Introduction: Program a Servo, Build a Catapult, and Solve for Π (on a Raspberry Pi) (EASY!)
So, you've built your 'Hello World' blinky light and want to do something a little more useful and practical? Maybe you're just learning how to code for the first time? Maybe you just want a fun activity for the kids this pi day? Any way you look at it this project is for you, and it takes no prior experience to be completely successful!
I will provide all the code, the wiring diagram, the printer files, and the launch range, so you can just copy and paste it all in and you're good to go.
The end result is launching projectiles ~2 feet to determine an approximate value for π (3.14, or to be more accurate in our case 22/7)
Supplies
1x Raspberry Pi (You can use any microcontroller you're comfortable with)
3x Jumper Wires (Brown, Red, and Orange preferable)
1x Servo (I'll be using a sg90. You can get these 5 for $9 on amazon)
2+ toothpicks
2x sheets of printer paper
Tape
EITHER: 1 x small stick (half size skewer), OR 3D printer with filament and M2 screws
Step 1: Gather Your Supplies
SERVO:
The servo I'm using came as part of a kit, and likely if you've bought a kit to go with your Raspberry Pi, or microcontroller you probably have one already. It's the SG90, and can easily cost less than $2-3 if you don't have one already
RASPBERRY PI:
The raspberry pi is a small Single Board Computer (SBC) a little bigger than a credit card. It has all the power you need to run your computing needs (web, e-mail, word processor, etc.) for as low as $35. You'll need some other supplies to go with it (power cable, SD card mouse, keyboard, monitor (you can also control it from your computer remotely)). What we're most interested in is the GPIO (General Purpose Input Output pins) that are on the right side of the board. I'll be using a RP4 with 2GB of RAM, but any will do. You can also perform similar steps with any microcontroller, but that won't be covered here.
JUMPER WIRES:
I am using male/female jumpers. The female end goes on the GPIO on the RPi, while the male end goes in the servo. Color doesn't matter, but usually black/white/brown/green are ground, red is power, and other colors are data.
TOOTHPICKS:
These will be our munition when firing the catapult. Use anything long/skinny for this project. I tape two of them together to prevent them from rolling in the direction of their inertia. You may also use one as a crossmember on your catapult.
3D PRINTER:
This is OPTIONAL. If you do have access to this I'll provide the STL files for you. If you go this route you'll need some M2 screws, a screwdriver, and something heavy to put on the base (I used a pumpkin pi filling can). I used 6mm and 10mm screws, but many sizes will work. (If you're going to use this I would start printing now so it's done when you're onto that step)
SMALL STICK:
You can use anything light/rigid including an actual branch from an actual tree. I used a half size skewer we usually use for fruit on my prototype to see if the servo would work for this project, and it worked great. If you go this route you might need another toothpick or two to make your launcher, and some more tape. You can hold the servo down, or tape it down. this is BY FAR the faster easier route, but why wouldn't you use your 3d printer if you have one?
Step 2: Hook Up the Servo
Wiring with the jumper wires is SUPER Easy. This step is best done with the RPI off. I used the same color wires as my servo for simplicity. Slide the female wires over jumper pins 2 (5.5V power - red), 3 (GPIO pin, will control the servo - orange), and 9 (ground, GND - brown).
Hint: with the boards ports facing you you count top to bottom left to right just like reading a book
1 2
3 4
5 6
7 8
9 10
...
Once you have the wires hooked up to your pi slot the male ends into the servo.
If you've purchased a different servo you might need to look at the data sheet to see which color wires go where, but it should be very similar. You'll have to ensure if you're using different microcontrollers or servos that you wire things correctly, and have purchased items with the right power requirements. You may also run the servo from a standalone power supply. Just make sure to ground them all together.
Step 3: Time to Write Your Code!
OK, I said write, but really you can just copy/paste it in. I won't cover setting up your raspberry pi in this servo tutorial. There are many great references that cover this already. Google is your friend. Once your pi is up and running launch Thonny (or your preferred coding environment) and get started.
As you can see in the picture there is not a whole lot of code there. Basically, I set everything up, then repeatedly raise/lower the arm of the catapult! There are much simpler ways to do this, but this allows more flexibility, and lets you work through some issues that may arise.
The most important thing here is that we're firing our ammunition - specifically that we're randomly firing it because that will be required to solve for pi.
A COUPLE NOTES:
1. 90 degrees would be 1.5 per the spec sheet. since I'm pausing the code for a set period of time for my pulses you might think i should put in 1.5. I used 1.4 because all the other things that the code is doing between raising and lowing the GPIO power take time too. 1.4 worked great for me to get 90 degrees. you can tweak this number if you're not going to 90 degrees.
(It was actually pretty cool to switch to multitasking on the pi and watch the arm freak out as all these 'other tasks' slowed down the pulses to our servo. It was a live haptic feedback for our processor load)
2. I chose the time high so that it would DELIBERATELY not get all the way to the top all of the time. when things weren't random I found that .3 seconds is all it took to go all the way to the top. I cut this in half so that it could randomly stop at any point on the way up for it's shot. If you tweak this number make sure you're getting about half of the time required to go to a full 90. Guess check and revise works great (turn the random off if you do this).
