Introduction: Domotica Con Arduino + IPhone (ipharduino)
Utilizzando Processing come Ide master comunicazione IP tra iPhone - Router - Processing, tramite questo piccolo
sketch ho sintetizzato:
-Server
-Controller Arduino
-Controller Usb
Di seguito incollo il codice Processing.
Vi ricordo ch e per poter funzionare occorre scaricare le 3 librerie fondamentali
OscP5, NetP5, CC.Arduino,
----------------------------------------------------------
import oscP5.*; // Load OSC P5 library
import netP5.*; // Load net P5 library
import processing.serial.*; // Load serial library
import cc.arduino.*;
Serial arduinoPort; // Set arduinoPort as serial connection
OscP5 oscP5; // Set oscP5 as OSC connection
int redLED = 0; // redLED lets us know if the LED is on or off
int [] led = new int [2]; // Array allows us to add more toggle buttons in TouchOSC
void setup() {
size(100,100); // Processing screen size
noStroke(); // We don’t want an outline or Stroke on our graphics
oscP5 = new OscP5(this,8000); // Start oscP5, listening for incoming messages at port 8000
arduinoPort = new Serial(this, Serial.list()[2], 9600); // Set arduino to 9600 baud
}
void oscEvent(OscMessage theOscMessage) { // This runs whenever there is a new OSC message
String addr = theOscMessage.addrPattern(); // Creates a string out of the OSC message
if(addr.indexOf("/1/toggle") !=-1){ // Filters out any toggle buttons
int i = int((addr.charAt(9) )) - 0x30; // returns the ASCII number so convert into a real number by subtracting 0x30
led[i] = int(theOscMessage.get(0).floatValue()); // Puts button value into led[i]
// Button values can be read by using led[0], led[1], led[2], etc.
}
}
void draw() {
background(50); // Sets the background to a dark grey, can be 0-255
if(led[1] == 0){ // If led button 1 if off do....
arduinoPort.write("a"); // Sends the character “r” to Arduino
redLED = 0; // Sets redLED color to 0, can be 0-255
}
if(led[1] == 1){ // If led button 1 is ON do...
arduinoPort.write("A"); // Send the character “R” to Arduino
redLED = 255; // Sets redLED color to 255, can be 0-255
}
fill(redLED,0,0); // Fill rectangle with redLED amount
ellipse(50, 50, 50, 50); // Created an ellipse at 50 pixels from the left...
// 50 pixels from the top and a width of 50 and height of 50 pixels
}
----------------------------------------------------------
Guardando il codice noterete che in base all'output captato in rete dal touchosc
0 o 1, Processing scriverà nella seriale di arduino 2 valori:
a,A.
Quindi in arduino bisogna installare uno script che capti questi 2 valori
e li trasformi in funzioni, ad es. LedOn/LedOff .. ecc...
di seguito vi incollo il codice da installare dentro arduino:
----------------------------------------------------------
#define LED_PIN1 4
#define LED_PIN2 5
#define LED_PIN3 6
#define LED_PIN4 7
#define LED_PIN5 8
#define LED_PIN6 9
#define LED_PIN7 10
#define LED_PIN8 11
int firstSensor = 0; // first analog sensor
int secondSensor = 0; // second analog sensor
int thirdSensor = 0; // digital sensor
int inByte = 0; // incoming serial byte
boolean status_unlock;
boolean status_bluetooth;
long interval = 1000; // interval at which to blink (milliseconds)
long previousMillis = 0; // will store last time LED was update
int minite,sec;
void setup()
{
// start serial port at 9600 bps:
Serial.begin(9600);
//pinMode(2, INPUT); // digital sensor is on digital pin 2
