Introduction: Self Made Smart Home With Amazon Alexa
From lights and plugs to thermostats and cameras, Alexa can help make your home smarter and more automated by simplifying your everyday routines.
Combined with Node-RED on your Raspberry Pi, you can easily set up a smart home, compatible with Amazon Alexa.
You can create delightful experiences with lights, switches, thermostats, cameras, door locks, and many more device types in a few minutes.
Supplies
For your Smart Home you need the following equipment:
- Raspberry Pi
- Microcontroller MEGA 2560
- MicroSD Card with Raspbian
- Amazon Echo with Portable Speaker
- Sensor Pack
- LED Stripe
- Computer Fan
- Ethernet Cable or WiFi Dongle (Pi 3 has WiFi inbuilt)
- USB A/B Cable
- Jumper Cables
- Breadboard
- Power Adapter
- Alexa App
Recommended:
Step 1: Upload the Code to the Microcontroller
If you want to turn your LEDs on and off, you need to upload some code to the microcontroller.
First of all you have to install Arduino IDE, a tool for programming microcontrollers, on your computer. After installing, start the app.
To upload code, you need to connect the microcontroller to your computer and install the drivers. (In Windows this should work automatically)
I decided to use the ATmega 2560 microcontroller, because it is the best one for beginners. You have a lot of opportunities for programs with this model.
Configure the IDE by clicking on Tools > Board and select "Arduino/Genuino Mega or Mega 2560".
Next, select the port of your microcontroller, by navigating to Tools > Port and select a COM Port. Usually there should be at least one, if you have connected your microcontroller. Select the COMX Port (X is a number).
Copy or download the following code into the editor:
#define RED 49 #define GREEN 53 #define BLUE 51 int input = 0; int states[3] = {0, 0, 0}; int colors[3] = {RED, GREEN, BLUE}; void setup() { Serial.begin(9600); pinMode(RED, OUTPUT); pinMode(GREEN, OUTPUT); pinMode(BLUE, OUTPUT); resetLEDs(); } void loop() { if(Serial.available() > 0) { input = Serial.read(); if (input == 'n') { digitalWrite(RED, states[0]); digitalWrite(GREEN, states[1]); digitalWrite(BLUE, states[2]); } else if (input == 'f') { resetLEDs(); } else if (input == '1') { states[0] = 0; states[1] = 1; states[2] = 1; setLEDs(); } else if (input == '2') { states[0] = 1; states[1] = 0; states[2] = 1; setLEDs(); } else if (input == '3') { states[0] = 1; states[1] = 1; states[2] = 0; setLEDs(); } else if (input == '4') { states[0] = 0; states[1] = 0; states[2] = 0; setLEDs(); } else if(input == '0') { resetLEDs(); } } } void setLEDs() { for(int i = 0; i < 3; i++) { digitalWrite(colors[i], states[i]); } } void resetLEDs() { digitalWrite(RED, 1); digitalWrite(GREEN, 1); digitalWrite(BLUE, 1); }
Then upload the code by clicking on the upload button (button with an arrow pointing to the right).
Wait until the code has been uploaded and disconnect the microcontroller from your computer.
Attachments
Step 2: Construction
Set up the Raspberry Pi with a fresh installation of Raspbian. How to do that? Here is some help.
To make the smart home work, you need to connect the sensor, the fan, and the microcontroller to the Raspberry Pi. The LEDs need to be connected to the microcontroller.
Instead of the fan I used a red LED, to keep it simple on the picture.
The microcontroller needs to be connected to the Raspberry Pi via USB.
Build the components together, like they are displayed on the wiring picture.
Unfortunatelly I could not display LED Strips on the image, so I used those green small LEDs, you can still connect them as displayed.
Step 3: Raspberry Pi and Node-RED Setup
Install Packages
Check for updates first:
sudo apt-get update
Set up Python
To get the temperature and the humidity you need a python app. Python needs some packages first to work.
- Clone the Adafruit Python library
git clone https://github.com/adafruit/Adafruit_Python_DHT.git - Change the directory
cd Adafruit_Python_DHT - Now enter this:
sudo apt-get install build-essential python-dev - Then install the library
sudo python setup.py install
Create a new folder called python in your home directory:
mkdir python
Create a new file called 'getTempHum.py' in this folder
sudo nano getTempHum.py
Paste the following code in the file:
#!/usr/bin/python import sys import Adafruit_DHT humidity, temperature = Adafruit_DHT.read_retry(11, 4) print '{0:0.1f};{1:0.1f}'.format(temperature, humidity)
Set up Node-RED
To run the smart home app, Node-RED needs to be installed. You can find out how to install Node-RED here.
