Introduction: Matlab-based ROS Robotic Controller
Ever since i was a child ,I have always dreamed of being Iron Man and still do so. Iron Man is one of those characters which is realistically possible and simply put I aspire to become Iron Man someday even if people laugh at me or say it's impossible because "it's only impossible until someone does it"-Arnold Schwarzenegger.
ROS is an emerging framework that is used for developing complex robotics systems. It's applications include: Automated Assembly System,Teleoperation,Prosthetic Arms and Heavy Machinery of the industrial sector.
Researchers and engineers leverage ROS for developing the prototypes, while different vendors use it for creating their products.It has a complex architecture that makes it difficult to be managed by a lame man. Using MATLAB for creating the interface link with ROS is a novel approach that can help researchers,engineers and vendors in developing more robust solutions.
So this instructable is about how to make a Matlab-based ROS Robotic Controller, this is going to be one of the very few tutorials on this out there and amongst the few ROS instructables. The goal of this project is to design a controller that can control any ROS-robot connected to your network . So let's get started!
video-editing credits: Ammar Akher, at AmmarAkhter2000@gmail.com
Supplies
Following components are required for the project:
(1) ROS PC/Robot
(2) Router
(3) PC with MATLAB ( version : 2014 or above )
Step 1: Getting Everything Setup
For this instructable, I am using Ubuntu 16.04 for for my linux pc and ros-kinetic, so to avoid confusion i recommend using ros kinetic and ubuntu 16.04 since it has the best support for ros-kinetic. For more information on how to install ros kinetic go to http://wiki.ros.org/kinetic/Installation/Ubuntu . For MATLAB you either purchase a license or download a trail version from here.
Step 2: Understanding How the Controller Works
A pc runs the robotic controller on MATLAB. The controller takes in the IP address and the port of the ros pc/robot.
A ros-topic is used to communicate between the controller and the ros pc/robot, which is also taken as input by the controller. The modem is required to create a LAN ( local area network ) and is what assigns the Ip addresses to all the devices connected to its network. Therefore the ros pc/robot and the pc running the controller must both be connected to the same network (ie. the network of the modem) . So now that you know "how it works", let's get to the "how it's built"...
Step 3: Creating a ROS-MATLAB Interface
The ROS-MATLABInterface is a useful interface for researchers and students for prototyping their robot algorithms in MATLAB and testing it on ROS-compatible robots.This interface can be created by the robotics system toolbox in matlab and we can prototype our algorithm and test it on a ROS-enabled robot or in robot simulators such as Gazebo and V-REP.
To install robotics system toolbox on your MATLAB, simply go to the Add-on option on the toolbar and search for robotic toolbox in the add-on explorer. Using the robotic toolbox we can publish or subscribe to a topic, such as a ROS node, and we can make it a ROS master. The MATLAB-ROS interface has most of the ROS functionalities that you could require for your projects.
Step 4: Getting the IP Address
For the controller to work it is imperative that you know the ip address of your ROS robot/pc and the pc running the controller on MATLAB.
To get your pc's ip:
On Windows:
Open command prompt and type ipconfig command and note down the IPv4 address
For Linux:
Type ifconfig command and note down the inet address. Now that you have the ip address , it's time to build the GUI...
Step 5: Create a GUI for the Controller
To create the GUI, open MATLAB and type guide in the command window. This will open up the guide app though which we will be creating our GUI. You can also use the app designer on MATLAB to design your GUI.
We will be creating 9 buttons in total (as shown in fig):
6 push-buttons: Foward, Backward, Left,Right,Connect to Robot ,Disconnect
3 Editable-buttons: Ros pc ip, port and Topic name.
The Editable-buttons are the buttons which will take ROS pc's ip, it's port and theTopic nameas input. The Topic name is what the MATLAB controller and ROS robot/pc communicate through. To edit the string on the editable button, right-click on the button >> go to Inspector properties >>String and edit the text of the button.
Once your GUI is complete, you can program the buttons. This is where the real fun begins...
