Introduction: BWOOM Mask Reimagined + Arduino
BWOOM Mask is from the Democratic Republic of the Congo in Central Africa. Unlike most art, it was designed to be used in African masquerades. The mask was used in dance and other rituals and shook back and forth on a person's face making its mobile appearance differ from its stationary one. Using persistence of vision optical effects, I've made some interesting visual illusions with this mask and other objects/figures. I use a motor setup, LED lighting and an arduino microcontroller to create these images.
If curious:
Step 1: Imagine and Design
I calculated the motor speed necessary along with the frequency of light needed to make my rotating motor appear stationary. In essence, the light must flash at the same rate that the motor spins. If you would like, review the calculations and please notify me of any error. I will rectify it.
Materials Used:
- Male to Female Jumper Wires (non specific)
- Male to Male Jumper Wires
- Arduino Nano (non specific)
- 3V White LEDs
- Breadboard (non specific)
- Motor
- ESC(Electronic Speed Controller)
- 11.1V 25C 2200mAh battery (non-specific)
Equipment:
- 3D Printer and filament (non specific)
Step 2: Code
It is necessary to download the servo library for Arduino. The servo library controls the esc thus changing motor speed. Read the comments in the code for further detail. If you'd like to download Arduino click here: https://www.arduino.cc/en/Main/Software
/*By Patrick B., (Aeropib, Pib2)<br> *Sources/Contributors: https://gist.github.com/col/8170414 *Thank you col! */ #include <Servo.h><servo.h> Servo esc; int escPin = 12; int minPulseRate = 1000; int maxPulseRate = 2000; int throttleChangeDelay = 100; int LED_BUILTIN_2 = 2; //Declare red/green/white light int LED_BUILTIN_3 = 3; int LED_BUILTIN_4 = 4; int LED_BUILTIN_5 = 5; int LED_BUILTIN_6 = 6; int LED_BUILTIN_7 = 7; int LED_BUILTIN_8 = 8; int LED_BUILTIN_9 = 9; int LED_BUILTIN_10 = 10; int LED_BUILTIN_11 = 11; unsigned long previousMillis = 0; int x =25;//x is motor speed variable int loop_num=0; void setup() { Serial.begin(9600); Serial.setTimeout(500); // Attach the the servo to the correct pin and set the pulse range esc.attach(escPin, minPulseRate, maxPulseRate); // Write a minimum value (most ESCs require this correct startup) esc.write(0); pinMode(LED_BUILTIN_2, OUTPUT); pinMode(LED_BUILTIN_3, OUTPUT); pinMode(LED_BUILTIN_4, OUTPUT); pinMode(LED_BUILTIN_5, OUTPUT); pinMode(LED_BUILTIN_6, OUTPUT); pinMode(LED_BUILTIN_7, OUTPUT); pinMode(LED_BUILTIN_8, OUTPUT); pinMode(LED_BUILTIN_9, OUTPUT); pinMode(LED_BUILTIN_10, OUTPUT); pinMode(LED_BUILTIN_11, OUTPUT); } //likes 27,44 on 11.5 v batt, turnigy motor/esc, under load 25,45, just use 25,0(safety) //use 25,29 void loop() { unsigned long currentMillis = millis(); digitalWrite(LED_BUILTIN_2, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_3, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_4, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_5, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_6, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_7, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_8, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_9, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_10, HIGH); //500, 14000 is final digitalWrite(LED_BUILTIN_11, HIGH); //500, 14000 is final // delay(1); //8.97,14.5,1.5 works well, prop alone delayMicroseconds(250); //500 digitalWrite(LED_BUILTIN_2, LOW); digitalWrite(LED_BUILTIN_3, LOW); digitalWrite(LED_BUILTIN_4, LOW); digitalWrite(LED_BUILTIN_5, LOW); digitalWrite(LED_BUILTIN_6, LOW); digitalWrite(LED_BUILTIN_7, LOW); digitalWrite(LED_BUILTIN_8, LOW); digitalWrite(LED_BUILTIN_9, LOW); digitalWrite(LED_BUILTIN_10, LOW); digitalWrite(LED_BUILTIN_11, LOW); // delay(35);//(12.7,40 