Introduction: How to Build a Car Crash Warning System
Well this is my first instructable. And the objective of this project is to build a car warning system based on the real car crash warning system using Arduino, sensors and some other stuffs.There are many ways to build this project and you can modify the code as your purpose. Don't worry about the code or the connections because it is not that hard. Actually it is pretty simple. Now let us get down to business.
Step 1: Things You Will Need:
- Arduino Uno
- LCD display (white letters on blue display will look cool)
- Ultrasonic sensor(I'm using HC - SR04, works well !)
- Jumper wires (male to male)
- Breadboard
- Piezo buzzer
- 10k resistor
- potensiometer
Step 2: Connect the Things
Now I will tell you briefly how an ultrasonic sensor (if it sounds boring please fell free to skip to the next paragraph). the trig pin sends an ultrasonic sound to an obstacle. When the sound hits an objects it reflects the sound back and it falls on the eco pin. Then the sensor measures the amount of time take for the sounds to come back. then you can convert it into centimeters or inches using the code.
What is the use of a phone without a battery ? We do not want that right ? So let us set the things. And be ready for a bumpy ride especially while connecting the LCD. We have many things to take care of while connecting different components. A small mistake will ruin the project. now let me let about the connection. This project will use about 9 digital pins of Arduino. The pins of LCD are connected to the digital pins 12, 11, 5, 3, 2. the buzzer is connected to the digital pin 8. the ultrasonic sensor has two pin - trig pin and Eco pin. I'm using HC-SR04 as the ultrasonic sensor as it is very cheap and accurate. You can also use other models of ultrasonic sensors such as the parallax pin))) which is very accurate but a bit expensive, the trig pin is connected to the digital pin 7 and the eco pin is connected to the digital pin 6.sounds hard ? The diagram in the top will clear all your doubts. The top picture shows how my project looks after connecting it.
Step 3: The Code for Your Car
Now this is the big boys game. lets begin, shall we ?
I have tried my best to explain the code to you. well what this code does is that when the ultrasonic sensor detects an objects less than or equal to 20 inch it turn the buzzer on and also displays the distance in inch. well this is almost like the real car crash warning system.
If you want to change the minimum distance all you need is to change the code "if (distance <=20)" to your required distance. I have used the LCD.display() and LCD.nodisplay() function to turn the lcd display on and off. and I also want to give you a tip NEVER COPY PASTE A CODE because it is Important that you make mistakes while writing a code. It helps you to lean it much easily.
Now lets go for the code. You might see it as a long code, but once you read it will be just a piece of cake !
#include <LiquidCrystal.h>
LiquidCrystal LCD(12, 11, 5, 4, 3, 2); //Create Liquid Crystal Object called LCD
int trigPin=7; //Sensor Trip pin connected to Arduino pin 13
int echoPin=6; //Sensor Echo pin connected to Arduino pin 11
float pingTime; //time for ping to travel from sensor to target and return
float targetDistance; //Distance to Target in inches
float speedOfSound=776.5; //Speed of sound in miles per hour when temp is 77 degrees.
int buzzer = 8; //the positive wire of the buzzer is connected to pin 8
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
pinMode(trigPin, OUTPUT); //declare trig pin as output
pinMode(echoPin, INPUT); //declare echo pin as input
pinMode(buzzer,OUTPUT);//declare buzzer as output
LCD.begin(16,2); //Tell Arduino to start your 16 column 2 row LCD
}
void loop() {
// put your main code here, to run repeatedly:
digitalWrite(trigPin, LOW); //Set trigger pin low
delayMicroseconds(2000); //Let signal settle
digitalWrite(trigPin, HIGH); //Set trigPin high
delayMicroseconds(15); //Delay in high state
digitalWrite(trigPin, LOW); //ping has now been sent
delayMicroseconds(10); //Delay in high state
pingTime = pulseIn(echoPin, HIGH); //pingTime is presented in microceconds
pingTime=pingTime/1000000; //convert pingTime to seconds by dividing by 1000000 (microseconds in a second)
pingTime=pingTime/3600; //convert pingtime to hourse by dividing by 3600 (seconds in an hour)
targetDistance= speedOfSound * pingTime; //This will be in miles, since speed of sound was miles per hour
targetDistance=targetDistance/2; //Remember ping travels to target and back from target, so you must divide by 2 for actual target distance.
targetDistance= targetDistance*63360; //Convert miles to inches by multipling by 63360 (inches per mile)
if (targetDistance <=20) //if the distance of the obstacle is less than or equal to 20 inches
{
LCD.display(); //turn the lcd display on
LCD.setCursor(0,0); //Set cursor to first column of second row
LCD.print("WARNING"); //Print blanks to clear the row
LCD.setCursor(0,1); //Set Cursor again to first column of second row
LCD.print(targetDistance); //Print measured distance
LCD.print("inch"); //Print your units.
digitalWrite(buzzer,HIGH); //let the buzzer high
delay(250); //pause to let things settle
}
else //if the distance of the object is greater than 20 inches.
{
digitalWrite(buzzer,LOW); //set the buzzer low
LCD.noDisplay(); //set the lcd display off
}
}
So as you can see this the code. Now type this code into your Arduino IDE. Verify and upload it. now you can do your own versions of this project as your need. So thank you for reading. And make your imagination grow and develop for projects. And for that Arduino is the best tool.
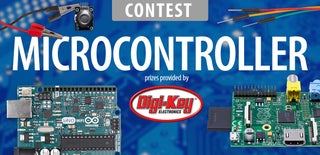
Participated in the
Microcontroller Contest 2017