Introduction: Self Made Smart Home With Apple HomeKit
With the Home app, you can easily and securely control all your HomeKit accessories. Ask Siri to turn off the lights from your iPhone, check the temperature on your iPad, and get notified if somebody is moving around in your House.
Together with Node-RED on your Raspberry Pi, you can set up your smart home, compatible with Apple HomeKit, easily.
Supplies
For your Smart Home you need the following equipment:
- Raspberry Pi
- Microcontroller MEGA 2560
- MicroSD Card with Raspbian
- Sensor Pack (or Motion Sensor, Fire Sensor and Temperature Sensor)
- LED Stripe
- Ethernet Cable or WiFi Dongle (Pi 3 has WiFi inbuilt)
- USB A/B Cable
- Jumper Cables
- Breadboard
- Power Adapter
- Apple iPhone, iPad or Mac
Recommended:
Step 1: Upload the Code to the Microcontroller
If you want to turn your LEDs on and off, you need to upload some code to the microcontroller.
First of all you have to install Arduino IDE, a tool for programming microcontrollers, on your computer. After installing, start the app.
To upload code, you need to connect the microcontroller to your computer and install the drivers. (In Windows this should work automatically)
I decided to use the ATmega 2560 microcontroller, because it is the best one for beginners. You have a lot of opportunities for programs with this model.
Configure the IDE by clicking on Tools > Board and select "Arduino/Genuino Mega or Mega 2560".
Next, select the port of your microcontroller, by navigating to Tools > Port and select a COM Port. Usually there should be at least one, if you have connected your microcontroller. Select the COMX Port (X is a number).
Copy or download the following code into the editor:
#define RED 49
#define GREEN 53
#define BLUE 51
int input = 0;
void setup() {
Serial.begin(9600);
pinMode(RED, OUTPUT);
pinMode(GREEN, OUTPUT);
pinMode(BLUE, OUTPUT);
resetLEDs();
}
void loop() {
if(Serial.available() > 0) {
input = Serial.read();
if (input == '0') {
resetLEDs();
} else if (input == '1') {
digitalWrite(RED, 0);
digitalWrite(GREEN, 1);
digitalWrite(BLUE, 1);
} else if (input == '2') {
digitalWrite(RED, 1);
digitalWrite(GREEN, 0);
digitalWrite(BLUE, 1);
} else if (input == '3') {
digitalWrite(RED, 1);
digitalWrite(GREEN, 1);
digitalWrite(BLUE, 0);
} else if (input == '4') {
digitalWrite(RED, 0);
digitalWrite(GREEN, 0);
digitalWrite(BLUE, 0);
}
}
}
void resetLEDs() {
digitalWrite(RED, 1);
digitalWrite(GREEN, 1);
digitalWrite(BLUE, 1);
}
Then upload the code by clicking on the upload button (button with an arrow pointing to the right).
Wait until the code has been uploaded and disconnect the microcontroller from your computer.
Attachments
Step 2: Construction
Set up the Raspberry Pi with a fresh installation of Raspbian. How to do that? Here is some help.
To make the smart home work, you need to connect the sensors, and the microcontroller to the Raspberry Pi. The LEDs need to be connected to the microcontroller.
The microcontroller needs to be connected to the Raspberry Pi via USB.
Build the components together, like they are displayed on the wiring picture.
Unfortunatelly I could not display LED Strips on the image, so I used those green small LEDs, you can still connect them as displayed.
Step 3: Raspberry Pi and Node-RED Setup
Install Packages
Check for updates first:
sudo apt-get update
Install this packages:
sudo apt-get install libavahi-compat-libdnssd-dev
Set up Python
To get the temperature and the humidity you need a python app. Python needs some packages first to work.
- Clone the Adafruit Python library
git clone https://github.com/adafruit/Adafruit_Python_DHT.git - Change the directory
cd Adafruit_Python_DHT - Now enter this:
sudo apt-get install build-essential python-dev - Then install the library
sudo python setup.py install
Create a new folder called python in your home directory:
mkdir python
Create a new file called 'getTempHum.py' in this folder
sudo nano getTempHum.py
Paste the following code in the file:
#!/usr/bin/python
import sys
import Adafruit_DHT
humidity, temperature = Adafruit_DHT.read_retry(11, 4)
print '{0:0.1f};{1:0.1f}'.format(temperature, humidity)
Set up Node-RED
To run the smart home app, Node-RED needs to be installed. You can find out how to install Node-RED here.