Step 6: Programming the GUI Editable Buttons
The GUI is saved as a .fig file but the code/callback functions are saved in .m format.The .m file contains the code for all your buttons.To add call-back functions to your buttons, right-click the button>>View callbacks>>callback. This will open up the .m file for your GUI to where that particular button is defined .
The first callback we are going to code is for the ROS IP editable button. Under function edit1_Callback write the following code:
function edit1_Callback(hObject, eventdata, handles)
global ros_master_ip
ros_master_ip = get(hObject,'String')
Here the function is defined as edit1_Callback,which is referring to the first editable button. When we enter an IP address from the ROS network in this editable button, it will store the IP-address as a string in a global variable called ros_master_ip.
Then just under _OpeningFcn(hObject, eventdata, handles, varargin) define the following(see fig):
global ros_master_ip
global ros_master_port
global teleop_topic_name
ros_master_ip = '192.168.1.102';
ros_master_port = '11311';
teleop_topic_name = '/cmd_vel_mux/input/teleop';
You just globally hard-coded the ros-pc ip (ros_master_ip) , port(ros_master_port) and the Teleop Topic name. What this does is that if you leave the editable buttons empty, these pre-defined values will be used when you connect.
The next callback we are going to code is for the Port editable button.
Under function edit2_Callback write the following code:
function edit2_Callback(hObject, eventdata, handles)
global ros_master_port
ros_master_port = get(hObject,'String')
Here the function is defined as edit2_Callback,which is referring to the second editable button. When we enter the ros pc/robot's port here from the ROS network in this editable button, it will store the port as a string in a global variable called ros_master_port.
Similarly the next callback we are going to code is for the Topic name editable button.
Under function edit3_Callback write the following code:
function edit3_Callback(hObject, eventdata, handles)
global teleop_topic_name
teleop_topic_name = get(hObject,'String')
Similar to ros_master_port, this too is stored as string in a global variable.
Next we are going to look at the call-back functions for the push buttons...
Step 7: Programming the GUI Push Buttons
The push buttons we created previously are the ones we will be using to move, connect and disconnect the robot from the controller. The push button callbacks are defined as follows:
eg. function pushbutton6_Callback(hObject, eventdata, handles)
Note: depending upon the order in which you created your push buttons, they would be numbered accordingly. Therefore function pushbutton6 in my .m file could be for Forward whereas in your .m file it could be for Backwards so do keep that in mind. To know which the exact function for your push button, simply right-click>>View callbacks>>callbacks and it will open up the function for your pushbutton but for this instructable I'm assuming it's the same as mine.
For the Connect to robot button:
Under the function pushbutton6_Callback(hObject, eventdata, handles):
function pushbutton6_Callback(hObject, eventdata, handles)
global ros_master_ip
global ros_master_port
global teleop_topic_name
global robot
global velmsg
ros_master_uri = strcat('http://',ros_master_ip,':',ros_master_port)
setenv('ROS_MASTER_URI',ros_master_uri)
rosinit
robot = rospublisher(teleop_topic_name,'geometry_msgs/Twist');
velmsg = rosmessage(robot);
This callback will set the ROS_MASTER_URI variable by concatenating ros_master_ip and
the port.Then the rosinit command will initialize the connection. After connecting, it will create a publisher of geometry_msgs/Twist, which will be used for sending the command velocity. The topic name is the name that we give in the edit box. Once the connection is successful, we will be able to operate the Forward,Backward, Left,Right push buttons.
Before adding callbacks to the Forward, Backward push buttoins, we need to initialize the speeds of linear and angular velocity.
Therefore below <your .m file name>_OpeningFcn(hObject, eventdata, handles, varargin) define the following (see fig):
global left_spinVelocity
global right_spinVelocity
global forwardVelocity
global backwardVelocity
left_spinVelocity = 2;
right_spinVelocity = -2;
forwardVelocity = 3;
backwardVelocity = -3;
Note: all velocities are in rad/s
Now that the global variables are defined let's program the motion pushbuttons.