3. The reason I didn't use a simpler library implementation was that I ran into issues where my arm wasn't going to the positions I desired. Now you're in full control!
YOU CAN COPY/PASTE THE CODE BELOW OR DOWNLOAD THE FILE. THE FILE HAS COMMENTS THAT EXPLAIN WHAT EACH STEP OF THE CODE DOES.
import RPi.GPIO as GPIO import time import random ServoPin = 3 DutyCycleHigh = 1.4 DutyCycleLow = .2 Period = 20 TimeHigh = .15 TimeLow = 2 GPIO.setmode(GPIO.BOARD) GPIO.setwarnings(False) GPIO.setup(ServoPin, GPIO.OUT) while True: for i in range(int(TimeHigh*random.randrange(1,4)*1000/(Period*2))): GPIO.output(ServoPin, GPIO.HIGH) time.sleep(DutyCycleHigh/1000) GPIO.output(ServoPin, GPIO.LOW) time.sleep((Period-DutyCycleHigh)/1000) for i in range(int(TimeLow*1000/Period)): GPIO.output(ServoPin, GPIO.HIGH) time.sleep(DutyCycleLow/1000) GPIO.output(ServoPin, GPIO.LOW) time.sleep((Period-DutyCycleLow)/1000)
Attachments
Step 4: Build Your Catapult
OK, before you build it you might want to push the little green 'run' arrow on your code just to make sure it's all working.
EZ STICK METHOD:
The easiest way to build the catapult is to place a toothpick on a strip of 3 inch tape (I'm using gaffers, but duct, and wide masking/paint/packing tape should all do about the same). Then stick this on one end perpendicular to your small stick (popsicle stick, metal rod, whatever you used). Next, put a piece of tape also 3 inches on top of that sandwiching everything together as seen in the picture.
Finally, cut a small strip, and put it loosly on the other end of the stick. If your servo didn't come with an arm attached do so now. Then stick the tape around the arm and wrap it up. YOU'RE DONE!
3D PRINTER
You'll need to screw the servo to the base then screw the arm to the servo's arm. Just make sure to mount the arm the right way.
EITHER WAY, CLEAR THE RANGE. THE RANGE IS HOT. ALL CATAPULTS COMMENSE TEST FIRING! Load a toothpick and check it out.
I dare you not to giggle ;-)
Step 5: Prepare the Test Range
You'll want to print off 2 sheets of paper with lines the distance apart of your toothpick. This is required only if you want to calculate the value of pi with your catapult. I've got a word document with a 'table' that you can select row height to adjust your measurements as needed.
Also, I taped together two toothpicks for my tests. The reason for this is that toothpicks can roll, and they'll roll in whatever direction they're already traveling (with some twists possible at landing). We want our tests to be RANDOM. that's why the launcher goes to a random height. Rolling in the direction of fire isn't random, and will greatly ruin your chances of getting a good value of pi.
I placed several notches on the launcher. Use them ALL when firing to make sure your launch is random too. This may be more difficult to do with the EZ launcher. If you want to get creative with your toothpicks/tape feel free. It will definitely help your results to remain random.
Finally, if you do change your munition use something long/slender to find pi. I actually discovered this from somebody recommending hot dogs!
Attachments
Step 6: FIRE THE CATAPULT! (for Science)
Between me and my kids we had a great time playing with this, and it was the first thing they wanted to do after school the next day after showing it to them.
We used our launch range as our score card. For each projectile you fire mark whether it landed on a line or not (some may leave your test range).
Fire 29 shots. If 7 of them land BETWEEN the lines and the other 22 land ON the lines you've done it! Your experiment to solve for pi was MORE accurate than 3.14 is by 25% !!!!! We got this on the first round of 29, but the next round we 24/5, which is way higher than the expected 22/7. Like all statistics repetition will provide random results, but it will eventually trend towards the mean.
The crux of this problem is known as the 'Buffon's Needle'. Basically, since you're using a thin object like a needle it represents the diameter of a circle. Since your diameter can fall anywhere within a 'circle' (between the lines) at any angle the pin is 3.14 times more likely to land on the line than not.
22/7 was the value for pi given by the Egyptians. It is still more accurate than the 3.14 we use today and takes the same number of keystrokes on your calculator. For more fun facts about pi (26 more than in this instructable to be exact) you can watch that over on YouTube. This contest was actually the inspiration for me (and reminder) to make that episode. There's also a dozen very nerdy pi jokes (you've been warned) You can see our catapult in action there too!
Thanks for reading, and let me know if you have any questions!
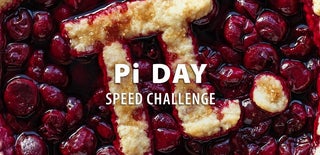
Second Prize in the
Pi Day Speed Challenge