After installing Node-RED, start it by typing 'node-red' into the terminal. Node-RED can now be accessed by its IP and the port 1880. e.g. 10.0.0.11:1880 in the browser.
Always start Node-RED in your home folder!
Open Node-RED in the browser.
- Click on the Menu button in the top right corner
- Click on 'Manage palette'
- Click on the tab 'Install' right next to 'Nodes'
- Type in 'node-red-contrib-homekit' in the search bar
- Hit enter
- Click on install at the corresponding package
- Wait until the package is downloaded and installed
- Close the 'Manage palette' panel
Attachments
Step 4: Import the Smart Home Flow
Open Node-RED in the browser.
- Click on the Menu on the top right corner
- Click on 'Import'
- Click on 'Clipboard'
- Paste the following JSON-Code into the input field or download and import the file
<p>[{"id":"f82b01a9.ad217","type":"tab","label":"Smart Home","disabled":false,"info":""},{"id":"f896553f.039a58","type":"exec","z":"f82b01a9.ad217","command":"python python/getTempHum.py","addpay":false,"append":"","useSpawn":"false","timer":"","oldrc":false,"name":"Measure Temperature","x":388,"y":196,"wires":[["7cb6ec2.3523714","2f2f5183.c1c7ee"],[],[]]},{"id":"7cb6ec2.3523714","type":"function","z":"f82b01a9.ad217","name":"set Temperature Characteristics","func":"var data = msg.payload.split(';');\n\nmsg.payload={\n CurrentTemperature: parseFloat(data[0])\n}\nreturn msg;","outputs":1,"noerr":0,"x":678.0000152587891,"y":82.0000057220459,"wires":[["3745371d.c56a98"]]},{"id":"bef05283.9b3d5","type":"inject","z":"f82b01a9.ad217","name":"","topic":"","payload":"","payloadType":"date","repeat":"30","crontab":"","once":true,"onceDelay":0.1,"x":183.16667556762695,"y":169.00000524520874,"wires":[["f896553f.039a58"]]},{"id":"3745371d.c56a98","type":"homekit-service","z":"f82b01a9.ad217","accessory":"750b4ff0.a74dc","name":"Temperature","serviceName":"TemperatureSensor","x":923.1667728424072,"y":80.66669273376465,"wires":[[]]},{"id":"df4a14ae.1f21a8","type":"serial out","z":"f82b01a9.ad217","name":"Send to Microcontroller","serial":"26959c9e.84a984","x":554.0000534057617,"y":350.0000514984131,"wires":[]},{"id":"49dad744.6126a8","type":"homekit-service","z":"f82b01a9.ad217","accessory":"1ad72034.b39e","name":"LEDs","serviceName":"Switch","x":159.00000381469727,"y":352.00002574920654,"wires":[["a78d8a62.927818"]]},{"id":"a78d8a62.927818","type":"function","z":"f82b01a9.ad217","name":"set LEDs","func":"if(msg.payload.On === true) {\n msg = {\n payload: 4\n };\n} else {\n msg = {\n payload: 0\n };\n}\nreturn msg;","outputs":1,"noerr":0,"x":313.0000114440918,"y":351.0001096725464,"wires":[["df4a14ae.1f21a8"]]},{"id":"2f2f5183.c1c7ee","type":"function","z":"f82b01a9.ad217","name":"set Humidity Characteristics","func":"var data = msg.payload.split(';');\n\nmsg.payload={\n CurrentRelativeHumidity: parseFloat(data[1])\n}\nreturn msg;","outputs":1,"noerr":0,"x":666.0000152587891,"y":126.00000762939453,"wires":[["bfd0a26f.24ab"]]},{"id":"bfd0a26f.24ab","type":"homekit-service","z":"f82b01a9.ad217","accessory":"a0611849.850d48","name":"Humidity","serviceName":"HumiditySensor","x":917.0000267028809,"y":127.00000762939453,"wires":[[]]},{"id":"46202059.d6439","type":"homekit-service","z":"f82b01a9.ad217","accessory":"1a680f91.1b1b8","name":"Motion","serviceName":"MotionSensor","x":624.1000061035156,"y":540.8000316619873,"wires":[[]]},{"id":"c8423cb6.71657","type":"rpi-gpio in","z":"f82b01a9.ad217","name":"Motion","pin":"11","intype":"tri","debounce":"25","read":false,"x":129.10000610351562,"y":539.8000326156616,"wires":[["32208579.539c9a"]]},{"id":"32208579.539c9a","type":"function","z":"f82b01a9.ad217","name":"set