After installing Node-RED, start it by typing 'node-red' into the terminal. Node-RED can now be accessed by its IP and the port 1880. e.g. 10.0.0.11:1880 in the browser.
Always start Node-RED in your home folder!
Open Node-RED in the browser.
- Click on the Menu button in the top right corner
- Click on 'Manage palette'
- Click on the tab 'Install' right next to 'Nodes'
- Type in 'node-red-contrib-alexa-home-skill' in the search bar
- Hit enter
- Click on install at the corresponding package
- Wait until the package is downloaded and installed
- Close the 'Manage palette' panel
Attachments
Step 4: Import the Smart Home Flow
Open Node-RED in the browser.
- Click on the Menu on the top right corner
- Click on 'Import'
- Click on 'Clipboard'
- Paste the following JSON-Code into the input field or download and import the file
[{"id":"595e20f0.0af0d","type":"tab","label":"Alexa","disabled":false,"info":""},{"id":"4a719902.29c758","type":"alexa-home","z":"595e20f0.0af0d","conf":"","device":"61344","acknoledge":true,"name":"","topic":"LEDs","x":168.83336639404297,"y":239.00000667572021,"wires":[["89697a3d.4d7e78"]]},{"id":"89697a3d.4d7e78","type":"switch","z":"595e20f0.0af0d","name":"getRequest","property":"command","propertyType":"msg","rules":[{"t":"eq","v":"TurnOnRequest","vt":"str"},{"t":"eq","v":"TurnOffRequest","vt":"str"},{"t":"eq","v":"SetColorRequest","vt":"str"}],"checkall":"true","repair":false,"outputs":3,"x":387.83335369825363,"y":241,"wires":[["c32c7952.6d8c68"],["26b71ece.b226f2"],["b01cd8ad.1b0fd8"]]},{"id":"c32c7952.6d8c68","type":"function","z":"595e20f0.0af0d","name":"setOnCommand","func":"msg = {\n payload: 'n'\n};\nreturn msg;","outputs":1,"noerr":0,"x":669.1666831970215,"y":166.0000057220459,"wires":[["575a6909.2605a8"]]},{"id":"26b71ece.b226f2","type":"function","z":"595e20f0.0af0d","name":"setOffCommand","func":"msg = {\n payload: 'f'\n};\nreturn msg;","outputs":1,"noerr":0,"x":668.1666831970215,"y":241.00004768371582,"wires":[["575a6909.2605a8"]]},{"id":"b01cd8ad.1b0fd8","type":"function","z":"595e20f0.0af0d","name":"setColorCommand","func":"if (msg.payload.hue === 0 && msg.payload.saturation === 1 && msg.payload.brightness === 1) {\n msg = {\n payload: 1\n };\n} else if (msg.payload.hue === 120 && msg.payload.saturation === 1 && msg.payload.brightness === 1) {\n msg = {\n payload: 2\n };\n} else if (msg.payload.hue === 240 && msg.payload.saturation === 1 && msg.payload.brightness === 1) {\n msg = {\n payload: 3\n };\n} else if (msg.payload.hue === 0 && msg.payload.saturation === 0 && msg.payload.brightness === 1) {\n msg = {\n payload: 4\n };\n} else {\n msg = {\n payload: 'e'\n };\n}\nreturn msg;","outputs":1,"noerr":0,"x":677.0000190734863,"y":328.00000953674316,"wires":[["575a6909.2605a8"]]},{"id":"24bb6d4b.1c4d82","type":"alexa-home","z":"595e20f0.0af0d","conf":"","device":"62720","acknoledge":true,"name":"Living Room Thermostat","topic":"Living Room Thermostat","x":203.83335369825363,"y":526,"wires":[["2720a8cc.059fe8"]]},{"id":"fd211531.b27f48","type":"function","z":"595e20f0.0af0d","name":"setThermostatResponse","func":"var data = msg.payload.split(';');\n\nmsg.extra = {\n \"temperatureReading\": {\n \"value\": parseFloat(data[0])\n },\n \"applianceResponseTimestamp\": new Date().toISOString()\n};\nmsg.payload = true;\n\nreturn msg;","outputs":1,"noerr":0,"x":760.8333740234375,"y":526.0000352859497,"wires":[["9b96a4ef.260218"]]},{"id":"9b96a4ef.260218","type":"alexa-home-resp","z":"595e20f0.0af0d","x":1036.8334197998047,"y":529.000036239624,"wires":[]},{"id":"fc24f72f.323928","type":"alexa-home","z":"595e20f0.0af0d","conf":"","device":"62724","acknoledge":true,"name":"Fan","topic":"Fan","x":143.16668510437012,"y":638.0000677108765,"wires":[["2148e5a5.790a1a"]]},{"id":"575a6909.2605a8","type":"serial out","z":"595e20f0.0af0d","name":"Send