Introduction: Plant Monitoring Using Alexa
In this project we will be using Arduino, Raspberry Pi and Alexa for voice controlled IOT system of a plant monitoring device.
This system can measure the temperature and light density of the surrounding in this plant and give the values to anyone who accesses this skill.
The sensors are connected to the Arduino to read the analog values coming from the sensor. These sensor values are converted into digital values and are sent serially to the raspberry pi.
The raspberry pi has a server for Alexa running in it. The values are attached to the invoked indents user asks for.
Thus it solves for plant monitoring using voice commands.
This is a sample project and you can expand the project to connect any number of sensors to the arduino or Raspberry Pi to solve your problem.
Let's get through the steps involved in the prototyping of the plant monitoring system.
Step 1:
Step 2: Hardware Requirements
Hardware used
· Arduino uno
· Raspberry pi
· Temperature sensor
· LDR voltage divider
Arduino Uno
Arduino Uno is a microcontroller board based on the ATmega328P (datasheet). It has 14 digital input/output pins (of which 6 can be used as PWM outputs), 6 analog inputs, a 16 MHz quartz crystal, a USB connection, a power jack, an ICSP header and a reset button. It contains everything needed to support the microcontroller; simply connect it to a computer with a USB cable or power it with a AC-to-DC adapter or battery to get started.
Raspberry Pi 2
Raspberry Pi 2 is a “Model B,” though there is no Model A yet for the second generation hardware.
Here is the full list of specs for Raspberry Pi 2 Model B:
SoC: Broadcom BCM2836 (CPU, GPU, DSP, SDRAM)CPU: 900 MHz quad-core ARM Cortex A7 (ARMv7 instruction set)GPU: Broadcom VideoCore IV @ 250 MHzMore GPU info: OpenGL ES 2.0 (24 GFLOPS); 1080p30 MPEG-2 and VC-1 decoder (with license); 1080p30 h.264/MPEG-4 AVC high-profile decoder and encoderMemory: 1 GB (shared with GPU)USB ports: 4Video input: 15-pin MIPI camera interface (CSI) connectorVideo outputs: HDMI, composite video (PAL and NTSC) via 3.5 mm jackAudio input: I²SAudio outputs: Analog via 3.5 mm jack; digital via HDMI and I²SStorage: MicroSDNetwork: 10/100Mbps EthernetPeripherals: 17 GPIO plus specific functions, and HAT ID busPower rating: 800 mA (4.0 W)Power source: 5 V via MicroUSB or GPIO headerSize: 85.60mm × 56.5mmWeight: 45g (1.6 oz)
Temperature sensor (lm35)
LM35 is a precision IC temperature sensor with its output proportional to the temperature (in oC). The sensor circuitry is sealed and therefore it is not subjected to oxidation and other processes. With LM35, temperature can be measured more accurately than with a thermistor. It also possess low self heating and does not cause more than 0.1 oC temperature rise in still air. The operating temperature range is from -55°C to 150°C. The output voltage varies by 10mV in response to every oC rise/fall in ambient temperature, i.e., its scale factor is 0.01V/ oC.
LDR
A photoresistor (or light-dependent resistor, LDR, or photoconductivecell) is a light-controlled variable resistor. The resistance of a photoresistor decreases with increasing incident light intensity; in other words, it exhibits photoconductivity. A photoresistor can be applied in light-sensitive detector circuits, and light- and dark-activated switching circuits.
Step 3: Connections
LDR voltage divider
A light-dependent resistor (LDR) connects to a voltage divider circuit, also known as a potential divider (PD), for proper circuit operation. There are two configurations of the circuit depending upon the position of the LDR within the potential divider network.
You can have a configuration where the voltage output (Vout) increases as light increases or one where the voltage output decreases as light increases. The following calculators and their respective formulas show how both configurations work
Vout is connected to arduino analog pin 1
Temperature sensor vout is connected to arduino analog pin 0
Step 4: Arduino Code
You have to upload this arduino code into the arduino uno board
int ldr = 1;
int temperature = 0;
int tempc;
void setup() {
Serial.begin(9600);
}
void loop() {
float val1 = analogRead(0);
tempc =(5 * val1 * 100) / 1024.00;
Serial.print(tempc);
int val2 = analogRead(1);
val2 = val2 * 0.10;
Serial.println(val2);
delay(1000);
}
Step 5: Interfacing With Raspberry Pi
Step 6: Raspberry Pi Package Installations
I used a Raspberry Pi 3 and a fresh Raspbian Jessie-lite image downloaded from https://www.raspberrypi.org/downloads/raspbian/
Two terminal sessions will be needed so using SSH to access the Pi is recommended. Once logged in, enter the following commands to install the required packages and python libraries:
sudo apt-get update && sudo apt-get upgrade -y sudo apt-get install python2.7-dev python-dev python-pip
sudo pip install Flask flask-ask
Step 7: Ngrok Setup
ngrok is a command-line program that opens a secure tunnel to localhost and exposes that tunnel behind an HTTPS endpoint. ngrok makes it so Alexa can talk to your code right away. Follow the next three steps to generate a public HTTPS endpoint to 127.0.0.1:5000.