Motion Detected","func":"if(msg.payload === 1) {\n msg.payload = {\n MotionDetected: 1\n }\n}\nreturn msg;","outputs":1,"noerr":0,"x":423.1000061035156,"y":541.2000122070312,"wires":[["46202059.d6439"]]},{"id":"19ab65c8.7996fa","type":"rpi-gpio in","z":"f82b01a9.ad217","name":"Fire","pin":"13","intype":"tri","debounce":"25","read":false,"x":129.1000099182129,"y":638.0000190734863,"wires":[["894eb946.0149b8"]]},{"id":"894eb946.0149b8","type":"function","z":"f82b01a9.ad217","name":"set Smoke Detected","func":"if(msg.payload === 0) {\n msg.payload = {\n SmokeDetected: 1\n }\n}\nreturn msg;","outputs":1,"noerr":0,"x":403,"y":634.7999877929688,"wires":[["bb1a1a58.4402e8"]]},{"id":"bb1a1a58.4402e8","type":"homekit-service","z":"f82b01a9.ad217","accessory":"4f079dd7.d86094","name":"Fire Detector","serviceName":"SmokeSensor","x":703.1000366210938,"y":635,"wires":[[]]},{"id":"750b4ff0.a74dc","type":"homekit-accessory","z":"","accessoryName":"Temperature","pinCode":"111-11-113","port":"","manufacturer":"Raspberry","model":"Pi 3B+","serialNo":"123","accessoryType":"10"},{"id":"26959c9e.84a984","type":"serial-port","z":"","serialport":"/dev/ttyUSB0","serialbaud":"9600","databits":"8","parity":"none","stopbits":"1","waitfor":"","newline":"\\n","bin":"false","out":"char","addchar":"","responsetimeout":"10000"},{"id":"1ad72034.b39e","type":"homekit-accessory","z":"","accessoryName":"LEDs","pinCode":"222-22-222","port":"","manufacturer":"Default Manufacturer","model":"Default Model","serialNo":"Default Serial Number","accessoryType":"8"},{"id":"a0611849.850d48","type":"homekit-accessory","z":"","accessoryName":"Humidity","pinCode":"333-33-333","port":"","manufacturer":"Default Manufacturer","model":"Default Model","serialNo":"Default Serial Number","accessoryType":"10"},{"id":"1a680f91.1b1b8","type":"homekit-accessory","z":"","accessoryName":"Motion","pinCode":"444-44-444","port":"","manufacturer":"Default Manufacturer","model":"Default Model","serialNo":"Default Serial Number","accessoryType":"10"},{"id":"4f079dd7.d86094","type":"homekit-accessory","z":"","accessoryName":"Fire Detector","pinCode":"555-55-555","port":"","manufacturer":"Default Manufacturer","model":"Default Model","serialNo":"Default Serial Number","accessoryType":"10"}]</p>
Click on 'Import'.
After importing click on the red 'Deploy' button. The code should now be deployed to your Raspberry Pi
Attachments
Step 5: Add the Devices to Your Apple Home App
To add all your devices to Apple Home, open the Home app first.
If you haven't already set up the Home App, read the introduction first and follow the steps to set up the app.
- Click on 'Add Device' or the Plus symbol on the top right corner to add an accessory. Now the camera opens.
- Click on 'Don't Have a Code or Can't Scan?'
- Select an accessory.
- If a popup comes up click on 'Add anyway'
- Type in the code for the corresponding accessory (The list of codes is below)
- Add them to a room or let them be in the default room
- Follow the remaining instructions
List of codes for devices:
LEDs: 222-22-222
Temperature Sensor: 111-11-113
Humidity Sensor: 333-33-333
Motion Sensor: 444-44-444
Fire Detector: 555-55-555
-
Congratulations! You have made yourself a pretty impressive Smart Home!
You can now control the LEDs over your iPhone or iPad and get notified, if fire or motion is detected.
You can also ask Siri to turn the LEDs on and off or what the current temperature is.
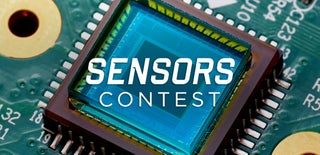
Participated in the
Sensors Contest