to Microcontroller","serial":"26959c9e.84a984","x":1015,"y":239,"wires":[]},{"id":"2720a8cc.059fe8","type":"exec","z":"595e20f0.0af0d","command":"python python/getTempHum.py","addpay":false,"append":"","useSpawn":"false","timer":"","oldrc":false,"name":"Measure Temperature","x":475.3333435058594,"y":525.3333129882812,"wires":[["fd211531.b27f48"],[],[]]},{"id":"d669f22d.ad6a9","type":"rpi-gpio out","z":"595e20f0.0af0d","name":"","pin":"11","set":"","level":"0","freq":"","out":"out","x":675.1666831970215,"y":639.0001401901245,"wires":[]},{"id":"2148e5a5.790a1a","type":"function","z":"595e20f0.0af0d","name":"setFanState","func":"if(msg.command === 'TurnOnRequest') {\n msg = {\n payload: 1\n };\n} else if (msg.command === 'TurnOffRequest') {\n msg = {\n payload: 0\n };\n}\nreturn msg;","outputs":1,"noerr":0,"x":392.1666774749756,"y":638.6667642593384,"wires":[["d669f22d.ad6a9"]]},{"id":"26959c9e.84a984","type":"serial-port","z":"","serialport":"/dev/ttyUSB0","serialbaud":"9600","databits":"8","parity":"none","stopbits":"1","waitfor":"","newline":"\\n","bin":"false","out":"char","addchar":"","responsetimeout":"10000"}]
Click on 'Import'.
Attachments
Step 5: Add Devices to Alexa
To turn on the lights, the fan or to ask for the room temperature, you need to register the devices in your Alexa App.
First of all, go to https://alexa-node-red.bm.hardill.me.uk/newuser and create a new account. Then sign in with the just created account on https://alexa-node-red.bm.hardill.me.uk/login .
When you signed in, you are at the devices tab.
Then, open the Alexa App and install the Node-RED Alexa Skill. Sign in with your created account.
How to add devices?
- Open the devices tab of the website
- Click on 'Add device'
- Enter a name and a description and check all checkboxes, you need for your device
- Click on 'OK'
In this example, we have three devices: LEDs, Living Room Thermostat (or Thermostat) and Fan
Here are the options, you need to check, to make the devices work:
LEDs
- Name: LEDs
- Description: LED Strips
- Actions:
- On
- Off
- Application Type:
- LIGHT
Thermostat
- Name: Thermostat
- Description: Thermostat
- Actions:
- Query Current Temp
- Application Type:
- THERMOSTAT
Fan
- Name: Fan
- Description: Fan
- Actions:
- On
- Off
- Application Type:
- SWITCH
Now you have to link your user account to Node-RED. To do that, click on an Alexa Node (LEDs, Living Room Thermostat, or Fan) and click on the edit button, next to the alexa-home-conf input field.
Enter the username and password of your account, you created at the beginning. Then click on 'Add'.
After creating the new alexa-home-conf you should see your username at the account input field.
Click on the refresh button next to the Devices input field. You should now see your previously created devices in the selection list.
Select your account and the corresponding device in every Alexa Node. For LEDs, Thermostat, and Fan.
After that click on the red 'Deploy' button. The code should now be deployed to your Raspberry Pi.
Step 6: Ask Alexa
Ask Alexa to discover devices. Just say: "Alexa, discover devices."
Alexa should now find all your created devices.
To test the devices, try to ask Alexa the following:
- "Alexa, turn on LEDs."
- "Alexa, turn off LEDs."
- "Alexa, turn LEDs to blue."
- "Alexa, turn LEDs to red."
- "Alexa, whats the temperature of Thermostat?"
- "Alexa, turn on the Fan."
- "Alexa, turn off the Fan."
-
That's it! Your Alexa Smart Home is ready for you.
You can now ask Alexa, to turn on the LEDs, change the color, get the current room temperature and many more things. If you want, you can expand this tutorial and add your own devices. You could try using a door lock for example. If you want, you can share it with me!
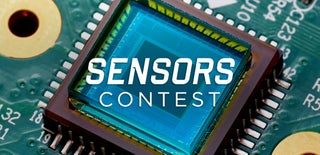
Participated in the
Sensors Contest