Visit https://ngrok.com/download and get the latest Linux ARM release as a zip and unzip inside the home directory:
unzip /home/pi/ngrok-stable-linux-arm.zip
Next, run it from the command line with:
sudo ./ngrok http 5000
Your screen should look like the image above. Note the 'Forwarding' URL that starts with https, it will be used later. Note: Unfortunately the ngrok URL changes every time the service is started so it is not a permanent solution if you are trying to run this full time. I'd recommend a service like Yaler or Page Kite if you need a more permanent URL to use with your new Skill.
Step 8: Raspberry Pi Code
Open a new terminal session and create a new python file named plant.py:
nano plant.py
Copy/paste the following code into the new file:
from flask import Flask
from flask_ask import Ask, statement, convert_errors
import RPi.GPIO as GPIO
import logging
import serial
import time
temp = 0
light = 0
serial1 = serial.Serial('/dev/ttyUSB0',9600)
def caller1():
read_data = 0
read1 = serial1.readline()
read_data = read1.rstrip('\n\r')
n1 = str (read_data)
n2 = str (read_data)
temp = n1[:2]
print temp
light = n2[2:]
return(temp)
def caller2():
read_data = 0
read1 = serial1.readline()
read_data = read1.rstrip('\n\r')
n2 = str (read_data)
light = n2[2:]
return(light)
app = Flask(__name__)
ask = Ask(app, '/')
logging.getLogger("flask_ask").setLevel(logging.DEBUG)
@ask.intent('temperature')
def temperature():
x = 'The temperature is ' + caller1()
return statement(x)
@ask.intent('light')
def light():
x = 'The intensity of light is ' + caller2()
return statement(x)
if __name__ == '__main__':
app.run(debug=True)
Save and close the file.
Start the flask server with:
sudo python gpio_control.pyLeave both ngrok and plant.py
running while we setup the new skill in AWS...
Step 9: Amazon Website Configurations
First create or login to your AWS Developer Account and open your list of Alexa skills.
Step 10: Skill Sheet Page
Set the Skill Name to 'plant monitor' and the Invocation Name to the word(s) you want to use to activate the skill. Click 'Next' to continue.
Step 11: Interaction Model
Build the interaction model with the following indents mentioned in the image. This can help us to invoke the actions with respect to the commands a user says.
Step 12: Configuration Page
Select 'HTTPS' as the Service Endpoint Type and select a region. Enter the ngrok URL from step 2 and click 'Next'. The URL should be something like:
<a href="https://ed6ea04d.ngrok.io" rel="nofollow">https://ed6ea04d.ngrok.io </a>
Select the 'My development endpoint is a sub-domain of a domain that has a wildcard certificate from a certificate authority' option and click 'Next'.
Step 13: Testing Page
If everything was setup correctly you should now see a screen similar to the first image above. The Skill is now enabled and can be accessed through any Amazon Echo device(or at http://echosim.io/) that is connected to your AWS Developer Account using the following syntax:
Alexa, ask plant to tell the temperature
As far as I can tell there is nothing stopping someone from running this continuously in a 'development' mode for personal use though... My ultimate goal is to use this to integrate Alexa into my existing Raspberry Pi-based Smart Home setup so that everything can be controlled hands-free. This was my first Instructable so any feedback is appreciated. If you have any questions or trouble setting this up I will do my best to help. Good luck!
Step 14: Output Video
Thank you.
If you like this instructable, please like share and vote me.
Happy prototyping.
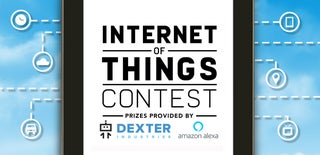
Judges Prize in the
Internet of Things Contest 2017
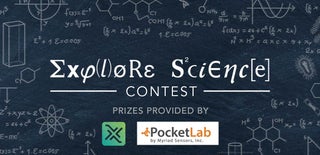
Participated in the
Explore Science Contest 2017