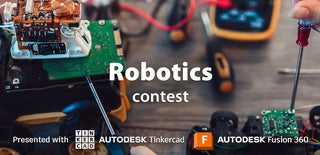
Participated in the
Robotics Contest