Introduction: Wifi Recording Sign Controlled by Your DAW
Recording your own music at home — especially if you don’t live alone — can be a nightmare at times. The last thing you want to have to deal with is screaming children in the background of your best take EVER! 😒🙄😩 But what if we could avoid all this agony in advance?
Let’s make a recording sign that lights up when you’re recording in your DAW (digital audio workstation) to let everyone know that they need to be quiet — for less than $20! And we’ll make it work over your local wifi network so you can put it anywhere in your house without being physically connected to your computer. It will also automatically connect to your DAW and you’ll never have to worry about the recording sign again!
How will it work?
It’s actually simpler than you might think. Have you ever heard of rtpMIDI or network midi? It basically lets you send and receive midi messages to and from your DAW over the internet or your local wifi network. We’ll use this to send a midi message to an ESP32 when the recording starts and stops in your DAW. An ESP32 is a tiny programmable microcontroller with wifi and Bluetooth capabilities. We’ll program it to receive these midi messages and light up an LED strip red.
Supplies
Note: this project can handle multiple recording signs at the same time, so you can buy more if you want to.
An ESP32 — $10
We’ll use this gizmo to receive midi signals from your DAW over your local wifi network and light up an LED strip. There are two things to note when buying this:
- You’ll probably be better off buying one without the pin headers already soldered onto the board. We only need to use three pins, so the rest is just a waste of space.
- You’ll want to make sure that it has a 5V pin or a Vin pin that can be used as a 5V pin. Check the pinout of your board before buying it, although in most cases the Vin pin should work fine.
A micro USB 2.0 cable
We need this cable to power and program the ESP32. You’ve probably got lots of these already. It’s the same as any old phone charger cable.
A NeoPixel strip — $10
This is similar to an ordinary RGB-LED strip that you might already have in your house - but better. It lets you control the color of each individual LED so you can create fun animations. But the real reason why we’ll use this is so that we don’t have to worry about powering it with a 12V power supply. It doesn't matter how long it is, as we’ll only use about 10 LEDs. If you wanted to use more than that, you would need an additional 5V power supply because the LEDs would draw too much power and you might damage your ESP32.
Some jumper cables or wires (min. 3)
We’ll use these to connect the NeoPixel strip to the ESP32. You’ll probably have some sort of wires lying around your house and most wires will do. Though you can buy a pack of jumper cables if you like.
A soldering iron or a friend with one
There’s no way around it, I’m afraid! You’ll need a soldering iron to solder the jumper cables to the NeoPixel strip and the ESP32. But I’m sure a friend has one and won’t mind soldering a few jumper cables for you !
Some kind of case
It‘s important to keep the ESP32 in a case, as dust and other stuff can damage the board. You can use anything really, e.g. a matchbox. For harder cases, it may be a good idea to add a little opening so the wifi antenna on the board has more signal. If you own a 3D printer, you can download my printable case from Thingiverse. Otherwise, you can get a bit crafty!
Step 1: Connecting the NeoPixel Strip to the ESP32 (15 Min)
This is the hardest part of the whole project so let’s get it out of the way first!
Solder the jumper cables to connect the NeoPixel to ESP32 with the following connections:
NeoPixel pin → ESP32 pin
+5V → 5V or Vin (depending on your board)
Din → 13
GND → GND
Step 2: Programming the ESP32 (10 Min)
We need to program the ESP32 so it knows what to do. For this we’ll use the Arduino IDE:
1. Download and install the Arduino IDE with the latest installer. (Your IDE will have different colors than mine, but that's ok.)
Because the Arduino IDE doesn’t support ESP32s out of the box, we need to install some additional software in the IDE:
2. Open the IDE, navigate to Files → Preferences, paste https://dl.espressif.com/dl/package_esp32_index.js... into Additional Boards Manager URLs and click OK.
3. Go to Tools → Board → Boards Manager, search for ESP32(by Espressif Systems), and click Install.
4. Select your ESP32 board in Tools → Board → ESP32 Arduino. (If your board isn’t listed, ESP32 Dev Module should be fine.)