//establishContact(); // send a byte to establish contact until receiver responds
pinMode(LED_PIN1, OUTPUT);
pinMode(LED_PIN2, OUTPUT);
pinMode(LED_PIN3, OUTPUT);
pinMode(LED_PIN4, OUTPUT);
pinMode(LED_PIN5, OUTPUT);
pinMode(LED_PIN6, OUTPUT);
pinMode(LED_PIN7, OUTPUT);
pinMode(LED_PIN8, OUTPUT);
digitalWrite(LED_PIN1, LOW); // switch off LED
digitalWrite(LED_PIN2, LOW); // switch off LED
digitalWrite(LED_PIN3, LOW); // switch off LED
digitalWrite(LED_PIN4, LOW); // switch off LED
digitalWrite(LED_PIN5, LOW); // switch off LED
digitalWrite(LED_PIN6, LOW); // switch off LED
digitalWrite(LED_PIN7, LOW); // switch off LED
digitalWrite(LED_PIN8, LOW); // switch off LED
status_bluetooth = true;
status_unlock = false;
sec = 0;
}
void loop()
{
if (Serial.available() > 0) {
inByte = Serial.read(); // get incoming byte:
if(inByte == 'A'){
digitalWrite(LED_PIN1, HIGH); // switch on LED
Serial.print('A', BYTE); // send a char
delay(800);
digitalWrite(LED_PIN1, LOW); // switch off LED
status_unlock = false;
inByte = 0;
}
if(inByte == 'a'){
digitalWrite(LED_PIN2, HIGH); // switch on LED
Serial.print('a', BYTE); // send a char
delay(800);
digitalWrite(LED_PIN2, LOW); // switch off LED
status_unlock = true;
sec = 0;
inByte = 0;
}
if(inByte == 'B'){
digitalWrite(LED_PIN3, HIGH); // switch on LED
Serial.print('B', BYTE); // send a char
inByte = 0;
}
if(inByte == 'b'){
digitalWrite(LED_PIN3, LOW); // switch off LED
Serial.print('b', BYTE); // send a char
inByte = 0;
}
if(inByte == 'C'){
digitalWrite(LED_PIN4, HIGH); // switch on LED
Serial.print('C', BYTE); // send a char
inByte = 0;
}
if(inByte == 'c'){
digitalWrite(LED_PIN4, LOW); // switch off LED
Serial.print('c', BYTE); // send a char
inByte = 0;
}
if(inByte == 'D'){
digitalWrite(LED_PIN5, HIGH); // switch on LED
Serial.print('D', BYTE); // send a char
inByte = 0;
}
if(inByte == 'd'){
digitalWrite(LED_PIN5, LOW); // switch off LED
Serial.print('d', BYTE); // send a char
inByte = 0;
}
if(inByte == 'E'){
digitalWrite(LED_PIN6, HIGH); // switch on LED
Serial.print('E', BYTE); // send a char
inByte = 0;
}
if(inByte == 'e'){
digitalWrite(LED_PIN6, LOW); // switch off LED
Serial.print('e', BYTE); // send a char
inByte = 0;
}
if(inByte == 'F'){
digitalWrite(LED_PIN7, HIGH); // switch on LED
Serial.print('F', BYTE); // send a char
inByte = 0;
}
if(inByte == 'f'){
digitalWrite(LED_PIN7, LOW); // switch off LED
Serial.print('f', BYTE); // send a char
inByte = 0;
}
if(inByte == 'G'){
digitalWrite(LED_PIN8, HIGH); // switch on LED
Serial.print('G', BYTE); // send a char
inByte = 0;
}
if(inByte == 'g'){
digitalWrite(LED_PIN8, LOW); // switch off LED
Serial.print('g', BYTE); // send a char
inByte = 0;
}
if(inByte == 'S'){
Serial.print('S', BYTE); // send a char
status_bluetooth = true;
sec = 0;
}
} // if(Serial
/*
unsigned long currentMillis = millis();
if(currentMillis - previousMillis > interval) {
previousMillis = currentMillis; // save the last time you blinked the LED
sec++;
if(status_unlock==true){
if(sec== 11){
digitalWrite(LED_PIN1, HIGH); // switch on LED
delay(800);
digitalWrite(LED_PIN1, LOW); // switch off LED
status_unlock = false;
sec = 0;
}
}
else sec = 0;
}
*/
} //Loop
void establishContact() {
while (Serial.available() <= 0) {
Serial.print('.', BYTE); // send a capital A
delay(500);
}
}
----------------------------------------------------------
sketch ho sintetizzato:
-Server
-Controller Arduino
-Controller Usb
Di seguito incollo il codice Processing.
