Introduction: Shake & Wake
A lot of couples encounter this problem, they have to wake up at a different time. With a normal alarm clock they both wake up when the alarm goes of. Shake & Wake is the solution for this problem. Using a wireless RF signal the clock sends a signal to the pillow. In the pillow there is a little vibrating motor that wakes you, and only you.
Step 1: What Do We Need
Components:
-A alarm clock (doesn't have to work it's only for the casing)
-2 arduinos
-a potentiometer
-an RTC module
-a switch
-an LCD screen
-2 resistors
-an RF sender and receiver
-a vibration motor
-a thyristor
-a lot of wire
-solder
Tools:
-soldering iron
-pliers
Step 2: Soldering
Solder everything in place like in the scheme. Make sure everything fits inside the alarm clock casing.
Step 3: Coding
First you have to upload the time to the RTC (alarm clock arduino):
#include
"Wire.h"
#define DS1307_I2C_ADDRESS 0x68
byte decToBcd(byte val)
{
return ( (val/10*16) + (val%10) );
}
void setDateDs1307(
byte second, // 0-59
byte minute, // 0-59
byte hour, // 1-23
byte dayOfWeek, // 1-7 1=Mon, 7=Sun
byte dayOfMonth, // 1-28/29/30/31
byte month, // 1-12
byte year // 0-99
)
{
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.write(decToBcd(second));
Wire.write(decToBcd(minute));
Wire.write(decToBcd(hour));
Wire.write(decToBcd(dayOfWeek));
Wire.write(decToBcd(dayOfMonth));
Wire.write(decToBcd(month));
Wire.write(decToBcd(year));
Wire.endTransmission();
}
void setup()
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
Wire.begin();
pinMode(13, OUTPUT);
// Change these values to what you want to set your clock to.
// It is best to add 30 seconds to a minute to allow time for your computer to compile and upload the current time.
// Only run this script once, as running it again will overwrite the time set in the RTC chip!
// Hours are in 24 hour format
// Day of week starts with Monday = 1 up to Sunday = 7
// Year is in YY format, so only use the last 2 digits of the year
//
// Once you have run the program, the LED on pin 13 will flash to say it has finished, DO NOT UNPLUG OR RESET.
// Simply follow the tutorial and upload the LCD code to avoid overwriting the correct time with this time again.
//
second = 0;
minute = 13;
hour = 15;
dayOfWeek = 1;
dayOfMonth = 11;
month = 1;
year = 16;
setDateDs1307(second, minute, hour, dayOfWeek, dayOfMonth, month, year);
//*/
}
void loop()
{
digitalWrite(13, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(13, LOW); // turn the LED off by making the voltage LOW
delay(1000);
}
Then you have to upload the time you want to wake up (alarm clock arduino):
#include "Wire.h"
#include
#include
#define DS1307_I2C_ADDRESS 0x68
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
byte bcdToDec(byte val)
{
return ( (val/16*10) + (val%16) );
}
void getDateDs1307(byte *second,byte *minute,byte *hour,byte *dayOfWeek,byte *dayOfMonth,byte *month,byte *year)
{
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.endTransmission();
Wire.requestFrom(DS1307_I2C_ADDRESS, 7);
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f);
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
void setup()
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
Wire.begin();
// AMEND IF YOUR USING A DIFFERENT LCD SCREEN //
lcd.begin(16, 2);
pinMode(7, OUTPUT);
}
void loop()
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
String s, m, d, mth, h;
getDateDs1307(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month, &year);
if (second < 10) { s = "0" + String(second); } else { s = String(second); }
if (minute < 10) { m = "0" + String(minute); } else { m = String(minute); }
h = String(hour);
if (dayOfMonth < 10) { d = "0" + String(dayOfMonth); } else { d = String(dayOfMonth); }
if (month < 10) { mth = "0" + String(month); } else { mth = String(month); }
char* days[] = { "NA", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun" };
lcd.clear();
// JUMP TO CENTER ON A 16X2 SCREEN //
lcd.setCursor(4,0);
// CHANGE THE FOLLOWING TO SET THE DATE IN TO YOUR PREFERED ORDER //
lcd.print(h + ":" + m + ":" + s);
// NEXT LINE, 1 SPACE IN FROM THE LEFT //
lcd.setCursor(1,1);
// PREFIX THE 20 AS THE RTC CHIP ONLY USES 2 DIGITS FOR THE YEAR //
lcd.print(String(days[dayOfWeek]) + " " + d + "/" + mth + "/20" + year);
delay(1000); // Wait 1 second
if (hour == 15 && minute == 18) digitalWrite(7, HIGH);
if (minute != 18) digitalWrite(7, LOW);
}
The last step is to upload the code to the receiver (pilow arduino):
#define rfReceivePin A0 //RF Receiver pin = Analog pin 0
#define ledPin 13 //Onboard LED = digital pin 13
unsigned int data = 0; // variable used to store received data
const unsigned int upperThreshold = 70; //upper threshold value
const unsigned int lowerThreshold = 50; //lower threshold value
void setup(){
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop(){
data=analogRead(rfReceivePin); //listen for data on Analog pin 0
if(data>upperThreshold){
digitalWrite(ledPin, LOW); //If a LOW signal is received, turn LED OFF
Serial.println(data);
}
if(data
digitalWrite(ledPin, HIGH); //If a HIGH signal is received, turn LED ON
Serial.println(data);
}
}
Step 4: Install the Alarm Clock
If everything is working you can place the alarm clock next to your bed, make sure everything stays charged. You could also make a charging station with magnetic contacts.
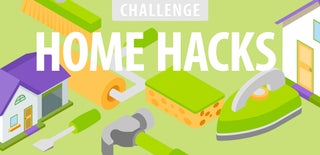
Participated in the
Home Hacks Challenge