For the Forward pushbutton:
function pushbutton4_Callback(hObject, eventdata, handles)
global velmsg
global robot
global teleop_topic_name
global forwardVelocity
velmsg.Angular.Z = 0;
velmsg.Linear.X = forwardVelocity;
send(robot,velmsg);
latchpub = rospublisher(teleop_topic_name, 'IsLatching', true);
Similarly for the Backward pushbutton:
function pushbutton5_Callback(hObject, eventdata, handles)
global velmsg
global robot
global backwardVelocity
global teleop_topic_name
velmsg.Angular.Z = 0;
velmsg.Linear.X = backwardVelocity;
send(robot,velmsg);
latchpub = rospublisher(teleop_topic_name, 'IsLatching', true);
Similarly for the Left pushbutton:
function pushbutton3_Callback(hObject, eventdata, handles)
global velmsg
global robot global left_spinVelocity
global teleop_topic_name
velmsg.Angular.Z = left_spinVelocity;
velmsg.Linear.X = 0;
send(robot,velmsg);
latchpub = rospublisher(teleop_topic_name, 'IsLatching', true);
Similarly for the Right pushbutton:
global velmsg
global robot
global right_spinVelocity
global teleop_topic_name
velmsg.Angular.Z = right_spinVelocity;
velmsg.Linear.X = 0;
send(robot,velmsg);
latchpub = rospublisher(teleop_topic_name, 'IsLatching', true);
Once all the callback functions have been added and the files saved, we can test our controller.
Step 8: Setting Up Network Configuration on ROS PC(Linux)
We will be testing the controller on a ros pc (Linux),which will require setting up the network configuration.If you are also running the controller on a linux pc, you will have to set up the network configuration there as well.
Network Configuration :
Open your terminal window and typegedit .bashrc
Once the file is open add the following :
<em><strong>#Robot Machine Configuration</strong></em>
<em><strong>export ROS_MASTER_URI=http://localhost:11311</strong></em>
<em><strong>#IP address of ROS master node</strong></em>
<em><strong>export ROS_HOSTNAME=</strong></em>
<em><strong>export ROS_IP=</strong></em>
<em><strong>echo "ROS_HOSTNAME: " $ROS_HOSTNAME</strong></em>
<em><strong>echo "ROS_IP:"$ROS_IP</strong></em>
<em><strong>echo "ROS_MASTER_URI:"$ROS_MASTER_URI</strong></em>
You have to follow this step every time due to dynamic IP assignment.
Step 9: Run the Controller
We are going to test our controller on a Turtle bot in Gazebo.
To install Gazebo, please refer to http://gazebosim.org/tutorials?tut=install_ubuntu&cat=install
To install Turtle bot, please refer to https://yainnoware.blogspot.com/2018/09/install-turtlebot-on-ros-kinetic-ubuntu.html
Open the folder where you saved your .fig and .m files on MATLAB and press Run (as shown in picture ).This will open up the controller on the PC. Before pressing connect, make sure your turtle bot simulator is working.
To test your TurtleBot simulation:
Open Terminal on Ros pc and type: $ roslaunch turtlebot_gazebo turtlebot_world.launch.This will open up a simulation of Turtlebot on that PC. The topic name of TurtleBot is /cmd_vel_mux/input/teleop, which we have already provided in the application.Type in the ros pc Ip address , port and topic name in the editable buttons and press the.Connect to Robot button. Your turtle bot should start moving when you press Forward, Backward etc.
To view the linear and angular velocities:
Open a new terminal and type the command: $ rostopic echo /cmd_vel_mux/input/teleop
And there you have, your very own Matlab-based ROS Robotic Controller. If you liked my instructable please give it a vote on the First Time Author Contest and share it with as many people as possible. Thank you.
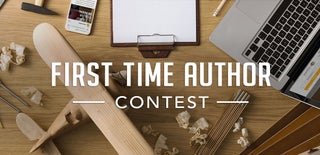
Participated in the
First Time Author Contest