Vi ricordo ch e per poter funzionare occorre scaricare le 3 librerie fondamentali
OscP5, NetP5, CC.Arduino,
----------------------------------------------------------
import oscP5.*; // Load OSC P5 library
import netP5.*; // Load net P5 library
import processing.serial.*; // Load serial library
import cc.arduino.*;
Serial arduinoPort; // Set arduinoPort as serial connection
OscP5 oscP5; // Set oscP5 as OSC connection
int redLED = 0; // redLED lets us know if the LED is on or off
int [] led = new int [2]; // Array allows us to add more toggle buttons in TouchOSC
void setup() {
size(100,100); // Processing screen size
noStroke(); // We don’t want an outline or Stroke on our graphics
oscP5 = new OscP5(this,8000); // Start oscP5, listening for incoming messages at port 8000
arduinoPort = new Serial(this, Serial.list()[2], 9600); // Set arduino to 9600 baud
}
void oscEvent(OscMessage theOscMessage) { // This runs whenever there is a new OSC message
String addr = theOscMessage.addrPattern(); // Creates a string out of the OSC message
if(addr.indexOf("/1/toggle") !=-1){ // Filters out any toggle buttons
int i = int((addr.charAt(9) )) - 0x30; // returns the ASCII number so convert into a real number by subtracting 0x30
led[i] = int(theOscMessage.get(0).floatValue()); // Puts button value into led[i]
// Button values can be read by using led[0], led[1], led[2], etc.
}
}
void draw() {
background(50); // Sets the background to a dark grey, can be 0-255
if(led[1] == 0){ // If led button 1 if off do....
arduinoPort.write("a"); // Sends the character “r” to Arduino
redLED = 0; // Sets redLED color to 0, can be 0-255
}
if(led[1] == 1){ // If led button 1 is ON do...
arduinoPort.write("A"); // Send the character “R” to Arduino
redLED = 255; // Sets redLED color to 255, can be 0-255
}
fill(redLED,0,0); // Fill rectangle with redLED amount
ellipse(50, 50, 50, 50); // Created an ellipse at 50 pixels from the left...
// 50 pixels from the top and a width of 50 and height of 50 pixels
}
----------------------------------------------------------
Guardando il codice noterete che in base all'output captato in rete dal touchosc
0 o 1, Processing scriverà nella seriale di arduino 2 valori:
a,A.
Quindi in arduino bisogna installare uno script che capti questi 2 valori
e li trasformi in funzioni, ad es. LedOn/LedOff .. ecc...
di seguito vi incollo il codice da installare dentro arduino:
----------------------------------------------------------
#define LED_PIN1 4
#define LED_PIN2 5
#define LED_PIN3 6
#define LED_PIN4 7
#define LED_PIN5 8
#define LED_PIN6 9
#define LED_PIN7 10
#define LED_PIN8 11
int firstSensor = 0; // first analog sensor
int secondSensor = 0; // second analog sensor
int thirdSensor = 0; // digital sensor
int inByte = 0; // incoming serial byte
boolean status_unlock;
boolean status_bluetooth;
long interval = 1000; // interval at which to blink (milliseconds)
long previousMillis = 0; // will store last time LED was update
int minite,sec;
void setup()
{
// start serial port at 9600 bps:
Serial.begin(9600);
//pinMode(2, INPUT); // digital sensor is on digital pin 2
//establishContact(); // send a byte to establish contact until receiver responds
pinMode(LED_PIN1, OUTPUT);
pinMode(LED_PIN2, OUTPUT);
pinMode(LED_PIN3, OUTPUT);
pinMode(LED_PIN4, OUTPUT);
pinMode(LED_PIN5, OUTPUT);
pinMode(LED_PIN6, OUTPUT);
