Introduction: Sensor for Railway Layout Automation
Over time, I will add steps in this post, gradually complicating the project. The first three steps explain the choice of sensors, their installation and working example. I use Arduino, but you can use whatever electronic devices.
In this part, an simple example of signaling of a interlocking. The train turns on the red signal when passing the traffic light, and returns the green signal as leaving the block section.
The same principle can be applied to the railway crossing for the movement of the gates and all that.
Step 1: Hall Effect Magnetic Sensor Module
I used Hall sensors as train detector triggers on the line, known Arduino devices and URB block, but you can use breadboard. There are many types of Hall Effect sensors. For applications where the speed of detection is not crucial, ordinary Hall Effect sensors like 44E can be used.
I apply cylinder neodymium magnets because it's convenient. You can fasten them anywhere metal part of the train, both at the beginning and at the end. Also you can put magnets on both the first and the last cars, then the logic of switching signals will become even more interesting.
Step 2: Schematics
This is a very simple example and it works when the locomotive moves in any direction. You can add to it your logic of signaling behavior, for example parity or counter. Also you can install more sensors and so on.
Step 3: Code
// arduinorailwaycontrol.com // SimpleHall.ino
// 19.10.2017 // Author: Steve Massikker //// GPIO PINS //// #define HALL_1 2 #define HALL_2 3 #define RED_SIGNAL 10 #define GREEN_SIGNAL 11 //// VARIABLES //// boolean interlocking_state; void setup() { // Initialize GPIO pinMode(HALL_1, INPUT); pinMode(HALL_2, INPUT); pinMode(RED_SIGNAL, OUTPUT); pinMode(GREEN_SIGNAL, OUTPUT); // On start or reset interlocking_state = true; // Line free digitalWrite(RED_SIGNAL, LOW); digitalWrite(GREEN_SIGNAL, HIGH); } void loop() { if (digitalRead(HALL_1) == LOW || digitalRead(HALL_2) == LOW) { interlocking_state = !interlocking_state; delay(200); } if (interlocking_state) { //true digitalWrite(RED_SIGNAL, LOW); digitalWrite(GREEN_SIGNAL, HIGH); } else { digitalWrite(RED_SIGNAL, HIGH); digitalWrite(GREEN_SIGNAL, LOW); } }
Step 4: Interlocking
Let's complicate our scheme. Now we divide the line into two sections. At the entrance of the each section we'll put the signal and let's go the train in a circle. There is one problem: if the train stops right above the sensor, then our previous sketch will get chaos.
The problem can be solved in many ways, but the most obvious and simple (at the same time get rid of "delay (200);" in the sketch) to put two sensors, instead of one. These modules are very cheap.
Let's change the scheme (see the code of the sketch):
// arduinorailwaycontrol.com // SimpleInterlocking.ino // 16.09.2017 // Author: Steve Massikker //// GPIO PINS //// #define HALL_1 2 #define HALL_2 3 #define HALL_3 4 #define HALL_4 5 #define HALL_5 6 #define HALL_6 7 #define RED_SIGNAL_1 8 #define GREEN_SIGNAL_1 9 #define RED_SIGNAL_2 10 #define GREEN_SIGNAL_2 11 //// VARIABLES //// boolean latch_hall_1 = false, latch_hall_2 = false; boolean latch_hall_3 = false, latch_hall_4 = false; boolean latch_hall_5 = false, latch_hall_6 = false; boolean point_1, point_2, point_3; void setup() { // Initialize GPIO pinMode(HALL_1, INPUT); pinMode(HALL_2, INPUT); pinMode(HALL_3, INPUT); pinMode(HALL_4, INPUT); pinMode(HALL_5, INPUT); pinMode(HALL_6, INPUT); pinMode(RED_SIGNAL_1, OUTPUT); pinMode(GREEN_SIGNAL_1, OUTPUT); pinMode(RED_SIGNAL_2, OUTPUT); pinMode(GREEN_SIGNAL_2, OUTPUT); // On start or reset digitalWrite(RED_SIGNAL_1, LOW); digitalWrite(GREEN_SIGNAL_1, HIGH); digitalWrite(RED_SIGNAL_2, LOW); digitalWrite(GREEN_SIGNAL_2, HIGH); } void loop() { // HALL // if (digitalRead(HALL_1) == LOW) { latch_hall_1 = true; } if (digitalRead(HALL_2) == LOW) { latch_hall_2 = true; } if (digitalRead(HALL_3) == LOW) { latch_hall_3 = true; } if (digitalRead(HALL_4) == LOW) { latch_hall_4 = true; } if (digitalRead(HALL_5) == LOW) { latch_hall_5 = true; } if (digitalRead(HALL_6) == LOW) { latch_hall_6 = true; } // POINT // if (latch_hall_1 && latch_hall_2) { latch_hall_1 = false; latch_hall_2 = false; point_1 = false; } if (latch_hall_2 && latch_hall_3) { latch_hall_3 = false; latch_hall_4 = false; point_2 = false; } if (latch_hall_4 && latch_hall_5) { latch_hall_4 = false; latch_hall_5 = false; point_3 = false; } // LOGIC // if (!point_1) { digitalWrite(RED_SIGNAL_1, HIGH); // red digitalWrite(GREEN_SIGNAL_1, LOW); } if (!point_2) { digitalWrite(RED_SIGNAL_1, LOW); // green digitalWrite(GREEN_SIGNAL_1, HIGH); digitalWrite(RED_SIGNAL_2, HIGH); // red digitalWrite(GREEN_SIGNAL_2, LOW); point_1 = true; } if (!point_3) { digitalWrite(RED_SIGNAL_2, LOW); // green digitalWrite(GREEN_SIGNAL_2, HIGH); point_2 = true; point_3 = true; } }
Coming soon next step "Stop train at signal"!
And the gift video - how to make a two-wire signal.
Step 5: Bidirectional Line
Before I bring the sketch from my real layout, in this post I will give a general solution. It is quite workable and you can apply it in your designs.
In this example, I use a variable of byte type to write the state of the rail blocks. As a result, we are able to transmit this number via bus I2C or serial port. It also makes it possible to perform mathematical operations with data (see the end of the sketch). The principle of recording data in the pictures.
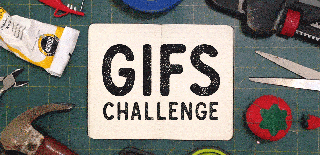
Participated in the
GIFs Challenge 2017