Introduction: Candypult--Computer Controlled Candy Catapult
Step 1:
Parts:
Arduino
(2) Hitec HS-311 servo motors (Amazon.com)
(1) Hitec HS-645MG servo motor (high torque)--Amazon.com
(1) mini Servo YKS SG90 (Amazon.com)
(1) Two channel remote control toy--I got a car for $11.99 at a local pharmacy
(3) 5 volt relays--Jameco.com #139977 (these are polarized so that positive voltage on one coil lead causes it to pick up, but the other polarity doesn't; you can produce the same result with a 5 volt relay and a diode. Relays "B" and "C" only operate one at a time--based on the polarity provided.)
1/4" x 3 1/4" x .025 spring (available at Lowes)
(6) 12" servo extension cables
Spring from a ballpoint pen
4" lazy susan http://www.amazon.com/Capacity-Bearing-Turntable-Bearings-VXB/dp/B002TIKEQ6/ref=sr_1_2?ie=UTF8&qid=1372692771&sr=8-2&keywords=lazy+susan+4+inch
9 volt, 500 ma DC power supply
6 volt, 1 amp DC power supply
3D printed parts, design and print files at:
http://www.thingiverse.com/thing:110350
Wood, screws,paint, wire, jumpers
Step 2:
Take a 4" x 15" x 1/2 inch piece of plywood and paint it orange. Diagonally wrap painters tape to make the stripes.
Step 3:
Step 4:
Step 5:
Step 6:
Step 7:
Step 8:
Step 9:
Step 10:
Step 11:
Step 12:
Step 13:
Step 14:
Step 15:
Step 16:
Step 17:
Step 18:
Step 19:
Step 20:
Super glue the servo motor holder to the candy tower.
Step 21:
Step 22:
Step 23:
Step 24:
Step 25:
Step 26:
Step 27:
Step 28:
Step 29:
Note the use of servo extension cords and a wire guide to protect the wires that move through the rotation process.
Step 30:
Software:
#include <Servo.h>
Servo candy;
Servo rotate;
Servo latch;
Servo spring;
int fire=2;
int right=4;
int left=6;
int val=0;
int val1=0;
int val2=0;
int val3=0;
void setup()
{pinMode(fire,INPUT);
pinMode(right,INPUT);
pinMode(left,INPUT);
candy.attach(13);
rotate.attach(12);
latch.attach(8);
spring.attach(7);
rotate.write(45);
delay(500);
rotate.write(30);
latch.write(60);
spring.write(200);
candy.write(25);
candy.write(140);
delay(500);
candy.write(25);
}
void loop()
{
val=digitalRead(left);
val1=digitalRead(right);
val2=digitalRead(fire);
if(val==HIGH or val1==HIGH or val2==HIGH)
{
if (val==HIGH)
{val3=(val3+5);}
if (val3>=145)
{
val3=(val3-5);
}
if (val3<65)
{
val3=65;
}
rotate.write(val3);
delay(50);
}
else
val=digitalRead(left);
val1=digitalRead(right);
val2=digitalRead(fire);
if(val1==HIGH)
{val3=(val3-5);
if(val3<65)
{val3=(val3+5);
}
rotate.write(val3);
delay(50);
}
else
if(val2==HIGH)
{spring.write(50);
delay(1000);
latch.write(200);
delay(1000);
spring.write(200);
delay(500);
latch.write(60);
delay(1000);
rotate.write(50);
delay(500);
rotate.write(30);
candy.write(140);
delay(500);
candy.write(25);
val3=0;
}}
Step 31: EXTRA CREDIT!
This is about 60% reliable, so more work needs to be done (probably in shielding the microphones).
The concept is this--when a loud sound arrives, it will probably be louder at the microphone pointed in the direction of the sound.
Step 32:
Microphones are Jameco #320179
Step 33:
Step 34:
In a room without a lot of people and clutter, I can achieve very high accuracy; but I think the machine trained me instead of the other way around.
Software:
#include <Servo.h>
Servo candy;
Servo rotate;
Servo latch;
Servo spring;
int led2=3;
int val2=0;
int ledcenter=6;
int ledPin=5;
int valm=0;
int val1=0;
void setup()
{pinMode(ledPin, OUTPUT);
pinMode(led2,OUTPUT);
pinMode(ledcenter,OUTPUT);
candy.attach(13);
rotate.attach(12);
latch.attach(8);
spring.attach(7);
rotate.write(45);
delay(500);
rotate.write(30);
latch.write(60);
spring.write(200);
candy.write(25);
candy.write(140);
delay(500);
candy.write(25);
}
void loop()
{
valm=analogRead(0);
val2=analogRead(3);
val1=analogRead(1);
if(valm>400 || val1>400 || val2>400)
//val1=val1-60;
{if(valm>val2 && valm>val1)
{digitalWrite(ledPin, HIGH);
rotate.write(130);
delay(500);
rotate.write(145);
spring.write(50);
delay(1000);
latch.write(200);
delay(1000);
spring.write(200);
delay(500);
latch.write(60);
delay(1000);
rotate.write(50);
delay(500);
rotate.write(30);
candy.write(140);
delay(500);
candy.write(25);
digitalWrite(ledPin, LOW);
delay(1000);
valm=0;
val2=0;
val1=0;
}
else
{
if(val2>valm && val2>val1)
{digitalWrite(led2,HIGH);
rotate.write(75);
spring.write(50);
delay(1000);
latch.write(200);
delay(1000);
spring.write(200);
delay(500);
latch.write(60);
delay(1000);
rotate.write(40);
delay(500);
rotate.write(30);
candy.write(140);
delay(500);
candy.write(25);
digitalWrite(led2,LOW);
delay(1000);
valm=0;
val2=0;
val1=0;
}
else
{if(val1>valm && val1>val2)
{digitalWrite(ledcenter,HIGH);
rotate.write(110);
spring.write(50);
delay(1000);
latch.write(200);
delay(1000);
spring.write(200);
delay(500);
latch.write(60);
delay(1000);
rotate.write(40);
delay(500);
rotate.write(30);
candy.write(140);
delay(500);
candy.write(25);
digitalWrite(ledcenter,LOW);
delay(1000);
valm=0;
val2=0;
val1=0;
}}}}}
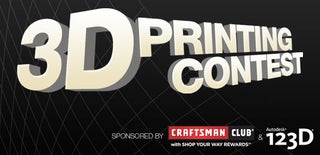
Participated in the
3D Printing Contest
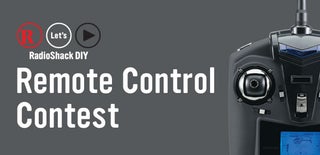
Participated in the
Remote Control Contest
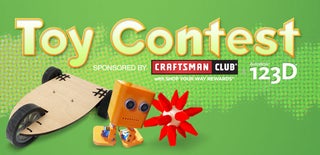
Participated in the
Toy Contest