Next, we’ll install a library that helps us control the NeoPixel strip (Adafruit NeoPixel) and a library that does all the rtpMidi stuff for us (AppleMidi):
5. Go to Tools → Manage Libraries… and search for and install Adafruit NeoPixel (not Adafruit DMA neopixel library!!) and AppleMidi. (If it asks you to install other libraries, click Install all.)
Now we’ll download and edit the code for the ESP32 so that it can log onto your wifi network:
6. Download and extract my Recording-Sign repository(go to Code → Download ZIP) and open Recording-Sign.ino with the Arduino IDE.
7. Replace the value of NUM_LEDS (in the code) with the number of LEDs on your NeoPixel strip, ssid with the name of your wifi network, and password with the password for your network.
(If you wired up the NeoPixel strips exactly like in the image under 1. Connecting the NeoPixel strip to the ESP32, you’ll need to edit some more code to look like this:
<pre>#define NUM_LEDS 7 // change the number of LEDs // ... void colorWipe(int red, int green, int blue) { for (int i = 0; i<NUM_LEDS/2+1; i++) { // edit this line strip.setPixelColor(i, red, green, blue); strip.setPixelColor(NUM_LEDS-1-i, red, green, blue); // add this strip.show(); delay(70); } }
)
Finally, we’ll compile and upload the code to the ESP32:
8. Plug your ESP32 into your computer, go to Tools → Port, and select the USB port your ESP32 is plugged in.
9. Open the serial monitor by clicking 🔎 and set the baud rate to 115200.
10. Compile and upload the sketch to your ESP32 by clicking ➡️ in the IDE. (Note: on some boards, you may need to press the boot button on the ESP32 while it uploads.)
11. Remember/write down the IP address from the serial monitor (we’ll need it later).
Step 3: Setting Up the RtpMIDI Session (5 Min)
The rtpMIDI is the part that makes this whole project possible in the first place. It allows us to send and receive midi messages over a wifi network. This is preinstalled on Mac OS but not on Windows, so we’ll have to install it:
1.
Windows: Install rtpMIDI with the latest installer and launch it. (If the Apple Bonjour isn’t already installed, you’ll need to install that as well. The rtpMIDI installer will suggest visiting the Apple-download-page for Bonjour if it isn’t installed.)
Mac OS: Open the Audio MIDI Setup utility, go to Window → Show MIDI Studio and click 🌐 to open the MIDI Network Setup.
Now we’ll create a new midi session that the DAW can see:
2. Under My Sessions, press + to add a new session, and select it.
3. Under Session, rename the session to Recording Sign, set the port to 5004, and select Enabled.
Next, we’ll connect the ESP32 to the session:
4. Under Directory, press + to add a remote peer, then enter a name, the IP address of the ESP32 and the port 5004, and click OK.
5. Under Directory, select the remote peer you just added and click Connect.
Step 4: Mapping the RtpMIDI Session to the Record Button in Your DAW (2 Min)
Finally, we want the DAW to send a midi message to the rtpMIDI session when the recording starts and stops. Because the mapping procedure differs from DAW to DAW, please visit the documentation of your DAW for instructions, though there are a few important things you need to note:
- Set the control to transmit. We want the DAW to transmit the updates to the ESP32 and not just listen for midi messages from it.
- Set the control to toggle. This way the DAW will send the on/max value when recording starts and the off/min value when recording stops.
- The control number must match the CONTROL_NUMBER value in Recording-Sign.ino (default: 1 — you can change this in the code if you need).
- The on/max value must match the ON_VALUE value in Recording-Sign.ino (default: 127 — you can change this in the code if you need).
- The ESP32 sends a midi message when it connects to the rtpMIDI session. So if your DAW requires a midi message to map, either disconnect and reconnect the ESP32 to the rtpMIDI session or restart the ESP32.
The image shows what it should look like in Cubase.
Step 5: We’re Done!
You did it! You can now plug in the recording sign anywhere in your house where there is a wifi signal using a 5V phone charger. Windows users will never have to think about anything ever again! It will just work all on its own! But Mac OS users will still have to remember to enable the Network MIDI session before recording, I’m afraid. But that’s not really a big deal, is it?
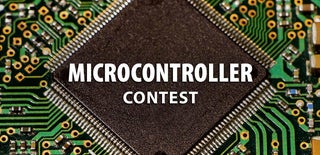
Participated in the
Microcontroller Contest