Introduction: Using Arduino in Industry (on Paper Guillotine Machine)
Hello.
On this instruction i'll give some tricks how to use arduino (or other MK) in industry. What problems will appear and how I fix it.
I have order to fix broken PC on Wohlenberg cutting machine(on video you can see one of the first edition of my arduino). New original PC for this machine costs 3000+$.
What do my arduino do
1. Display size using internal high speed encoder (25imp/mm) and tft screen
2. Create 40 programs with 40 position for cutting
3. Fully control motors (in auto mode enable)
4. Next step position after cutting
5. Autocallibration in 15 steps (for machine in video, and operator controlled calibration call)
6. Fully configurable system for any cutting machine
7. Support 3 buttons and matrix keyboard (in new revision I added USB keyboard support)
8. Power filter
9. Debounce filters
10. And many other function
In the next steps i'll describe some problems and how i fix it.
Step 1: Casing. How to Create Custom Case for Any Project
When you build your project in the same step you'll need casing for mounting.
I create drawing in CAD software. 3D model with all holes. I use usually 3-4mm acryl. 3d model export to dxf drowing than to laser cutting. Cutted model i fold to finished case like in photo
Step 2: Power Supply
Ihere is a realy big problem with power supply in industrial machines.
What I have
1. 36 V, 24 V (usually),15 V (yes i saw this), 12 V, 9 V, 5 V.
2. All voltages have a bounce. If you'll needed i can attach data from oscilograph
3. Internal power supply of machine dislike when you connect something strange
4. when you add external power supply 110-220V to 5v to arduino - your arduino will work terrible. in some cases voltage will fall to 4V!!!
How i fix it
1. I install my own industrial pulse power supply 380-220-100V ACto 24VDC or to 12VDC (30$+)
2. To my power supply i add 3A DC-DC converter and configure it to 5VDC out.
3. DC-DC i connect directly to ARDUINO. It means, i connect DC-DC out-negative to arduino ground, DC-DC out-positive to arduino +5V to the pins directly on board. In this case i'll have stabilized 5V for all my components.
Step 3: Incoming Signals Filtering
Also is another big problem that incoming signals in machines can be 36-24-12VDC on logical 1 with bounce. Using resistors or optocouples can help only partial. When signal changes, its state thousand times per second and it become a real problem. Thats why i constructed a filter.
I connect a signal wire to input channel, Machine ground and Machine +24(36,12,etc.).
To output channel i connect arduino.
In this case MK ground and Machine ground will be separated.
Note! - inverted logic
Step 4: Keyboard
After 3 month of working matrix keyboard became broken. I added support of usb keyboad to arduino.
Connect USB port:
1. USB positive-to arduino +5
2. USB negative - arduino ground
3. USB data+ - Arduino port 21 (or any port with interrupt)
4. USB data- - Arduino port 20 (or any port with interrupt)
Connect Keyboard to new USB port
Now download PS2Keyboard library from github or my fixed library from included files
Add to your sketch
#include PS2Keyboard.h (download this library)
void setup()
{
...
keyboard.begin(DataPin, IRQpin, PS2Keymap_US);
...
}
call this code inside encoder IRQ function
void encoder_irq()
{
....
char USB_key()
{key_filterred=NO_KEY; if (keyboard.available()) { int c = keyboard.read(); switch (c) { case 48: return '0'; break; case 46: return '#'; break; // . cancel case 13: return '*'; break;//enter case 49: return '1'; break; case 50: return '2'; break; case 51: return '3'; break; case 52: return '4'; break; case 53: return '5'; break; case 54: return '6'; break; case 55: return '7'; break; case 56: return '8'; break; case 57: return '9'; break; case 43: return '+'; break;//+ case 45: return '-'; break;//- case 127: return 'R'; break;//back space case 42: return 'e'; break;//** case 47: return 'd'; break;// / //- case 118: return 'B'; break;//Button1 cancel case 117: return 'A'; break; case 116: return 'D'; break; case 115: return 'C'; break; case 119: return 'n'; break;//num }
Step 5: Encoder
Power encoder from arduino (usually it will be 5V encoder).
Info lines from encoder connect to filter.
Call encoder using interupt!!! when youll use timer you'll miss steps.
Connecting encoder directly to arduino - you'll miss or get extrastep when motors working. Long wires and electrical noise... I spend many nights and test ideas but... only this is a good solution. Encoder-filter-microcontroller
If someone will ask about encoder code or its connection - i'll add this part
upd 30.08.2016 added encoder source
void setup ()
{
detachInterrupt(0);
pinMode(encoder0PinA, INPUT);
digitalWrite(encoder0PinA, HIGH); // turn on pullup resistor
pinMode(encoder0PinB, INPUT);
digitalWrite(encoder0PinB, HIGH); // turn on pullup resistor
attachInterrupt(0, doEncoder, RISING); // encoder pin on interrupt 0 - pin 2
}
void doEncoder()
{
unsigned long now = micros();
unsigned long interval = now - lastPulseTime;
if (interval > 5)
{
if (digitalRead(encoder0PinB) == HIGH) { // found a low-to-high on channel A
if (digitalRead(encoder0PinA) == LOW) { // check channel B to see which way // encoder is turning
encoder0Pos = encoder0Pos + 1; // CCW
} else {
encoder0Pos = encoder0Pos - 1; // CW
}
}
else // found a high-to-low on channel A
{
if (digitalRead(encoder0PinA) == LOW) { // check channel B to see which way // encoder is turning encoder0Pos = encoder0Pos - 1; // CW
} else {
encoder0Pos = encoder0Pos + 1; // CCW
}
}
}
Step 6: Finally
Out signals are the simplest task. You can use relay module....
Finally using this tricks i build more than 10 machines for different tasks
Thanks for reading
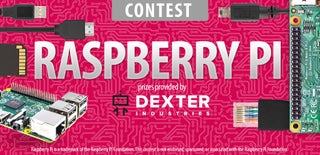
Participated in the
Raspberry Pi Contest 2016