all w/1.5) delayMicroseconds(14000); //2800000 work, also 14000,7000,28000 loop_num+=1; //loop number counter if ( (loop_num % 2) == 0) //if even loop number make x=25 (slow down motor) { x = 25; } else { x=29; //if odd loop number make x=29 (speed up motor) } // Wait for some input if (currentMillis-previousMillis>5000) { previousMillis=currentMillis;//5 second timer } // // if (Serial.available() > 0) {</servo.h></p><p> if (currentMillis-previousMillis > 4900) { //100millis to reset int currentThrottle = readThrottle(); // if (currentThrottle != 25 ) // { // Read the new throttle value // int throttle = normalizeThrottle( Serial.parseInt() ); int throttle = normalizeThrottle( x ); // int throttle = 25; // Print it out Serial.print("Setting throttle to: "); Serial.println(throttle); // Change throttle to the new value changeThrottle(throttle); } } void changeThrottle(int throttle) { // Read the current throttle value int currentThrottle = readThrottle(); // Are we going up or down? int step = 1; if( throttle < currentThrottle ) step = -1; // Slowly move to the new throttle value while( currentThrottle != throttle ) { esc.write(currentThrottle + step); currentThrottle = readThrottle(); delay(throttleChangeDelay); } } int readThrottle() { int throttle = esc.read(); Serial.print("Current throttle is: "); Serial.println(throttle); return throttle; } // Ensure the throttle value is between 0 - 180 int normalizeThrottle(int value) { if( value < 0 ) return 0; if( value > 180 ) return 180; return value; }
Step 3: 3D Print
This is a time lapse where each photo is taken at the 1 hour mark. It took approximately 6 hours to print. The STL file is attached if you'd like to print one as well.
Attachments
Step 4: Integrate Electronics
Due to 3D printer tolerance issues, the diameter of the holes for the LED may need to be enlarged slightly. I used a drill bit to do this. Then, insert the LEDs and connect the positive lead of each LED to digital pins 2-11 of the Arduino and the negative leads to ground of the Arduino. I powered the Arduino through the VIN pin with the 5V output from the ESC and connected the signal pin (white) to pin 12 of the Arduino. I connected ground of the ESC to ground of the Arduino. Make sure there is a universal ground by linking all ground pins together.
Make sure to solder the 3 leads on the other side of the esc to the 3 leads on the motor. Then connect the battery to the 2 leads coming from the esc. See the diagram for a visual explanation.
I did not use any resistors because the light was on for so little time. In the span of 1 minute, the light is only on for 1.05 seconds given the 1.75% duty cycle. Therefore, a relatively low amount of current was used (25.6 microAmps). The Voltage was also relatively low at (0.161 Volts). I used AC current and AC voltage because the light is oscillating on and off.
Step 5: Cover Electronics and Finalize
I used metallic silver duct tape to cover the wires. I wrapped paper around the structure, then wrapped the duct tape around the paper so that the paper-duct tape material can slide off at anytime an LED needs to be replaced or a wire needs to be reinserted. If you've gotten this far, you may also need to clean your workspace if you're anything like me.
Step 6: Art You Say?
Perhaps the process of creating this project is more of an art form than the piece itself. This concept was not modeled off anything else specifically, rather its a reinterpretation of the BWOOM mask of 19th century Kuba, Central Africa.
Many additional applications can be used for this such as animation, inspection of rotating objects and so on...
Thank you Instructables for allowing the world-wide sharing of ideas!
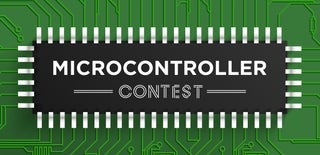
Participated in the
Microcontroller Contest