pinMode(LED_PIN7, OUTPUT);
pinMode(LED_PIN8, OUTPUT);
digitalWrite(LED_PIN1, LOW); // switch off LED
digitalWrite(LED_PIN2, LOW); // switch off LED
digitalWrite(LED_PIN3, LOW); // switch off LED
digitalWrite(LED_PIN4, LOW); // switch off LED
digitalWrite(LED_PIN5, LOW); // switch off LED
digitalWrite(LED_PIN6, LOW); // switch off LED
digitalWrite(LED_PIN7, LOW); // switch off LED
digitalWrite(LED_PIN8, LOW); // switch off LED
status_bluetooth = true;
status_unlock = false;
sec = 0;
}
void loop()
{
if (Serial.available() > 0) {
inByte = Serial.read(); // get incoming byte:
if(inByte == 'A'){
digitalWrite(LED_PIN1, HIGH); // switch on LED
Serial.print('A', BYTE); // send a char
delay(800);
digitalWrite(LED_PIN1, LOW); // switch off LED
status_unlock = false;
inByte = 0;
}
if(inByte == 'a'){
digitalWrite(LED_PIN2, HIGH); // switch on LED
Serial.print('a', BYTE); // send a char
delay(800);
digitalWrite(LED_PIN2, LOW); // switch off LED
status_unlock = true;
sec = 0;
inByte = 0;
}
if(inByte == 'B'){
digitalWrite(LED_PIN3, HIGH); // switch on LED
Serial.print('B', BYTE); // send a char
inByte = 0;
}
if(inByte == 'b'){
digitalWrite(LED_PIN3, LOW); // switch off LED
Serial.print('b', BYTE); // send a char
inByte = 0;
}
if(inByte == 'C'){
digitalWrite(LED_PIN4, HIGH); // switch on LED
Serial.print('C', BYTE); // send a char
inByte = 0;
}
if(inByte == 'c'){
digitalWrite(LED_PIN4, LOW); // switch off LED
Serial.print('c', BYTE); // send a char
inByte = 0;
}
if(inByte == 'D'){
digitalWrite(LED_PIN5, HIGH); // switch on LED
Serial.print('D', BYTE); // send a char
inByte = 0;
}
if(inByte == 'd'){
digitalWrite(LED_PIN5, LOW); // switch off LED
Serial.print('d', BYTE); // send a char
inByte = 0;
}
if(inByte == 'E'){
digitalWrite(LED_PIN6, HIGH); // switch on LED
Serial.print('E', BYTE); // send a char
inByte = 0;
}
if(inByte == 'e'){
digitalWrite(LED_PIN6, LOW); // switch off LED
Serial.print('e', BYTE); // send a char
inByte = 0;
}
if(inByte == 'F'){
digitalWrite(LED_PIN7, HIGH); // switch on LED
Serial.print('F', BYTE); // send a char
inByte = 0;
}
if(inByte == 'f'){
digitalWrite(LED_PIN7, LOW); // switch off LED
Serial.print('f', BYTE); // send a char
inByte = 0;
}
if(inByte == 'G'){
digitalWrite(LED_PIN8, HIGH); // switch on LED
Serial.print('G', BYTE); // send a char
inByte = 0;
}
if(inByte == 'g'){
digitalWrite(LED_PIN8, LOW); // switch off LED
Serial.print('g', BYTE); // send a char
inByte = 0;
}
if(inByte == 'S'){
Serial.print('S', BYTE); // send a char
status_bluetooth = true;
sec = 0;
}
} // if(Serial
/*
unsigned long currentMillis = millis();
if(currentMillis - previousMillis > interval) {
previousMillis = currentMillis; // save the last time you blinked the LED
sec++;
if(status_unlock==true){
if(sec== 11){
digitalWrite(LED_PIN1, HIGH); // switch on LED
delay(800);
digitalWrite(LED_PIN1, LOW); // switch off LED
status_unlock = false;
sec = 0;
}
}
else sec = 0;
}
*/
} //Loop
void establishContact() {
while (Serial.available() <= 0) {
Serial.print('.', BYTE); // send a capital A
delay(500);
}
}
----------------------------------------------------------
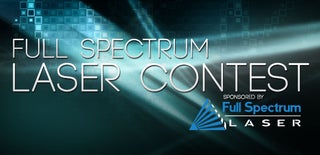
Participated in the
Full Spectrum Laser Contest
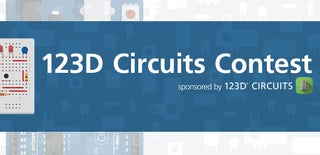
Participated in the
123D Circuits Contest
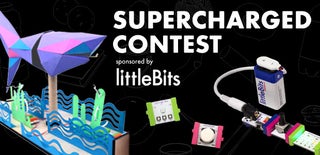
Participated in the
Supercharged Contest